Fixed point math
[Math]
Defines | |
#define | FIX_SHIFT 8 |
#define | FIX_SCALE ( 1<<FIX_SHIFT ) |
#define | FIX_MASK ( FIX_SCALE-1 ) |
#define | FIX_SCALEF ( (float)FIX_SCALE ) |
#define | FIX_SCALEF_INV ( 1.0/FIX_SCALEF ) |
#define | FIX_ONE FIX_SCALE |
#define | FX_RECIPROCAL(a, fp) ( ((1<<(fp))+(a)-1)/(a) ) |
Get the fixed point reciprocal of a, in fp fractional bits. | |
#define | FX_RECIMUL(x, a, fp) ( ((x)*((1<<(fp))+(a)-1)/(a))>>(fp) ) |
Perform the division x/ a by reciprocal multiplication. | |
Functions | |
INLINE FIXED | int2fx (int d) |
Convert an integer to fixed-point. | |
INLINE FIXED | float2fx (float f) |
Convert a float to fixed-point. | |
INLINE u32 | fx2uint (FIXED fx) |
Convert a FIXED point value to an unsigned integer (orly?). | |
INLINE u32 | fx2ufrac (FIXED fx) |
Get the unsigned fractional part of a fixed point value (orly?). | |
INLINE int | fx2int (FIXED fx) |
Convert a FIXED point value to an signed integer. | |
INLINE float | fx2float (FIXED fx) |
Convert a fixed point value to floating point. | |
INLINE FIXED | fxadd (FIXED fa, FIXED fb) |
Add two fixed point values. | |
INLINE FIXED | fxsub (FIXED fa, FIXED fb) |
Subtract two fixed point values. | |
INLINE FIXED | fxmul (FIXED fa, FIXED fb) |
Multiply two fixed point values. | |
INLINE FIXED | fxdiv (FIXED fa, FIXED fb) |
Divide two fixed point values. | |
INLINE FIXED | fxmul64 (FIXED fa, FIXED fb) |
Multiply two fixed point values using 64bit math. | |
INLINE FIXED | fxdiv64 (FIXED fa, FIXED fb) |
Divide two fixed point values using 64bit math. |
Detailed Description
Define Documentation
#define FIX_SHIFT 8 |
#define FX_RECIMUL | ( | x, | |||
a, | |||||
fp | ) | ( ((x)*((1<<(fp))+(a)-1)/(a))>>(fp) ) |
Perform the division x/ a by reciprocal multiplication.
Division is slow, but you can approximate division by a constant by multiplying with its reciprocal: x/a vs x*(1/a). This routine gives the reciprocal of a as a fixed point number with fp fractional bits.
- Parameters:
-
a Value to take the reciprocal of. fp Number of fixed point bits
- Note:
- The routine does do a division, but the compiler will optimize it to a single constant ... if both a and fp are constants!
Rules for safe reciprocal division, using n = 2fp and m = (n+a-1)/a (i.e., rounding up)
- Maximum safe numerator x: x < n/(m*a-n)
- Minimum n for known x: n > x*(a-1)
#define FX_RECIPROCAL | ( | a, | |||
fp | ) | ( ((1<<(fp))+(a)-1)/(a) ) |
Get the fixed point reciprocal of a, in fp fractional bits.
- Parameters:
-
a Value to take the reciprocal of. fp Number of fixed point bits
- Note:
- The routine does do a division, but the compiler will optimize it to a single constant ... if both a and fp are constants!
- See also:
- FX_RECIMUL
Generated on Mon Aug 25 17:03:57 2008 for libtonc by
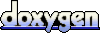