tonc_tte.h File Reference
#include <stdio.h>
#include "tonc_memmap.h"
#include "tonc_surface.h"
Data Structures | |
struct | TFont |
Font description struct. More... | |
struct | TTC |
TTE context struct. More... | |
Defines | |
#define | TTE_TAB_WIDTH 24 |
#define | tte_printf iprintf |
Color lut indices | |
#define | TTE_INK 0 |
#define | TTE_SHADOW 1 |
#define | TTE_PAPER 2 |
#define | TTE_SPECIAL 3 |
drawg helper macros | |
#define | TTE_BASE_VARS(_tc, _font) |
Declare and define base drawg variables. | |
#define | TTE_CHAR_VARS(font, gid, src_t, _sD, _sL, _chW, _chH) |
Declare and define basic source drawg variables. | |
#define | TTE_DST_VARS(tc, dst_t, _dD, _dL, _dP, _x, _y) |
Declare and define basic destination drawg variables. | |
Default fonts | |
#define | fwf_default sys8Font |
Default fixed-width font. | |
#define | vwf_default verdana9Font |
Default vairable-width font. | |
Default glyph renderers | |
#define | ase_drawg_default ((fnDrawg)ase_drawg_s) |
#define | bmp8_drawg_default ((fnDrawg)bmp8_drawg_b1cts) |
#define | bmp16_drawg_default ((fnDrawg)bmp16_drawg_b1cts) |
#define | chr4c_drawg_default ((fnDrawg)chr4c_drawg_b1cts) |
#define | chr4r_drawg_default ((fnDrawg)chr4r_drawg_b1cts) |
#define | obj_drawg_default ((fnDrawg)obj_drawg) |
#define | se_drawg_default ((fnDrawg)se_drawg_s) |
Default initializers | |
#define | tte_init_se_default(bgnr, bgcnt) tte_init_se( bgnr, bgcnt, 0xF000, CLR_YELLOW, 0, &fwf_default, NULL) |
#define | tte_init_ase_default(bgnr, bgcnt) tte_init_ase(bgnr, bgcnt, 0x0000, CLR_YELLOW, 0, &fwf_default, NULL) |
#define | tte_init_chr4c_default(bgnr, bgcnt) |
#define | tte_init_chr4r_default(bgnr, bgcnt) |
#define | tte_init_chr4c_b4_default(bgnr, bgcnt) |
#define | tte_init_bmp_default(mode) tte_init_bmp(mode, &vwf_default, NULL) |
#define | tte_init_obj_default(pObj) tte_init_obj(pObj, 0, 0, 0xF000, CLR_YELLOW, 0, &fwf_default, NULL) |
Typedefs | |
typedef void(* | fnDrawg )(uint gid) |
Glyph render function format. | |
typedef void(* | fnErase )(int left, int top, int right, int bottom) |
Erase rectangle function format. | |
Functions | |
void | tte_set_context (TTC *tc) |
Set the master context pointer. | |
INLINE TTC * | tte_get_context (void) |
Get the master text-system. | |
INLINE uint | tte_get_glyph_id (int ch) |
Get the glyph index of character ch. | |
INLINE int | tte_get_glyph_width (uint gid) |
Get the width of glyph id. | |
INLINE int | tte_get_glyph_height (uint gid) |
Get the height of glyph id. | |
INLINE const void * | tte_get_glyph_data (uint gid) |
Get the glyph data of glyph id. | |
void | tte_set_color (eint type, u16 clr) |
Set color attribute of type to cattr. | |
void | tte_set_colors (const u16 colors[]) |
Load important color data. | |
void | tte_set_color_attr (eint type, u16 cattr) |
Set color attribute of type to cattr. | |
void | tte_set_color_attrs (const u16 cattrs[]) |
Load important color attribute data. | |
char * | tte_cmd_default (const char *str) |
Text command handler. | |
int | tte_putc (int ch) |
Plot a single character; does wrapping too. | |
int | tte_write (const char *text) |
Render a string. | |
int | tte_write_ex (int x, int y, const char *text, const u16 *clrlut) |
Extended string writer, with positional and color info. | |
void | tte_erase_rect (int left, int top, int right, int bottom) |
Erase a porttion of the screen (ignores margins). | |
void | tte_erase_screen (void) |
Erase the screen (within the margins). | |
void | tte_erase_line (void) |
Erase the whole line (within the margins). | |
POINT16 | tte_get_text_size (const char *str) |
Get the size taken up by a string. | |
void | tte_init_base (const TFont *font, fnDrawg drawProc, fnErase eraseProc) |
Base initializer of a TTC. | |
INLINE void | tte_get_pos (int *x, int *y) |
Get cursor position. | |
INLINE u16 | tte_get_ink (void) |
Get ink color attribute. | |
INLINE u16 | tte_get_shadow (void) |
Get shadow color attribute. | |
INLINE u16 | tte_get_paper (void) |
Get paper color attribute. | |
INLINE u16 | tte_get_special (void) |
Get special color attribute. | |
INLINE TSurface * | tte_get_surface () |
Get a pointer to the text surface. | |
INLINE TFont * | tte_get_font (void) |
Get the active font. | |
INLINE fnDrawg | tte_get_drawg (void) |
Get the active character plotter. | |
INLINE fnErase | tte_get_erase (void) |
Get the character plotter. | |
INLINE char ** | tte_get_string_table (void) |
Get string table. | |
INLINE TFont ** | tte_get_font_table (void) |
Get font table. | |
INLINE void | tte_set_pos (int x, int y) |
Set cursor position. | |
INLINE void | tte_set_ink (u16 cattr) |
Set ink color attribute. | |
INLINE void | tte_set_shadow (u16 cattr) |
Set shadow color attribute. | |
INLINE void | tte_set_paper (u16 cattr) |
Set paper color attribute. | |
INLINE void | tte_set_special (u16 cattr) |
Set special color attribute. | |
INLINE void | tte_set_surface (const TSurface *srf) |
Set the text surface. | |
INLINE void | tte_set_font (const TFont *font) |
Set the font. | |
INLINE void | tte_set_drawg (fnDrawg proc) |
Set the character plotter. | |
INLINE void | tte_set_erase (fnErase proc) |
Set the character plotter. | |
INLINE void | tte_set_string_table (const char *table[]) |
Set string table. | |
INLINE void | tte_set_font_table (const TFont *table[]) |
Set font table. | |
void | tte_set_margins (int left, int top, int right, int bottom) |
void | tte_init_con (void) |
Init stdio capabilities. | |
int | tte_cmd_vt100 (const char *text) |
Parse for VT100-sequences. | |
int | tte_con_write (struct _reent *r, int fd, const char *text, int len) |
Internal routine for stdio functionality. | |
int | tte_con_nocash (struct _reent *r, int fd, const char *text, int len) |
void | tte_init_bmp (int vmode, const TFont *font, fnDrawg proc) |
Initialize text system for bitmap fonts. | |
void | tte_init_obj (OBJ_ATTR *dst, u32 attr0, u32 attr1, u32 attr2, u32 clrs, u32 bupofs, const TFont *font, fnDrawg proc) |
Initialize text system for screen-entry fonts. | |
void | obj_erase (int left, int top, int right, int bottom) |
Unwind the object text-buffer. | |
void | obj_drawg (uint gid) |
Character-plot for objects. | |
Regular tilemaps | |
void | tte_init_se (int bgnr, u16 bgcnt, SCR_ENTRY se0, u32 clrs, u32 bupofs, const TFont *font, fnDrawg proc) |
Initialize text system for screen-entry fonts. | |
void | se_erase (int left, int top, int right, int bottom) |
Erase part of the regular tilemap canvas. | |
void | se_drawg_w8h8 (uint gid) |
Character-plot for reg BGs using an 8x8 font. | |
void | se_drawg_w8h16 (uint gid) |
Character-plot for reg BGs using an 8x16 font. | |
void | se_drawg (uint gid) |
Character-plot for reg BGs, any sized font. | |
void | se_drawg_s (uint gid) |
Character-plot for reg BGs, any sized, vertically tiled font. | |
Affine tilemaps | |
void | tte_init_ase (int bgnr, u16 bgcnt, u8 ase0, u32 clrs, u32 bupofs, const TFont *font, fnDrawg proc) |
Initialize text system for affine screen-entry fonts. | |
void | ase_erase (int left, int top, int right, int bottom) |
Erase part of the affine tilemap canvas. | |
void | ase_drawg_w8h8 (uint gid) |
Character-plot for affine BGs using an 8x8 font. | |
void | ase_drawg_w8h16 (uint gid) |
Character-plot for affine BGs using an 8x16 font. | |
void | ase_drawg (uint gid) |
Character-plot for affine Bgs, any size. | |
void | ase_drawg_s (uint gid) |
Character-plot for affine BGs, any sized,vertically oriented font. | |
4bpp tiles | |
void | tte_init_chr4c (int bgnr, u16 bgcnt, u16 se0, u32 cattrs, u32 clrs, const TFont *font, fnDrawg proc) |
Initialize text system for 4bpp tiled, column-major surfaces. | |
void | chr4c_erase (int left, int top, int right, int bottom) |
Erase part of the 4bpp text canvas. | |
void | chr4c_drawg_b1cts (uint gid) |
Render 1bpp fonts to 4bpp tiles. | |
IWRAM_CODE void | chr4c_drawg_b1cts_fast (uint gid) |
Initialize text system for 4bpp tiled, column-major surfaces. | |
void | chr4c_drawg_b4cts (uint gid) |
Initialize text system for 4bpp tiled, column-major surfaces. | |
IWRAM_CODE void | chr4c_drawg_b4cts_fast (uint gid) |
Initialize text system for 4bpp tiled, column-major surfaces. | |
4bpp tiles | |
void | tte_init_chr4r (int bgnr, u16 bgcnt, u16 se0, u32 cattrs, u32 clrs, const TFont *font, fnDrawg proc) |
Initialize text system for 4bpp tiled, column-major surfaces. | |
void | chr4r_erase (int left, int top, int right, int bottom) |
Erase part of the 4bpp text canvas. | |
void | chr4r_drawg_b1cts (uint gid) |
Render 1bpp fonts to 4bpp tiles. | |
IWRAM_CODE void | chr4r_drawg_b1cts_fast (uint gid) |
Initialize text system for 4bpp tiled, column-major surfaces. | |
8bpp bitmaps | |
void | bmp8_erase (int left, int top, int right, int bottom) |
Erase part of the 8bpp text canvas. | |
void | bmp8_drawg (uint gid) |
Linear 8 bpp bitmap glyph renderer, opaque. | |
void | bmp8_drawg_t (uint gid) |
Linear 8 bpp bitmap glyph renderer, transparent. | |
void | bmp8_drawg_b1cts (uint gid) |
Erase part of the 8bpp text canvas. | |
IWRAM_CODE void | bmp8_drawg_b1cts_fast (uint gid) |
Erase part of the 8bpp text canvas. | |
void | bmp8_drawg_b1cos (uint gid) |
Erase part of the 8bpp text canvas. | |
16bpp bitmaps | |
void | bmp16_erase (int left, int top, int right, int bottom) |
Erase part of the 16bpp text canvas. | |
void | bmp16_drawg (uint gid) |
Linear 16bpp bitmap glyph renderer, opaque. | |
void | bmp16_drawg_t (uint gid) |
Linear 16bpp bitmap glyph renderer, transparent. | |
void | bmp16_drawg_b1cts (uint gid) |
Linear bitmap, 16bpp transparent character plotter. | |
void | bmp16_drawg_b1cos (uint gid) |
Linear bitmap, 16bpp opaque character plotter. | |
Variables | |
TTC * | gp_tte_context |
Internal fonts | |
const TFont | sys8Font |
System font ' '-127. FWF 8x 8@1. | |
const TFont | verdana9Font |
Verdana 9 ' '-'�'. VWF 8x12@1. | |
const TFont | verdana9bFont |
Verdana 9 bold ' '-'�'. VWF 8x12@1. | |
const TFont | verdana9iFont |
Verdana 9 italic ' '-'�'. VWF 8x12@1. | |
const TFont | verdana10Font |
Verdana 10 ' '-'�'. VWF 16x14@1. | |
const TFont | verdana9_b4Font |
Verdana 9 ' '-'�'. VWF 8x12@4. | |
const unsigned int | sys8Glyphs [192] |
System font ' '-127. FWF 8x 8@1. | |
const unsigned int | verdana9Glyphs [896] |
System font ' '-127. FWF 8x 8@1. | |
const unsigned char | verdana9Widths [224] |
System font ' '-127. FWF 8x 8@1. | |
const unsigned int | verdana9bGlyphs [896] |
System font ' '-127. FWF 8x 8@1. | |
const unsigned char | verdana9bWidths [224] |
System font ' '-127. FWF 8x 8@1. | |
const unsigned int | verdana9iGlyphs [896] |
System font ' '-127. FWF 8x 8@1. | |
const unsigned char | verdana9iWidths [224] |
System font ' '-127. FWF 8x 8@1. | |
const unsigned int | verdana10Glyphs [1792] |
System font ' '-127. FWF 8x 8@1. | |
const unsigned char | verdana10Widths [224] |
System font ' '-127. FWF 8x 8@1. | |
const unsigned int | verdana9_b4Glyphs [3584] |
System font ' '-127. FWF 8x 8@1. | |
const unsigned char | verdana9_b4Widths [224] |
System font ' '-127. FWF 8x 8@1. |
Detailed Description
- Author:
- J Vijn
- Date:
- 20070517 - 20080503
Generated on Mon Aug 25 17:03:56 2008 for libtonc by
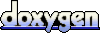