Colors
[Video]
Base Color constants | |
#define | CLR_BLACK 0x0000 |
#define | CLR_RED 0x001F |
#define | CLR_LIME 0x03E0 |
#define | CLR_YELLOW 0x03FF |
#define | CLR_BLUE 0x7C00 |
#define | CLR_MAG 0x7C1F |
#define | CLR_CYAN 0x7FE0 |
#define | CLR_WHITE 0x7FFF |
Additional colors | |
#define | CLR_DEAD 0xDEAD |
#define | CLR_MAROON 0x0010 |
#define | CLR_GREEN 0x0200 |
#define | CLR_OLIVE 0x0210 |
#define | CLR_ORANGE 0x021F |
#define | CLR_NAVY 0x4000 |
#define | CLR_PURPLE 0x4010 |
#define | CLR_TEAL 0x4200 |
#define | CLR_GRAY 0x4210 |
#define | CLR_MEDGRAY 0x5294 |
#define | CLR_SILVER 0x6318 |
#define | CLR_MONEYGREEN 0x6378 |
#define | CLR_FUCHSIA 0x7C1F |
#define | CLR_SKYBLUE 0x7B34 |
#define | CLR_CREAM 0x7BFF |
Defines | |
#define | CLR_MASK 0x001F |
#define | RED_MASK 0x001F |
#define | RED_SHIFT 0 |
#define | GREEN_MASK 0x03E0 |
#define | GREEN_SHIFT 5 |
#define | BLUE_MASK 0x7C00 |
#define | BLUE_SHIFT 10 |
Functions | |
void | clr_rotate (COLOR *clrs, uint nclrs, int ror) |
Rotate nclrs colors at clrs to the right by ror. | |
void | clr_blend (const COLOR *srca, const COLOR *srcb, COLOR *dst, u32 nclrs, u32 alpha) |
Blends color arrays srca and srcb into dst. | |
void | clr_fade (const COLOR *src, COLOR clr, COLOR *dst, u32 nclrs, u32 alpha) |
Fades color arrays srca to clr into dst. | |
void | clr_grayscale (COLOR *dst, const COLOR *src, uint nclrs) |
Transform colors to grayscale. | |
void | clr_rgbscale (COLOR *dst, const COLOR *src, uint nclrs, COLOR clr) |
Transform colors to an rgb-scale. | |
void | clr_adj_brightness (COLOR *dst, const COLOR *src, uint nclrs, FIXED bright) |
Adjust brightness by bright. | |
void | clr_adj_contrast (COLOR *dst, const COLOR *src, uint nclrs, FIXED contrast) |
Adjust contrast by contrast. | |
void | clr_adj_intensity (COLOR *dst, const COLOR *src, uint nclrs, FIXED intensity) |
Adjust intensity by intensity. | |
void | pal_gradient (COLOR *pal, int first, int last) |
Create a gradient between pal[first] and pal[last]. | |
void | pal_gradient_ex (COLOR *pal, int first, int last, COLOR clr_first, COLOR clr_last) |
Create a gradient between pal[first] and pal[last]. | |
IWRAM_CODE void | clr_blend_fast (COLOR *srca, COLOR *srcb, COLOR *dst, uint nclrs, u32 alpha) |
Blends color arrays srca and srcb into dst. | |
IWRAM_CODE void | clr_fade_fast (COLOR *src, COLOR clr, COLOR *dst, uint nclrs, u32 alpha) |
Fades color arrays srca to clr into dst. | |
INLINE COLOR | RGB15 (int red, int green, int blue) |
Create a 15bit BGR color. | |
INLINE COLOR | RGB15_SAFE (int red, int green, int blue) |
Create a 15bit BGR color, with proper masking of R,G,B components. | |
INLINE COLOR | RGB8 (u8 red, u8 green, u8 blue) |
Create a 15bit BGR color, using 8bit components. |
Function Documentation
Adjust brightness by bright.
Operation: color= color+dB;
- Parameters:
-
dst Destination color array src Source color array. nclrs Number of colors. bright Brightness difference, dB (in .8f)
- Note:
- Might be faster if preformed via lut.
Adjust contrast by contrast.
Operation: color = color*(1+dC) - MAX*dC/2
- Parameters:
-
dst Destination color array src Source color array. nclrs Number of colors. contrast Contrast differencem dC (in .8f)
- Note:
- Might be faster if preformed via lut.
Adjust intensity by intensity.
Operation: color = (1+dI)*color.
- Parameters:
-
dst Destination color array src Source color array. nclrs Number of colors. intensity Intensity difference, dI (in .8f)
- Note:
- Might be faster if preformed via lut.
Blends color arrays srca and srcb into dst.
Specific transitional blending effects can be created by making a 'target' color array with other routines, then using alpha to morph into it.
- Parameters:
-
srca Source array A. srcb Source array B dst Destination array. nclrs Number of colors. alpha Blend weight (range: 0-32). 0 Means full srca
Blends color arrays srca and srcb into dst.
- Parameters:
-
srca Source array A. srcb Source array B dst Destination array. nclrs Number of colors. alpha Blend weight (range: 0-32).
- Note:
- Handles 2 colors per loop. Very fast.
Fades color arrays srca to clr into dst.
- Parameters:
-
src Source array. clr Final color (at alpha=32). dst Destination array. nclrs Number of colors. alpha Blend weight (range: 0-32). 0 Means full srca
Fades color arrays srca to clr into dst.
- Parameters:
-
src Source array. clr Final color (at alpha=32). dst Destination array. nclrs Number of colors. alpha Blend weight (range: 0-32).
- Note:
- Handles 2 colors per loop. Very fast.
Transform colors to grayscale.
- Parameters:
-
dst Destination color array src Source color array. nclrs Number of colors.
Transform colors to an rgb-scale.
clr indicates a color vector in RGB-space. Each source color is converted to a brightness value (i.e., grayscale) and then mapped onto that color vector. A grayscale is a special case of this, using a color with R=G=B.
- Parameters:
-
dst Destination color array src Source color array. nclrs Number of colors. clr Destination color vector.
void clr_rotate | ( | COLOR * | clrs, | |
uint | nclrs, | |||
int | ror | |||
) |
Rotate nclrs colors at clrs to the right by ror.
- Note:
- I can't help but think there's a faster way ... I just can't see it atm.
void pal_gradient | ( | COLOR * | pal, | |
int | first, | |||
int | last | |||
) |
Create a gradient between pal[first] and pal[last].
- Parameters:
-
pal Palette to work on. first First index of gradient. last Last index of gradient.
Create a gradient between pal[first] and pal[last].
- Parameters:
-
pal Palette to work on. first First index of gradient. last Last index of gradient. clr_first Color of first index. clr_last Color of last index.
Generated on Mon Aug 25 17:03:57 2008 for libtonc by
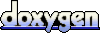