Base math
[Math]
Basic math macros and functions like MIN, MAX.
More...core math macros | |
INLINE int | sgn (int x) |
Get the sign of x. | |
INLINE int | sgn3 (int x) |
Tri-state sign of x: -1 for negative, 0 for 0, +1 for positive. | |
INLINE int | max (int a, int b) |
Get the maximum of a and b. | |
INLINE int | min (int a, int b) |
Get the minimum of a and b. | |
#define | ABS(x) ( (x)>=0 ? (x) : -(x) ) |
Get the absolute value of x. | |
#define | SGN(x) ( (x)>=0 ? 1 : -1 ) |
Get the sign of x. | |
#define | SGN2 SGN |
Get the absolute value of x. | |
#define | SGN3(x) ( (x)>0 ? 1 : ( (x)<0 ? -1 : 0) ) |
Tri-state sign: -1 for negative, 0 for 0, +1 for positive. | |
#define | MAX(a, b) ( ((a) > (b)) ? (a) : (b) ) |
Get the maximum of a and b. | |
#define | MIN(a, b) ( ((a) < (b)) ? (a) : (b) ) |
Get the minimum of a and b. | |
#define | SWAP2(a, b) do { a=(a)-(b); b=(a)+(b); a=(b)-(a); } while(0) |
In-place swap. | |
#define | SWAP SWAP2 |
Get the absolute value of x. | |
#define | SWAP3(a, b, tmp) do { (tmp)=(a); (a)=(b); (b)=(tmp); } while(0) |
Swaps a and b, using tmp as a temporary. | |
Boundary response macros | |
INLINE BOOL | in_range (int x, int min, int max) |
Range check. | |
INLINE int | clamp (int x, int min, int max) |
Truncates x to stay in range [min, max>. | |
INLINE int | reflect (int x, int min, int max) |
Reflects x at boundaries min and max. | |
INLINE int | wrap (int x, int min, int max) |
Wraps x to stay in range [min, max>. | |
#define | IN_RANGE(x, min, max) ( ((x)>=(min)) && ((x)<(max)) ) |
Range check. | |
#define | CLAMP(x, min, max) ( (x)>=(max) ? ((max)-1) : ( ((x)<(min)) ? (min) : (x) ) ) |
Truncates x to stay in range [min, max>. | |
#define | REFLECT(x, min, max) ( (x)>=(max) ? 2*((max)-1)-(x) : ( ((x)<(min)) ? 2*(min)-(x) : (x) ) ) |
Reflects x at boundaries min and max. | |
#define | WRAP(x, min, max) ( (x)>=(max) ? (x)+(min)-(max) : ( ((x)<(min)) ? (x)+(max)-(min) : (x) ) ) |
Wraps x to stay in range [min, max>. |
Detailed Description
Basic math macros and functions like MIN, MAX.
Define Documentation
#define CLAMP | ( | x, | |||
min, | |||||
max | ) | ( (x)>=(max) ? ((max)-1) : ( ((x)<(min)) ? (min) : (x) ) ) |
Truncates x to stay in range [min, max>.
- Returns:
- Truncated value of x.
- Note:
- max is exclusive!
#define REFLECT | ( | x, | |||
min, | |||||
max | ) | ( (x)>=(max) ? 2*((max)-1)-(x) : ( ((x)<(min)) ? 2*(min)-(x) : (x) ) ) |
Reflects x at boundaries min and max.
If x is outside the range [min, max>, it'll be placed inside again with the same distance to the 'wall', but on the other side. Example for lower border: y = min - (x- min) = 2*min + x.
- Returns:
- Reflected value of x.
- Note:
- max is exclusive!
Function Documentation
INLINE int clamp | ( | int | x, | |
int | min, | |||
int | max | |||
) |
Truncates x to stay in range [min, max>.
- Returns:
- Truncated value of x.
- Note:
- max is exclusive!
INLINE int reflect | ( | int | x, | |
int | min, | |||
int | max | |||
) |
Reflects x at boundaries min and max.
If x is outside the range [min, max>, it'll be placed inside again with the same distance to the 'wall', but on the other side. Example for lower border: y = min - (x- min) = 2*min + x.
- Returns:
- Reflected value of x.
- Note:
- max is exclusive!
Generated on Mon Aug 25 17:03:57 2008 for libtonc by
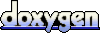