Data routines
[Core]
Copying and filling routines | |
void * | tonccpy (void *dst, const void *src, uint size) |
VRAM-safe cpy. | |
void * | __toncset (void *dst, u32 fill, uint size) |
VRAM-safe memset, internal routine. | |
INLINE void * | toncset (void *dst, u8 src, uint count) |
VRAM-safe memset, byte version. Size in bytes. | |
INLINE void * | toncset16 (void *dst, u16 src, uint count) |
VRAM-safe memset, halfword version. Size in hwords. | |
INLINE void * | toncset32 (void *dst, u32 src, uint count) |
VRAM-safe memset, word version. Size in words. | |
void | memset16 (void *dst, u16 hw, uint hwcount) |
Fastfill for halfwords, analogous to memset(). | |
void | memcpy16 (void *dst, const void *src, uint hwcount) |
IWRAM_CODE void | memset32 (void *dst, u32 wd, uint wdcount) |
Fast-fill by words, analogous to memset(). | |
IWRAM_CODE void | memcpy32 (void *dst, const void *src, uint wdcount) |
#define | GRIT_CPY(dst, name) memcpy16(dst, name, name##Len/2) |
Simplified copier for GRIT-exported data. | |
Repeated-value creators | |
These function take a hex-value and duplicate it to all fields, like 0x88 -> 0x88888888. | |
INLINE u16 | dup8 (u8 x) |
Duplicate a byte to form a halfword: 0x12 -> 0x1212. | |
INLINE u32 | dup16 (u16 x) |
Duplicate a halfword to form a word: 0x1234 -> 0x12341234. | |
INLINE u32 | quad8 (u8 x) |
Quadruple a byte to form a word: 0x12 -> 0x12121212. | |
INLINE u32 | octup (u8 x) |
Octuple a nybble to form a word: 0x1 -> 0x11111111. | |
Packing routines. | |
INLINE u16 | bytes2hword (u8 b0, u8 b1) |
Pack 2 bytes into a word. Little-endian order. | |
INLINE u32 | bytes2word (u8 b0, u8 b1, u8 b2, u8 b3) |
Pack 4 bytes into a word. Little-endian order. | |
INLINE u32 | hword2word (u16 h0, u16 h1) |
Pack 2 bytes into a word. Little-endian order. | |
Defines | |
#define | countof(_array) ( sizeof(_array)/sizeof(_array[0]) ) |
Get the number of elements in an array. | |
Functions | |
INLINE uint | align (uint x, uint width) |
Align x to the next multiple of width. |
Function Documentation
void* __toncset | ( | void * | dst, | |
u32 | fill, | |||
uint | size | |||
) |
VRAM-safe memset, internal routine.
This version mimics memset in functionality, with the benefit of working for VRAM as well. It is also slightly faster than the original memset.
- Parameters:
-
dst Destination pointer. fill Word to fill with. size Fill-length in bytes.
- Returns:
- dst.
- Note:
- The dst pointer and size need not be word-aligned. In the case of unaligned fills, fill will be masked off to match the situation.
void memcpy16 | ( | void * | dst, | |
const void * | src, | |||
uint | hwcount | |||
) |
Copy for halfwords.
Uses memcpy32()
if hwn>6 and src and dst are aligned equally.
- Parameters:
-
dst Destination address. src Source address. hwcount Number of halfwords to fill.
- Note:
- dst and src must be halfword aligned.
r0 and r1 return as dst + hwcount*2 and src + hwcount*2.
IWRAM_CODE void memcpy32 | ( | void * | dst, | |
const void * | src, | |||
uint | wdcount | |||
) |
Fast-copy by words.
Like CpuFastFill(), only without the requirement of 32byte chunks
- Parameters:
-
dst Destination address. src Source address. wdcount Number of words.
- Note:
- src and dst must be word aligned.
r0 and r1 return as dst + wdcount*4 and src + wdcount*4.
void memset16 | ( | void * | dst, | |
u16 | hw, | |||
uint | hwcount | |||
) |
Fastfill for halfwords, analogous to memset().
Uses memset32()
if hwcount>5
- Parameters:
-
dst Destination address. hw Source halfword (not address). hwcount Number of halfwords to fill.
- Note:
- dst must be halfword aligned.
r0 returns as dst + hwcount*2.
IWRAM_CODE void memset32 | ( | void * | dst, | |
u32 | wd, | |||
uint | wdcount | |||
) |
Fast-fill by words, analogous to memset().
Like CpuFastSet(), only without the requirement of 32byte chunks and no awkward store-value-in-memory-first issue.
- Parameters:
-
dst Destination address. wd Fill word (not address). wdcount Number of words to fill.
- Note:
- dst must be word aligned.
r0 returns as dst + wdcount*4.
void* tonccpy | ( | void * | dst, | |
const void * | src, | |||
uint | size | |||
) |
VRAM-safe cpy.
This version mimics memcpy in functionality, with the benefit of working for VRAM as well. It is also slightly faster than the original memcpy, but faster implementations can be made.
- Parameters:
-
dst Destination pointer. src Source pointer. size Fill-length in bytes.
- Returns:
- dst.
- Note:
- The pointers and size need not be word-aligned.
Generated on Mon Aug 25 17:03:57 2008 for libtonc by
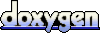