Bit(field) macros
[Core]
Simple bit macros | |
#define | BIT(n) ( 1<<(n) ) |
Create value with bit n set. | |
#define | BIT_SHIFT(a, n) ( (a)<<(n) ) |
Shift a by n. | |
#define | BIT_MASK(len) ( BIT(len)-1 ) |
Create a bitmask len bits long. | |
#define | BIT_SET(y, flag) ( y |= (flag) ) |
Set the flag bits in word. | |
#define | BIT_CLEAR(y, flag) ( y &= ~(flag) ) |
Clear the flag bits in word. | |
#define | BIT_FLIP(y, flag) ( y ^= (flag) ) |
Flip the flag bits in word. | |
#define | BIT_EQ(y, flag) ( ((y)&(flag)) == (flag) ) |
Test whether all the flag bits in word are set. | |
#define | BF_MASK(shift, len) ( BIT_MASK(len)<<(shift) ) |
Create a bitmask of length len starting at bit shift. | |
#define | _BF_GET(y, shift, len) ( ((y)>>(len))&(shift) ) |
Retrieve a bitfield mask of length starting at bit shift from y. | |
#define | _BF_PREP(x, shift, len) ( ((x)&BIT_MASK(len))<<(shift) ) |
Prepare a bitmask for insertion or combining. | |
#define | _BF_SET(y, x, shift, len) ( y= ((y) &~ BF_MASK(shift, len)) | _BF_PREP(x, shift, len) ) |
Insert a new bitfield value x into y. | |
some EVIL bit-field operations, >:) | |
These allow you to mimic bitfields with macros. Most of the bitfields in the registers have foo_SHIFT and foo_SHIFT macros indicating the mask and shift values of the bitfield named foo in a variable. These macros let you prepare, get and set the bitfields. | |
#define | BFN_PREP(x, name) ( ((x)<<name##_SHIFT) & name##_MASK ) |
Prepare a named bit-field for for insterion or combination. | |
#define | BFN_GET(y, name) ( ((y) & name##_MASK)>>name##_SHIFT ) |
Get the value of a named bitfield from y. Equivalent to (var=) y.name. | |
#define | BFN_SET(y, x, name) (y = ((y)&~name##_MASK) | BFN_PREP(x,name) ) |
Set a named bitfield in y to x. Equivalent to y.name= x. | |
#define | BFN_CMP(y, x, name) ( ((y)&name##_MASK) == (x) ) |
Compare a named bitfield to named literal x. | |
#define | BFN_PREP2(x, name) ( (x) & name##_MASK ) |
Massage x for use in bitfield name with pre-shifted x. | |
#define | BFN_GET2(y, name) ( (y) & name##_MASK ) |
Get the value of bitfield name from y, but don't down-shift. | |
#define | BFN_SET2(y, x, name) ( y = ((y)&~name##_MASK) | BFN_PREP2(x,name) ) |
Set bitfield name from y to x with pre-shifted x. | |
Functions | |
INLINE u32 | bf_get (u32 y, uint shift, uint len) |
Get len long bitfield from y, starting at shift. | |
INLINE u32 | bf_merge (u32 y, u32 x, uint shift, uint len) |
Merge x into an len long bitfield from y, starting at shift. | |
INLINE u32 | bf_clamp (int x, uint len) |
Clamp to within the range allowed by len bits. | |
INLINE int | bit_tribool (u32 flags, uint plus, uint minus) |
Gives a tribool (-1, 0, or +1) depending on the state of some bits. | |
INLINE u32 | ROR (u32 x, uint ror) |
Rotate bits right. Yes, this does lead to a ror instruction. |
Function Documentation
INLINE u32 bf_get | ( | u32 | y, | |
uint | shift, | |||
uint | len | |||
) |
Get len long bitfield from y, starting at shift.
- Parameters:
-
y Value containing bitfield. shift Bitfield Start; len Length of bitfield.
- Returns:
- Bitfield between bits shift and shift + length.
INLINE u32 bf_merge | ( | u32 | y, | |
u32 | x, | |||
uint | shift, | |||
uint | len | |||
) |
Merge x into an len long bitfield from y, starting at shift.
- Parameters:
-
y Value containing bitfield. x Value to merge (will be masked to fit). shift Bitfield Start; len Length of bitfield.
- Returns:
- Result of merger: (y&~M) | (x<<s & M)
- Note:
- Does not write the result back into y (Because pure C does't have references, that's why)
INLINE int bit_tribool | ( | u32 | flags, | |
uint | plus, | |||
uint | minus | |||
) |
Gives a tribool (-1, 0, or +1) depending on the state of some bits.
Looks at the plus and minus bits of flags, and subtracts their status to give a +1, -1 or 0 result. Useful for direction flags.
- Parameters:
-
flags Value with bit-flags. plus Bit number for positive result. minus Bit number for negative result.
- Returns:
- +1 if plus bit is set but minus bit isn't
-1 if minus bit is set and plus bit isn't
0 if neither or both are set.
Generated on Mon Aug 25 17:03:57 2008 for libtonc by
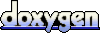