Tonc Text Engine
A generalized raster text system. More...Modules | |
Operations | |
Basic operations. | |
Attributes | |
Basic getters and setters. | |
Console IO | |
Stdio functionality. | |
Tilemap text | |
Text for regular and affine tilemaps. | |
Character text, column-major | |
Text on surface composed of 4bpp tiles, mapped in column-major order. | |
Character text, row-major | |
Text on surface composed of 4bpp tiles, mapped in row-major order. | |
Bitmap text | |
Text for 16bpp and 8bpp bitmap surfaces: modes 3, 4 and 5. | |
Object text | |
Text using objects. | |
Data Structures | |
struct | TFont |
Font description struct. More... | |
struct | TTC |
TTE context struct. More... | |
Internal fonts | |
const TFont | sys8Font |
System font ' '-127. FWF 8x 8@1. | |
const TFont | verdana9Font |
Verdana 9 ' '-'�'. VWF 8x12@1. | |
const TFont | verdana9bFont |
Verdana 9 bold ' '-'�'. VWF 8x12@1. | |
const TFont | verdana9iFont |
Verdana 9 italic ' '-'�'. VWF 8x12@1. | |
const TFont | verdana10Font |
Verdana 10 ' '-'�'. VWF 16x14@1. | |
const TFont | verdana9_b4Font |
Verdana 9 ' '-'�'. VWF 8x12@4. | |
const unsigned int | sys8Glyphs [192] |
System font ' '-127. FWF 8x 8@1. | |
const unsigned int | verdana9Glyphs [896] |
System font ' '-127. FWF 8x 8@1. | |
const unsigned char | verdana9Widths [224] |
System font ' '-127. FWF 8x 8@1. | |
const unsigned int | verdana9bGlyphs [896] |
System font ' '-127. FWF 8x 8@1. | |
const unsigned char | verdana9bWidths [224] |
System font ' '-127. FWF 8x 8@1. | |
const unsigned int | verdana9iGlyphs [896] |
System font ' '-127. FWF 8x 8@1. | |
const unsigned char | verdana9iWidths [224] |
System font ' '-127. FWF 8x 8@1. | |
const unsigned int | verdana10Glyphs [1792] |
System font ' '-127. FWF 8x 8@1. | |
const unsigned char | verdana10Widths [224] |
System font ' '-127. FWF 8x 8@1. | |
const unsigned int | verdana9_b4Glyphs [3584] |
System font ' '-127. FWF 8x 8@1. | |
const unsigned char | verdana9_b4Widths [224] |
System font ' '-127. FWF 8x 8@1. | |
Color lut indices | |
#define | TTE_INK 0 |
#define | TTE_SHADOW 1 |
#define | TTE_PAPER 2 |
#define | TTE_SPECIAL 3 |
drawg helper macros | |
#define | TTE_BASE_VARS(_tc, _font) |
Declare and define base drawg variables. | |
#define | TTE_CHAR_VARS(font, gid, src_t, _sD, _sL, _chW, _chH) |
Declare and define basic source drawg variables. | |
#define | TTE_DST_VARS(tc, dst_t, _dD, _dL, _dP, _x, _y) |
Declare and define basic destination drawg variables. | |
Default fonts | |
#define | fwf_default sys8Font |
Default fixed-width font. | |
#define | vwf_default verdana9Font |
Default vairable-width font. | |
Default glyph renderers | |
#define | ase_drawg_default ((fnDrawg)ase_drawg_s) |
#define | bmp8_drawg_default ((fnDrawg)bmp8_drawg_b1cts) |
#define | bmp16_drawg_default ((fnDrawg)bmp16_drawg_b1cts) |
#define | chr4c_drawg_default ((fnDrawg)chr4c_drawg_b1cts) |
#define | chr4r_drawg_default ((fnDrawg)chr4r_drawg_b1cts) |
#define | obj_drawg_default ((fnDrawg)obj_drawg) |
#define | se_drawg_default ((fnDrawg)se_drawg_s) |
Default initializers | |
#define | tte_init_se_default(bgnr, bgcnt) tte_init_se( bgnr, bgcnt, 0xF000, CLR_YELLOW, 0, &fwf_default, NULL) |
#define | tte_init_ase_default(bgnr, bgcnt) tte_init_ase(bgnr, bgcnt, 0x0000, CLR_YELLOW, 0, &fwf_default, NULL) |
#define | tte_init_chr4c_default(bgnr, bgcnt) |
#define | tte_init_chr4r_default(bgnr, bgcnt) |
#define | tte_init_chr4c_b4_default(bgnr, bgcnt) |
#define | tte_init_bmp_default(mode) tte_init_bmp(mode, &vwf_default, NULL) |
#define | tte_init_obj_default(pObj) tte_init_obj(pObj, 0, 0, 0xF000, CLR_YELLOW, 0, &fwf_default, NULL) |
Defines | |
#define | TTE_TAB_WIDTH 24 |
Typedefs | |
typedef void(* | fnDrawg )(uint gid) |
Glyph render function format. | |
typedef void(* | fnErase )(int left, int top, int right, int bottom) |
Erase rectangle function format. | |
Variables | |
TTC * | gp_tte_context |
Detailed Description
A generalized raster text system.As of v1.3, Tonc has a completely new way of handling text. It can handle (practically) all modes, VRAM types and font sizes and brings them together under a unified interface. It uses function pointers to store drawg and erase functions of each rendering family. The families currently supported are:
- ase: Affine screen entries (Affine tiled BG)
- bmp8: 8bpp bitmaps (Mode 4)
- bmp16 16bpp bitmaps (Mode 3/5)
- chr4c 4bpp characters, column-major (Regular tiled BG)
- chr4r 4bpp characters, row-major (Regular tiled BG)
- obj Objects
- se Regular screen entries (Regular tiled BG)
Each of these consists of an initializer, tte_init_foo
, and one or more glyph rendering functions, foo_puts_bar
, The bar
part of the renderer denotes the style of the particular renderer, which can indicate:
- Expected bitdepth of font data (
b1
for 1bpp, etc) - Expected sizes of the character (
w8
and h8, for example). - Application of system colors (
c
). - Transparent or opaque background pixels (
t
oro
). - Whether the font-data is in 'strip' layout (
s
)
The included renderers here are usually transparent, recolored, using 1bpp strip glyphs (_b1cts
). The initializer takes a bunch of options specific to each family, as well as font and renderer pointers. You can provide your own font and renderers, provided they're formatted correcty. For the default font/renderers, use NULL
.
After the calling the initializer, you can write utf-8 encoded text with tte_write() or tte_write_ex(). You can also enable stdio-related functions by calling tte_init_con().
The system also supposed rudimentary scripting for positions, colors, margins and erases. See tte_cmd_default() and con_cmd_parse() for details.
- See also:
- Surface functions
Define Documentation
#define TTE_BASE_VARS | ( | _tc, | |||
_font | ) |
Value:
TTC *_tc= tte_get_context(); \ TFont *_font= tc->font; \
Each drawg
renderer usually starts with the same thing:
- Get the system and font pointers.
- Translate from ascii-character to glyph offset.
- Get the glyph (and glyph-cell) dimensions.
- Get the source and destination pointers and positions. These macros will make declarint and defining that easier.
#define TTE_CHAR_VARS | ( | font, | |||
gid, | |||||
src_t, | |||||
_sD, | |||||
_sL, | |||||
_chW, | |||||
_chH | ) |
Value:
src_t *_sD= (src_t*)(font->data+gid*font->cellSize), *_sL= _sD; \ uint _chW= font->widths ? font->widths[gid] : font->charW; \ uint _chH= font->charH;
#define TTE_DST_VARS | ( | tc, | |||
dst_t, | |||||
_dD, | |||||
_dL, | |||||
_dP, | |||||
_x, | |||||
_y | ) |
Value:
uint _x= tc->cursorX, _y= tc->cursorY, _dP= tc->dst.pitch; \ dst_t *_dD= (dst_t*)(tc->dst.data+_y*_dP), *_dL;
#define tte_init_chr4c_b4_default | ( | bgnr, | |||
bgcnt | ) |
Value:
tte_init_chr4c(bgnr, bgcnt, 0xF000, 0x0201, CLR_ORANGE<<16|CLR_YELLOW, \ &verdana9_b4Font, chr4c_drawg_b4cts)
#define tte_init_chr4c_default | ( | bgnr, | |||
bgcnt | ) |
Value:
tte_init_chr4c(bgnr, bgcnt, 0xF000, 0x0201, CLR_ORANGE<<16|CLR_YELLOW, \ &vwf_default, NULL)
#define tte_init_chr4r_default | ( | bgnr, | |||
bgcnt | ) |
Value:
tte_init_chr4r(bgnr, bgcnt, 0xF000, 0x0201, CLR_ORANGE<<16|CLR_YELLOW, \ &vwf_default, NULL)
#define TTE_TAB_WIDTH 24 |
Generated on Mon Aug 25 17:03:57 2008 for libtonc by
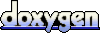