Bitmaps
[Video]
Generic 8bpp bitmaps | |
void | bmp8_plot (int x, int y, u32 clr, void *dstBase, uint dstP) |
Plot a single pixel on a 8-bit buffer. | |
void | bmp8_hline (int x1, int y, int x2, u32 clr, void *dstBase, uint dstP) |
Draw a horizontal line on an 8bit buffer. | |
void | bmp8_vline (int x, int y1, int y2, u32 clr, void *dstBase, uint dstP) |
Draw a vertical line on an 8bit buffer. | |
void | bmp8_line (int x1, int y1, int x2, int y2, u32 clr, void *dstBase, uint dstP) |
Draw a line on an 8bit buffer. | |
void | bmp8_rect (int left, int top, int right, int bottom, u32 clr, void *dstBase, uint dstP) |
Draw a rectangle in 8bit mode; internal routine. | |
void | bmp8_frame (int left, int top, int right, int bottom, u32 clr, void *dstBase, uint dstP) |
Draw a rectangle in 8bit mode; internal routine. | |
Generic 16bpp bitmaps | |
void | bmp16_plot (int x, int y, u32 clr, void *dstBase, uint dstP) |
Plot a single pixel on a 16-bit buffer. | |
void | bmp16_hline (int x1, int y, int x2, u32 clr, void *dstBase, uint dstP) |
Draw a horizontal line on an 16bit buffer. | |
void | bmp16_vline (int x, int y1, int y2, u32 clr, void *dstBase, uint dstP) |
Draw a vertical line on an 16bit buffer. | |
void | bmp16_line (int x1, int y1, int x2, int y2, u32 clr, void *dstBase, uint dstP) |
Draw a line on an 16bit buffer. | |
void | bmp16_rect (int left, int top, int right, int bottom, u32 clr, void *dstBase, uint dstP) |
Draw a rectangle in 16bit mode; internal routine. | |
void | bmp16_frame (int left, int top, int right, int bottom, u32 clr, void *dstBase, uint dstP) |
Draw a rectangle in 16bit mode; internal routine. | |
mode 3 | |
INLINE void | m3_fill (COLOR clr) |
Fill the mode 3 background with color clr. | |
INLINE void | m3_plot (int x, int y, COLOR clr) |
Plot a single clr colored pixel in mode 3 at (x, y). | |
INLINE void | m3_hline (int x1, int y, int x2, COLOR clr) |
Draw a clr colored horizontal line in mode 3. | |
INLINE void | m3_vline (int x, int y1, int y2, COLOR clr) |
Draw a clr colored vertical line in mode 3. | |
INLINE void | m3_line (int x1, int y1, int x2, int y2, COLOR clr) |
Draw a clr colored line in mode 3. | |
INLINE void | m3_rect (int left, int top, int right, int bottom, COLOR clr) |
Draw a clr colored rectangle in mode 3. | |
INLINE void | m3_frame (int left, int top, int right, int bottom, COLOR clr) |
Draw a clr colored frame in mode 3. | |
#define | M3_CLEAR() memset32(vid_mem, 0, M3_SIZE/4) |
Fill the mode 3 background with color clr. | |
mode 4 | |
INLINE void | m4_fill (u8 clrid) |
Fill the current mode 4 backbuffer with clrid. | |
INLINE void | m4_plot (int x, int y, u8 clrid) |
Plot a clrid pixel on the current mode 4 backbuffer. | |
INLINE void | m4_hline (int x1, int y, int x2, u8 clrid) |
Draw a clrid colored horizontal line in mode 4. | |
INLINE void | m4_vline (int x, int y1, int y2, u8 clrid) |
Draw a clrid colored vertical line in mode 4. | |
INLINE void | m4_line (int x1, int y1, int x2, int y2, u8 clrid) |
Draw a clrid colored line in mode 4. | |
INLINE void | m4_rect (int left, int top, int right, int bottom, u8 clrid) |
Draw a clrid colored rectangle in mode 4. | |
INLINE void | m4_frame (int left, int top, int right, int bottom, u8 clrid) |
Draw a clrid colored frame in mode 4. | |
#define | M4_CLEAR() memset32(vid_page, 0, M4_SIZE/4) |
Fill the current mode 4 backbuffer with clrid. | |
mode 5 | |
INLINE void | m5_fill (COLOR clr) |
Fill the current mode 5 backbuffer with clr. | |
INLINE void | m5_plot (int x, int y, COLOR clr) |
Plot a clrid pixel on the current mode 5 backbuffer. | |
INLINE void | m5_hline (int x1, int y, int x2, COLOR clr) |
Draw a clr colored horizontal line in mode 5. | |
INLINE void | m5_vline (int x, int y1, int y2, COLOR clr) |
Draw a clr colored vertical line in mode 5. | |
INLINE void | m5_line (int x1, int y1, int x2, int y2, COLOR clr) |
Draw a clr colored line in mode 5. | |
INLINE void | m5_rect (int left, int top, int right, int bottom, COLOR clr) |
Draw a clr colored rectangle in mode 5. | |
INLINE void | m5_frame (int left, int top, int right, int bottom, COLOR clr) |
Draw a clr colored frame in mode 5. | |
#define | M5_CLEAR() memset32(vid_page, 0, M5_SIZE/4) |
Fill the current mode 5 backbuffer with clr. |
Detailed Description
Basic functions for dealing with bitmapped graphics.- Deprecated:
- The bmp8/bmp16 functions have been superceded by the surface functions (sbmp8/sbmp16) for the most part. The former group has been kept mostly for reference purposes.
Function Documentation
void bmp16_frame | ( | int | left, | |
int | top, | |||
int | right, | |||
int | bottom, | |||
u32 | clr, | |||
void * | dstBase, | |||
uint | dstP | |||
) |
Draw a rectangle in 16bit mode; internal routine.
- Parameters:
-
left Left side of rectangle; top Top side of rectangle. right Right side of rectangle. bottom Bottom side of rectangle. clr Color. dstBase Canvas pointer. dstP Canvas pitch in bytes
- Note:
- Does normalization, but not bounds checks.
PONDER: RB in- or exclusive?
void bmp16_hline | ( | int | x1, | |
int | y, | |||
int | x2, | |||
u32 | clr, | |||
void * | dstBase, | |||
uint | dstP | |||
) |
Draw a horizontal line on an 16bit buffer.
- Parameters:
-
x1 First X-coord. y Y-coord. x2 Second X-coord. clr Color. dstBase Canvas pointer (halfword-aligned plz). dstP Canvas pitch in bytes.
- Note:
- Does normalization, but not bounds checks.
void bmp16_line | ( | int | x1, | |
int | y1, | |||
int | x2, | |||
int | y2, | |||
u32 | clr, | |||
void * | dstBase, | |||
uint | dstP | |||
) |
Draw a line on an 16bit buffer.
- Parameters:
-
x1 First X-coord. y1 First Y-coord. x2 Second X-coord. y2 Second Y-coord. clr Color. dstBase Canvas pointer (halfword-aligned plz). dstP Canvas pitch in bytes.
- Note:
- Does normalization, but not bounds checks.
void bmp16_plot | ( | int | x, | |
int | y, | |||
u32 | clr, | |||
void * | dstBase, | |||
uint | dstP | |||
) |
Plot a single pixel on a 16-bit buffer.
- Parameters:
-
x X-coord. y Y-coord. clr Color. dstBase Canvas pointer (halfword-aligned plz). dstP Canvas pitch in bytes.
- Note:
- Slow as fuck. Inline plotting functionality if possible.
void bmp16_rect | ( | int | left, | |
int | top, | |||
int | right, | |||
int | bottom, | |||
u32 | clr, | |||
void * | dstBase, | |||
uint | dstP | |||
) |
Draw a rectangle in 16bit mode; internal routine.
- Parameters:
-
left Left side of rectangle; top Top side of rectangle. right Right side of rectangle. bottom Bottom side of rectangle. clr Color. dstBase Canvas pointer. dstP Canvas pitch in bytes
- Note:
- Does normalization, but not bounds checks.
void bmp16_vline | ( | int | x, | |
int | y1, | |||
int | y2, | |||
u32 | clr, | |||
void * | dstBase, | |||
uint | dstP | |||
) |
Draw a vertical line on an 16bit buffer.
- Parameters:
-
x X-coord. y1 First Y-coord. y2 Second Y-coord. clr Color. dstBase Canvas pointer (halfword-aligned plz). dstP Canvas pitch in bytes.
- Note:
- Does normalization, but not bounds checks.
void bmp8_frame | ( | int | left, | |
int | top, | |||
int | right, | |||
int | bottom, | |||
u32 | clr, | |||
void * | dstBase, | |||
uint | dstP | |||
) |
Draw a rectangle in 8bit mode; internal routine.
- Parameters:
-
left Left side of rectangle; top Top side of rectangle. right Right side of rectangle. bottom Bottom side of rectangle. clr Color-index. dstBase Canvas pointer. dstP Canvas pitch in bytes
- Note:
- Does normalization, but not bounds checks.
PONDER: RB in- or exclusive?
void bmp8_hline | ( | int | x1, | |
int | y, | |||
int | x2, | |||
u32 | clr, | |||
void * | dstBase, | |||
uint | dstP | |||
) |
Draw a horizontal line on an 8bit buffer.
- Parameters:
-
x1 First X-coord. y Y-coord. x2 Second X-coord. clr Color index. dstBase Canvas pointer (halfword-aligned plz). dstP canvas pitch in bytes.
- Note:
- Does normalization, but not bounds checks.
void bmp8_line | ( | int | x1, | |
int | y1, | |||
int | x2, | |||
int | y2, | |||
u32 | clr, | |||
void * | dstBase, | |||
uint | dstP | |||
) |
Draw a line on an 8bit buffer.
- Parameters:
-
x1 First X-coord. y1 First Y-coord. x2 Second X-coord. y2 Second Y-coord. clr Color index. dstBase Canvas pointer (halfword-aligned plz). dstP Canvas pitch in bytes.
- Note:
- Does normalization, but not bounds checks.
void bmp8_plot | ( | int | x, | |
int | y, | |||
u32 | clr, | |||
void * | dstBase, | |||
uint | dstP | |||
) |
Plot a single pixel on a 8-bit buffer.
- Parameters:
-
x X-coord. y Y-coord. clr Color. dstBase Canvas pointer (halfword-aligned plz). dstP Canvas pitch in bytes.
- Note:
- Slow as fuck. Inline plotting functionality if possible.
void bmp8_rect | ( | int | left, | |
int | top, | |||
int | right, | |||
int | bottom, | |||
u32 | clr, | |||
void * | dstBase, | |||
uint | dstP | |||
) |
Draw a rectangle in 8bit mode; internal routine.
- Parameters:
-
left Left side of rectangle; top Top side of rectangle. right Right side of rectangle. bottom Bottom side of rectangle. clr Color-index. dstBase Canvas pointer. dstP Canvas pitch in bytes
- Note:
- Does normalization, but not bounds checks.
void bmp8_vline | ( | int | x, | |
int | y1, | |||
int | y2, | |||
u32 | clr, | |||
void * | dstBase, | |||
uint | dstP | |||
) |
Draw a vertical line on an 8bit buffer.
- Parameters:
-
x X-coord. y1 First Y-coord. y2 Second Y-coord. clr Color index. dstBase Canvas pointer (halfword-aligned plz). dstP canvas pitch in bytes.
- Note:
- Does normalization, but not bounds checks.
INLINE void m3_frame | ( | int | left, | |
int | top, | |||
int | right, | |||
int | bottom, | |||
COLOR | clr | |||
) |
Draw a clr colored frame in mode 3.
- Parameters:
-
left Left side, inclusive. top Top size, inclusive. right Right size, exclusive. bottom Bottom size, exclusive. clr Color.
- Note:
- Normalized, but not clipped.
INLINE void m3_rect | ( | int | left, | |
int | top, | |||
int | right, | |||
int | bottom, | |||
COLOR | clr | |||
) |
Draw a clr colored rectangle in mode 3.
- Parameters:
-
left Left side, inclusive. top Top size, inclusive. right Right size, exclusive. bottom Bottom size, exclusive. clr Color.
- Note:
- Normalized, but not clipped.
INLINE void m4_frame | ( | int | left, | |
int | top, | |||
int | right, | |||
int | bottom, | |||
u8 | clrid | |||
) |
Draw a clrid colored frame in mode 4.
- Parameters:
-
left Left side, inclusive. top Top size, inclusive. right Right size, exclusive. bottom Bottom size, exclusive. clrid color index.
- Note:
- Normalized, but not clipped.
INLINE void m4_rect | ( | int | left, | |
int | top, | |||
int | right, | |||
int | bottom, | |||
u8 | clrid | |||
) |
Draw a clrid colored rectangle in mode 4.
- Parameters:
-
left Left side, inclusive. top Top size, inclusive. right Right size, exclusive. bottom Bottom size, exclusive. clrid color index.
- Note:
- Normalized, but not clipped.
INLINE void m5_frame | ( | int | left, | |
int | top, | |||
int | right, | |||
int | bottom, | |||
COLOR | clr | |||
) |
Draw a clr colored frame in mode 5.
- Parameters:
-
left Left side, inclusive. top Top size, inclusive. right Right size, exclusive. bottom Bottom size, exclusive. clr Color.
- Note:
- Normalized, but not clipped.
INLINE void m5_rect | ( | int | left, | |
int | top, | |||
int | right, | |||
int | bottom, | |||
COLOR | clr | |||
) |
Draw a clr colored rectangle in mode 5.
- Parameters:
-
left Left side, inclusive. top Top size, inclusive. right Right size, exclusive. bottom Bottom size, exclusive. clr Color.
- Note:
- Normalized, but not clipped.
Generated on Mon Aug 25 17:03:57 2008 for libtonc by
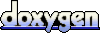