Assimp
v3.1.1 (June 2014)
|
Defines the C-API to the Open Asset Import Library. More...
Classes | |
struct | aiLogStream |
C-API: Represents a log stream. More... | |
struct | aiPropertyStore |
C-API: Represents an opaque set of settings to be used during importing. More... | |
Macros | |
#define | AI_FALSE 0 |
#define | AI_TRUE 1 |
Typedefs | |
typedef int | aiBool |
Our own C boolean type. More... | |
typedef void(* | aiLogStreamCallback) (const char *, char *) |
Functions | |
ASSIMP_API const aiScene * | aiApplyPostProcessing (const aiScene *pScene, unsigned int pFlags) |
Apply post-processing to an already-imported scene. More... | |
ASSIMP_API void | aiAttachLogStream (const aiLogStream *stream) |
Attach a custom log stream to the libraries' logging system. More... | |
ASSIMP_API aiPropertyStore * | aiCreatePropertyStore (void) |
Create an empty property store. More... | |
ASSIMP_API void | aiCreateQuaternionFromMatrix (aiQuaternion *quat, const aiMatrix3x3 *mat) |
Construct a quaternion from a 3x3 rotation matrix. More... | |
ASSIMP_API void | aiDecomposeMatrix (const aiMatrix4x4 *mat, aiVector3D *scaling, aiQuaternion *rotation, aiVector3D *position) |
Decompose a transformation matrix into its rotational, translational and scaling components. More... | |
ASSIMP_API void | aiDetachAllLogStreams (void) |
Detach all active log streams from the libraries' logging system. More... | |
ASSIMP_API aiReturn | aiDetachLogStream (const aiLogStream *stream) |
Detach a custom log stream from the libraries' logging system. More... | |
ASSIMP_API void | aiEnableVerboseLogging (aiBool d) |
Enable verbose logging. More... | |
ASSIMP_API const char * | aiGetErrorString () |
Returns the error text of the last failed import process. More... | |
ASSIMP_API void | aiGetExtensionList (aiString *szOut) |
Get a list of all file extensions supported by ASSIMP. More... | |
ASSIMP_API size_t | aiGetImportFormatCount (void) |
Returns the number of import file formats available in the current Assimp build. More... | |
ASSIMP_API const aiImporterDesc * | aiGetImportFormatDescription (size_t pIndex) |
Returns a description of the nth import file format. More... | |
ASSIMP_API void | aiGetMemoryRequirements (const aiScene *pIn, aiMemoryInfo *in) |
Get the approximated storage required by an imported asset. More... | |
ASSIMP_API aiLogStream | aiGetPredefinedLogStream (aiDefaultLogStream pStreams, const char *file) |
Get one of the predefine log streams. More... | |
ASSIMP_API void | aiIdentityMatrix3 (aiMatrix3x3 *mat) |
Get a 3x3 identity matrix. More... | |
ASSIMP_API void | aiIdentityMatrix4 (aiMatrix4x4 *mat) |
Get a 4x4 identity matrix. More... | |
ASSIMP_API const aiScene * | aiImportFile (const char *pFile, unsigned int pFlags) |
Reads the given file and returns its content. More... | |
ASSIMP_API const aiScene * | aiImportFileEx (const char *pFile, unsigned int pFlags, aiFileIO *pFS) |
Reads the given file using user-defined I/O functions and returns its content. More... | |
ASSIMP_API const aiScene * | aiImportFileExWithProperties (const char *pFile, unsigned int pFlags, aiFileIO *pFS, const aiPropertyStore *pProps) |
Same as aiImportFileEx, but adds an extra parameter containing importer settings. More... | |
ASSIMP_API const aiScene * | aiImportFileFromMemory (const char *pBuffer, unsigned int pLength, unsigned int pFlags, const char *pHint) |
Reads the given file from a given memory buffer,. More... | |
ASSIMP_API const aiScene * | aiImportFileFromMemoryWithProperties (const char *pBuffer, unsigned int pLength, unsigned int pFlags, const char *pHint, const aiPropertyStore *pProps) |
Same as aiImportFileFromMemory, but adds an extra parameter containing importer settings. More... | |
ASSIMP_API aiBool | aiIsExtensionSupported (const char *szExtension) |
Returns whether a given file extension is supported by ASSIMP. More... | |
ASSIMP_API void | aiMultiplyMatrix3 (aiMatrix3x3 *dst, const aiMatrix3x3 *src) |
Multiply two 3x3 matrices. More... | |
ASSIMP_API void | aiMultiplyMatrix4 (aiMatrix4x4 *dst, const aiMatrix4x4 *src) |
Multiply two 4x4 matrices. More... | |
ASSIMP_API void | aiReleaseImport (const aiScene *pScene) |
Releases all resources associated with the given import process. More... | |
ASSIMP_API void | aiReleasePropertyStore (aiPropertyStore *p) |
Delete a property store. More... | |
ASSIMP_API void | aiSetImportPropertyFloat (aiPropertyStore *store, const char *szName, float value) |
Set a floating-point property. More... | |
ASSIMP_API void | aiSetImportPropertyInteger (aiPropertyStore *store, const char *szName, int value) |
Set an integer property. More... | |
ASSIMP_API void | aiSetImportPropertyMatrix (aiPropertyStore *store, const char *szName, const aiMatrix4x4 *mat) |
Set a matrix property. More... | |
ASSIMP_API void | aiSetImportPropertyString (aiPropertyStore *store, const char *szName, const aiString *st) |
Set a string property. More... | |
ASSIMP_API void | aiTransformVecByMatrix3 (aiVector3D *vec, const aiMatrix3x3 *mat) |
Transform a vector by a 3x3 matrix. More... | |
ASSIMP_API void | aiTransformVecByMatrix4 (aiVector3D *vec, const aiMatrix4x4 *mat) |
Transform a vector by a 4x4 matrix. More... | |
ASSIMP_API void | aiTransposeMatrix3 (aiMatrix3x3 *mat) |
Transpose a 3x3 matrix. More... | |
ASSIMP_API void | aiTransposeMatrix4 (aiMatrix4x4 *mat) |
Transpose a 4x4 matrix. More... | |
Detailed Description
Defines the C-API to the Open Asset Import Library.
Macro Definition Documentation
#define AI_FALSE 0 |
#define AI_TRUE 1 |
Typedef Documentation
typedef int aiBool |
Our own C boolean type.
typedef void(* aiLogStreamCallback) (const char *, char *) |
Function Documentation
ASSIMP_API const aiScene* aiApplyPostProcessing | ( | const aiScene * | pScene, |
unsigned int | pFlags | ||
) |
Apply post-processing to an already-imported scene.
This is strictly equivalent to calling aiImportFile()/aiImportFileEx with the same flags. However, you can use this separate function to inspect the imported scene first to fine-tune your post-processing setup.
- Parameters
-
pScene Scene to work on. pFlags Provide a bitwise combination of the aiPostProcessSteps flags.
- Returns
- A pointer to the post-processed data. Post processing is done in-place, meaning this is still the same aiScene which you passed for pScene. However, if post-processing failed, the scene could now be NULL. That's quite a rare case, post processing steps are not really designed to 'fail'. To be exact, the aiProcess_ValidateDataStructure flag is currently the only post processing step which can actually cause the scene to be reset to NULL.
ASSIMP_API void aiAttachLogStream | ( | const aiLogStream * | stream | ) |
Attach a custom log stream to the libraries' logging system.
Attaching a log stream can slightly reduce Assimp's overall import performance. Multiple log-streams can be attached.
- Parameters
-
stream Describes the new log stream.
- Note
- To ensure proper destruction of the logging system, you need to manually call aiDetachLogStream() on every single log stream you attach. Alternatively (for the lazy folks) aiDetachAllLogStreams is provided.
ASSIMP_API aiPropertyStore* aiCreatePropertyStore | ( | void | ) |
Create an empty property store.
Property stores are used to collect import settings.
- Returns
- New property store. Property stores need to be manually destroyed using the aiReleasePropertyStore API function.
ASSIMP_API void aiCreateQuaternionFromMatrix | ( | aiQuaternion * | quat, |
const aiMatrix3x3 * | mat | ||
) |
Construct a quaternion from a 3x3 rotation matrix.
- Parameters
-
quat Receives the output quaternion. mat Matrix to 'quaternionize'.
- See also
- aiQuaternion(const aiMatrix3x3& pRotMatrix)
ASSIMP_API void aiDecomposeMatrix | ( | const aiMatrix4x4 * | mat, |
aiVector3D * | scaling, | ||
aiQuaternion * | rotation, | ||
aiVector3D * | position | ||
) |
Decompose a transformation matrix into its rotational, translational and scaling components.
- Parameters
-
mat Matrix to decompose scaling Receives the scaling component rotation Receives the rotational component position Receives the translational component.
- See also
- aiMatrix4x4::Decompose (aiVector3D&, aiQuaternion&, aiVector3D&) const;
ASSIMP_API void aiDetachAllLogStreams | ( | void | ) |
Detach all active log streams from the libraries' logging system.
This ensures that the logging system is terminated properly and all resources allocated by it are actually freed. If you attached a stream, don't forget to detach it again.
- See also
- aiAttachLogStream
- aiDetachLogStream
ASSIMP_API aiReturn aiDetachLogStream | ( | const aiLogStream * | stream | ) |
Detach a custom log stream from the libraries' logging system.
This is the counterpart of aiAttachLogStream. If you attached a stream, don't forget to detach it again.
- Parameters
-
stream The log stream to be detached.
- Returns
- AI_SUCCESS if the log stream has been detached successfully.
- See also
- aiDetachAllLogStreams
ASSIMP_API void aiEnableVerboseLogging | ( | aiBool | d | ) |
Enable verbose logging.
Verbose logging includes debug-related stuff and detailed import statistics. This can have severe impact on import performance and memory consumption. However, it might be useful to find out why a file didn't read correctly.
- Parameters
-
d AI_TRUE or AI_FALSE, your decision.
ASSIMP_API const char* aiGetErrorString | ( | ) |
Returns the error text of the last failed import process.
- Returns
- A textual description of the error that occurred at the last import process. NULL if there was no error. There can't be an error if you got a non-NULL aiScene from aiImportFile/aiImportFileEx/aiApplyPostProcessing.
ASSIMP_API void aiGetExtensionList | ( | aiString * | szOut | ) |
Get a list of all file extensions supported by ASSIMP.
If a file extension is contained in the list this does, of course, not mean that ASSIMP is able to load all files with this extension.
- Parameters
-
szOut String to receive the extension list. Format of the list: "*.3ds;*.obj;*.dae". NULL is not a valid parameter.
ASSIMP_API size_t aiGetImportFormatCount | ( | void | ) |
Returns the number of import file formats available in the current Assimp build.
Use aiGetImportFormatDescription() to retrieve infos of a specific import format.
ASSIMP_API const aiImporterDesc* aiGetImportFormatDescription | ( | size_t | pIndex | ) |
Returns a description of the nth import file format.
Use aiGetImportFormatCount() to learn how many import formats are supported.
- Parameters
-
pIndex Index of the import format to retrieve information for. Valid range is 0 to aiGetImportFormatCount()
- Returns
- A description of that specific import format. NULL if pIndex is out of range.
ASSIMP_API void aiGetMemoryRequirements | ( | const aiScene * | pIn, |
aiMemoryInfo * | in | ||
) |
Get the approximated storage required by an imported asset.
- Parameters
-
pIn Input asset. in Data structure to be filled.
ASSIMP_API aiLogStream aiGetPredefinedLogStream | ( | aiDefaultLogStream | pStreams, |
const char * | file | ||
) |
Get one of the predefine log streams.
This is the quick'n'easy solution to access Assimp's log system. Attaching a log stream can slightly reduce Assimp's overall import performance.
Usage is rather simple (this will stream the log to a file, named log.txt, and the stdout stream of the process:
- Parameters
-
pStreams One of the aiDefaultLogStream enumerated values. file Solely for the aiDefaultLogStream_FILE flag: specifies the file to write to. Pass NULL for all other flags.
- Returns
- The log stream. callback is set to NULL if something went wrong.
ASSIMP_API void aiIdentityMatrix3 | ( | aiMatrix3x3 * | mat | ) |
Get a 3x3 identity matrix.
- Parameters
-
mat Matrix to receive its personal identity
ASSIMP_API void aiIdentityMatrix4 | ( | aiMatrix4x4 * | mat | ) |
Get a 4x4 identity matrix.
- Parameters
-
mat Matrix to receive its personal identity
ASSIMP_API const aiScene* aiImportFile | ( | const char * | pFile, |
unsigned int | pFlags | ||
) |
Reads the given file and returns its content.
If the call succeeds, the imported data is returned in an aiScene structure. The data is intended to be read-only, it stays property of the ASSIMP library and will be stable until aiReleaseImport() is called. After you're done with it, call aiReleaseImport() to free the resources associated with this file. If the import fails, NULL is returned instead. Call aiGetErrorString() to retrieve a human-readable error text.
- Parameters
-
pFile Path and filename of the file to be imported, expected to be a null-terminated c-string. NULL is not a valid value. pFlags Optional post processing steps to be executed after a successful import. Provide a bitwise combination of the aiPostProcessSteps flags.
- Returns
- Pointer to the imported data or NULL if the import failed.
ASSIMP_API const aiScene* aiImportFileEx | ( | const char * | pFile, |
unsigned int | pFlags, | ||
aiFileIO * | pFS | ||
) |
Reads the given file using user-defined I/O functions and returns its content.
If the call succeeds, the imported data is returned in an aiScene structure. The data is intended to be read-only, it stays property of the ASSIMP library and will be stable until aiReleaseImport() is called. After you're done with it, call aiReleaseImport() to free the resources associated with this file. If the import fails, NULL is returned instead. Call aiGetErrorString() to retrieve a human-readable error text.
- Parameters
-
pFile Path and filename of the file to be imported, expected to be a null-terminated c-string. NULL is not a valid value. pFlags Optional post processing steps to be executed after a successful import. Provide a bitwise combination of the aiPostProcessSteps flags. pFS aiFileIO structure. Will be used to open the model file itself and any other files the loader needs to open. Pass NULL to use the default implementation.
- Returns
- Pointer to the imported data or NULL if the import failed.
- Note
- Include <aiFileIO.h> for the definition of aiFileIO.
ASSIMP_API const aiScene* aiImportFileExWithProperties | ( | const char * | pFile, |
unsigned int | pFlags, | ||
aiFileIO * | pFS, | ||
const aiPropertyStore * | pProps | ||
) |
Same as aiImportFileEx, but adds an extra parameter containing importer settings.
- Parameters
-
pFile Path and filename of the file to be imported, expected to be a null-terminated c-string. NULL is not a valid value. pFlags Optional post processing steps to be executed after a successful import. Provide a bitwise combination of the aiPostProcessSteps flags. pFS aiFileIO structure. Will be used to open the model file itself and any other files the loader needs to open. Pass NULL to use the default implementation. pProps aiPropertyStore instance containing import settings.
- Returns
- Pointer to the imported data or NULL if the import failed.
- Note
- Include <aiFileIO.h> for the definition of aiFileIO.
- See also
- aiImportFileEx
ASSIMP_API const aiScene* aiImportFileFromMemory | ( | const char * | pBuffer, |
unsigned int | pLength, | ||
unsigned int | pFlags, | ||
const char * | pHint | ||
) |
Reads the given file from a given memory buffer,.
If the call succeeds, the contents of the file are returned as a pointer to an aiScene object. The returned data is intended to be read-only, the importer keeps ownership of the data and will destroy it upon destruction. If the import fails, NULL is returned. A human-readable error description can be retrieved by calling aiGetErrorString().
- Parameters
-
pBuffer Pointer to the file data pLength Length of pBuffer, in bytes pFlags Optional post processing steps to be executed after a successful import. Provide a bitwise combination of the aiPostProcessSteps flags. If you wish to inspect the imported scene first in order to fine-tune your post-processing setup, consider to use aiApplyPostProcessing(). pHint An additional hint to the library. If this is a non empty string, the library looks for a loader to support the file extension specified by pHint and passes the file to the first matching loader. If this loader is unable to completely the request, the library continues and tries to determine the file format on its own, a task that may or may not be successful. Check the return value, and you'll know ...
- Returns
- A pointer to the imported data, NULL if the import failed.
- Note
- This is a straightforward way to decode models from memory buffers, but it doesn't handle model formats that spread their data across multiple files or even directories. Examples include OBJ or MD3, which outsource parts of their material info into external scripts. If you need full functionality, provide a custom IOSystem to make Assimp find these files and use the regular aiImportFileEx()/aiImportFileExWithProperties() API.
ASSIMP_API const aiScene* aiImportFileFromMemoryWithProperties | ( | const char * | pBuffer, |
unsigned int | pLength, | ||
unsigned int | pFlags, | ||
const char * | pHint, | ||
const aiPropertyStore * | pProps | ||
) |
Same as aiImportFileFromMemory, but adds an extra parameter containing importer settings.
- Parameters
-
pBuffer Pointer to the file data pLength Length of pBuffer, in bytes pFlags Optional post processing steps to be executed after a successful import. Provide a bitwise combination of the aiPostProcessSteps flags. If you wish to inspect the imported scene first in order to fine-tune your post-processing setup, consider to use aiApplyPostProcessing(). pHint An additional hint to the library. If this is a non empty string, the library looks for a loader to support the file extension specified by pHint and passes the file to the first matching loader. If this loader is unable to completely the request, the library continues and tries to determine the file format on its own, a task that may or may not be successful. Check the return value, and you'll know ... pProps aiPropertyStore instance containing import settings.
- Returns
- A pointer to the imported data, NULL if the import failed.
- Note
- This is a straightforward way to decode models from memory buffers, but it doesn't handle model formats that spread their data across multiple files or even directories. Examples include OBJ or MD3, which outsource parts of their material info into external scripts. If you need full functionality, provide a custom IOSystem to make Assimp find these files and use the regular aiImportFileEx()/aiImportFileExWithProperties() API.
- See also
- aiImportFileFromMemory
ASSIMP_API aiBool aiIsExtensionSupported | ( | const char * | szExtension | ) |
Returns whether a given file extension is supported by ASSIMP.
- Parameters
-
szExtension Extension for which the function queries support for. Must include a leading dot '.'. Example: ".3ds", ".md3"
- Returns
- AI_TRUE if the file extension is supported.
ASSIMP_API void aiMultiplyMatrix3 | ( | aiMatrix3x3 * | dst, |
const aiMatrix3x3 * | src | ||
) |
Multiply two 3x3 matrices.
- Parameters
-
dst First factor, receives result. src Matrix to be multiplied with 'dst'.
ASSIMP_API void aiMultiplyMatrix4 | ( | aiMatrix4x4 * | dst, |
const aiMatrix4x4 * | src | ||
) |
Multiply two 4x4 matrices.
- Parameters
-
dst First factor, receives result. src Matrix to be multiplied with 'dst'.
ASSIMP_API void aiReleaseImport | ( | const aiScene * | pScene | ) |
Releases all resources associated with the given import process.
Call this function after you're done with the imported data.
- Parameters
-
pScene The imported data to release. NULL is a valid value.
ASSIMP_API void aiReleasePropertyStore | ( | aiPropertyStore * | p | ) |
Delete a property store.
- Parameters
-
p Property store to be deleted.
ASSIMP_API void aiSetImportPropertyFloat | ( | aiPropertyStore * | store, |
const char * | szName, | ||
float | value | ||
) |
Set a floating-point property.
This is the C-version of Assimp::Importer::SetPropertyFloat(). In the C interface, properties are always shared by all imports. It is not possible to specify them per import.
- Parameters
-
store Store to modify. Use aiCreatePropertyStore to obtain a store. szName Name of the configuration property to be set. All supported public properties are defined in the config.h header file (AI_CONFIG_XXX). value New value for the property
ASSIMP_API void aiSetImportPropertyInteger | ( | aiPropertyStore * | store, |
const char * | szName, | ||
int | value | ||
) |
Set an integer property.
This is the C-version of Assimp::Importer::SetPropertyInteger(). In the C interface, properties are always shared by all imports. It is not possible to specify them per import.
- Parameters
-
store Store to modify. Use aiCreatePropertyStore to obtain a store. szName Name of the configuration property to be set. All supported public properties are defined in the config.h header file (AI_CONFIG_XXX). value New value for the property
ASSIMP_API void aiSetImportPropertyMatrix | ( | aiPropertyStore * | store, |
const char * | szName, | ||
const aiMatrix4x4 * | mat | ||
) |
Set a matrix property.
This is the C-version of Assimp::Importer::SetPropertyMatrix(). In the C interface, properties are always shared by all imports. It is not possible to specify them per import.
- Parameters
-
store Store to modify. Use aiCreatePropertyStore to obtain a store. szName Name of the configuration property to be set. All supported public properties are defined in the config.h header file (AI_CONFIG_XXX). mat New value for the property
ASSIMP_API void aiSetImportPropertyString | ( | aiPropertyStore * | store, |
const char * | szName, | ||
const aiString * | st | ||
) |
Set a string property.
This is the C-version of Assimp::Importer::SetPropertyString(). In the C interface, properties are always shared by all imports. It is not possible to specify them per import.
- Parameters
-
store Store to modify. Use aiCreatePropertyStore to obtain a store. szName Name of the configuration property to be set. All supported public properties are defined in the config.h header file (AI_CONFIG_XXX). st New value for the property
ASSIMP_API void aiTransformVecByMatrix3 | ( | aiVector3D * | vec, |
const aiMatrix3x3 * | mat | ||
) |
Transform a vector by a 3x3 matrix.
- Parameters
-
vec Vector to be transformed. mat Matrix to transform the vector with.
ASSIMP_API void aiTransformVecByMatrix4 | ( | aiVector3D * | vec, |
const aiMatrix4x4 * | mat | ||
) |
Transform a vector by a 4x4 matrix.
- Parameters
-
vec Vector to be transformed. mat Matrix to transform the vector with.
ASSIMP_API void aiTransposeMatrix3 | ( | aiMatrix3x3 * | mat | ) |
Transpose a 3x3 matrix.
- Parameters
-
mat Pointer to the matrix to be transposed
ASSIMP_API void aiTransposeMatrix4 | ( | aiMatrix4x4 * | mat | ) |
Transpose a 4x4 matrix.
- Parameters
-
mat Pointer to the matrix to be transposed
Generated on Sun Feb 21 2016 19:42:29 for Assimp by
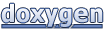