Assimp
v3.1.1 (June 2014)
|
CPP-API: The Importer class forms an C++ interface to the functionality of the Open Asset Import Library. More...
Public Member Functions | |
const aiScene * | ApplyCustomizedPostProcessing (BaseProcess *rootProcess, bool requestValidation) |
const aiScene * | ApplyPostProcessing (unsigned int pFlags) |
Apply post-processing to an already-imported scene. More... | |
void | FreeScene () |
Frees the current scene. More... | |
const char * | GetErrorString () const |
Returns an error description of an error that occurred in ReadFile(). More... | |
void | GetExtensionList (aiString &szOut) const |
Get a full list of all file extensions supported by ASSIMP. More... | |
void | GetExtensionList (std::string &szOut) const |
Get a full list of all file extensions supported by ASSIMP. More... | |
BaseImporter * | GetImporter (size_t index) const |
Find the importer corresponding to a specific index. More... | |
BaseImporter * | GetImporter (const char *szExtension) const |
Find the importer corresponding to a specific file extension. More... | |
size_t | GetImporterCount () const |
Get the number of importrs currently registered with Assimp. More... | |
size_t | GetImporterIndex (const char *szExtension) const |
Find the importer index corresponding to a specific file extension. More... | |
const aiImporterDesc * | GetImporterInfo (size_t index) const |
Get meta data for the importer corresponding to a specific index. More... | |
IOSystem * | GetIOHandler () const |
Retrieves the IO handler that is currently set. More... | |
void | GetMemoryRequirements (aiMemoryInfo &in) const |
Returns the storage allocated by ASSIMP to hold the scene data in memory. More... | |
aiScene * | GetOrphanedScene () |
Returns the scene loaded by the last successful call to ReadFile() and releases the scene from the ownership of the Importer instance. More... | |
ProgressHandler * | GetProgressHandler () const |
Retrieves the progress handler that is currently set. More... | |
bool | GetPropertyBool (const char *szName, bool bErrorReturn=false) const |
Get a boolean configuration property. More... | |
float | GetPropertyFloat (const char *szName, float fErrorReturn=10e10f) const |
Get a floating-point configuration property. More... | |
int | GetPropertyInteger (const char *szName, int iErrorReturn=0xffffffff) const |
Get a configuration property. More... | |
const aiMatrix4x4 | GetPropertyMatrix (const char *szName, const aiMatrix4x4 &sErrorReturn=aiMatrix4x4()) const |
Get a matrix configuration property. More... | |
const std::string | GetPropertyString (const char *szName, const std::string &sErrorReturn="") const |
Get a string configuration property. More... | |
const aiScene * | GetScene () const |
Returns the scene loaded by the last successful call to ReadFile() More... | |
Importer () | |
Constructor. More... | |
Importer (const Importer &other) | |
Copy constructor. More... | |
bool | IsDefaultIOHandler () const |
Checks whether a default IO handler is active A default handler is active as long the application doesn't supply its own custom IO handler via SetIOHandler(). More... | |
bool | IsDefaultProgressHandler () const |
Checks whether a default progress handler is active A default handler is active as long the application doesn't supply its own custom progress handler via SetProgressHandler(). More... | |
bool | IsExtensionSupported (const char *szExtension) const |
Returns whether a given file extension is supported by ASSIMP. More... | |
bool | IsExtensionSupported (const std::string &szExtension) const |
Returns whether a given file extension is supported by ASSIMP. More... | |
ImporterPimpl * | Pimpl () |
Private, do not use. More... | |
const ImporterPimpl * | Pimpl () const |
const aiScene * | ReadFile (const char *pFile, unsigned int pFlags) |
Reads the given file and returns its contents if successful. More... | |
const aiScene * | ReadFile (const std::string &pFile, unsigned int pFlags) |
Reads the given file and returns its contents if successful. More... | |
const aiScene * | ReadFileFromMemory (const void *pBuffer, size_t pLength, unsigned int pFlags, const char *pHint="") |
Reads the given file from a memory buffer and returns its contents if successful. More... | |
aiReturn | RegisterLoader (BaseImporter *pImp) |
Registers a new loader. More... | |
aiReturn | RegisterPPStep (BaseProcess *pImp) |
Registers a new post-process step. More... | |
void | SetExtraVerbose (bool bDo) |
Enables "extra verbose" mode. More... | |
void | SetIOHandler (IOSystem *pIOHandler) |
Supplies a custom IO handler to the importer to use to open and access files. More... | |
void | SetProgressHandler (ProgressHandler *pHandler) |
Supplies a custom progress handler to the importer. More... | |
bool | SetPropertyBool (const char *szName, bool value) |
Set a boolean configuration property. More... | |
bool | SetPropertyFloat (const char *szName, float fValue) |
Set a floating-point configuration property. More... | |
bool | SetPropertyInteger (const char *szName, int iValue) |
Set an integer configuration property. More... | |
bool | SetPropertyMatrix (const char *szName, const aiMatrix4x4 &sValue) |
Set a matrix configuration property. More... | |
bool | SetPropertyString (const char *szName, const std::string &sValue) |
Set a string configuration property. More... | |
aiReturn | UnregisterLoader (BaseImporter *pImp) |
Unregisters a loader. More... | |
aiReturn | UnregisterPPStep (BaseProcess *pImp) |
Unregisters a post-process step. More... | |
bool | ValidateFlags (unsigned int pFlags) const |
Check whether a given set of postprocessing flags is supported. More... | |
~Importer () | |
Destructor. More... | |
Static Public Attributes | |
static const unsigned int | MaxLenHint = 200 |
The upper limit for hints. More... | |
Protected Attributes | |
ImporterPimpl * | pimpl |
Detailed Description
CPP-API: The Importer class forms an C++ interface to the functionality of the Open Asset Import Library.
Create an object of this class and call ReadFile() to import a file. If the import succeeds, the function returns a pointer to the imported data. The data remains property of the object, it is intended to be accessed read-only. The imported data will be destroyed along with the Importer object. If the import fails, ReadFile() returns a NULL pointer. In this case you can retrieve a human-readable error description be calling GetErrorString(). You can call ReadFile() multiple times with a single Importer instance. Actually, constructing Importer objects involves quite many allocations and may take some time, so it's better to reuse them as often as possible.
If you need the Importer to do custom file handling to access the files, implement IOSystem and IOStream and supply an instance of your custom IOSystem implementation by calling SetIOHandler() before calling ReadFile(). If you do not assign a custion IO handler, a default handler using the standard C++ IO logic will be used.
Constructor & Destructor Documentation
Assimp::Importer::Importer | ( | ) |
Constructor.
Creates an empty importer object.
Call ReadFile() to start the import process. The configuration property table is initially empty.
Assimp::Importer::Importer | ( | const Importer & | other | ) |
Copy constructor.
This copies the configuration properties of another Importer. If this Importer owns a scene it won't be copied. Call ReadFile() to start the import process.
Assimp::Importer::~Importer | ( | ) |
Destructor.
The object kept ownership of the imported data, which now will be destroyed along with the object.
Member Function Documentation
const aiScene* Assimp::Importer::ApplyCustomizedPostProcessing | ( | BaseProcess * | rootProcess, |
bool | requestValidation | ||
) |
const aiScene* Assimp::Importer::ApplyPostProcessing | ( | unsigned int | pFlags | ) |
Apply post-processing to an already-imported scene.
This is strictly equivalent to calling ReadFile() with the same flags. However, you can use this separate function to inspect the imported scene first to fine-tune your post-processing setup.
- Parameters
-
pFlags Provide a bitwise combination of the aiPostProcessSteps flags.
- Returns
- A pointer to the post-processed data. This is still the same as the pointer returned by ReadFile(). However, if post-processing fails, the scene could now be NULL. That's quite a rare case, post processing steps are not really designed to 'fail'. To be exact, the #aiProcess_ValidateDS flag is currently the only post processing step which can actually cause the scene to be reset to NULL.
- Note
- The method does nothing if no scene is currently bound to the Importer instance.
void Assimp::Importer::FreeScene | ( | ) |
Frees the current scene.
The function does nothing if no scene has previously been read via ReadFile(). FreeScene() is called automatically by the destructor and ReadFile() itself.
const char* Assimp::Importer::GetErrorString | ( | ) | const |
Returns an error description of an error that occurred in ReadFile().
Returns an empty string if no error occurred.
- Returns
- A description of the last error, an empty string if no error occurred. The string is never NULL.
- Note
- The returned function remains valid until one of the following methods is called: ReadFile(), FreeScene().
void Assimp::Importer::GetExtensionList | ( | aiString & | szOut | ) | const |
Get a full list of all file extensions supported by ASSIMP.
If a file extension is contained in the list this does of course not mean that ASSIMP is able to load all files with this extension — it simply means there is an importer loaded which claims to handle files with this file extension.
- Parameters
-
szOut String to receive the extension list. Format of the list: "*.3ds;*.obj;*.dae". This is useful for use with the WinAPI call GetOpenFileName(Ex).
|
inline |
Get a full list of all file extensions supported by ASSIMP.
This function is provided for backward compatibility. See the aiString version for detailed and up-to-date docs.
- See also
- GetExtensionList(aiString&)
BaseImporter* Assimp::Importer::GetImporter | ( | size_t | index | ) | const |
Find the importer corresponding to a specific index.
- Parameters
-
index Index to query, must be within [0,GetImporterCount())
- Returns
- Importer instance. NULL if the index does not exist.
BaseImporter* Assimp::Importer::GetImporter | ( | const char * | szExtension | ) | const |
Find the importer corresponding to a specific file extension.
This is quite similar to IsExtensionSupported except a BaseImporter instance is returned.
- Parameters
-
szExtension Extension to check for. The following formats are recognized (BAH being the file extension): "BAH" (comparison is case-insensitive), ".bah", "*.bah" (wild card and dot characters at the beginning of the extension are skipped).
- Returns
- NULL if no importer is found
size_t Assimp::Importer::GetImporterCount | ( | ) | const |
Get the number of importrs currently registered with Assimp.
size_t Assimp::Importer::GetImporterIndex | ( | const char * | szExtension | ) | const |
Find the importer index corresponding to a specific file extension.
- Parameters
-
szExtension Extension to check for. The following formats are recognized (BAH being the file extension): "BAH" (comparison is case-insensitive), ".bah", "*.bah" (wild card and dot characters at the beginning of the extension are skipped).
- Returns
- (size_t)-1 if no importer is found
const aiImporterDesc* Assimp::Importer::GetImporterInfo | ( | size_t | index | ) | const |
Get meta data for the importer corresponding to a specific index.
For the declaration of aiImporterDesc, include <assimp/importerdesc.h>.
- Parameters
-
index Index to query, must be within [0,GetImporterCount())
- Returns
- Importer meta data structure, NULL if the index does not exist or if the importer doesn't offer meta information ( importers may do this at the cost of being hated by their peers).
IOSystem* Assimp::Importer::GetIOHandler | ( | ) | const |
Retrieves the IO handler that is currently set.
You can use IsDefaultIOHandler() to check whether the returned interface is the default IO handler provided by ASSIMP. The default handler is active as long the application doesn't supply its own custom IO handler via SetIOHandler().
- Returns
- A valid IOSystem interface, never NULL.
void Assimp::Importer::GetMemoryRequirements | ( | aiMemoryInfo & | in | ) | const |
Returns the storage allocated by ASSIMP to hold the scene data in memory.
This refers to the currently loaded file, see ReadFile().
- Parameters
-
in Data structure to be filled.
- Note
- The returned memory statistics refer to the actual size of the use data of the aiScene. Heap-related overhead is (naturally) not included.
aiScene* Assimp::Importer::GetOrphanedScene | ( | ) |
Returns the scene loaded by the last successful call to ReadFile() and releases the scene from the ownership of the Importer instance.
The application is now responsible for deleting the scene. Any further calls to GetScene() or GetOrphanedScene() will return NULL - until a new scene has been loaded via ReadFile().
- Returns
- Current scene or NULL if there is currently no scene loaded
- Note
- Use this method with maximal caution, and only if you have to. By design, aiScene's are exclusively maintained, allocated and deallocated by Assimp and no one else. The reasoning behind this is the golden rule that deallocations should always be done by the module that did the original allocation because heaps are not necessarily shared. GetOrphanedScene() enforces you to delete the returned scene by yourself, but this will only be fine if and only if you're using the same heap as assimp. On Windows, it's typically fine provided everything is linked against the multithreaded-dll version of the runtime library. It will work as well for static linkage with Assimp.
ProgressHandler* Assimp::Importer::GetProgressHandler | ( | ) | const |
Retrieves the progress handler that is currently set.
You can use IsDefaultProgressHandler() to check whether the returned interface is the default handler provided by ASSIMP. The default handler is active as long the application doesn't supply its own custom handler via SetProgressHandler().
- Returns
- A valid ProgressHandler interface, never NULL.
|
inline |
Get a boolean configuration property.
Boolean properties are stored on the integer stack internally so it's possible to set them via SetPropertyBool and query them with GetPropertyBool and vice versa.
- See also
- GetPropertyInteger()
float Assimp::Importer::GetPropertyFloat | ( | const char * | szName, |
float | fErrorReturn = 10e10f |
||
) | const |
Get a floating-point configuration property.
- See also
- GetPropertyInteger()
int Assimp::Importer::GetPropertyInteger | ( | const char * | szName, |
int | iErrorReturn = 0xffffffff |
||
) | const |
Get a configuration property.
- Parameters
-
szName Name of the property. All supported properties are defined in the aiConfig.g header (all constants share the prefix AI_CONFIG_XXX). iErrorReturn Value that is returned if the property is not found.
- Returns
- Current value of the property
- Note
- Property of different types (float, int, string ..) are kept on different lists, so calling SetPropertyInteger() for a floating-point property has no effect - the loader will call GetPropertyFloat() to read the property, but it won't be there.
const aiMatrix4x4 Assimp::Importer::GetPropertyMatrix | ( | const char * | szName, |
const aiMatrix4x4 & | sErrorReturn = aiMatrix4x4() |
||
) | const |
Get a matrix configuration property.
The return value remains valid until the property is modified.
- See also
- GetPropertyInteger()
const std::string Assimp::Importer::GetPropertyString | ( | const char * | szName, |
const std::string & | sErrorReturn = "" |
||
) | const |
Get a string configuration property.
The return value remains valid until the property is modified.
- See also
- GetPropertyInteger()
const aiScene* Assimp::Importer::GetScene | ( | ) | const |
Returns the scene loaded by the last successful call to ReadFile()
- Returns
- Current scene or NULL if there is currently no scene loaded
bool Assimp::Importer::IsDefaultIOHandler | ( | ) | const |
Checks whether a default IO handler is active A default handler is active as long the application doesn't supply its own custom IO handler via SetIOHandler().
- Returns
- true by default
bool Assimp::Importer::IsDefaultProgressHandler | ( | ) | const |
Checks whether a default progress handler is active A default handler is active as long the application doesn't supply its own custom progress handler via SetProgressHandler().
- Returns
- true by default
bool Assimp::Importer::IsExtensionSupported | ( | const char * | szExtension | ) | const |
Returns whether a given file extension is supported by ASSIMP.
- Parameters
-
szExtension Extension to be checked. Must include a trailing dot '.'. Example: ".3ds", ".md3". Cases-insensitive.
- Returns
- true if the extension is supported, false otherwise
|
inline |
Returns whether a given file extension is supported by ASSIMP.
This function is provided for backward compatibility. See the const char* version for detailed and up-to-date docs.
- See also
- IsExtensionSupported(const char*)
|
inline |
Private, do not use.
|
inline |
const aiScene* Assimp::Importer::ReadFile | ( | const char * | pFile, |
unsigned int | pFlags | ||
) |
Reads the given file and returns its contents if successful.
If the call succeeds, the contents of the file are returned as a pointer to an aiScene object. The returned data is intended to be read-only, the importer object keeps ownership of the data and will destroy it upon destruction. If the import fails, NULL is returned. A human-readable error description can be retrieved by calling GetErrorString(). The previous scene will be deleted during this call.
- Parameters
-
pFile Path and filename to the file to be imported. pFlags Optional post processing steps to be executed after a successful import. Provide a bitwise combination of the aiPostProcessSteps flags. If you wish to inspect the imported scene first in order to fine-tune your post-processing setup, consider to use ApplyPostProcessing().
- Returns
- A pointer to the imported data, NULL if the import failed. The pointer to the scene remains in possession of the Importer instance. Use GetOrphanedScene() to take ownership of it.
- Note
- Assimp is able to determine the file format of a file automatically.
AI_FORCE_INLINE const aiScene * Assimp::Importer::ReadFile | ( | const std::string & | pFile, |
unsigned int | pFlags | ||
) |
Reads the given file and returns its contents if successful.
class Importer
This function is provided for backward compatibility. See the const char* version for detailled docs.
- See also
- ReadFile(const char*, pFlags)
const aiScene* Assimp::Importer::ReadFileFromMemory | ( | const void * | pBuffer, |
size_t | pLength, | ||
unsigned int | pFlags, | ||
const char * | pHint = "" |
||
) |
Reads the given file from a memory buffer and returns its contents if successful.
If the call succeeds, the contents of the file are returned as a pointer to an aiScene object. The returned data is intended to be read-only, the importer object keeps ownership of the data and will destroy it upon destruction. If the import fails, NULL is returned. A human-readable error description can be retrieved by calling GetErrorString(). The previous scene will be deleted during this call. Calling this method doesn't affect the active IOSystem.
- Parameters
-
pBuffer Pointer to the file data pLength Length of pBuffer, in bytes pFlags Optional post processing steps to be executed after a successful import. Provide a bitwise combination of the aiPostProcessSteps flags. If you wish to inspect the imported scene first in order to fine-tune your post-processing setup, consider to use ApplyPostProcessing(). pHint An additional hint to the library. If this is a non empty string, the library looks for a loader to support the file extension specified by pHint and passes the file to the first matching loader. If this loader is unable to completely the request, the library continues and tries to determine the file format on its own, a task that may or may not be successful. Check the return value, and you'll know ...
- Returns
- A pointer to the imported data, NULL if the import failed. The pointer to the scene remains in possession of the Importer instance. Use GetOrphanedScene() to take ownership of it.
- Note
- This is a straightforward way to decode models from memory buffers, but it doesn't handle model formats that spread their data across multiple files or even directories. Examples include OBJ or MD3, which outsource parts of their material info into external scripts. If you need full functionality, provide a custom IOSystem to make Assimp find these files and use the regular ReadFile() API.
aiReturn Assimp::Importer::RegisterLoader | ( | BaseImporter * | pImp | ) |
Registers a new loader.
- Parameters
-
pImp Importer to be added. The Importer instance takes ownership of the pointer, so it will be automatically deleted with the Importer instance.
- Returns
- AI_SUCCESS if the loader has been added. The registration fails if there is already a loader for a specific file extension.
aiReturn Assimp::Importer::RegisterPPStep | ( | BaseProcess * | pImp | ) |
Registers a new post-process step.
At the moment, there's a small limitation: new post processing steps are added to end of the list, or in other words, executed last, after all built-in steps.
- Parameters
-
pImp Post-process step to be added. The Importer instance takes ownership of the pointer, so it will be automatically deleted with the Importer instance.
- Returns
- AI_SUCCESS if the step has been added correctly.
void Assimp::Importer::SetExtraVerbose | ( | bool | bDo | ) |
Enables "extra verbose" mode.
'Extra verbose' means the data structure is validated after every single post processing step to make sure everyone modifies the data structure in a well-defined manner. This is a debug feature and not intended for use in production environments.
void Assimp::Importer::SetIOHandler | ( | IOSystem * | pIOHandler | ) |
Supplies a custom IO handler to the importer to use to open and access files.
If you need the importer to use custion IO logic to access the files, you need to provide a custom implementation of IOSystem and IOFile to the importer. Then create an instance of your custion IOSystem implementation and supply it by this function.
The Importer takes ownership of the object and will destroy it afterwards. The previously assigned handler will be deleted. Pass NULL to take again ownership of your IOSystem and reset Assimp to use its default implementation.
- Parameters
-
pIOHandler The IO handler to be used in all file accesses of the Importer.
void Assimp::Importer::SetProgressHandler | ( | ProgressHandler * | pHandler | ) |
Supplies a custom progress handler to the importer.
This interface exposes a #Update() callback, which is called more or less periodically (please don't sue us if it isn't as periodically as you'd like it to have ...). This can be used to implement progress bars and loading timeouts.
- Parameters
-
pHandler Progress callback interface. Pass NULL to disable progress reporting.
- Note
- Progress handlers can be used to abort the loading at almost any time.
|
inline |
Set a boolean configuration property.
Boolean properties are stored on the integer stack internally so it's possible to set them via SetPropertyBool and query them with GetPropertyBool and vice versa.
- See also
- SetPropertyInteger()
bool Assimp::Importer::SetPropertyFloat | ( | const char * | szName, |
float | fValue | ||
) |
Set a floating-point configuration property.
- See also
- SetPropertyInteger()
bool Assimp::Importer::SetPropertyInteger | ( | const char * | szName, |
int | iValue | ||
) |
Set an integer configuration property.
- Parameters
-
szName Name of the property. All supported properties are defined in the aiConfig.g header (all constants share the prefix AI_CONFIG_XXX and are simple strings). iValue New value of the property
- Returns
- true if the property was set before. The new value replaces the previous value in this case.
- Note
- Property of different types (float, int, string ..) are kept on different stacks, so calling SetPropertyInteger() for a floating-point property has no effect - the loader will call GetPropertyFloat() to read the property, but it won't be there.
bool Assimp::Importer::SetPropertyMatrix | ( | const char * | szName, |
const aiMatrix4x4 & | sValue | ||
) |
Set a matrix configuration property.
- See also
- SetPropertyInteger()
bool Assimp::Importer::SetPropertyString | ( | const char * | szName, |
const std::string & | sValue | ||
) |
Set a string configuration property.
- See also
- SetPropertyInteger()
aiReturn Assimp::Importer::UnregisterLoader | ( | BaseImporter * | pImp | ) |
aiReturn Assimp::Importer::UnregisterPPStep | ( | BaseProcess * | pImp | ) |
Unregisters a post-process step.
- Parameters
-
pImp Step to be unregistered.
- Returns
- AI_SUCCESS if the step has been removed. The function fails if the step is currently in use (this could happen if the Importer instance is used by more than one thread) or if it has not yet been registered.
bool Assimp::Importer::ValidateFlags | ( | unsigned int | pFlags | ) | const |
Check whether a given set of postprocessing flags is supported.
Some flags are mutually exclusive, others are probably not available because your excluded them from your Assimp builds. Calling this function is recommended if you're unsure.
- Parameters
-
pFlags Bitwise combination of the aiPostProcess flags.
- Returns
- true if this flag combination is fine.
Member Data Documentation
|
static |
The upper limit for hints.
|
protected |
The documentation for this class was generated from the following file:
Generated on Sun Feb 21 2016 19:42:29 for Assimp by
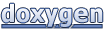