Assimp
v3.1.1 (June 2014)
|
Overview
All materials are stored in an array of aiMaterial inside the aiScene.
Each aiMesh refers to one material by its index in the array. Due to the vastly diverging definitions and usages of material parameters there is no hard definition of a material structure. Instead a material is defined by a set of properties accessible by their names. Have a look at assimp/material.h to see what types of properties are defined. In this file there are also various functions defined to test for the presence of certain properties in a material and retrieve their values.
Textures
Textures are organized in stacks, each stack being evaluated independently. The final color value from a particular texture stack is used in the shading equation. For example, the computed color value of the diffuse texture stack (aiTextureType_DIFFUSE) is multipled with the amount of incoming diffuse light to obtain the final diffuse color of a pixel.
Constants
All material key constants start with 'AI_MATKEY' (it's an ugly macro for historical reasons, don't ask).
Name | Data Type | Default Value | Meaning | Notes |
---|---|---|---|---|
NAME | aiString | n/a | The name of the material, if available. | Ignored by aiProcess_RemoveRedundantMaterials . Materials are considered equal even if their names are different. |
COLOR_DIFFUSE | aiColor3D | black (0,0,0) | Diffuse color of the material. This is typically scaled by the amount of incoming diffuse light (e.g. using gouraud shading) | — |
COLOR_SPECULAR | aiColor3D | black (0,0,0) | Specular color of the material. This is typically scaled by the amount of incoming specular light (e.g. using phong shading) | — |
COLOR_AMBIENT | aiColor3D | black (0,0,0) | Ambient color of the material. This is typically scaled by the amount of ambient light | — |
COLOR_EMISSIVE | aiColor3D | black (0,0,0) | Emissive color of the material. This is the amount of light emitted by the object. In real time applications it will usually not affect surrounding objects, but raytracing applications may wish to treat emissive objects as light sources. | — |
COLOR_TRANSPARENT | aiColor3D | black (0,0,0) | Defines the transparent color of the material, this is the color to be multiplied with the color of translucent light to construct the final 'destination color' for a particular position in the screen buffer. T | — |
WIREFRAME | int | false | Specifies whether wireframe rendering must be turned on for the material. 0 for false, !0 for true. | — |
TWOSIDED | int | false | Specifies whether meshes using this material must be rendered without backface culling. 0 for false, !0 for true. | Some importers set this property if they don't know whether the output face oder is right. As long as it is not set, you may safely enable backface culling. |
SHADING_MODEL | int | gouraud | One of the aiShadingMode enumerated values. Defines the library shading model to use for (real time) rendering to approximate the original look of the material as closely as possible. | The presence of this key might indicate a more complex material. If absent, assume phong shading only if a specular exponent is given. |
BLEND_FUNC | int | false | One of the aiBlendMode enumerated values. Defines how the final color value in the screen buffer is computed from the given color at that position and the newly computed color from the material. Simply said, alpha blending settings. | - |
OPACITY | float | 1.0 | Defines the opacity of the material in a range between 0..1. | Use this value to decide whether you have to activate alpha blending for rendering. |
SHININESS | float | 0.f | Defines the shininess of a phong-shaded material. This is actually the exponent of the phong specular equation |
|
SHININESS_STRENGTH | float | 1.0 | Scales the specular color of the material. | This value is kept separate from the specular color by most modelers, and so do we. |
REFRACTI | float | 1.0 | Defines the Index Of Refraction for the material. That's not supported by most file formats. | Might be of interest for raytracing. |
TEXTURE(t,n) | aiString | n/a | Defines the path to the n'th texture on the stack 't', where 'n' is any value >= 0 and 't' is one of the aiTextureType enumerated values. | See the 'Textures' section above. |
TEXBLEND(t,n) | float | n/a | Defines the strength the n'th texture on the stack 't'. All color components (rgb) are multipled with this factor before any further processing is done. | - |
TEXOP(t,n) | int | n/a | One of the aiTextureOp enumerated values. Defines the arithmetic operation to be used to combine the n'th texture on the stack 't' with the n-1'th. TEXOP(t,0) refers to the blend operation between the base color for this stack (e.g. COLOR_DIFFUSE for the diffuse stack) and the first texture. | - |
MAPPING(t,n) | int | n/a | Defines how the input mapping coordinates for sampling the n'th texture on the stack 't' are computed. Usually explicit UV coordinates are provided, but some model file formats might also be using basic shapes, such as spheres or cylinders, to project textures onto meshes. | See the 'Textures' section below. aiProcess_GenUVCoords can be used to let Assimp compute proper UV coordinates from projective mappings. |
UVWSRC(t,n) | int | n/a | Defines the UV channel to be used as input mapping coordinates for sampling the n'th texture on the stack 't'. All meshes assigned to this material share the same UV channel setup | Presence of this key implies |
MAPPINGMODE_U(t,n) | int | n/a | Any of the aiTextureMapMode enumerated values. Defines the texture wrapping mode on the x axis for sampling the n'th texture on the stack 't'. 'Wrapping' occurs whenever UVs lie outside the 0..1 range. | - |
MAPPINGMODE_V(t,n) | int | n/a | Wrap mode on the v axis. See MAPPINGMODE_U . | - |
TEXMAP_AXIS(t,n) | aiVector3D | n/a | Defines the base axis to to compute the mapping coordinates for the n'th texture on the stack 't' from. This is not required for UV-mapped textures. For instance, if MAPPING(t,n) is aiTextureMapping_SPHERE, U and V would map to longitude and latitude of a sphere around the given axis. The axis is given in local mesh space. | - |
TEXFLAGS(t,n) | int | n/a | Defines miscellaneous flag for the n'th texture on the stack 't'. This is a bitwise combination of the aiTextureFlags enumerated values. | - |
C++-API
Retrieving a property from a material is done using various utility functions. For C++ it's simply calling aiMaterial::Get()
Simple, isn't it? To get the name of a material you would use
Or for the diffuse color ('color' won't be modified if the property is not set)
Note: Get() is actually a template with explicit specializations for aiColor3D, aiColor4D, aiString, float, int and some others. Make sure that the type of the second parameter matches the expected data type of the material property (no compile-time check yet!). Don't follow this advice if you wish to encounter very strange results.
C-API
For good old C it's slightly different. Take a look at the aiGetMaterialGet<data-type> functions.
To get the name of a material you would use
Or for the diffuse color ('color' won't be modified if the property is not set)
How to map UV channels to textures (MATKEY_UVWSRC)
The MATKEY_UVWSRC property is only present if the source format doesn't specify an explicit mapping from textures to UV channels. Many formats don't do this and assimp is not aware of a perfect rule either.
Your handling of UV channels needs to be flexible therefore. Our recommendation is to use logic like this to handle most cases properly:
have only one uv channel? assign channel 0 to all textures and break for all textures have uvwsrc for this texture? assign channel specified in uvwsrc else assign channels in ascending order for all texture stacks, i.e. diffuse1 gets channel 1, opacity0 gets channel 0.
Pseudo Code Listing
For completeness, the following is a very rough pseudo-code sample showing how to evaluate Assimp materials in your shading pipeline. You'll probably want to limit your handling of all those material keys to a reasonable subset suitable for your purposes (for example most 3d engines won't support highly complex multi-layer materials, but many 3d modellers do).
Also note that this sample is targeted at a (shader-based) rendering pipeline for real time graphics.
Generated on Sun Feb 21 2016 19:42:29 for Assimp by
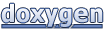