Assimp
v3.1.1 (June 2014)
|
Public Types | |
typedef std::map< KeyType, float > | FloatPropertyMap |
typedef std::map< KeyType, int > | IntPropertyMap |
typedef unsigned int | KeyType |
typedef std::map< KeyType, aiMatrix4x4 > | MatrixPropertyMap |
typedef std::map< KeyType, std::string > | StringPropertyMap |
Public Member Functions | |
ExportProperties () | |
Standard constructor. More... | |
ExportProperties (const ExportProperties &other) | |
Copy constructor. More... | |
bool | GetPropertyBool (const char *szName, bool bErrorReturn=false) const |
Get a boolean configuration property. More... | |
float | GetPropertyFloat (const char *szName, float fErrorReturn=10e10f) const |
Get a floating-point configuration property. More... | |
int | GetPropertyInteger (const char *szName, int iErrorReturn=0xffffffff) const |
Get a configuration property. More... | |
const aiMatrix4x4 | GetPropertyMatrix (const char *szName, const aiMatrix4x4 &sErrorReturn=aiMatrix4x4()) const |
Get a matrix configuration property. More... | |
const std::string | GetPropertyString (const char *szName, const std::string &sErrorReturn="") const |
Get a string configuration property. More... | |
bool | HasPropertyBool (const char *szName) const |
Determine a boolean configuration property has been set. More... | |
bool | HasPropertyFloat (const char *szName) const |
Determine a boolean configuration property has been set. More... | |
bool | HasPropertyInteger (const char *szName) const |
Determine a integer configuration property has been set. More... | |
bool | HasPropertyMatrix (const char *szName) const |
Determine a Matrix configuration property has been set. More... | |
bool | HasPropertyString (const char *szName) const |
Determine a String configuration property has been set. More... | |
bool | SetPropertyBool (const char *szName, bool value) |
Set a boolean configuration property. More... | |
bool | SetPropertyFloat (const char *szName, float fValue) |
Set a floating-point configuration property. More... | |
bool | SetPropertyInteger (const char *szName, int iValue) |
Set an integer configuration property. More... | |
bool | SetPropertyMatrix (const char *szName, const aiMatrix4x4 &sValue) |
Set a matrix configuration property. More... | |
bool | SetPropertyString (const char *szName, const std::string &sValue) |
Set a string configuration property. More... | |
Protected Attributes | |
FloatPropertyMap | mFloatProperties |
List of floating-point properties. More... | |
IntPropertyMap | mIntProperties |
List of integer properties. More... | |
MatrixPropertyMap | mMatrixProperties |
List of Matrix properties. More... | |
StringPropertyMap | mStringProperties |
List of string properties. More... | |
Member Typedef Documentation
typedef std::map<KeyType, float> Assimp::ExportProperties::FloatPropertyMap |
typedef std::map<KeyType, int> Assimp::ExportProperties::IntPropertyMap |
typedef unsigned int Assimp::ExportProperties::KeyType |
typedef std::map<KeyType, aiMatrix4x4> Assimp::ExportProperties::MatrixPropertyMap |
typedef std::map<KeyType, std::string> Assimp::ExportProperties::StringPropertyMap |
Constructor & Destructor Documentation
Assimp::ExportProperties::ExportProperties | ( | ) |
Standard constructor.
- See also
- ExportProperties()
Assimp::ExportProperties::ExportProperties | ( | const ExportProperties & | other | ) |
Copy constructor.
This copies the configuration properties of another ExportProperties.
Member Function Documentation
|
inline |
Get a boolean configuration property.
Boolean properties are stored on the integer stack internally so it's possible to set them via SetPropertyBool and query them with GetPropertyBool and vice versa.
- See also
- GetPropertyInteger()
float Assimp::ExportProperties::GetPropertyFloat | ( | const char * | szName, |
float | fErrorReturn = 10e10f |
||
) | const |
Get a floating-point configuration property.
- See also
- GetPropertyInteger()
int Assimp::ExportProperties::GetPropertyInteger | ( | const char * | szName, |
int | iErrorReturn = 0xffffffff |
||
) | const |
Get a configuration property.
- Parameters
-
szName Name of the property. All supported properties are defined in the aiConfig.g header (all constants share the prefix AI_CONFIG_XXX). iErrorReturn Value that is returned if the property is not found.
- Returns
- Current value of the property
- Note
- Property of different types (float, int, string ..) are kept on different lists, so calling SetPropertyInteger() for a floating-point property has no effect - the loader will call GetPropertyFloat() to read the property, but it won't be there.
const aiMatrix4x4 Assimp::ExportProperties::GetPropertyMatrix | ( | const char * | szName, |
const aiMatrix4x4 & | sErrorReturn = aiMatrix4x4() |
||
) | const |
Get a matrix configuration property.
The return value remains valid until the property is modified.
- See also
- GetPropertyInteger()
const std::string Assimp::ExportProperties::GetPropertyString | ( | const char * | szName, |
const std::string & | sErrorReturn = "" |
||
) | const |
Get a string configuration property.
The return value remains valid until the property is modified.
- See also
- GetPropertyInteger()
bool Assimp::ExportProperties::HasPropertyBool | ( | const char * | szName | ) | const |
Determine a boolean configuration property has been set.
- See also
- HasPropertyBool()
bool Assimp::ExportProperties::HasPropertyFloat | ( | const char * | szName | ) | const |
Determine a boolean configuration property has been set.
- See also
- HasPropertyFloat()
bool Assimp::ExportProperties::HasPropertyInteger | ( | const char * | szName | ) | const |
Determine a integer configuration property has been set.
- See also
- HasPropertyInteger()
bool Assimp::ExportProperties::HasPropertyMatrix | ( | const char * | szName | ) | const |
Determine a Matrix configuration property has been set.
- See also
- HasPropertyMatrix()
bool Assimp::ExportProperties::HasPropertyString | ( | const char * | szName | ) | const |
Determine a String configuration property has been set.
- See also
- HasPropertyString()
|
inline |
Set a boolean configuration property.
Boolean properties are stored on the integer stack internally so it's possible to set them via SetPropertyBool and query them with GetPropertyBool and vice versa.
- See also
- SetPropertyInteger()
bool Assimp::ExportProperties::SetPropertyFloat | ( | const char * | szName, |
float | fValue | ||
) |
Set a floating-point configuration property.
- See also
- SetPropertyInteger()
bool Assimp::ExportProperties::SetPropertyInteger | ( | const char * | szName, |
int | iValue | ||
) |
Set an integer configuration property.
- Parameters
-
szName Name of the property. All supported properties are defined in the aiConfig.g header (all constants share the prefix AI_CONFIG_XXX and are simple strings). iValue New value of the property
- Returns
- true if the property was set before. The new value replaces the previous value in this case.
- Note
- Property of different types (float, int, string ..) are kept on different stacks, so calling SetPropertyInteger() for a floating-point property has no effect - the loader will call GetPropertyFloat() to read the property, but it won't be there.
bool Assimp::ExportProperties::SetPropertyMatrix | ( | const char * | szName, |
const aiMatrix4x4 & | sValue | ||
) |
Set a matrix configuration property.
- See also
- SetPropertyInteger()
bool Assimp::ExportProperties::SetPropertyString | ( | const char * | szName, |
const std::string & | sValue | ||
) |
Set a string configuration property.
- See also
- SetPropertyInteger()
Member Data Documentation
|
protected |
List of floating-point properties.
|
protected |
List of integer properties.
|
protected |
List of Matrix properties.
|
protected |
List of string properties.
The documentation for this class was generated from the following file:
Generated on Sun Feb 21 2016 19:42:29 for Assimp by
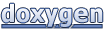