Assimp
v3.1.1 (June 2014)
|
Represents a row-major 4x4 matrix, use this for homogeneous coordinates. More...
Public Member Functions | |
aiMatrix4x4t () | |
set to identity More... | |
aiMatrix4x4t (TReal _a1, TReal _a2, TReal _a3, TReal _a4, TReal _b1, TReal _b2, TReal _b3, TReal _b4, TReal _c1, TReal _c2, TReal _c3, TReal _c4, TReal _d1, TReal _d2, TReal _d3, TReal _d4) | |
construction from single values More... | |
aiMatrix4x4t (const aiMatrix3x3t< TReal > &m) | |
construction from 3x3 matrix, remaining elements are set to identity More... | |
aiMatrix4x4t (const aiVector3t< TReal > &scaling, const aiQuaterniont< TReal > &rotation, const aiVector3t< TReal > &position) | |
construction from position, rotation and scaling components More... | |
void | Decompose (aiVector3t< TReal > &scaling, aiQuaterniont< TReal > &rotation, aiVector3t< TReal > &position) const |
Decompose a trafo matrix into its original components. More... | |
void | DecomposeNoScaling (aiQuaterniont< TReal > &rotation, aiVector3t< TReal > &position) const |
Decompose a trafo matrix with no scaling into its original components. More... | |
TReal | Determinant () const |
bool | Equal (const aiMatrix4x4t &m, TReal epsilon=1e-6) const |
aiMatrix4x4t & | FromEulerAnglesXYZ (TReal x, TReal y, TReal z) |
Creates a trafo matrix from a set of euler angles. More... | |
aiMatrix4x4t & | FromEulerAnglesXYZ (const aiVector3t< TReal > &blubb) |
aiMatrix4x4t & | Inverse () |
Invert the matrix. More... | |
bool | IsIdentity () const |
Returns true of the matrix is the identity matrix. More... | |
template<typename TOther > | |
operator aiMatrix4x4t< TOther > () const | |
bool | operator!= (const aiMatrix4x4t &m) const |
aiMatrix4x4t | operator* (const aiMatrix4x4t &m) const |
aiMatrix4x4t & | operator*= (const aiMatrix4x4t &m) |
bool | operator== (const aiMatrix4x4t &m) const |
TReal * | operator[] (unsigned int p_iIndex) |
const TReal * | operator[] (unsigned int p_iIndex) const |
aiMatrix4x4t & | Transpose () |
Transpose the matrix. More... | |
Static Public Member Functions | |
static aiMatrix4x4t & | FromToMatrix (const aiVector3t< TReal > &from, const aiVector3t< TReal > &to, aiMatrix4x4t &out) |
A function for creating a rotation matrix that rotates a vector called "from" into another vector called "to". More... | |
static aiMatrix4x4t & | Rotation (TReal a, const aiVector3t< TReal > &axis, aiMatrix4x4t &out) |
Returns a rotation matrix for a rotation around an arbitrary axis. More... | |
static aiMatrix4x4t & | RotationX (TReal a, aiMatrix4x4t &out) |
Returns a rotation matrix for a rotation around the x axis. More... | |
static aiMatrix4x4t & | RotationY (TReal a, aiMatrix4x4t &out) |
Returns a rotation matrix for a rotation around the y axis. More... | |
static aiMatrix4x4t & | RotationZ (TReal a, aiMatrix4x4t &out) |
Returns a rotation matrix for a rotation around the z axis. More... | |
static aiMatrix4x4t & | Scaling (const aiVector3t< TReal > &v, aiMatrix4x4t &out) |
Returns a scaling matrix. More... | |
static aiMatrix4x4t & | Translation (const aiVector3t< TReal > &v, aiMatrix4x4t &out) |
Returns a translation matrix. More... | |
Public Attributes | |
union { | |
struct { | |
TReal a1 | |
TReal a2 | |
TReal a3 | |
TReal a4 | |
TReal b1 | |
TReal b2 | |
TReal b3 | |
TReal b4 | |
TReal c1 | |
TReal c2 | |
TReal c3 | |
TReal c4 | |
TReal d1 | |
TReal d2 | |
TReal d3 | |
TReal d4 | |
} | |
TReal m [4][4] | |
TReal mData [16] | |
}; | |
Detailed Description
template<typename TReal>
class aiMatrix4x4t< TReal >
Represents a row-major 4x4 matrix, use this for homogeneous coordinates.
There's much confusion about matrix layouts (column vs. row order). This is always a row-major matrix. Not even with the aiProcess_ConvertToLeftHanded flag, which absolutely does not affect matrix order - it just affects the handedness of the coordinate system defined thereby.
Constructor & Destructor Documentation
aiMatrix4x4t< TReal >::aiMatrix4x4t | ( | ) |
set to identity
aiMatrix4x4t< TReal >::aiMatrix4x4t | ( | TReal | _a1, |
TReal | _a2, | ||
TReal | _a3, | ||
TReal | _a4, | ||
TReal | _b1, | ||
TReal | _b2, | ||
TReal | _b3, | ||
TReal | _b4, | ||
TReal | _c1, | ||
TReal | _c2, | ||
TReal | _c3, | ||
TReal | _c4, | ||
TReal | _d1, | ||
TReal | _d2, | ||
TReal | _d3, | ||
TReal | _d4 | ||
) |
construction from single values
|
inlineexplicit |
construction from 3x3 matrix, remaining elements are set to identity
|
inline |
construction from position, rotation and scaling components
- Parameters
-
scaling The scaling for the x,y,z axes rotation The rotation as a hamilton quaternion position The position for the x,y,z axes
Member Function Documentation
|
inline |
Decompose a trafo matrix into its original components.
- Parameters
-
scaling Receives the output scaling for the x,y,z axes rotation Receives the output rotation as a hamilton quaternion position Receives the output position for the x,y,z axes
|
inline |
Decompose a trafo matrix with no scaling into its original components.
- Parameters
-
rotation Receives the output rotation as a hamilton quaternion position Receives the output position for the x,y,z axes
|
inline |
|
inline |
|
inline |
Creates a trafo matrix from a set of euler angles.
- Parameters
-
x Rotation angle for the x-axis, in radians y Rotation angle for the y-axis, in radians z Rotation angle for the z-axis, in radians
|
inline |
|
inlinestatic |
A function for creating a rotation matrix that rotates a vector called "from" into another vector called "to".
Input : from[3], to[3] which both must be normalized non-zero vectors Output: mtx[3][3] – a 3x3 matrix in column-major form Authors: Tomas Mueller, John Hughes "Efficiently Building a Matrix to Rotate One Vector to Another" Journal of Graphics Tools, 4(4):1-4, 1999
Input : from[3], to[3] which both must be normalized non-zero vectors Output: mtx[3][3] – a 3x3 matrix in colum-major form Authors: Tomas M�ller, John Hughes "Efficiently Building a Matrix to Rotate One Vector to Another" Journal of Graphics Tools, 4(4):1-4, 1999
|
inline |
Invert the matrix.
If the matrix is not invertible all elements are set to qnan. Beware, use (f != f) to check whether a TReal f is qnan.
|
inline |
Returns true of the matrix is the identity matrix.
The check is performed against a not so small epsilon.
aiMatrix4x4t< TReal >::operator aiMatrix4x4t< TOther > | ( | ) | const |
|
inline |
|
inline |
|
inline |
|
inline |
|
inline |
|
inline |
|
inlinestatic |
Returns a rotation matrix for a rotation around an arbitrary axis.
- Parameters
-
a Rotation angle, in radians axis Rotation axis, should be a normalized vector. out Receives the output matrix
- Returns
- Reference to the output matrix
|
inlinestatic |
Returns a rotation matrix for a rotation around the x axis.
- Parameters
-
a Rotation angle, in radians out Receives the output matrix
- Returns
- Reference to the output matrix
|
inlinestatic |
Returns a rotation matrix for a rotation around the y axis.
- Parameters
-
a Rotation angle, in radians out Receives the output matrix
- Returns
- Reference to the output matrix
|
inlinestatic |
Returns a rotation matrix for a rotation around the z axis.
- Parameters
-
a Rotation angle, in radians out Receives the output matrix
- Returns
- Reference to the output matrix
|
inlinestatic |
Returns a scaling matrix.
- Parameters
-
v Scaling vector out Receives the output matrix
- Returns
- Reference to the output matrix
|
inlinestatic |
Returns a translation matrix.
- Parameters
-
v Translation vector out Receives the output matrix
- Returns
- Reference to the output matrix
|
inline |
Transpose the matrix.
Member Data Documentation
union { ... } |
TReal aiMatrix4x4t< TReal >::a1 |
TReal aiMatrix4x4t< TReal >::a2 |
TReal aiMatrix4x4t< TReal >::a3 |
TReal aiMatrix4x4t< TReal >::a4 |
TReal aiMatrix4x4t< TReal >::b1 |
TReal aiMatrix4x4t< TReal >::b2 |
TReal aiMatrix4x4t< TReal >::b3 |
TReal aiMatrix4x4t< TReal >::b4 |
TReal aiMatrix4x4t< TReal >::c1 |
TReal aiMatrix4x4t< TReal >::c2 |
TReal aiMatrix4x4t< TReal >::c3 |
TReal aiMatrix4x4t< TReal >::c4 |
TReal aiMatrix4x4t< TReal >::d1 |
TReal aiMatrix4x4t< TReal >::d2 |
TReal aiMatrix4x4t< TReal >::d3 |
TReal aiMatrix4x4t< TReal >::d4 |
TReal aiMatrix4x4t< TReal >::m[4][4] |
TReal aiMatrix4x4t< TReal >::mData[16] |
The documentation for this class was generated from the following files:
Generated on Sun Feb 21 2016 19:42:29 for Assimp by
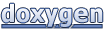