Assimp
v3.1.1 (June 2014)
|
Data structure for a material. More...
Public Member Functions | |
aiReturn | AddBinaryProperty (const void *pInput, unsigned int pSizeInBytes, const char *pKey, unsigned int type, unsigned int index, aiPropertyTypeInfo pType) |
Add a property with a given key and type info to the material structure. More... | |
aiReturn | AddProperty (const aiString *pInput, const char *pKey, unsigned int type=0, unsigned int index=0) |
Add a string property with a given key and type info to the material structure. More... | |
template<class TYPE > | |
aiReturn | AddProperty (const TYPE *pInput, unsigned int pNumValues, const char *pKey, unsigned int type=0, unsigned int index=0) |
Add a property with a given key to the material structure. More... | |
aiReturn | AddProperty (const aiVector3D *pInput, unsigned int pNumValues, const char *pKey, unsigned int type=0, unsigned int index=0) |
aiReturn | AddProperty (const aiColor3D *pInput, unsigned int pNumValues, const char *pKey, unsigned int type=0, unsigned int index=0) |
aiReturn | AddProperty (const aiColor4D *pInput, unsigned int pNumValues, const char *pKey, unsigned int type=0, unsigned int index=0) |
aiReturn | AddProperty (const int *pInput, unsigned int pNumValues, const char *pKey, unsigned int type=0, unsigned int index=0) |
aiReturn | AddProperty (const float *pInput, unsigned int pNumValues, const char *pKey, unsigned int type=0, unsigned int index=0) |
aiReturn | AddProperty (const aiUVTransform *pInput, unsigned int pNumValues, const char *pKey, unsigned int type=0, unsigned int index=0) |
aiMaterial () | |
void | Clear () |
Removes all properties from the material. More... | |
template<typename Type > | |
aiReturn | Get (const char *pKey, unsigned int type, unsigned int idx, Type *pOut, unsigned int *pMax) const |
Retrieve an array of Type values with a specific key from the material. More... | |
aiReturn | Get (const char *pKey, unsigned int type, unsigned int idx, int *pOut, unsigned int *pMax) const |
aiReturn | Get (const char *pKey, unsigned int type, unsigned int idx, float *pOut, unsigned int *pMax) const |
template<typename Type > | |
aiReturn | Get (const char *pKey, unsigned int type, unsigned int idx, Type &pOut) const |
Retrieve a Type value with a specific key from the material. More... | |
aiReturn | Get (const char *pKey, unsigned int type, unsigned int idx, int &pOut) const |
aiReturn | Get (const char *pKey, unsigned int type, unsigned int idx, float &pOut) const |
aiReturn | Get (const char *pKey, unsigned int type, unsigned int idx, aiString &pOut) const |
aiReturn | Get (const char *pKey, unsigned int type, unsigned int idx, aiColor3D &pOut) const |
aiReturn | Get (const char *pKey, unsigned int type, unsigned int idx, aiColor4D &pOut) const |
aiReturn | Get (const char *pKey, unsigned int type, unsigned int idx, aiUVTransform &pOut) const |
aiReturn | GetTexture (aiTextureType type, unsigned int index, aiString *path, aiTextureMapping *mapping=NULL, unsigned int *uvindex=NULL, float *blend=NULL, aiTextureOp *op=NULL, aiTextureMapMode *mapmode=NULL) const |
Helper function to get all parameters pertaining to a particular texture slot from a material. More... | |
unsigned int | GetTextureCount (aiTextureType type) const |
Get the number of textures for a particular texture type. More... | |
aiReturn | RemoveProperty (const char *pKey, unsigned int type=0, unsigned int index=0) |
Remove a given key from the list. More... | |
~aiMaterial () | |
Static Public Member Functions | |
static void | CopyPropertyList (aiMaterial *pcDest, const aiMaterial *pcSrc) |
Copy the property list of a material. More... | |
Public Attributes | |
unsigned int | mNumAllocated |
Storage allocated. More... | |
unsigned int | mNumProperties |
Number of properties in the data base. More... | |
aiMaterialProperty ** | mProperties |
List of all material properties loaded. More... | |
Detailed Description
Data structure for a material.
Material data is stored using a key-value structure. A single key-value pair is called a 'material property'. C++ users should use the provided member functions of aiMaterial to process material properties, C users have to stick with the aiMaterialGetXXX family of unbound functions. The library defines a set of standard keys (AI_MATKEY_XXX).
Constructor & Destructor Documentation
aiMaterial::aiMaterial | ( | ) |
aiMaterial::~aiMaterial | ( | ) |
Member Function Documentation
aiReturn aiMaterial::AddBinaryProperty | ( | const void * | pInput, |
unsigned int | pSizeInBytes, | ||
const char * | pKey, | ||
unsigned int | type, | ||
unsigned int | index, | ||
aiPropertyTypeInfo | pType | ||
) |
Add a property with a given key and type info to the material structure.
- Parameters
-
pInput Pointer to input data pSizeInBytes Size of input data pKey Key/Usage of the property (AI_MATKEY_XXX) type Set by the AI_MATKEY_XXX macro index Set by the AI_MATKEY_XXX macro pType Type information hint
aiReturn aiMaterial::AddProperty | ( | const aiString * | pInput, |
const char * | pKey, | ||
unsigned int | type = 0 , |
||
unsigned int | index = 0 |
||
) |
Add a string property with a given key and type info to the material structure.
- Parameters
-
pInput Input string pKey Key/Usage of the property (AI_MATKEY_XXX) type Set by the AI_MATKEY_XXX macro index Set by the AI_MATKEY_XXX macro
aiReturn aiMaterial::AddProperty | ( | const TYPE * | pInput, |
unsigned int | pNumValues, | ||
const char * | pKey, | ||
unsigned int | type = 0 , |
||
unsigned int | index = 0 |
||
) |
Add a property with a given key to the material structure.
- Parameters
-
pInput Pointer to the input data pNumValues Number of values in the array pKey Key/Usage of the property (AI_MATKEY_XXX) type Set by the AI_MATKEY_XXX macro index Set by the AI_MATKEY_XXX macro
aiReturn aiMaterial::AddProperty | ( | const aiVector3D * | pInput, |
unsigned int | pNumValues, | ||
const char * | pKey, | ||
unsigned int | type = 0 , |
||
unsigned int | index = 0 |
||
) |
aiReturn aiMaterial::AddProperty | ( | const aiColor3D * | pInput, |
unsigned int | pNumValues, | ||
const char * | pKey, | ||
unsigned int | type = 0 , |
||
unsigned int | index = 0 |
||
) |
aiReturn aiMaterial::AddProperty | ( | const aiColor4D * | pInput, |
unsigned int | pNumValues, | ||
const char * | pKey, | ||
unsigned int | type = 0 , |
||
unsigned int | index = 0 |
||
) |
aiReturn aiMaterial::AddProperty | ( | const int * | pInput, |
unsigned int | pNumValues, | ||
const char * | pKey, | ||
unsigned int | type = 0 , |
||
unsigned int | index = 0 |
||
) |
aiReturn aiMaterial::AddProperty | ( | const float * | pInput, |
unsigned int | pNumValues, | ||
const char * | pKey, | ||
unsigned int | type = 0 , |
||
unsigned int | index = 0 |
||
) |
aiReturn aiMaterial::AddProperty | ( | const aiUVTransform * | pInput, |
unsigned int | pNumValues, | ||
const char * | pKey, | ||
unsigned int | type = 0 , |
||
unsigned int | index = 0 |
||
) |
void aiMaterial::Clear | ( | ) |
Removes all properties from the material.
The data array remains allocated so adding new properties is quite fast.
|
static |
Copy the property list of a material.
- Parameters
-
pcDest Destination material pcSrc Source material
aiReturn aiMaterial::Get | ( | const char * | pKey, |
unsigned int | type, | ||
unsigned int | idx, | ||
Type * | pOut, | ||
unsigned int * | pMax | ||
) | const |
Retrieve an array of Type values with a specific key from the material.
- Parameters
-
pKey Key to search for. One of the AI_MATKEY_XXX constants. type .. set by AI_MATKEY_XXX idx .. set by AI_MATKEY_XXX pOut Pointer to a buffer to receive the result. pMax Specifies the size of the given buffer, in Type's. Receives the number of values (not bytes!) read. NULL is a valid value for this parameter.
aiReturn aiMaterial::Get | ( | const char * | pKey, |
unsigned int | type, | ||
unsigned int | idx, | ||
int * | pOut, | ||
unsigned int * | pMax | ||
) | const |
aiReturn aiMaterial::Get | ( | const char * | pKey, |
unsigned int | type, | ||
unsigned int | idx, | ||
float * | pOut, | ||
unsigned int * | pMax | ||
) | const |
aiReturn aiMaterial::Get | ( | const char * | pKey, |
unsigned int | type, | ||
unsigned int | idx, | ||
Type & | pOut | ||
) | const |
Retrieve a Type value with a specific key from the material.
- Parameters
-
pKey Key to search for. One of the AI_MATKEY_XXX constants. type Specifies the type of the texture to be retrieved ( e.g. diffuse, specular, height map ...) idx Index of the texture to be retrieved. pOut Reference to receive the output value
aiReturn aiMaterial::Get | ( | const char * | pKey, |
unsigned int | type, | ||
unsigned int | idx, | ||
int & | pOut | ||
) | const |
aiReturn aiMaterial::Get | ( | const char * | pKey, |
unsigned int | type, | ||
unsigned int | idx, | ||
float & | pOut | ||
) | const |
aiReturn aiMaterial::Get | ( | const char * | pKey, |
unsigned int | type, | ||
unsigned int | idx, | ||
aiString & | pOut | ||
) | const |
aiReturn aiMaterial::Get | ( | const char * | pKey, |
unsigned int | type, | ||
unsigned int | idx, | ||
aiColor3D & | pOut | ||
) | const |
aiReturn aiMaterial::Get | ( | const char * | pKey, |
unsigned int | type, | ||
unsigned int | idx, | ||
aiColor4D & | pOut | ||
) | const |
aiReturn aiMaterial::Get | ( | const char * | pKey, |
unsigned int | type, | ||
unsigned int | idx, | ||
aiUVTransform & | pOut | ||
) | const |
aiReturn aiMaterial::GetTexture | ( | aiTextureType | type, |
unsigned int | index, | ||
aiString * | path, | ||
aiTextureMapping * | mapping = NULL , |
||
unsigned int * | uvindex = NULL , |
||
float * | blend = NULL , |
||
aiTextureOp * | op = NULL , |
||
aiTextureMapMode * | mapmode = NULL |
||
) | const |
Helper function to get all parameters pertaining to a particular texture slot from a material.
This function is provided just for convenience, you could also read the single material properties manually.
- Parameters
-
type Specifies the type of the texture to be retrieved ( e.g. diffuse, specular, height map ...) index Index of the texture to be retrieved. The function fails if there is no texture of that type with this index. GetTextureCount() can be used to determine the number of textures per texture type. path Receives the path to the texture. NULL is a valid value. mapping The texture mapping. NULL is allowed as value. uvindex Receives the UV index of the texture. NULL is a valid value. blend Receives the blend factor for the texture NULL is a valid value. op Receives the texture operation to be performed between this texture and the previous texture. NULL is allowed as value. mapmode Receives the mapping modes to be used for the texture. The parameter may be NULL but if it is a valid pointer it MUST point to an array of 3 aiTextureMapMode's (one for each axis: UVW order (=XYZ)).
unsigned int aiMaterial::GetTextureCount | ( | aiTextureType | type | ) | const |
Get the number of textures for a particular texture type.
- Parameters
-
type Texture type to check for
- Returns
- Number of textures for this type.
- Note
- A texture can be easily queried using GetTexture()
aiReturn aiMaterial::RemoveProperty | ( | const char * | pKey, |
unsigned int | type = 0 , |
||
unsigned int | index = 0 |
||
) |
Remove a given key from the list.
The function fails if the key isn't found
- Parameters
-
pKey Key to be deleted type Set by the AI_MATKEY_XXX macro index Set by the AI_MATKEY_XXX macro
Member Data Documentation
unsigned int aiMaterial::mNumAllocated |
Storage allocated.
unsigned int aiMaterial::mNumProperties |
Number of properties in the data base.
aiMaterialProperty** aiMaterial::mProperties |
List of all material properties loaded.
The documentation for this struct was generated from the following file:
Generated on Sun Feb 21 2016 19:42:29 for Assimp by
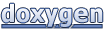