Assimp
v3.1.1 (June 2014)
|
A mesh represents a geometry or model with a single material. More...
Public Member Functions | |
aiMesh () | |
Default constructor. Initializes all members to 0. More... | |
unsigned int | GetNumColorChannels () const |
Get the number of vertex color channels the mesh contains. More... | |
unsigned int | GetNumUVChannels () const |
Get the number of UV channels the mesh contains. More... | |
bool | HasBones () const |
Check whether the mesh contains bones. More... | |
bool | HasFaces () const |
Check whether the mesh contains faces. More... | |
bool | HasNormals () const |
Check whether the mesh contains normal vectors. More... | |
bool | HasPositions () const |
Check whether the mesh contains positions. More... | |
bool | HasTangentsAndBitangents () const |
Check whether the mesh contains tangent and bitangent vectors It is not possible that it contains tangents and no bitangents (or the other way round). More... | |
bool | HasTextureCoords (unsigned int pIndex) const |
Check whether the mesh contains a texture coordinate set. More... | |
bool | HasVertexColors (unsigned int pIndex) const |
Check whether the mesh contains a vertex color set. More... | |
~aiMesh () | |
Deletes all storage allocated for the mesh. More... | |
Public Attributes | |
aiAnimMesh ** | mAnimMeshes |
NOT CURRENTLY IN USE. More... | |
aiVector3D * | mBitangents |
Vertex bitangents. More... | |
aiBone ** | mBones |
The bones of this mesh. More... | |
aiColor4D * | mColors [AI_MAX_NUMBER_OF_COLOR_SETS] |
Vertex color sets. More... | |
aiFace * | mFaces |
The faces the mesh is constructed from. More... | |
unsigned int | mMaterialIndex |
The material used by this mesh. More... | |
aiString | mName |
Name of the mesh. More... | |
aiVector3D * | mNormals |
Vertex normals. More... | |
unsigned int | mNumAnimMeshes |
NOT CURRENTLY IN USE. More... | |
unsigned int | mNumBones |
The number of bones this mesh contains. More... | |
unsigned int | mNumFaces |
The number of primitives (triangles, polygons, lines) in this mesh. More... | |
unsigned int | mNumUVComponents [AI_MAX_NUMBER_OF_TEXTURECOORDS] |
Specifies the number of components for a given UV channel. More... | |
unsigned int | mNumVertices |
The number of vertices in this mesh. More... | |
unsigned int | mPrimitiveTypes |
Bitwise combination of the members of the aiPrimitiveType enum. More... | |
aiVector3D * | mTangents |
Vertex tangents. More... | |
aiVector3D * | mTextureCoords [AI_MAX_NUMBER_OF_TEXTURECOORDS] |
Vertex texture coords, also known as UV channels. More... | |
aiVector3D * | mVertices |
Vertex positions. More... | |
Detailed Description
A mesh represents a geometry or model with a single material.
It usually consists of a number of vertices and a series of primitives/faces referencing the vertices. In addition there might be a series of bones, each of them addressing a number of vertices with a certain weight. Vertex data is presented in channels with each channel containing a single per-vertex information such as a set of texture coords or a normal vector. If a data pointer is non-null, the corresponding data stream is present. From C++-programs you can also use the comfort functions Has*() to test for the presence of various data streams.
A Mesh uses only a single material which is referenced by a material ID.
- Note
- The mPositions member is usually not optional. However, vertex positions could be missing if the AI_SCENE_FLAGS_INCOMPLETE flag is set in
Constructor & Destructor Documentation
|
inline |
Default constructor. Initializes all members to 0.
|
inline |
Deletes all storage allocated for the mesh.
Member Function Documentation
|
inline |
Get the number of vertex color channels the mesh contains.
|
inline |
Get the number of UV channels the mesh contains.
|
inline |
Check whether the mesh contains bones.
|
inline |
Check whether the mesh contains faces.
If no special scene flags are set this should always return true
|
inline |
Check whether the mesh contains normal vectors.
|
inline |
Check whether the mesh contains positions.
Provided no special scene flags are set, this will always be true
|
inline |
Check whether the mesh contains tangent and bitangent vectors It is not possible that it contains tangents and no bitangents (or the other way round).
The existence of one of them implies that the second is there, too.
|
inline |
Check whether the mesh contains a texture coordinate set.
- Parameters
-
pIndex Index of the texture coordinates set
|
inline |
Check whether the mesh contains a vertex color set.
- Parameters
-
pIndex Index of the vertex color set
Member Data Documentation
aiAnimMesh** aiMesh::mAnimMeshes |
NOT CURRENTLY IN USE.
Attachment meshes for this mesh, for vertex-based animation. Attachment meshes carry replacement data for some of the mesh'es vertex components (usually positions, normals).
aiVector3D* aiMesh::mBitangents |
Vertex bitangents.
The bitangent of a vertex points in the direction of the positive Y texture axis. The array contains normalized vectors, NULL if not present. The array is mNumVertices in size.
- Note
- If the mesh contains tangents, it automatically also contains bitangents.
aiBone** aiMesh::mBones |
The bones of this mesh.
A bone consists of a name by which it can be found in the frame hierarchy and a set of vertex weights.
aiColor4D* aiMesh::mColors[AI_MAX_NUMBER_OF_COLOR_SETS] |
Vertex color sets.
A mesh may contain 0 to AI_MAX_NUMBER_OF_COLOR_SETS vertex colors per vertex. NULL if not present. Each array is mNumVertices in size if present.
aiFace* aiMesh::mFaces |
The faces the mesh is constructed from.
Each face refers to a number of vertices by their indices. This array is always present in a mesh, its size is given in mNumFaces. If the AI_SCENE_FLAGS_NON_VERBOSE_FORMAT is NOT set each face references an unique set of vertices.
unsigned int aiMesh::mMaterialIndex |
The material used by this mesh.
A mesh uses only a single material. If an imported model uses multiple materials, the import splits up the mesh. Use this value as index into the scene's material list.
aiString aiMesh::mName |
Name of the mesh.
Meshes can be named, but this is not a requirement and leaving this field empty is totally fine. There are mainly three uses for mesh names:
- some formats name nodes and meshes independently.
- importers tend to split meshes up to meet the one-material-per-mesh requirement. Assigning the same (dummy) name to each of the result meshes aids the caller at recovering the original mesh partitioning.
- Vertex animations refer to meshes by their names.
aiVector3D* aiMesh::mNormals |
Vertex normals.
The array contains normalized vectors, NULL if not present. The array is mNumVertices in size. Normals are undefined for point and line primitives. A mesh consisting of points and lines only may not have normal vectors. Meshes with mixed primitive types (i.e. lines and triangles) may have normals, but the normals for vertices that are only referenced by point or line primitives are undefined and set to QNaN (WARN: qNaN compares to inequal to everything, even to qNaN itself. Using code like this to check whether a field is qnan is:
still dangerous because even 1.f == 1.f could evaluate to false! ( remember the subtleties of IEEE754 artithmetics). Use stuff like fpclassify
instead.
- Note
- Normal vectors computed by Assimp are always unit-length. However, this needn't apply for normals that have been taken directly from the model file.
unsigned int aiMesh::mNumAnimMeshes |
NOT CURRENTLY IN USE.
The number of attachment meshes
unsigned int aiMesh::mNumBones |
The number of bones this mesh contains.
Can be 0, in which case the mBones array is NULL.
unsigned int aiMesh::mNumFaces |
The number of primitives (triangles, polygons, lines) in this mesh.
This is also the size of the mFaces array. The maximum value for this member is AI_MAX_FACES.
unsigned int aiMesh::mNumUVComponents[AI_MAX_NUMBER_OF_TEXTURECOORDS] |
Specifies the number of components for a given UV channel.
Up to three channels are supported (UVW, for accessing volume or cube maps). If the value is 2 for a given channel n, the component p.z of mTextureCoords[n][p] is set to 0.0f. If the value is 1 for a given channel, p.y is set to 0.0f, too.
- Note
- 4D coords are not supported
unsigned int aiMesh::mNumVertices |
The number of vertices in this mesh.
This is also the size of all of the per-vertex data arrays. The maximum value for this member is AI_MAX_VERTICES.
unsigned int aiMesh::mPrimitiveTypes |
Bitwise combination of the members of the aiPrimitiveType enum.
This specifies which types of primitives are present in the mesh. The "SortByPrimitiveType"-Step can be used to make sure the output meshes consist of one primitive type each.
aiVector3D* aiMesh::mTangents |
Vertex tangents.
The tangent of a vertex points in the direction of the positive X texture axis. The array contains normalized vectors, NULL if not present. The array is mNumVertices in size. A mesh consisting of points and lines only may not have normal vectors. Meshes with mixed primitive types (i.e. lines and triangles) may have normals, but the normals for vertices that are only referenced by point or line primitives are undefined and set to qNaN. See the mNormals member for a detailled discussion of qNaNs.
- Note
- If the mesh contains tangents, it automatically also contains bitangents.
aiVector3D* aiMesh::mTextureCoords[AI_MAX_NUMBER_OF_TEXTURECOORDS] |
Vertex texture coords, also known as UV channels.
A mesh may contain 0 to AI_MAX_NUMBER_OF_TEXTURECOORDS per vertex. NULL if not present. The array is mNumVertices in size.
aiVector3D* aiMesh::mVertices |
Vertex positions.
This array is always present in a mesh. The array is mNumVertices in size.
The documentation for this struct was generated from the following file:
Generated on Sun Feb 21 2016 19:42:29 for Assimp by
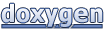