STSW-STLKT01
|
main.c
309 size = sprintf(data_s, "TimeStamp: %d\r\n Acc_X: %d, Acc_Y: %d, Acc_Z :%d\r\n Gyro_X:%d, Gyro_Y:%d, Gyro_Z:%d\r\n Magn_X:%d, Magn_Y:%d, Magn_Z:%d\r\n Press:%5.2f, Temp:%5.2f, Hum:%4.1f\r\n",
DrvStatusTypeDef BSP_HUMIDITY_Set_ODR_Value(void *handle, float odr)
Set the humidity sensor output data rate.
Definition: SensorTile_humidity.c:684
DrvStatusTypeDef BSP_PRESSURE_Init(PRESSURE_ID_t id, void **handle)
Initialize a pressure sensor.
Definition: SensorTile_pressure.c:101
DrvStatusTypeDef BSP_PRESSURE_Sensor_Enable(void *handle)
Enable pressure sensor.
Definition: SensorTile_pressure.c:258
DrvStatusTypeDef BSP_HUMIDITY_Init(HUMIDITY_ID_t id, void **handle)
Initialize a humidity sensor.
Definition: SensorTile_humidity.c:88
DrvStatusTypeDef BSP_GYRO_Sensor_Disable(void *handle)
Disable gyroscope sensor.
Definition: SensorTile_gyro.c:270
Definition: datalog_application.h:77
DrvStatusTypeDef BSP_HUMIDITY_Sensor_Disable(void *handle)
Disable humidity sensor.
Definition: SensorTile_humidity.c:250
DrvStatusTypeDef BSP_GYRO_Init(GYRO_ID_t id, void **handle)
Initialize a gyroscope sensor.
Definition: SensorTile_gyro.c:88
DrvStatusTypeDef BSP_MAGNETO_Set_ODR_Value(void *handle, float odr)
Set the magnetometer sensor output data rate.
Definition: SensorTile_magneto.c:682
DrvStatusTypeDef BSP_ACCELERO_Set_Tap_Threshold_Ext(void *handle, uint8_t thr)
Set the tap threshold (available only for LSM6DS3 sensor)
Definition: SensorTile_accelero.c:2187
DrvStatusTypeDef BSP_ACCELERO_Get_Double_Tap_Detection_Status_Ext(void *handle, uint8_t *status)
Get the double tap detection status (available only for LSM6DS3 sensor)
Definition: SensorTile_accelero.c:2137
DrvStatusTypeDef BSP_PRESSURE_Set_ODR_Value(void *handle, float odr)
Set the pressure sensor output data rate.
Definition: SensorTile_pressure.c:616
DrvStatusTypeDef BSP_ACCELERO_Enable_Double_Tap_Detection_Ext(void *handle)
Enable the double tap detection (available only for LSM6DS3 sensor)
Definition: SensorTile_accelero.c:2046
DrvStatusTypeDef BSP_ACCELERO_Sensor_Disable(void *handle)
Disable accelerometer sensor.
Definition: SensorTile_accelero.c:353
void Error_Handler(void)
This function is executed in case of error occurrence.
Definition: main.c:177
DrvStatusTypeDef BSP_ACCELERO_Set_ODR_Value(void *handle, float odr)
Set the accelerometer sensor output data rate.
Definition: SensorTile_accelero.c:757
DrvStatusTypeDef BSP_MAGNETO_Sensor_Enable(void *handle)
Enable magnetometer sensor.
Definition: SensorTile_magneto.c:245
DrvStatusTypeDef BSP_PRESSURE_Sensor_Disable(void *handle)
Disable pressure sensor.
Definition: SensorTile_pressure.c:292
Header for datalog_application.c module.
DrvStatusTypeDef BSP_GYRO_Sensor_Enable(void *handle)
Enable gyroscope sensor.
Definition: SensorTile_gyro.c:236
DrvStatusTypeDef BSP_MAGNETO_Init(MAGNETO_ID_t id, void **handle)
Initialize a magnetometer sensor.
Definition: SensorTile_magneto.c:101
uint8_t USBD_CDC_RegisterInterface(USBD_HandleTypeDef *pdev, USBD_CDC_ItfTypeDef *fops)
USBD_CDC_RegisterInterface.
Definition: usbd_cdc.c:790
DrvStatusTypeDef BSP_ACCELERO_Sensor_Enable(void *handle)
Enable accelerometer sensor.
Definition: SensorTile_accelero.c:319
DrvStatusTypeDef BSP_ACCELERO_Init(ACCELERO_ID_t id, void **handle)
Initialize an accelerometer sensor.
Definition: SensorTile_accelero.c:93
DrvStatusTypeDef BSP_TEMPERATURE_Set_ODR_Value(void *handle, float odr)
Set the temperature sensor output data rate.
Definition: SensorTile_temperature.c:670
uint8_t CDC_Fill_Buffer(uint8_t *Buf, uint32_t TotalLen)
Fill the usb tx buffer.
Definition: usbd_cdc_interface.c:224
DrvStatusTypeDef BSP_TEMPERATURE_Sensor_Disable(void *handle)
Disable temperature sensor.
Definition: SensorTile_temperature.c:346
DrvStatusTypeDef BSP_TEMPERATURE_Init(TEMPERATURE_ID_t id, void **handle)
Initialize a temperature sensor.
Definition: SensorTile_temperature.c:92
DrvStatusTypeDef BSP_HUMIDITY_Sensor_Enable(void *handle)
Enable humidity sensor.
Definition: SensorTile_humidity.c:216
DrvStatusTypeDef BSP_TEMPERATURE_Sensor_Enable(void *handle)
Enable temperature sensor.
Definition: SensorTile_temperature.c:312
DrvStatusTypeDef BSP_GYRO_Set_ODR_Value(void *handle, float odr)
Set the gyroscope sensor output data rate.
Definition: SensorTile_gyro.c:671
DrvStatusTypeDef BSP_MAGNETO_Sensor_Disable(void *handle)
Disable magnetometer sensor.
Definition: SensorTile_magneto.c:279
Generated by
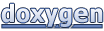