STSW-STLKT01
|
usbd_cdc.c
Go to the documentation of this file.
135 __ALIGN_BEGIN static uint8_t USBD_CDC_DeviceQualifierDesc[USB_LEN_DEV_QUALIFIER_DESC] __ALIGN_END =
Definition: usbd_cdc.h:111
USBD_StatusTypeDef USBD_LL_CloseEP(USBD_HandleTypeDef *pdev, uint8_t ep_addr)
Closes an endpoint of the Low Level Driver.
Definition: usbd_conf.c:327
uint8_t USBD_CDC_RegisterInterface(USBD_HandleTypeDef *pdev, USBD_CDC_ItfTypeDef *fops)
USBD_CDC_RegisterInterface.
Definition: usbd_cdc.c:790
uint8_t USBD_CDC_ReceivePacket(USBD_HandleTypeDef *pdev)
USBD_CDC_ReceivePacket prepare OUT Endpoint for reception.
Definition: usbd_cdc.c:883
uint8_t * USBD_CDC_GetDeviceQualifierDescriptor(uint16_t *length)
DeviceQualifierDescriptor return Device Qualifier descriptor.
Definition: usbd_cdc.c:778
static uint8_t USBD_CDC_DeInit(USBD_HandleTypeDef *pdev, uint8_t cfgidx)
USBD_CDC_Init DeInitialize the CDC layer.
Definition: usbd_cdc.c:563
uint8_t USBD_CDC_TransmitPacket(USBD_HandleTypeDef *pdev)
USBD_CDC_DataOut Data received on non-control Out endpoint.
Definition: usbd_cdc.c:846
uint8_t USBD_CDC_SetRxBuffer(USBD_HandleTypeDef *pdev, uint8_t *pbuff)
USBD_CDC_SetRxBuffer.
Definition: usbd_cdc.c:829
static uint8_t * USBD_CDC_GetOtherSpeedCfgDesc(uint16_t *length)
USBD_CDC_GetCfgDesc Return configuration descriptor.
Definition: usbd_cdc.c:766
static uint8_t USBD_CDC_DataIn(USBD_HandleTypeDef *pdev, uint8_t epnum)
USBD_CDC_DataIn Data sent on non-control IN endpoint.
Definition: usbd_cdc.c:664
static uint8_t * USBD_CDC_GetFSCfgDesc(uint16_t *length)
USBD_CDC_GetFSCfgDesc Return configuration descriptor.
Definition: usbd_cdc.c:740
__ALIGN_BEGIN uint8_t USBD_DeviceDesc [USB_LEN_DEV_DESC] __ALIGN_END
Definition: usbd_desc.c:91
USBD_StatusTypeDef USBD_LL_OpenEP(USBD_HandleTypeDef *pdev, uint8_t ep_addr, uint8_t ep_type, uint16_t ep_mps)
Opens an endpoint of the Low Level Driver.
Definition: usbd_conf.c:308
USBD_StatusTypeDef USBD_LL_PrepareReceive(USBD_HandleTypeDef *pdev, uint8_t ep_addr, uint8_t *pbuf, uint16_t size)
Prepares an endpoint for reception.
Definition: usbd_conf.c:426
Definition: usbd_cdc.h:101
static uint8_t USBD_CDC_Setup(USBD_HandleTypeDef *pdev, USBD_SetupReqTypedef *req)
USBD_CDC_Setup Handle the CDC specific requests.
Definition: usbd_cdc.c:599
static uint8_t * USBD_CDC_GetHSCfgDesc(uint16_t *length)
USBD_CDC_GetHSCfgDesc Return configuration descriptor.
Definition: usbd_cdc.c:753
header file for the usbd_cdc.c file.
static uint8_t USBD_CDC_EP0_RxReady(USBD_HandleTypeDef *pdev)
USBD_CDC_DataOut Data received on non-control Out endpoint.
Definition: usbd_cdc.c:718
USBD_StatusTypeDef USBD_LL_Transmit(USBD_HandleTypeDef *pdev, uint8_t ep_addr, uint8_t *pbuf, uint16_t size)
Transmits data over an endpoint.
Definition: usbd_conf.c:409
uint8_t USBD_CDC_SetTxBuffer(USBD_HandleTypeDef *pdev, uint8_t *pbuff, uint16_t length)
USBD_CDC_SetTxBuffer.
Definition: usbd_cdc.c:810
uint32_t USBD_LL_GetRxDataSize(USBD_HandleTypeDef *pdev, uint8_t ep_addr)
Returns the last transfered packet size.
Definition: usbd_conf.c:441
static uint8_t USBD_CDC_Init(USBD_HandleTypeDef *pdev, uint8_t cfgidx)
USBD_CDC_Init Initialize the CDC interface.
Definition: usbd_cdc.c:475
static uint8_t USBD_CDC_DataOut(USBD_HandleTypeDef *pdev, uint8_t epnum)
USBD_CDC_DataOut Data received on non-control Out endpoint.
Definition: usbd_cdc.c:688
Generated by
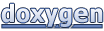