STSW-STLKT01
|
This file provides the USBD descriptors and string formating method. More...
#include "usbd_core.h"
#include "usbd_desc.h"
#include "usbd_conf.h"
Go to the source code of this file.
Functions | |
uint8_t * | USBD_VCP_DeviceDescriptor (USBD_SpeedTypeDef speed, uint16_t *length) |
Returns the device descriptor. More... | |
uint8_t * | USBD_VCP_LangIDStrDescriptor (USBD_SpeedTypeDef speed, uint16_t *length) |
Returns the LangID string descriptor. More... | |
uint8_t * | USBD_VCP_ManufacturerStrDescriptor (USBD_SpeedTypeDef speed, uint16_t *length) |
Returns the manufacturer string descriptor. More... | |
uint8_t * | USBD_VCP_ProductStrDescriptor (USBD_SpeedTypeDef speed, uint16_t *length) |
Returns the product string descriptor. More... | |
uint8_t * | USBD_VCP_SerialStrDescriptor (USBD_SpeedTypeDef speed, uint16_t *length) |
Returns the serial number string descriptor. More... | |
uint8_t * | USBD_VCP_ConfigStrDescriptor (USBD_SpeedTypeDef speed, uint16_t *length) |
Returns the configuration string descriptor. More... | |
uint8_t * | USBD_VCP_InterfaceStrDescriptor (USBD_SpeedTypeDef speed, uint16_t *length) |
Returns the interface string descriptor. More... | |
static void | IntToUnicode (uint32_t value, uint8_t *pbuf, uint8_t len) |
Convert Hex 32Bits value into char. More... | |
static void | Get_SerialNum (void) |
Create the serial number string descriptor. More... | |
Variables | |
USBD_DescriptorsTypeDef | VCP_Desc |
__ALIGN_BEGIN uint8_t USBD_DeviceDesc [USB_LEN_DEV_DESC] | __ALIGN_END |
uint8_t | USBD_StringSerial [USB_SIZ_STRING_SERIAL] |
Detailed Description
This file provides the USBD descriptors and string formating method.
- Author
- MCD Application Team
- Version
- V1.3.0
- Date
- 29-January-2016
- Attention
© Copyright (c) 2016 STMicroelectronics International N.V. All rights reserved.
Redistribution and use in source and binary forms, with or without modification, are permitted, provided that the following conditions are met:
- Redistribution of source code must retain the above copyright notice, this list of conditions and the following disclaimer.
- Redistributions in binary form must reproduce the above copyright notice, this list of conditions and the following disclaimer in the documentation and/or other materials provided with the distribution.
- Neither the name of STMicroelectronics nor the names of other contributors to this software may be used to endorse or promote products derived from this software without specific written permission.
- This software, including modifications and/or derivative works of this software, must execute solely and exclusively on microcontroller or microprocessor devices manufactured by or for STMicroelectronics.
- Redistribution and use of this software other than as permitted under this license is void and will automatically terminate your rights under this license.
THIS SOFTWARE IS PROVIDED BY STMICROELECTRONICS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS, IMPLIED OR STATUTORY WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NON-INFRINGEMENT OF THIRD PARTY INTELLECTUAL PROPERTY RIGHTS ARE DISCLAIMED TO THE FULLEST EXTENT PERMITTED BY LAW. IN NO EVENT SHALL STMICROELECTRONICS OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
Definition in file usbd_desc.c.
Function Documentation
◆ Get_SerialNum()
|
static |
Create the serial number string descriptor.
- Parameters
-
None
- Return values
-
None
Definition at line 231 of file usbd_desc.c.
◆ IntToUnicode()
|
static |
Convert Hex 32Bits value into char.
- Parameters
-
value value to convert pbuf pointer to the buffer len buffer length
- Return values
-
None
Definition at line 255 of file usbd_desc.c.
◆ USBD_VCP_ConfigStrDescriptor()
uint8_t * USBD_VCP_ConfigStrDescriptor | ( | USBD_SpeedTypeDef | speed, |
uint16_t * | length | ||
) |
Returns the configuration string descriptor.
- Parameters
-
speed Current device speed length Pointer to data length variable
- Return values
-
Pointer to descriptor buffer
Definition at line 208 of file usbd_desc.c.
◆ USBD_VCP_DeviceDescriptor()
uint8_t * USBD_VCP_DeviceDescriptor | ( | USBD_SpeedTypeDef | speed, |
uint16_t * | length | ||
) |
Returns the device descriptor.
- Parameters
-
speed Current device speed length Pointer to data length variable
- Return values
-
Pointer to descriptor buffer
Definition at line 144 of file usbd_desc.c.
◆ USBD_VCP_InterfaceStrDescriptor()
uint8_t * USBD_VCP_InterfaceStrDescriptor | ( | USBD_SpeedTypeDef | speed, |
uint16_t * | length | ||
) |
Returns the interface string descriptor.
- Parameters
-
speed Current device speed length Pointer to data length variable
- Return values
-
Pointer to descriptor buffer
Definition at line 220 of file usbd_desc.c.
◆ USBD_VCP_LangIDStrDescriptor()
uint8_t * USBD_VCP_LangIDStrDescriptor | ( | USBD_SpeedTypeDef | speed, |
uint16_t * | length | ||
) |
Returns the LangID string descriptor.
- Parameters
-
speed Current device speed length Pointer to data length variable
- Return values
-
Pointer to descriptor buffer
Definition at line 156 of file usbd_desc.c.
◆ USBD_VCP_ManufacturerStrDescriptor()
uint8_t * USBD_VCP_ManufacturerStrDescriptor | ( | USBD_SpeedTypeDef | speed, |
uint16_t * | length | ||
) |
Returns the manufacturer string descriptor.
- Parameters
-
speed Current device speed length Pointer to data length variable
- Return values
-
Pointer to descriptor buffer
Definition at line 180 of file usbd_desc.c.
◆ USBD_VCP_ProductStrDescriptor()
uint8_t * USBD_VCP_ProductStrDescriptor | ( | USBD_SpeedTypeDef | speed, |
uint16_t * | length | ||
) |
Returns the product string descriptor.
- Parameters
-
speed Current device speed length Pointer to data length variable
- Return values
-
Pointer to descriptor buffer
Definition at line 168 of file usbd_desc.c.
◆ USBD_VCP_SerialStrDescriptor()
uint8_t * USBD_VCP_SerialStrDescriptor | ( | USBD_SpeedTypeDef | speed, |
uint16_t * | length | ||
) |
Returns the serial number string descriptor.
- Parameters
-
speed Current device speed length Pointer to data length variable
- Return values
-
Pointer to descriptor buffer
Definition at line 192 of file usbd_desc.c.
Variable Documentation
◆ __ALIGN_END
__ALIGN_BEGIN uint8_t USBD_StrDesc [USBD_MAX_STR_DESC_SIZ] __ALIGN_END |
< IAR Compiler
Definition at line 91 of file usbd_desc.c.
◆ USBD_StringSerial
uint8_t USBD_StringSerial[USB_SIZ_STRING_SERIAL] |
IAR Compiler
Definition at line 123 of file usbd_desc.c.
◆ VCP_Desc
USBD_DescriptorsTypeDef VCP_Desc |
Definition at line 77 of file usbd_desc.c.
Generated by
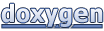