STSW-STLKT01
|
usbd_cdc_interface.c
Go to the documentation of this file.
68 uint8_t UserTxBuffer[APP_TX_DATA_SIZE];/* Received Data over UART (CDC interface) are stored in this buffer */
288 // memcpy((uint8_t*)&USB_RxBuffer[USB_RxBufferStart_idx], (uint8_t*)&Buf[Buf_idx], numByteToCopy);
292 // memcpy((uint8_t*)&USB_RxBuffer[USB_RxBufferStart_idx], (uint8_t*)&Buf[Buf_idx], numByteToCopy);
uint8_t USBD_CDC_SetTxBuffer(USBD_HandleTypeDef *pdev, uint8_t *pbuff, uint16_t length)
USBD_CDC_SetTxBuffer.
Definition: usbd_cdc.c:810
void HAL_TIM_PeriodElapsedCallback(TIM_HandleTypeDef *htim)
TIM period elapsed callback.
Definition: usbd_cdc_interface.c:241
uint8_t USBD_CDC_TransmitPacket(USBD_HandleTypeDef *pdev)
USBD_CDC_DataOut Data received on non-control Out endpoint.
Definition: usbd_cdc.c:846
uint8_t USBD_CDC_SetRxBuffer(USBD_HandleTypeDef *pdev, uint8_t *pbuff)
USBD_CDC_SetRxBuffer.
Definition: usbd_cdc.c:829
static int8_t CDC_Itf_DeInit(void)
CDC_Itf_DeInit DeInitializes the CDC media low layer.
Definition: usbd_cdc_interface.c:144
static int8_t CDC_Itf_Control(uint8_t cmd, uint8_t *pbuf, uint16_t length)
CDC_Itf_Control Manage the CDC class requests.
Definition: usbd_cdc_interface.c:158
static int8_t CDC_Itf_Init(void)
CDC_Itf_Init Initializes the CDC media low layer.
Definition: usbd_cdc_interface.c:108
Definition: usbd_cdc.h:93
Definition: usbd_cdc.h:101
uint8_t CDC_Fill_Buffer(uint8_t *Buf, uint32_t TotalLen)
Fill the usb tx buffer.
Definition: usbd_cdc_interface.c:224
static void Error_Handler(void)
This function is executed in case of error occurrence.
Definition: usbd_cdc_interface.c:343
Header for usbd_cdc_interface.c file.
static int8_t CDC_Itf_Receive(uint8_t *pbuf, uint32_t *Len)
CDC_Itf_DataRx Data received over USB OUT endpoint are sent over CDC interface through this function...
Definition: usbd_cdc_interface.c:281
Generated by
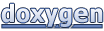