STSW-STLKT01
|
main.c
Go to the documentation of this file.
uint8_t BSP_AUDIO_OUT_SetVolume(void *handle, uint8_t Volume)
Controls the current audio volume level.
Definition: SensorTile_audio_out.c:317
uint8_t BSP_AUDIO_IN_Init(uint32_t AudioFreq, uint32_t BitRes, uint32_t ChnlNbr)
Initializes audio acquisition.
Definition: SensorTile_audio_in.c:146
void AudioProcess(void)
User function that is called when 1 ms of PDM data is available. In this application only PDM to PCM ...
Definition: main.c:125
void BSP_AUDIO_IN_TransferComplete_CallBack(void)
Transfer Complete user callback, called by BSP functions.
Definition: main.c:157
uint8_t BSP_AUDIO_OUT_Init(CODEX_ID_t id, void **handle, uint16_t OutputDevice, uint8_t Volume, uint32_t AudioFreq)
Configures the audio peripherals.
Definition: SensorTile_audio_out.c:129
void Error_Handler(void)
This function is executed in case of error occurrence.
Definition: main.c:177
void BSP_AUDIO_IN_HalfTransfer_CallBack(void)
Half Transfer Complete user callback, called by BSP functions.
Definition: main.c:167
void Send_Audio_to_USB(int16_t *audioData, uint16_t PCMSamples)
Fills USB audio buffer with the right amount of data, depending on the channel/frequency configuratio...
Definition: usbd_audio_if.c:183
uint8_t BSP_AUDIO_IN_Record(uint16_t *pbuf, uint32_t size)
Starts audio recording.
Definition: SensorTile_audio_in.c:209
uint8_t BSP_AUDIO_OUT_Play(void *handle, uint16_t *pBuffer, uint32_t Size)
Starts playing audio stream from a data buffer for a determined size.
Definition: SensorTile_audio_out.c:221
Generated by
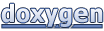