STSW-STLKT01
|
Functions | |
uint32_t | BSP_GetVersion (void) |
This method returns the STM32446E EVAL BSP Driver revision. More... | |
void | BSP_LED_Init (Led_TypeDef Led) |
Configures LEDs. More... | |
void | BSP_LED_DeInit (Led_TypeDef Led) |
DeInit LEDs. More... | |
void | BSP_LED_On (Led_TypeDef Led) |
Turns selected LED On. More... | |
void | BSP_LED_Off (Led_TypeDef Led) |
Turns selected LED Off. More... | |
void | BSP_LED_Toggle (Led_TypeDef Led) |
Toggles the selected LED. More... | |
DrvStatusTypeDef | Sensor_IO_I2C_Init (void) |
Configures sensor SPI interface. More... | |
DrvStatusTypeDef | Sensor_IO_SPI_Init (void) |
Configures sensor SPI interface. More... | |
uint8_t | Sensor_IO_SPI_CS_Init_All (void) |
uint8_t | Sensor_IO_SPI_CS_Init (void *handle) |
uint8_t | Sensor_IO_Write (void *handle, uint8_t WriteAddr, uint8_t *pBuffer, uint16_t nBytesToWrite) |
Writes a buffer to the sensor. More... | |
uint8_t | Sensor_IO_I2C_Write (void *handle, uint8_t WriteAddr, uint8_t *pBuffer, uint16_t nBytesToWrite) |
Writes a buffer to the sensor by I2C. More... | |
uint8_t | Sensor_IO_Read (void *handle, uint8_t ReadAddr, uint8_t *pBuffer, uint16_t nBytesToRead) |
Reads from the sensor to a buffer. More... | |
uint8_t | Sensor_IO_I2C_Read (void *handle, uint8_t ReadAddr, uint8_t *pBuffer, uint16_t nBytesToRead) |
Reads from the sensor to a buffer by I2C. More... | |
uint8_t | Sensor_IO_SPI_Write (void *handle, uint8_t WriteAddr, uint8_t *pBuffer, uint16_t nBytesToWrite) |
Writes a buffer to the sensor. More... | |
uint8_t | Sensor_IO_SPI_Read (void *handle, uint8_t ReadAddr, uint8_t *pBuffer, uint16_t nBytesToRead) |
Reads a from the sensor to buffer. More... | |
uint8_t | Sensor_IO_SPI_CS_Enable (void *handle) |
uint8_t | Sensor_IO_SPI_CS_Disable (void *handle) |
DrvStatusTypeDef | LSM6DSM_Sensor_IO_ITConfig (void) |
Configures sensor interrupts interface for LSM6DSM sensor. More... | |
void | SD_IO_CS_Init (void) |
void | SD_IO_CS_DeInit (void) |
void | SPI_Read (SPI_HandleTypeDef *xSpiHandle, uint8_t *val) |
This function reads a single byte on SPI 3-wire. More... | |
void | SPI_Read_nBytes (SPI_HandleTypeDef *xSpiHandle, uint8_t *val, uint16_t nBytesToRead) |
This function reads multiple bytes on SPI 3-wire. More... | |
void | SPI_Write (SPI_HandleTypeDef *xSpiHandle, uint8_t val) |
This function writes a single byte on SPI 3-wire. More... | |
static uint8_t | I2C_SENSORTILE_Init (void) |
Configures I2C interface. More... | |
static uint8_t | I2C_SENSORTILE_WriteData (uint8_t Addr, uint8_t Reg, uint8_t *pBuffer, uint16_t Size) |
Write data to the register of the device through BUS. More... | |
static uint8_t | I2C_SENSORTILE_ReadData (uint8_t Addr, uint8_t Reg, uint8_t *pBuffer, uint16_t Size) |
Read a register of the device through BUS. More... | |
static void | I2C_SENSORTILE_Error (uint8_t Addr) |
Manages error callback by re-initializing I2C. More... | |
static void | I2C_SENSORTILE_MspInit (void) |
I2C MSP Initialization. More... | |
static void | SD_IO_SPI_MspInit (SPI_HandleTypeDef *hspi) |
Initializes SPI MSP. More... | |
static void | SD_IO_SPI_Init_LS (void) |
Initializes SPI HAL. Low baundrate for initializazion phase. More... | |
static void | SD_IO_SPI_Init (void) |
Initializes SPI HAL. High baundrate. More... | |
static uint32_t | SD_IO_SPI_Read (void) |
SPI Read 4 bytes from device. More... | |
static void | SD_IO_SPI_Write (uint8_t Value) |
SPI Write a byte to device. More... | |
static void | SD_IO_SPI_Error (void) |
SPI error treatment function. More... | |
void | SD_IO_Init (void) |
Initializes the SD Card and put it into StandBy State (Ready for data transfer). More... | |
void | SD_IO_Init_LS () |
Initializes the SD Card and put it into StandBy State (Ready for data transfer). Low baundrate. More... | |
void | SD_IO_WriteByte (uint8_t Data) |
Writes a byte on the SD. More... | |
void | SD_IO_WriteDMA (uint8_t *pData, uint16_t Size) |
Writes a block by DMA on the SD. More... | |
uint8_t | SD_IO_ReadByte (void) |
Reads a byte from the SD. More... | |
uint8_t | SD_IO_WriteCmd_wResp (uint8_t Cmd, uint32_t Arg, uint8_t Crc) |
Sends 5 bytes command to the SD card and get response. More... | |
HAL_StatusTypeDef | SD_IO_WriteCmd (uint8_t Cmd, uint32_t Arg, uint8_t Crc, uint8_t Response) |
Sends 5 bytes command to the SD card and get response. More... | |
HAL_StatusTypeDef | SD_IO_WaitResponse (uint8_t Response) |
Waits response from the SD card. More... | |
void | SD_IO_WriteDummy (void) |
Sends dummy byte with CS High. More... | |
Detailed Description
Function Documentation
◆ BSP_GetVersion()
uint32_t BSP_GetVersion | ( | void | ) |
This method returns the STM32446E EVAL BSP Driver revision.
- Parameters
-
None
- Return values
-
version 0xXYZR (8bits for each decimal, R for RC)
Definition at line 159 of file SensorTile.c.
◆ BSP_LED_DeInit()
void BSP_LED_DeInit | ( | Led_TypeDef | Led | ) |
DeInit LEDs.
- Parameters
-
Led LED to be configured. This parameter can be one of the following values: - LED1
- LED2
- LED3
- LED4
- Note
- Led DeInit does not disable the GPIO clock nor disable the Mfx
- Return values
-
None
Definition at line 204 of file SensorTile.c.
◆ BSP_LED_Init()
void BSP_LED_Init | ( | Led_TypeDef | Led | ) |
Configures LEDs.
- Parameters
-
Led LED to be configured. This parameter can be one of the following values: - LED1
- Return values
-
None
Definition at line 172 of file SensorTile.c.
◆ BSP_LED_Off()
void BSP_LED_Off | ( | Led_TypeDef | Led | ) |
Turns selected LED Off.
- Parameters
-
Led LED to be set off This parameter can be one of the following values: - LED1
- LED2
- LED3
- LED4
- Return values
-
None
Definition at line 241 of file SensorTile.c.
◆ BSP_LED_On()
void BSP_LED_On | ( | Led_TypeDef | Led | ) |
Turns selected LED On.
- Parameters
-
Led LED to be set on This parameter can be one of the following values: - LED1
- LED2
- LED3
- LED4
- Return values
-
None
Definition at line 219 of file SensorTile.c.
◆ BSP_LED_Toggle()
void BSP_LED_Toggle | ( | Led_TypeDef | Led | ) |
Toggles the selected LED.
- Parameters
-
Led LED to be toggled This parameter can be one of the following values: - LED1
- LED2
- LED3
- LED4
- Return values
-
None
Definition at line 263 of file SensorTile.c.
◆ I2C_SENSORTILE_Error()
|
static |
Manages error callback by re-initializing I2C.
- Parameters
-
Addr I2C Address
- Return values
-
None
Definition at line 882 of file SensorTile.c.
◆ I2C_SENSORTILE_Init()
|
static |
Configures I2C interface.
- Parameters
-
None
- Return values
-
0 in case of success 1 in case of failure
Definition at line 789 of file SensorTile.c.
◆ I2C_SENSORTILE_MspInit()
|
static |
I2C MSP Initialization.
- Parameters
-
None
- Return values
-
None
Definition at line 897 of file SensorTile.c.
◆ I2C_SENSORTILE_ReadData()
|
static |
Read a register of the device through BUS.
- Parameters
-
Addr Device address on BUS Reg The target register address to read pBuffer The data to be read Size Number of bytes to be read
- Return values
-
0 in case of success 1 in case of failure
Definition at line 857 of file SensorTile.c.
◆ I2C_SENSORTILE_WriteData()
|
static |
Write data to the register of the device through BUS.
- Parameters
-
Addr Device address on BUS Reg The target register address to be written pBuffer The data to be written Size Number of bytes to be written
- Return values
-
0 in case of success 1 in case of failure
Definition at line 826 of file SensorTile.c.
◆ LSM6DSM_Sensor_IO_ITConfig()
DrvStatusTypeDef LSM6DSM_Sensor_IO_ITConfig | ( | void | ) |
Configures sensor interrupts interface for LSM6DSM sensor.
- Parameters
-
None
- Return values
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 664 of file SensorTile.c.
◆ SD_IO_Init()
void SD_IO_Init | ( | void | ) |
Initializes the SD Card and put it into StandBy State (Ready for data transfer).
- Parameters
-
None
- Return values
-
None
Definition at line 1140 of file SensorTile.c.
◆ SD_IO_Init_LS()
void SD_IO_Init_LS | ( | void | ) |
Initializes the SD Card and put it into StandBy State (Ready for data transfer). Low baundrate.
- Parameters
-
None
- Return values
-
None
Definition at line 1167 of file SensorTile.c.
◆ SD_IO_ReadByte()
uint8_t SD_IO_ReadByte | ( | void | ) |
Reads a byte from the SD.
- Parameters
-
None
- Return values
-
The received byte.
Definition at line 1253 of file SensorTile.c.
◆ SD_IO_SPI_Error()
|
static |
SPI error treatment function.
- Parameters
-
None
- Return values
-
None
Definition at line 1123 of file SensorTile.c.
◆ SD_IO_SPI_Init()
|
static |
Initializes SPI HAL. High baundrate.
- Parameters
-
None
- Return values
-
None
Definition at line 1049 of file SensorTile.c.
◆ SD_IO_SPI_Init_LS()
|
static |
Initializes SPI HAL. Low baundrate for initializazion phase.
- Parameters
-
None
- Return values
-
None
Definition at line 1023 of file SensorTile.c.
◆ SD_IO_SPI_MspInit()
|
static |
Initializes SPI MSP.
- Parameters
-
None
- Return values
-
None
Definition at line 945 of file SensorTile.c.
◆ SD_IO_SPI_Read()
|
static |
SPI Read 4 bytes from device.
- Parameters
-
None
- Return values
-
Read data
Definition at line 1081 of file SensorTile.c.
◆ SD_IO_SPI_Write()
|
static |
SPI Write a byte to device.
- Parameters
-
Value value to be written
- Return values
-
None
Definition at line 1104 of file SensorTile.c.
◆ SD_IO_WaitResponse()
HAL_StatusTypeDef SD_IO_WaitResponse | ( | uint8_t | Response | ) |
Waits response from the SD card.
- Parameters
-
Response Expected response from the SD card
- Return values
-
HAL_StatusTypeDef HAL Status
Definition at line 1347 of file SensorTile.c.
◆ SD_IO_WriteByte()
void SD_IO_WriteByte | ( | uint8_t | Data | ) |
Writes a byte on the SD.
- Parameters
-
Data byte to send.
- Return values
-
None
Definition at line 1224 of file SensorTile.c.
◆ SD_IO_WriteCmd()
HAL_StatusTypeDef SD_IO_WriteCmd | ( | uint8_t | Cmd, |
uint32_t | Arg, | ||
uint8_t | Crc, | ||
uint8_t | Response | ||
) |
Sends 5 bytes command to the SD card and get response.
- Parameters
-
Cmd The user expected command to send to SD card. Arg The command argument. Crc The CRC. Response Expected response from the SD card
- Return values
-
HAL_StatusTypeDef HAL Status
Definition at line 1312 of file SensorTile.c.
◆ SD_IO_WriteCmd_wResp()
uint8_t SD_IO_WriteCmd_wResp | ( | uint8_t | Cmd, |
uint32_t | Arg, | ||
uint8_t | Crc | ||
) |
Sends 5 bytes command to the SD card and get response.
- Parameters
-
Cmd The user expected command to send to SD card. Arg The command argument. Crc The CRC. Response Expected response from the SD card
- Return values
-
HAL_StatusTypeDef HAL Status
Definition at line 1273 of file SensorTile.c.
◆ SD_IO_WriteDMA()
void SD_IO_WriteDMA | ( | uint8_t * | pData, |
uint16_t | Size | ||
) |
Writes a block by DMA on the SD.
- Parameters
-
Data byte to send.
- Return values
-
None
Definition at line 1237 of file SensorTile.c.
◆ SD_IO_WriteDummy()
void SD_IO_WriteDummy | ( | void | ) |
Sends dummy byte with CS High.
- Parameters
-
None
- Return values
-
None
Definition at line 1374 of file SensorTile.c.
◆ Sensor_IO_I2C_Init()
DrvStatusTypeDef Sensor_IO_I2C_Init | ( | void | ) |
Configures sensor SPI interface.
- Parameters
-
None
- Return values
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 274 of file SensorTile.c.
◆ Sensor_IO_I2C_Read()
uint8_t Sensor_IO_I2C_Read | ( | void * | handle, |
uint8_t | ReadAddr, | ||
uint8_t * | pBuffer, | ||
uint16_t | nBytesToRead | ||
) |
Reads from the sensor to a buffer by I2C.
- Parameters
-
handle instance handle ReadAddr specifies the internal sensor address register to be read from pBuffer pointer to data buffer nBytesToRead number of bytes to be read
- Return values
-
0 in case of success 1 in case of failure
Definition at line 534 of file SensorTile.c.
◆ Sensor_IO_I2C_Write()
uint8_t Sensor_IO_I2C_Write | ( | void * | handle, |
uint8_t | WriteAddr, | ||
uint8_t * | pBuffer, | ||
uint16_t | nBytesToWrite | ||
) |
Writes a buffer to the sensor by I2C.
- Parameters
-
handle instance handle WriteAddr specifies the internal sensor address register to be written to pBuffer pointer to data buffer nBytesToWrite number of bytes to be written
- Return values
-
0 in case of success 1 in case of failure
Definition at line 472 of file SensorTile.c.
◆ Sensor_IO_Read()
uint8_t Sensor_IO_Read | ( | void * | handle, |
uint8_t | ReadAddr, | ||
uint8_t * | pBuffer, | ||
uint16_t | nBytesToRead | ||
) |
Reads from the sensor to a buffer.
- Parameters
-
handle instance handle ReadAddr specifies the internal sensor address register to be read from pBuffer pointer to data buffer nBytesToRead number of bytes to be read
- Return values
-
0 in case of success 1 in case of failure
Definition at line 490 of file SensorTile.c.
◆ Sensor_IO_SPI_Init()
DrvStatusTypeDef Sensor_IO_SPI_Init | ( | void | ) |
Configures sensor SPI interface.
- Parameters
-
None
- Return values
-
COMPONENT_OK in case of success COMPONENT_ERROR in case of failure
Definition at line 293 of file SensorTile.c.
◆ Sensor_IO_SPI_Read()
uint8_t Sensor_IO_SPI_Read | ( | void * | handle, |
uint8_t | ReadAddr, | ||
uint8_t * | pBuffer, | ||
uint16_t | nBytesToRead | ||
) |
Reads a from the sensor to buffer.
- Parameters
-
handle instance handle ReadAddr specifies the internal sensor address register to be read from pBuffer pointer to data buffer nBytesToRead number of bytes to be read
- Return values
-
0 in case of success 1 in case of failure
Definition at line 581 of file SensorTile.c.
◆ Sensor_IO_SPI_Write()
uint8_t Sensor_IO_SPI_Write | ( | void * | handle, |
uint8_t | WriteAddr, | ||
uint8_t * | pBuffer, | ||
uint16_t | nBytesToWrite | ||
) |
Writes a buffer to the sensor.
- Parameters
-
handle instance handle WriteAddr specifies the internal sensor address register to be written to pBuffer pointer to data buffer nBytesToWrite number of bytes to be written
- Return values
-
0 in case of success 1 in case of failure
Definition at line 553 of file SensorTile.c.
◆ Sensor_IO_Write()
uint8_t Sensor_IO_Write | ( | void * | handle, |
uint8_t | WriteAddr, | ||
uint8_t * | pBuffer, | ||
uint16_t | nBytesToWrite | ||
) |
Writes a buffer to the sensor.
- Parameters
-
handle instance handle WriteAddr specifies the internal sensor address register to be written to pBuffer pointer to data buffer nBytesToWrite number of bytes to be written
- Return values
-
0 in case of success 1 in case of failure
Definition at line 427 of file SensorTile.c.
◆ SPI_Read()
void SPI_Read | ( | SPI_HandleTypeDef * | xSpiHandle, |
uint8_t * | val | ||
) |
This function reads a single byte on SPI 3-wire.
- Parameters
-
xSpiHandle : SPI Handler. val : value.
- Return values
-
None
Definition at line 697 of file SensorTile.c.
◆ SPI_Read_nBytes()
void SPI_Read_nBytes | ( | SPI_HandleTypeDef * | xSpiHandle, |
uint8_t * | val, | ||
uint16_t | nBytesToRead | ||
) |
This function reads multiple bytes on SPI 3-wire.
- Parameters
-
xSpiHandle SPI Handler. val value. nBytesToRead number of bytes to read.
- Return values
-
None
Definition at line 726 of file SensorTile.c.
◆ SPI_Write()
void SPI_Write | ( | SPI_HandleTypeDef * | xSpiHandle, |
uint8_t | val | ||
) |
This function writes a single byte on SPI 3-wire.
- Parameters
-
xSpiHandle SPI Handler. val value.
- Return values
-
None
Definition at line 767 of file SensorTile.c.
Generated by
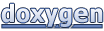