domSource Class Reference
#include <domSource.h>
Inheritance diagram for domSource:

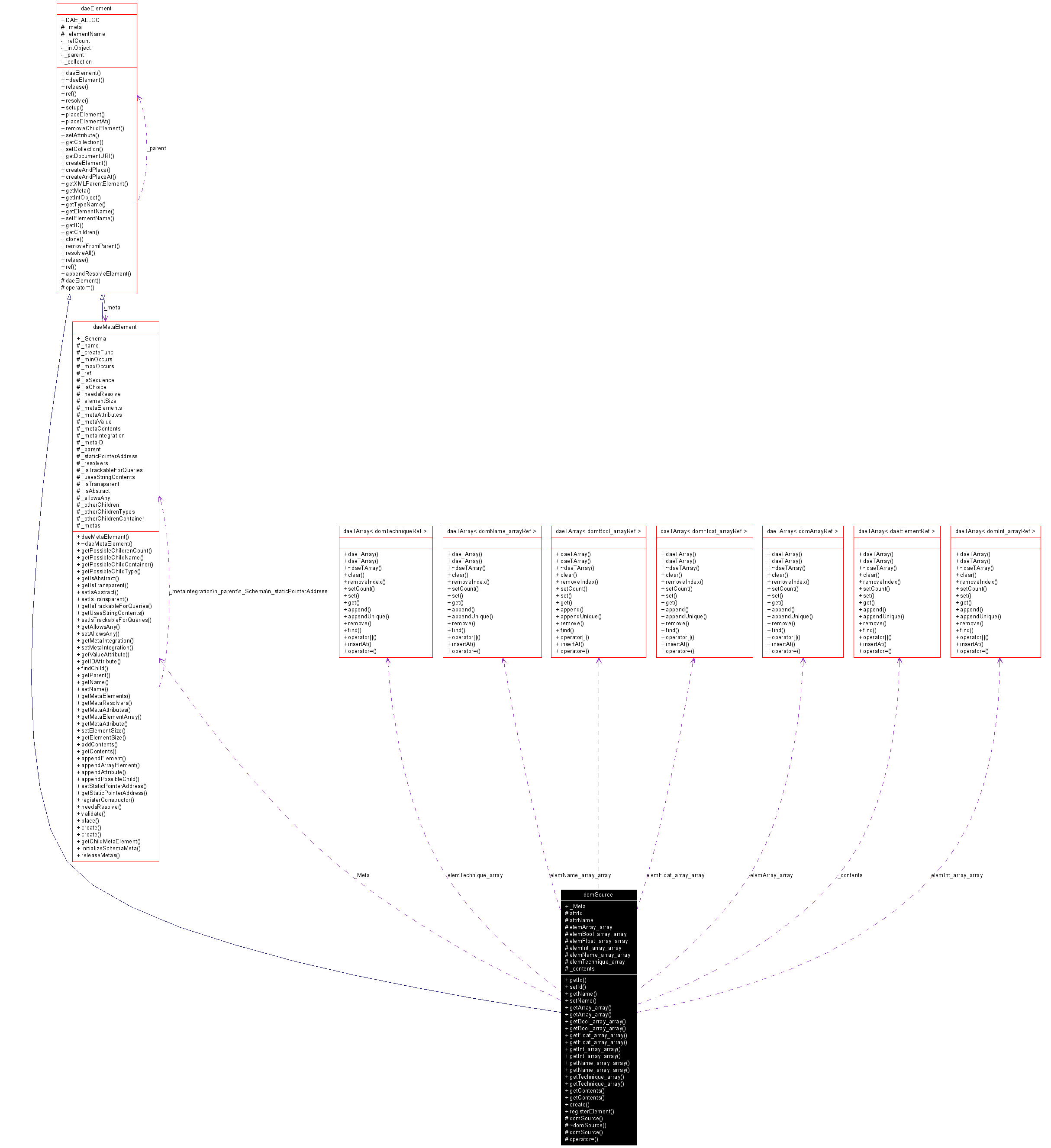
Detailed Description
The source element declares a data repository that provides values according to the semantics of an input element that refers to it.A data source is a well-known source of information that can be accessed through an established communication channel. The data source provides access methods to the information. These access methods implement various techniques according to the representation of the information. The information may be stored locally as an array of data or a program that generates the data. One of the array elements (array, bool_array, float_array, int_array, or Name_array) may occur zero or more times.
Public Types | |
typedef daeSmartRef< domTechnique > | domTechniqueRef |
typedef daeTArray< domTechniqueRef > | domTechnique_Array |
Public Member Functions | |
xsID | getId () const |
Gets the id attribute. | |
void | setId (xsID atId) |
Sets the id attribute. | |
xsNCName | getName () const |
Gets the name attribute. | |
void | setName (xsNCName atName) |
Sets the name attribute. | |
domArray_Array & | getArray_array () |
Gets the array element array. | |
const domArray_Array & | getArray_array () const |
Gets the array element array. | |
domBool_array_Array & | getBool_array_array () |
Gets the bool_array element array. | |
const domBool_array_Array & | getBool_array_array () const |
Gets the bool_array element array. | |
domFloat_array_Array & | getFloat_array_array () |
Gets the float_array element array. | |
const domFloat_array_Array & | getFloat_array_array () const |
Gets the float_array element array. | |
domInt_array_Array & | getInt_array_array () |
Gets the int_array element array. | |
const domInt_array_Array & | getInt_array_array () const |
Gets the int_array element array. | |
domName_array_Array & | getName_array_array () |
Gets the Name_array element array. | |
const domName_array_Array & | getName_array_array () const |
Gets the Name_array element array. | |
domTechnique_Array & | getTechnique_array () |
Gets the technique element array. | |
const domTechnique_Array & | getTechnique_array () const |
Gets the technique element array. | |
daeElementRefArray & | getContents () |
Gets the _contents array. | |
const daeElementRefArray & | getContents () const |
Gets the _contents array. | |
Static Public Member Functions | |
static daeElementRef | create (daeInt bytes) |
Creates an instance of this class and returns a daeElementRef referencing it. | |
static daeMetaElement * | registerElement () |
Creates a daeMetaElement object that describes this element in the meta object reflection framework. | |
Static Public Attributes | |
static daeMetaElement * | _Meta = NULL |
The daeMetaElement that describes this element in the meta object reflection framework. | |
Protected Member Functions | |
domSource () | |
Constructor. | |
virtual | ~domSource () |
Destructor. | |
domSource (const domSource &cpy) | |
Copy Constructor. | |
virtual domSource & | operator= (const domSource &cpy) |
Overloaded assignment operator. | |
Protected Attributes | |
xsID | attrId |
The id attribute is a text string containing the unique identifier of the source element. | |
xsNCName | attrName |
The name attribute is the text string name of this element. | |
domArray_Array | elemArray_array |
domBool_array_Array | elemBool_array_array |
domFloat_array_Array | elemFloat_array_array |
domInt_array_Array | elemInt_array_array |
domName_array_Array | elemName_array_array |
domTechnique_Array | elemTechnique_array |
The technique element must occur one or more times. | |
daeElementRefArray | _contents |
Used to preserve order in elements that do not specify strict sequencing of sub-elements. | |
Classes | |
class | domTechnique |
The technique element must occur one or more times. More... |
Member Function Documentation
|
Creates an instance of this class and returns a daeElementRef referencing it.
|
|
Gets the array element array.
|
|
Gets the array element array.
|
|
Gets the bool_array element array.
|
|
Gets the bool_array element array.
|
|
Gets the _contents array.
|
|
Gets the _contents array.
|
|
Gets the float_array element array.
|
|
Gets the float_array element array.
|
|
Gets the id attribute.
|
|
Gets the int_array element array.
|
|
Gets the int_array element array.
|
|
Gets the name attribute.
|
|
Gets the Name_array element array.
|
|
Gets the Name_array element array.
|
|
Gets the technique element array.
|
|
Gets the technique element array.
|
|
Creates a daeMetaElement object that describes this element in the meta object reflection framework. If a daeMetaElement already exists it will return that instead of creating a new one.
|
|
Sets the id attribute.
|
|
Sets the name attribute.
|
Member Data Documentation
|
The id attribute is a text string containing the unique identifier of the source element. This value must be unique within the instance document. Optional attribute. |
|
The name attribute is the text string name of this element. Optional attribute. |
|
The technique element must occur one or more times. Within the technique element, the common profile's accessor elements describe the output of the source element. The output is assembled from the accessor elements in the order they are specified.
|
The documentation for this class was generated from the following files:
- C:/SVN_wf/COLLADA_DOM/include/1.3/dom/domSource.h
- C:/SVN_wf/COLLADA_DOM/src/1.3/dom/domSource.cpp
©2005 Sony Computer Entertainment Inc.. All Rights Reserved.
Generated on Fri Feb 10 16:50:05 2006 for COLLADA 1.4 DOM by
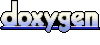