daeMetaElement Class Reference
#include <daeMetaElement.h>
Inheritance diagram for daeMetaElement:

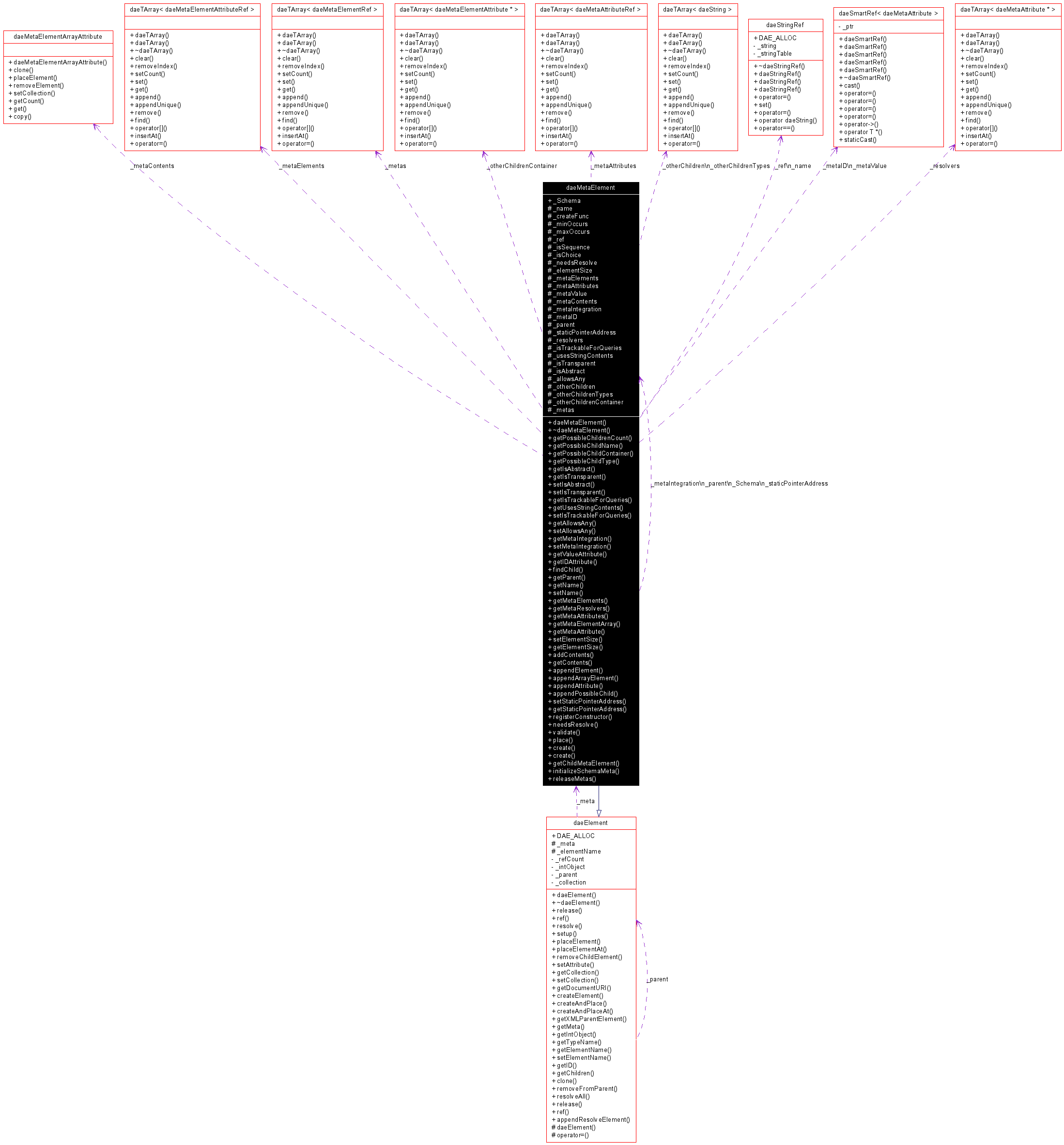
Detailed Description
Each instance of thedaeMetaElement
class describes a C++ COLLADA dom element type.
- The meta information in
daeMetaElement
is a combination of the information required to create and maintain C++ object instances and the information necessary to parse and construct a hierarchy of COLLADA elements.
daeMetaElement
objects also act as factories for C++ COLLADA dom classes where eachdaeElement
is capable of creating an instance of the class it describes. Further, eachdaeMetaElement
contains references to otherdaeMetaElements
for potential XML children elements. This enables this system to easily createdaeElements
of the appropriate type while navigating through XML recursive parse.
- See
daeElement
for information about the functionality that everydaeElement
implements.
Public Member Functions | |
daeMetaElement () | |
Constructor. | |
~daeMetaElement () | |
Destructor. | |
size_t | getPossibleChildrenCount () |
Gets the number of possible children of elements of this type that don't actually belong to this type. | |
daeString | getPossibleChildName (daeInt index) |
Gets the name of the possible child specified. | |
daeMetaElementAttribute * | getPossibleChildContainer (daeInt index) |
Gets the containing element for the possible child specified. | |
daeString | getPossibleChildType (daeInt index) |
Gets the type of the possible child specified. | |
daeBool | getIsAbstract () |
Determines if elements of this type can be placed in the object model. | |
daeBool | getIsTransparent () |
Determines if elements of this type should have an element tag printed when saving. | |
void | setIsAbstract (daeBool abstract) |
Sets if elements of this type are abstract. | |
void | setIsTransparent (daeBool transparent) |
Sets whether or not elements of this type should have an element tag printed when saving. | |
daeBool | getIsTrackableForQueries () |
Determines if elements of this type should be tracked for daeDatabase queries. | |
daeBool | getUsesStringContents () |
Gets whether elements of this type have "string" based contents; this is necessary to change the parsing mode for strings. | |
void | setIsTrackableForQueries (daeBool trackable) |
Sets whether elements of this type should be tracked for daeDatabase queries. | |
daeBool | getAllowsAny () |
Determines if elements of this type allow for any element as a child. | |
void | setAllowsAny (daeBool allows) |
Sets if elements of this type allow for any element as a child. | |
daeMetaElement * | getMetaIntegration () |
Gets the daeMetaElement for the corresponding integration object associated with this COLLADA element (if any). | |
void | setMetaIntegration (daeMetaElement *mI) |
Sets the daeMetaElement for the corresponding integration object associated with this COLLADA element (if any). | |
daeMetaAttribute * | getValueAttribute () |
Gets the daeMetaAttribute for the non-element contents of a daeElement . | |
daeMetaAttribute * | getIDAttribute () |
Gets the daeMetaAttribute for the ID attribute of a daeElement . | |
daeMetaElement * | findChild (daeString elementName) |
Gets the daeMetaElement associated with a child element of a given element type. | |
daeMetaElement * | getParent () |
Gets the container of this element type as defined by the COLLADA's XML schema. | |
daeStringRef | getName () |
Gets the name of this element type. | |
void | setName (daeString s) |
Sets the name of this element type. | |
daeMetaElementAttributeArray & | getMetaElements () |
Gets the array of element attributes associated with this element type. | |
daeMetaAttributePtrArray & | getMetaResolvers () |
Gets the array of attributes that represent URI fields that need to be "resolved" after the database is completely read in. | |
daeMetaAttributeRefArray & | getMetaAttributes () |
Gets the array of all known attributes on this element type. | |
daeMetaElementAttributeArray & | getMetaElementArray () |
Gets the array of element attributes associated with this element type. | |
daeMetaAttribute * | getMetaAttribute (daeString s) |
Gets the attribute which has a name as provided by the s parameter. | |
void | setElementSize (daeInt size) |
Sets the size in bytes of each instance of this element type. | |
daeInt | getElementSize () |
Gets the size in bytes of each instance of this element type. | |
void | addContents (daeInt offset) |
Resisters with the reflective object system that the dom class described by this daeMetaElement contains a _contents array. | |
daeMetaElementArrayAttribute * | getContents () |
Gets the attribute associated with the contents meta information. | |
void | appendElement (daeMetaElement *metaElement, daeInt offset, daeString name=NULL) |
Appends another element type to be a potential child element of this element type. | |
void | appendArrayElement (daeMetaElement *metaElement, daeInt offset, daeString name=NULL) |
Appends the potential child element as a list of potential child elements rather than as a singleton. | |
void | appendAttribute (daeMetaAttribute *attr) |
Appends a daeMetaAttribute that represents a field corresponding to an XML attribute to the C++ version of this element type. | |
void | appendPossibleChild (daeString name, daeMetaElementAttribute *cont, daeString type=NULL) |
Appends a possible child and maps the name to the actual container. | |
void | setStaticPointerAddress (daeMetaElement **addr) |
Sets the address where the static pointer lives for this element type's daeMetaElement . | |
daeMetaElement ** | getStaticPointerAddress () |
Gets the address where the static pointer lives for this element type's daeMetaElement . | |
void | registerConstructor (daeElementConstructFunctionPtr func) |
Registers the function that can construct a C++ instance of this class. | |
daeBool | needsResolve () |
Determines if this element contains attributes of type daeURI which need to be resolved after they are read or setup. | |
void | validate () |
Validates this class to be used by the runtime c++ object model including factory creation. | |
daeBool | place (daeElementRef parent, daeElementRef child) |
Places a child element into the parent element where the calling object is the daeMetaElement for the parent element. | |
daeElementRef | create () |
Invokes the factory element creation routine set by registerConstructor() to return a C++ COLLADA Object Model instance of this element type. | |
daeElementRef | create (daeString childElementTypeName) |
Looks through the list of potential child elements for this element type finding the corresponding element type; if a corresponding element type is found, use that type as a factory and return an instance of that child type. | |
daeMetaElement * | getChildMetaElement (daeString s) |
Gets the meta information for a given subelement. | |
Static Public Member Functions | |
static void | initializeSchemaMeta () |
Empty no-op function. | |
static void | releaseMetas () |
Releases all of the meta information contained in daeMetaElements . | |
Static Public Attributes | |
static daeMetaElement * | _Schema = NULL |
Unused. | |
Protected Attributes | |
daeStringRef | _name |
daeElementConstructFunctionPtr | _createFunc |
daeInt | _minOccurs |
daeInt | _maxOccurs |
daeStringRef | _ref |
daeBool | _isSequence |
daeBool | _isChoice |
daeBool | _needsResolve |
daeInt | _elementSize |
daeMetaElementAttributeArray | _metaElements |
daeMetaAttributeRefArray | _metaAttributes |
daeMetaAttributeRef | _metaValue |
daeMetaElementArrayAttribute * | _metaContents |
daeMetaElement * | _metaIntegration |
daeMetaAttributeRef | _metaID |
daeMetaElement * | _parent |
daeMetaElement ** | _staticPointerAddress |
daeMetaAttributePtrArray | _resolvers |
daeBool | _isTrackableForQueries |
daeBool | _usesStringContents |
daeBool | _isTransparent |
daeBool | _isAbstract |
daeBool | _allowsAny |
daeStringArray | _otherChildren |
daeStringArray | _otherChildrenTypes |
daeTArray< daeMetaElementAttribute * > | _otherChildrenContainer |
Static Protected Attributes | |
static daeMetaElementRefArray | _metas |
Member Function Documentation
|
Resisters with the reflective object system that the dom class described by this
This method is only for
|
|
Appends the potential child element as a list of potential child elements rather than as a singleton.
|
|
Appends a
|
|
Appends another element type to be a potential child element of this element type.
|
|
Appends a possible child and maps the name to the actual container.
|
|
Looks through the list of potential child elements for this element type finding the corresponding element type; if a corresponding element type is found, use that type as a factory and return an instance of that child type.
Typically
|
|
Invokes the factory element creation routine set by
|
|
Gets the
|
|
Determines if elements of this type allow for any element as a child.
|
|
Gets the meta information for a given subelement.
|
|
Gets the attribute associated with the contents meta information.
|
|
Gets the size in bytes of each instance of this element type. Used for factory element creation.
|
|
Gets the
|
|
Determines if elements of this type can be placed in the object model.
|
|
Determines if elements of this type should be tracked for daeDatabase queries.
|
|
Determines if elements of this type should have an element tag printed when saving.
|
|
Gets the attribute which has a name as provided by the
|
|
Gets the array of all known attributes on this element type. This includes all meta attributes except those describing child elements. It does include the value element.
|
|
Gets the array of element attributes associated with this element type.
|
|
Gets the array of element attributes associated with this element type.
|
|
Gets the
|
|
Gets the array of attributes that represent URI fields that need to be "resolved" after the database is completely read in.
|
|
Gets the name of this element type.
|
|
Gets the container of this element type as defined by the COLLADA's XML schema. This parent type controls where this element can be directly inlined inside of another element. Although an element can be referred to in multiple places, it is only included in one; thus a single parent.
|
|
Gets the containing element for the possible child specified.
|
|
Gets the name of the possible child specified.
|
|
Gets the number of possible children of elements of this type that don't actually belong to this type.
|
|
Gets the type of the possible child specified.
|
|
Gets the address where the static pointer lives for this element type's
For instance,
|
|
Gets whether elements of this type have "string" based contents; this is necessary to change the parsing mode for strings.
|
|
Gets the
This corresponds to a
|
|
Determines if this element contains attributes of type
|
|
Places a child element into the
|
|
Registers the function that can construct a C++ instance of this class.
Necessary for the factory system such that C++ can still call
|
|
Sets if elements of this type allow for any element as a child.
|
|
Sets the size in bytes of each instance of this element type. Used for factory element creation.
|
|
Sets if elements of this type are abstract.
|
|
Sets whether elements of this type should be tracked for
|
|
Sets whether or not elements of this type should have an element tag printed when saving.
|
|
Sets the
|
|
Sets the name of this element type.
|
|
Sets the address where the static pointer lives for this element type's
For instance,
|
The documentation for this class was generated from the following files:
- C:/SVN_wf/COLLADA_DOM/include/dae/daeMetaElement.h
- C:/SVN_wf/COLLADA_DOM/src/dae/daeMetaElement.cpp
©2005 Sony Computer Entertainment Inc.. All Rights Reserved.
Generated on Fri Feb 10 16:47:03 2006 for COLLADA 1.4 DOM by
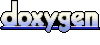