domNode Class Reference
#include <domNode.h>
Inheritance diagram for domNode:

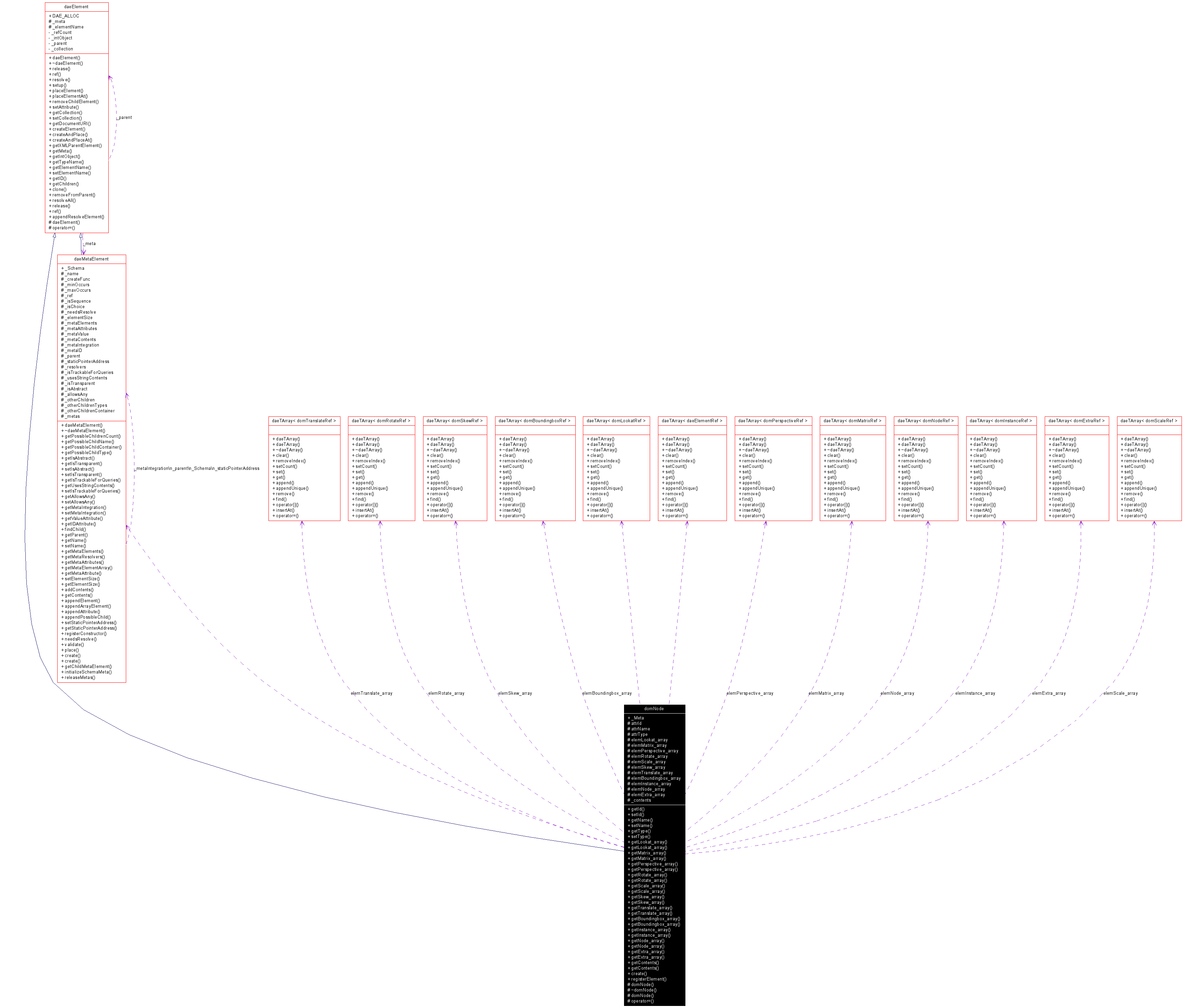
Detailed Description
Nodes embody the hierarchical relationship of elements in the scene.The node element declares a point of interest in the scene. A node denotes one point on a branch of the scene graph. The node element is essentially the root of a sub graph of the entire scene graph. The node element represents a context in which the child transform elements are composed in the order that they occur. All the other child elements are affected equally by the accumulated transform in the scope of the node element. The transform elements transform the coordinate system of the node element. Mathematically, this means that the transform elements are converted to matrices and post-multiplied in the order they are specified to compose the coordinate system.
Public Member Functions | |
xsID | getId () const |
Gets the id attribute. | |
void | setId (xsID atId) |
Sets the id attribute. | |
xsNCName | getName () const |
Gets the name attribute. | |
void | setName (xsNCName atName) |
Sets the name attribute. | |
domNodeType | getType () const |
Gets the type attribute. | |
void | setType (domNodeType atType) |
Sets the type attribute. | |
domLookat_Array & | getLookat_array () |
Gets the lookat element array. | |
const domLookat_Array & | getLookat_array () const |
Gets the lookat element array. | |
domMatrix_Array & | getMatrix_array () |
Gets the matrix element array. | |
const domMatrix_Array & | getMatrix_array () const |
Gets the matrix element array. | |
domPerspective_Array & | getPerspective_array () |
Gets the perspective element array. | |
const domPerspective_Array & | getPerspective_array () const |
Gets the perspective element array. | |
domRotate_Array & | getRotate_array () |
Gets the rotate element array. | |
const domRotate_Array & | getRotate_array () const |
Gets the rotate element array. | |
domScale_Array & | getScale_array () |
Gets the scale element array. | |
const domScale_Array & | getScale_array () const |
Gets the scale element array. | |
domSkew_Array & | getSkew_array () |
Gets the skew element array. | |
const domSkew_Array & | getSkew_array () const |
Gets the skew element array. | |
domTranslate_Array & | getTranslate_array () |
Gets the translate element array. | |
const domTranslate_Array & | getTranslate_array () const |
Gets the translate element array. | |
domBoundingbox_Array & | getBoundingbox_array () |
Gets the boundingbox element array. | |
const domBoundingbox_Array & | getBoundingbox_array () const |
Gets the boundingbox element array. | |
domInstance_Array & | getInstance_array () |
Gets the instance element array. | |
const domInstance_Array & | getInstance_array () const |
Gets the instance element array. | |
domNode_Array & | getNode_array () |
Gets the node element array. | |
const domNode_Array & | getNode_array () const |
Gets the node element array. | |
domExtra_Array & | getExtra_array () |
Gets the extra element array. | |
const domExtra_Array & | getExtra_array () const |
Gets the extra element array. | |
daeElementRefArray & | getContents () |
Gets the _contents array. | |
const daeElementRefArray & | getContents () const |
Gets the _contents array. | |
Static Public Member Functions | |
static daeElementRef | create (daeInt bytes) |
Creates an instance of this class and returns a daeElementRef referencing it. | |
static daeMetaElement * | registerElement () |
Creates a daeMetaElement object that describes this element in the meta object reflection framework. | |
Static Public Attributes | |
static daeMetaElement * | _Meta = NULL |
The daeMetaElement that describes this element in the meta object reflection framework. | |
Protected Member Functions | |
domNode () | |
Constructor. | |
virtual | ~domNode () |
Destructor. | |
domNode (const domNode &cpy) | |
Copy Constructor. | |
virtual domNode & | operator= (const domNode &cpy) |
Overloaded assignment operator. | |
Protected Attributes | |
xsID | attrId |
The id attribute is a text string containing the unique identifier of the node element. | |
xsNCName | attrName |
The name attribute is a text string containing the name of the node element. | |
domNodeType | attrType |
The type attribute indicates the type of the node element. | |
domLookat_Array | elemLookat_array |
Allows the node to express a lookat transform. | |
domMatrix_Array | elemMatrix_array |
Allows the node to express a matrix transform. | |
domPerspective_Array | elemPerspective_array |
Allows the node to express a perspective transform. | |
domRotate_Array | elemRotate_array |
Allows the node to express a rotational transform. | |
domScale_Array | elemScale_array |
Allows the node to express a scale transform. | |
domSkew_Array | elemSkew_array |
Allows the node to express a skew transform. | |
domTranslate_Array | elemTranslate_array |
Allows the node to express a translational transform. | |
domBoundingbox_Array | elemBoundingbox_array |
Allows the node to express a bounding box. | |
domInstance_Array | elemInstance_array |
Allows the node to instantiate another copy of an object. | |
domNode_Array | elemNode_array |
Allows the node to recursively define hierarchy. | |
domExtra_Array | elemExtra_array |
Allows the node to define extra information. | |
daeElementRefArray | _contents |
Used to preserve order in elements that do not specify strict sequencing of sub-elements. |
Member Function Documentation
|
Creates an instance of this class and returns a daeElementRef referencing it.
|
|
Gets the boundingbox element array.
|
|
Gets the boundingbox element array.
|
|
Gets the _contents array.
|
|
Gets the _contents array.
|
|
Gets the extra element array.
|
|
Gets the extra element array.
|
|
Gets the id attribute.
|
|
Gets the instance element array.
|
|
Gets the instance element array.
|
|
Gets the lookat element array.
|
|
Gets the lookat element array.
|
|
Gets the matrix element array.
|
|
Gets the matrix element array.
|
|
Gets the name attribute.
|
|
Gets the node element array.
|
|
Gets the node element array.
|
|
Gets the perspective element array.
|
|
Gets the perspective element array.
|
|
Gets the rotate element array.
|
|
Gets the rotate element array.
|
|
Gets the scale element array.
|
|
Gets the scale element array.
|
|
Gets the skew element array.
|
|
Gets the skew element array.
|
|
Gets the translate element array.
|
|
Gets the translate element array.
|
|
Gets the type attribute.
|
|
Creates a daeMetaElement object that describes this element in the meta object reflection framework. If a daeMetaElement already exists it will return that instead of creating a new one.
|
|
Sets the id attribute.
|
|
Sets the name attribute.
|
|
Sets the type attribute.
|
Member Data Documentation
|
The id attribute is a text string containing the unique identifier of the node element. This value must be unique within the instance document. Optional attribute. |
|
The name attribute is a text string containing the name of the node element. Optional attribute. |
|
The type attribute indicates the type of the node element. The default value is 'NODE'. Optional attribute. |
|
Allows the node to express a bounding box.
|
|
Allows the node to define extra information.
|
|
Allows the node to instantiate another copy of an object.
|
|
Allows the node to express a lookat transform.
|
|
Allows the node to express a matrix transform.
|
|
Allows the node to recursively define hierarchy.
|
|
Allows the node to express a perspective transform.
|
|
Allows the node to express a rotational transform.
|
|
Allows the node to express a scale transform.
|
|
Allows the node to express a skew transform.
|
|
Allows the node to express a translational transform.
|
The documentation for this class was generated from the following files:
- C:/SVN_wf/COLLADA_DOM/include/1.3/dom/domNode.h
- C:/SVN_wf/COLLADA_DOM/src/1.3/dom/domNode.cpp
©2005 Sony Computer Entertainment Inc.. All Rights Reserved.
Generated on Fri Feb 10 16:49:15 2006 for COLLADA 1.4 DOM by
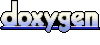