domLibrary Class Reference
#include <domLibrary.h>
Inheritance diagram for domLibrary:

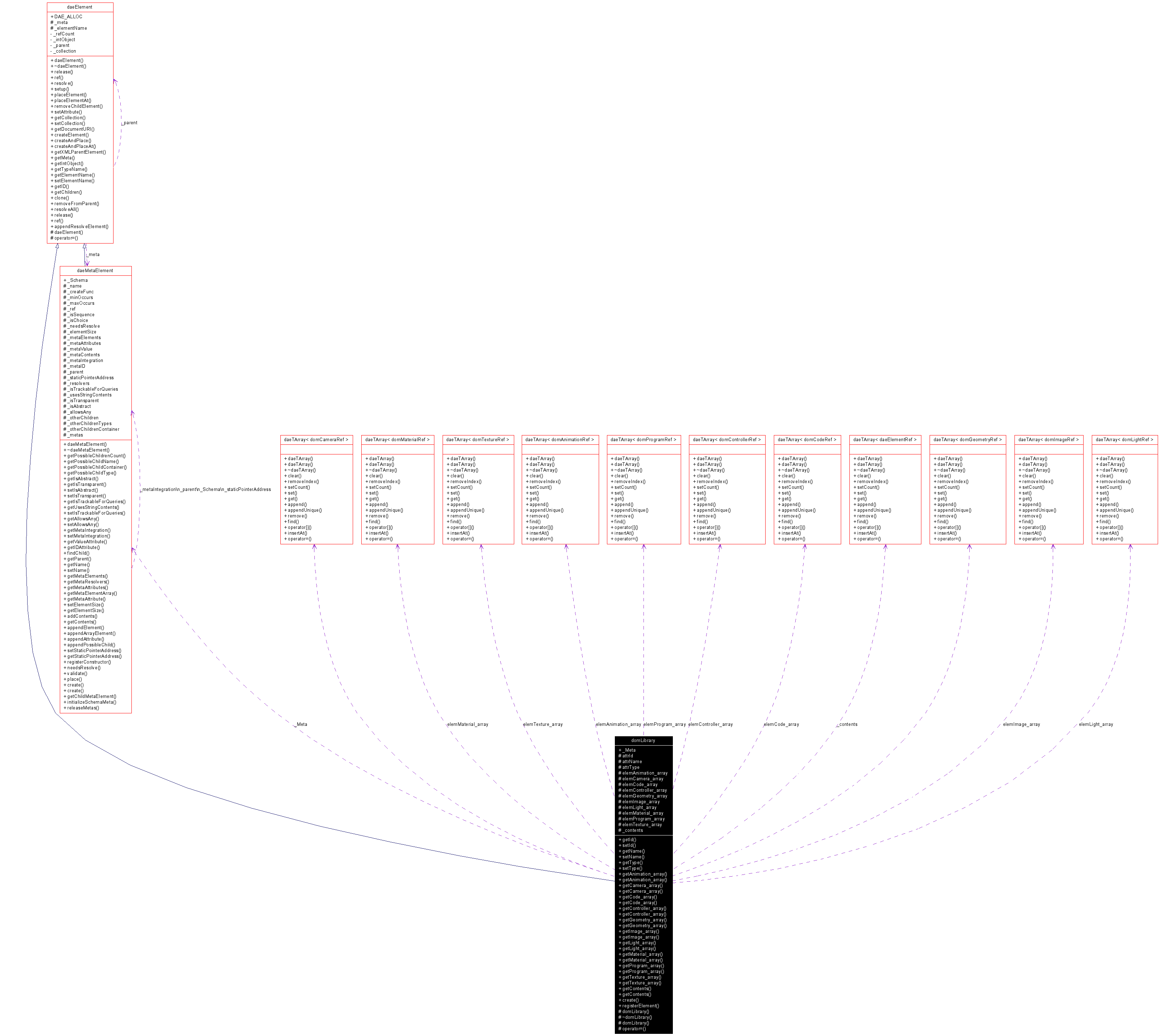
Detailed Description
The library element declares a module of elements of a single category.As data sets become larger and more complex they become harder to manipulate within a single container. One approach to manage this complexity is to divide the data into smaller pieces organized by some criteria. These modular pieces can then be stored in separate resources as libraries. The library element can have zero or more child elements that conform to the category of its type attribute. A library whose type attribute is 'MATERIAL' must contain only material elements, for example.
Public Member Functions | |
xsID | getId () const |
Gets the id attribute. | |
void | setId (xsID atId) |
Sets the id attribute. | |
xsNCName | getName () const |
Gets the name attribute. | |
void | setName (xsNCName atName) |
Sets the name attribute. | |
domLibraryType | getType () const |
Gets the type attribute. | |
void | setType (domLibraryType atType) |
Sets the type attribute. | |
domAnimation_Array & | getAnimation_array () |
Gets the animation element array. | |
const domAnimation_Array & | getAnimation_array () const |
Gets the animation element array. | |
domCamera_Array & | getCamera_array () |
Gets the camera element array. | |
const domCamera_Array & | getCamera_array () const |
Gets the camera element array. | |
domCode_Array & | getCode_array () |
Gets the code element array. | |
const domCode_Array & | getCode_array () const |
Gets the code element array. | |
domController_Array & | getController_array () |
Gets the controller element array. | |
const domController_Array & | getController_array () const |
Gets the controller element array. | |
domGeometry_Array & | getGeometry_array () |
Gets the geometry element array. | |
const domGeometry_Array & | getGeometry_array () const |
Gets the geometry element array. | |
domImage_Array & | getImage_array () |
Gets the image element array. | |
const domImage_Array & | getImage_array () const |
Gets the image element array. | |
domLight_Array & | getLight_array () |
Gets the light element array. | |
const domLight_Array & | getLight_array () const |
Gets the light element array. | |
domMaterial_Array & | getMaterial_array () |
Gets the material element array. | |
const domMaterial_Array & | getMaterial_array () const |
Gets the material element array. | |
domProgram_Array & | getProgram_array () |
Gets the program element array. | |
const domProgram_Array & | getProgram_array () const |
Gets the program element array. | |
domTexture_Array & | getTexture_array () |
Gets the texture element array. | |
const domTexture_Array & | getTexture_array () const |
Gets the texture element array. | |
daeElementRefArray & | getContents () |
Gets the _contents array. | |
const daeElementRefArray & | getContents () const |
Gets the _contents array. | |
Static Public Member Functions | |
static daeElementRef | create (daeInt bytes) |
Creates an instance of this class and returns a daeElementRef referencing it. | |
static daeMetaElement * | registerElement () |
Creates a daeMetaElement object that describes this element in the meta object reflection framework. | |
Static Public Attributes | |
static daeMetaElement * | _Meta = NULL |
The daeMetaElement that describes this element in the meta object reflection framework. | |
Protected Member Functions | |
domLibrary () | |
Constructor. | |
virtual | ~domLibrary () |
Destructor. | |
domLibrary (const domLibrary &cpy) | |
Copy Constructor. | |
virtual domLibrary & | operator= (const domLibrary &cpy) |
Overloaded assignment operator. | |
Protected Attributes | |
xsID | attrId |
The id attribute is a text string containing the unique identifier of the library element. | |
xsNCName | attrName |
The name attribute is the text string name of the element. | |
domLibraryType | attrType |
The type attribute indicates the category of child elements contained. | |
domAnimation_Array | elemAnimation_array |
domCamera_Array | elemCamera_array |
domCode_Array | elemCode_array |
domController_Array | elemController_array |
domGeometry_Array | elemGeometry_array |
domImage_Array | elemImage_array |
domLight_Array | elemLight_array |
domMaterial_Array | elemMaterial_array |
domProgram_Array | elemProgram_array |
domTexture_Array | elemTexture_array |
daeElementRefArray | _contents |
Used to preserve order in elements that do not specify strict sequencing of sub-elements. |
Member Function Documentation
|
Creates an instance of this class and returns a daeElementRef referencing it.
|
|
Gets the animation element array.
|
|
Gets the animation element array.
|
|
Gets the camera element array.
|
|
Gets the camera element array.
|
|
Gets the code element array.
|
|
Gets the code element array.
|
|
Gets the _contents array.
|
|
Gets the _contents array.
|
|
Gets the controller element array.
|
|
Gets the controller element array.
|
|
Gets the geometry element array.
|
|
Gets the geometry element array.
|
|
Gets the id attribute.
|
|
Gets the image element array.
|
|
Gets the image element array.
|
|
Gets the light element array.
|
|
Gets the light element array.
|
|
Gets the material element array.
|
|
Gets the material element array.
|
|
Gets the name attribute.
|
|
Gets the program element array.
|
|
Gets the program element array.
|
|
Gets the texture element array.
|
|
Gets the texture element array.
|
|
Gets the type attribute.
|
|
Creates a daeMetaElement object that describes this element in the meta object reflection framework. If a daeMetaElement already exists it will return that instead of creating a new one.
|
|
Sets the id attribute.
|
|
Sets the name attribute.
|
|
Sets the type attribute.
|
Member Data Documentation
|
The id attribute is a text string containing the unique identifier of the library element. This value must be unique within the instance document. Optional attribute. |
|
The name attribute is the text string name of the element. Optional attribute. |
|
The type attribute indicates the category of child elements contained. Required attribute. |
The documentation for this class was generated from the following files:
- C:/SVN_wf/COLLADA_DOM/include/1.3/dom/domLibrary.h
- C:/SVN_wf/COLLADA_DOM/src/1.3/dom/domLibrary.cpp
©2005 Sony Computer Entertainment Inc.. All Rights Reserved.
Generated on Fri Feb 10 16:48:43 2006 for COLLADA 1.4 DOM by
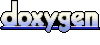