domImage Class Reference
#include <domImage.h>
Inheritance diagram for domImage:

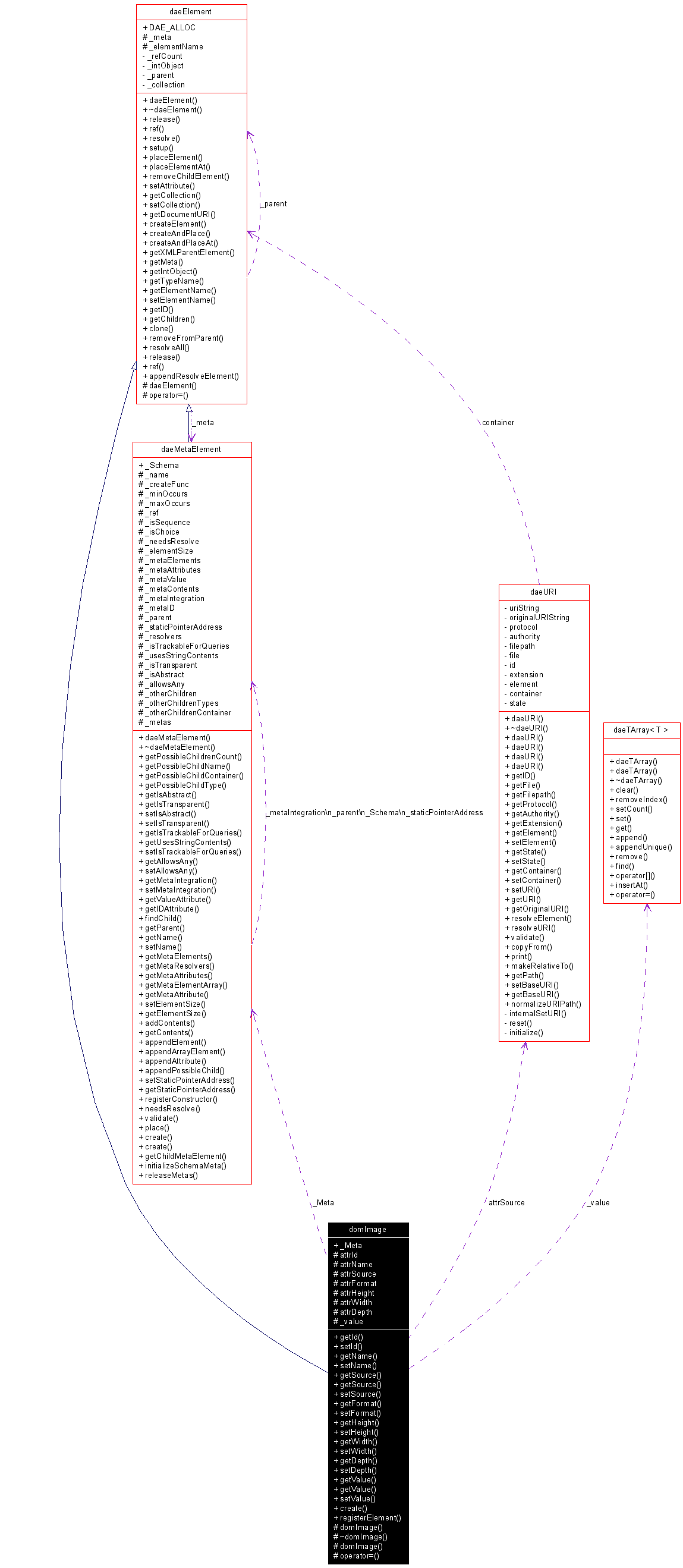
Detailed Description
Images embody the graphical representation of a scene or object.The image element declares the storage for the graphical representation of an object. The image element best describes raster image data, but can conceivably handle other forms of imagery. The image element may contain a sequence of hexadecimal, binary octets representing the embedded image data. These values are interpreted at pixel values according to the format attribute.
Public Member Functions | |
xsID | getId () const |
Gets the id attribute. | |
void | setId (xsID atId) |
Sets the id attribute. | |
xsNCName | getName () const |
Gets the name attribute. | |
void | setName (xsNCName atName) |
Sets the name attribute. | |
xsAnyURI & | getSource () |
Gets the source attribute. | |
const xsAnyURI & | getSource () const |
Gets the source attribute. | |
void | setSource (const xsAnyURI &atSource) |
Sets the source attribute. | |
xsString | getFormat () const |
Gets the format attribute. | |
void | setFormat (xsString atFormat) |
Sets the format attribute. | |
xsNonNegativeInteger | getHeight () const |
Gets the height attribute. | |
void | setHeight (xsNonNegativeInteger atHeight) |
Sets the height attribute. | |
xsNonNegativeInteger | getWidth () const |
Gets the width attribute. | |
void | setWidth (xsNonNegativeInteger atWidth) |
Sets the width attribute. | |
xsNonNegativeInteger | getDepth () const |
Gets the depth attribute. | |
void | setDepth (xsNonNegativeInteger atDepth) |
Sets the depth attribute. | |
domListOfHexBinary & | getValue () |
Gets the _value array. | |
const domListOfHexBinary & | getValue () const |
Gets the _value array. | |
void | setValue (const domListOfHexBinary &val) |
Sets the _value array. | |
Static Public Member Functions | |
static daeElementRef | create (daeInt bytes) |
Creates an instance of this class and returns a daeElementRef referencing it. | |
static daeMetaElement * | registerElement () |
Creates a daeMetaElement object that describes this element in the meta object reflection framework. | |
Static Public Attributes | |
static daeMetaElement * | _Meta = NULL |
The daeMetaElement that describes this element in the meta object reflection framework. | |
Protected Member Functions | |
domImage () | |
Constructor. | |
virtual | ~domImage () |
Destructor. | |
domImage (const domImage &cpy) | |
Copy Constructor. | |
virtual domImage & | operator= (const domImage &cpy) |
Overloaded assignment operator. | |
Protected Attributes | |
xsID | attrId |
The id attribute is a text string containing the unique identifier of the image element. | |
xsNCName | attrName |
The name attribute is the text string name of this element. | |
xsAnyURI | attrSource |
The source attribute is a valid URL of the image source data. | |
xsString | attrFormat |
The format attribute is a text string value that indicates the image format. | |
xsNonNegativeInteger | attrHeight |
The height attribute is an integer value that indicates the height of the image in pixel units. | |
xsNonNegativeInteger | attrWidth |
The width attribute is an integer value that indicates the width of the image in pixel units. | |
xsNonNegativeInteger | attrDepth |
The depth attribute is an integer value that indicates the depth of the image in pixel units. | |
domListOfHexBinary | _value |
The domListOfHexBinary value of the text data of this element. |
Member Function Documentation
|
Creates an instance of this class and returns a daeElementRef referencing it.
|
|
Gets the depth attribute.
|
|
Gets the format attribute.
|
|
Gets the height attribute.
|
|
Gets the id attribute.
|
|
Gets the name attribute.
|
|
Gets the source attribute.
|
|
Gets the source attribute.
|
|
Gets the _value array.
|
|
Gets the _value array.
|
|
Gets the width attribute.
|
|
Creates a daeMetaElement object that describes this element in the meta object reflection framework. If a daeMetaElement already exists it will return that instead of creating a new one.
|
|
Sets the depth attribute.
|
|
Sets the format attribute.
|
|
Sets the height attribute.
|
|
Sets the id attribute.
|
|
Sets the name attribute.
|
|
Sets the source attribute.
|
|
Sets the _value array.
|
|
Sets the width attribute.
|
Member Data Documentation
|
The depth attribute is an integer value that indicates the depth of the image in pixel units. A 2-D image has a depth of 1, which is also the default value. Optional attribute. |
|
The format attribute is a text string value that indicates the image format. Optional attribute. |
|
The height attribute is an integer value that indicates the height of the image in pixel units. Optional attribute. |
|
The id attribute is a text string containing the unique identifier of the image element. This value must be unique within the instance document. Optional attribute. |
|
The name attribute is the text string name of this element. Optional attribute. |
|
The source attribute is a valid URL of the image source data. If this attribute is present, then it refers to an image asset that may contain information about the dimensions and format of the image. Optional attribute. |
|
The width attribute is an integer value that indicates the width of the image in pixel units. Optional attribute. |
The documentation for this class was generated from the following files:
- C:/SVN_wf/COLLADA_DOM/include/1.3/dom/domImage.h
- C:/SVN_wf/COLLADA_DOM/src/1.3/dom/domImage.cpp
©2005 Sony Computer Entertainment Inc.. All Rights Reserved.
Generated on Fri Feb 10 16:48:25 2006 for COLLADA 1.4 DOM by
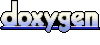