daeSTLDatabase Class Reference
#include <daeSTLDatabase.h>
Inheritance diagram for daeSTLDatabase:

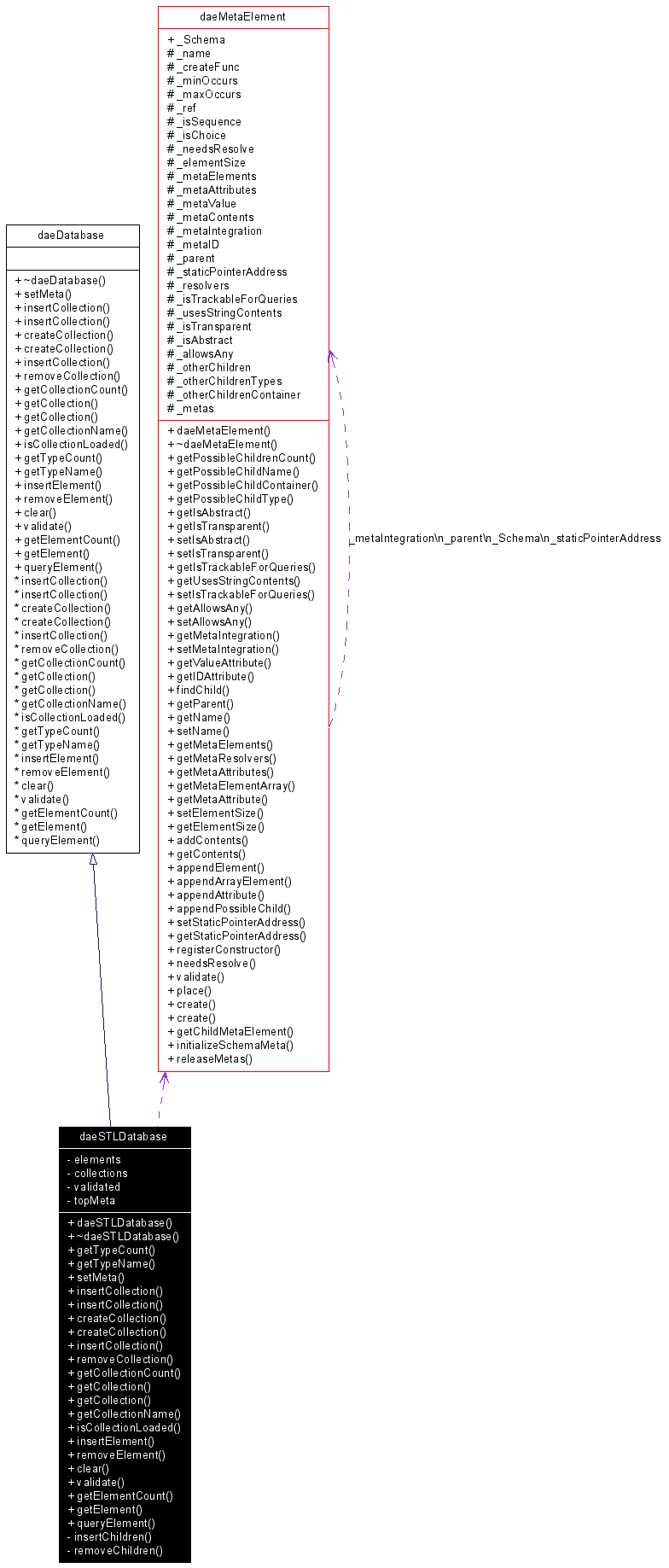
Detailed Description
ThedaeSTLDatabase
class derives from daeDatabase
and implements the default database.
Public Member Functions | |
daeSTLDatabase () | |
Constructor. | |
virtual | ~daeSTLDatabase () |
Destructor. | |
virtual daeUInt | getTypeCount () |
Gets the number of types in the database. | |
virtual daeString | getTypeName (daeUInt index) |
Retrieves the name of a type of object inserted in the database. | |
virtual daeInt | setMeta (daeMetaElement *_topMeta) |
Sets the top meta object. | |
virtual daeInt | insertCollection (const char *name, daeElement *dom, daeCollection **collection=NULL) |
Creates a new collection, defining its root as the dom object; returns an error if the collection name already exists. | |
virtual daeInt | insertCollection (daeString name, daeCollection **collection=NULL) |
Creates a new domCOLLADA root element and a new collection; returns an error if the collection name already exists. | |
virtual daeInt | createCollection (daeString name, daeElement *dom, daeCollection **collection=NULL) |
Creates a new collection, defining its root as the dom object; returns an error if the collection name already exists. | |
virtual daeInt | createCollection (daeString name, daeCollection **collection=NULL) |
Creates a new domCOLLADA root element and a new collection; returns an error if the collection name already exists. | |
virtual daeInt | insertCollection (daeCollection *c) |
Inserts an already existing collection into the database. | |
virtual daeInt | removeCollection (daeCollection *collection) |
Removes a collection from the database. | |
virtual daeUInt | getCollectionCount () |
Gets the number of collections. | |
virtual daeCollection * | getCollection (daeUInt index) |
Gets a collection based on the collection index. | |
virtual daeCollection * | getCollection (daeString name) |
Gets a collection based on the collection name. | |
virtual daeString | getCollectionName (daeUInt index) |
Gets a collection name. | |
virtual daeBool | isCollectionLoaded (daeString name) |
Indicates if a collection is loaded or not. | |
virtual daeInt | insertElement (daeCollection *collection, daeElement *element) |
Inserts a daeElement into the runtime database. | |
virtual daeInt | removeElement (daeCollection *collection, daeElement *element) |
Removes a daeElement from the runtime database; not implemented in the reference STL implementation. | |
virtual daeInt | clear () |
Unloads all of the collections of the runtime database. | |
virtual void | validate () |
Optimizes the database. | |
virtual daeUInt | getElementCount (daeString name=NULL, daeString type=NULL, daeString file=NULL) |
Gets the number of daeElement objects that match the search criteria Any combination of search criteria can be NULL, if a criterion is NULL all the parameters will match for this criterion. | |
virtual daeInt | getElement (daeElement **pElement, daeInt index, daeString name=NULL, daeString type=NULL, daeString file=NULL) |
Returns the daeElement which matches the search criteria. | |
virtual daeInt | queryElement (daeElement **pElement, daeString genericQuery) |
Returns the daeElement which matches the genericQuery parameter; not implemented. |
Member Function Documentation
|
Unloads all of the collections of the runtime database.
This function frees all the
Implements daeDatabase. |
|
Creates a new
Implements daeDatabase. |
|
Creates a new collection, defining its root as the
Implements daeDatabase. |
|
Gets a collection based on the collection name.
Implements daeDatabase. |
|
Gets a collection based on the collection index.
Implements daeDatabase. |
|
Gets the number of collections.
Implements daeDatabase. |
|
Gets a collection name.
Implements daeDatabase. |
|
Returns the
Any combination of search criteria can be NULL, if a criterion is NULL all the parameters will match for this criterion. The function operates on the set of assets that match the
Implements daeDatabase. |
|
Gets the number of daeElement objects that match the search criteria Any combination of search criteria can be NULL, if a criterion is NULL all the parameters will match for this criterion.
Hence
Implements daeDatabase. |
|
Gets the number of types in the database.
Implements daeDatabase. |
|
Retrieves the name of a type of object inserted in the database.
Implements daeDatabase. |
|
Inserts an already existing collection into the database.
Implements daeDatabase. |
|
Creates a new
Implements daeDatabase. |
|
Creates a new collection, defining its root as the
Implements daeDatabase. |
|
Inserts a
Implements daeDatabase. |
|
Indicates if a collection is loaded or not.
Implements daeDatabase. |
|
Returns the
Implements daeDatabase. |
|
Removes a collection from the database.
Implements daeDatabase. |
|
Removes a
Implements daeDatabase. |
|
Sets the top meta object.
Called by
Implements daeDatabase. |
|
Optimizes the database.
This function takes time; it is called by the interface at the end of a load operation. Some databases cannot be queried when items are being inserted; for instance, they may need to be sorted. All database search functions call Implements daeDatabase. |
The documentation for this class was generated from the following files:
- C:/SVN_wf/COLLADA_DOM/include/modules/daeSTLDatabase.h
- C:/SVN_wf/COLLADA_DOM/src/modules/STLDatabase/daeSTLDatabase.cpp
©2005 Sony Computer Entertainment Inc.. All Rights Reserved.
Generated on Fri Feb 10 16:47:04 2006 for COLLADA 1.4 DOM by
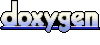