daeMetaAttribute Class Reference
#include <daeMetaAttribute.h>
Inheritance diagram for daeMetaAttribute:
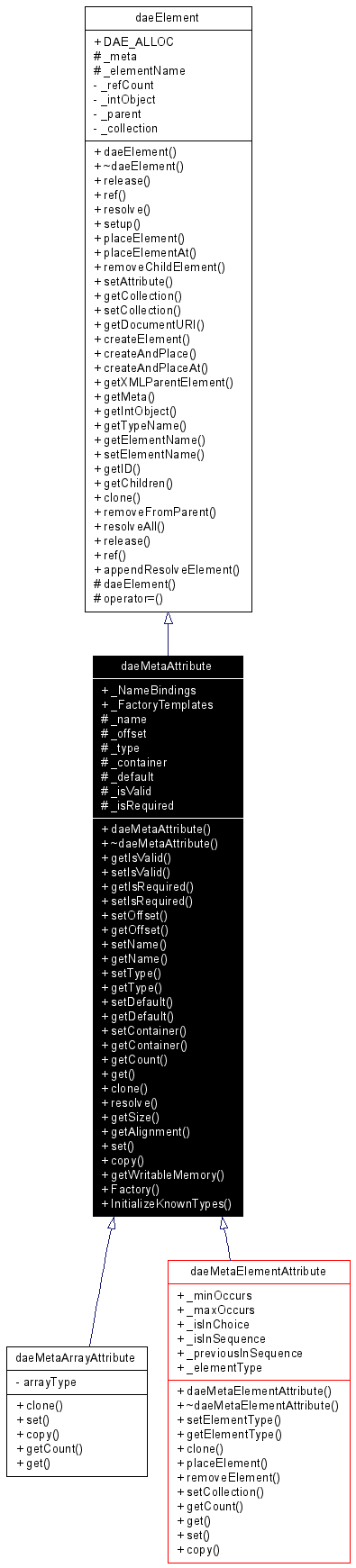
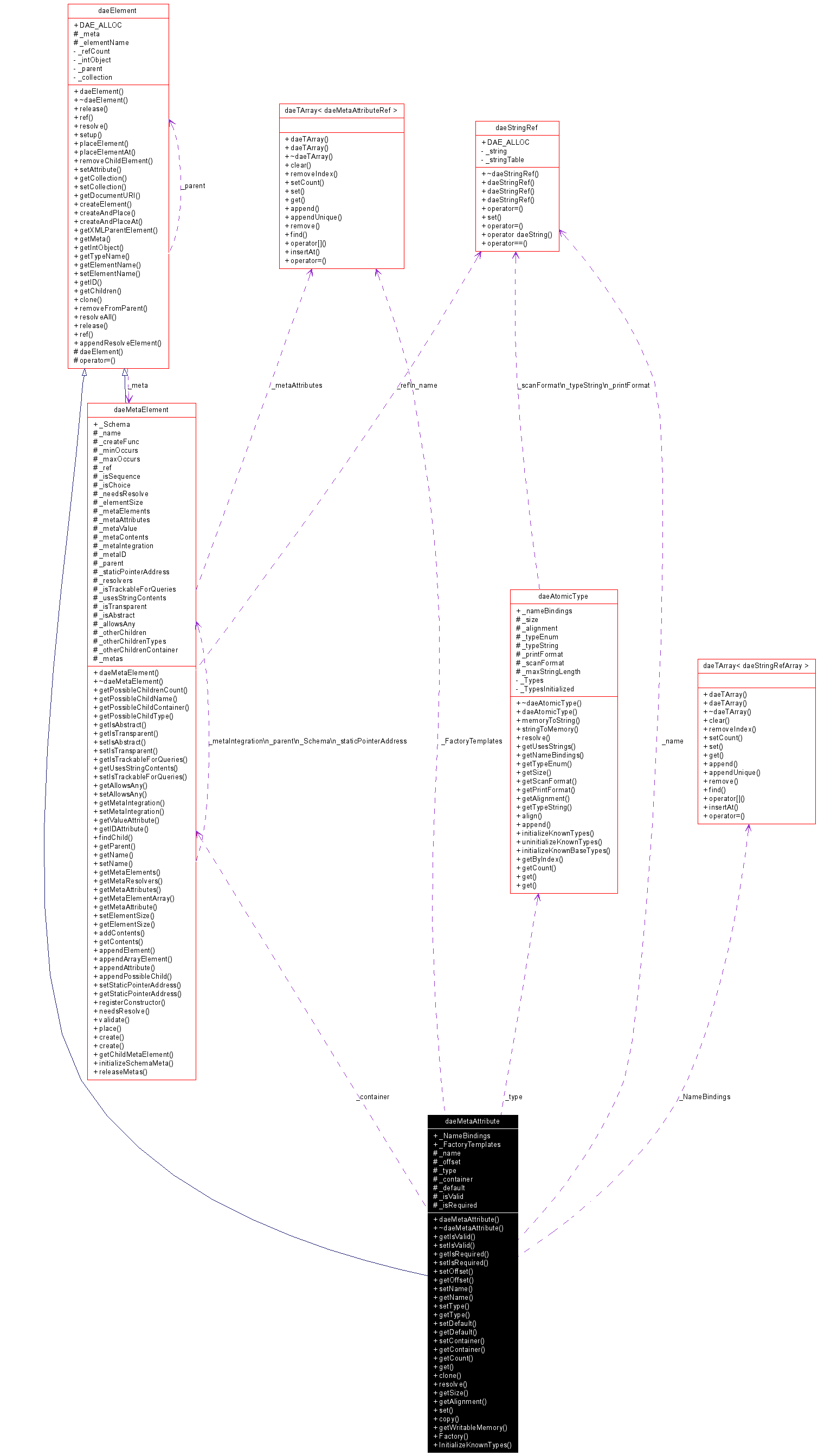
Detailed Description
ThedaeMetaAttribute
class describes one attribute in a C++ COLLADA dom element.
In the case of the C++ object model a conceptual attribute can be either a dom attribute, a dom element, or a dom value. Essentially, the meta attribute describes fields on the C++ class. However these attributes are stored separately in the containing meta daeMetaElement
. daeMetaAttributes
always exist inside of daeMetaElements
. Each daeMetaAttribute
has certain symantic operations it is capable of including set()
, get()
, print()
, and resolve()
. daeMetaAttributes
use the daeAtomicType
system as their underlying semantic implementation, but contain additional information about the packaging of the atomic types into the C++ dom classes such as offset, and array information.
Public Member Functions | |
daeMetaAttribute () | |
Constructor. | |
~daeMetaAttribute () | |
Destructor. | |
daeBool | getIsValid () |
Determines if the value of this attribute was ever set. | |
void | setIsValid (daeBool isValid) |
Sets the value that indicates if this attribute contains a valid value. | |
daeBool | getIsRequired () |
Determines if the schema indicates that this is a required attribute. | |
void | setIsRequired (daeBool isRequired) |
Sets the value that indicates that this attribute is required by the schema. | |
void | setOffset (daeInt offset) |
Sets the byte offset (from this ) where this attribute's storage is found in its container element class. | |
daeInt | getOffset () |
Gets the byte offset (from @ this) where this attribute's storage is found in its container element class. | |
void | setName (daeString name) |
Sets the name of the attribute. | |
daeStringRef | getName () |
Gets the name of this attribute. | |
void | setType (daeAtomicType *type) |
Sets the type of the attribute. | |
daeAtomicType * | getType () |
Gets the daeAtomicType used by this attribute. | |
void | setDefault (daeString defaultVal) |
Sets the default for this attribute via a string. | |
daeString | getDefault () |
Gets the default for this attribute via a string. | |
void | setContainer (daeMetaElement *container) |
Sets the containing daeMetaElement for this attribute. | |
daeMetaElement * | getContainer () |
Gets the containing daeMetaElement for this attribute. | |
virtual daeInt | getCount (daeElement *e) |
Gets the number of particles associated with this attribute in instance e. | |
virtual daeMemoryRef | get (daeElement *e, daeInt index) |
Gets a particle from containing element e based on index. | |
virtual daeMetaAttributeRef | clone () |
Clones the daeMetaAttribute . | |
virtual void | resolve (daeElementRef elem) |
Resolves a reference (if there is one) in the attribute type; only useful for reference types. | |
virtual daeInt | getSize () |
Gets the number of bytes for this attribute. | |
virtual daeInt | getAlignment () |
Gets the alignment in bytes on the class of this meta attribute type. | |
virtual void | set (daeElement *element, daeString s) |
Sets the value of this attribute on element by converting string s to a binary value and assigning it via the underlying daeAtomicType system. | |
virtual void | copy (daeElement *toElement, daeElement *fromElement) |
Copys the value of this attribute from fromElement into toElement. | |
daeChar * | getWritableMemory (daeElement *e) |
Gets the storage inside of e associated with this attribute. | |
Static Public Member Functions | |
static daeMetaAttributeRef | Factory (daeStringRef xmlTypeName) |
Obsolete. | |
static void | InitializeKnownTypes () |
Obsolete. | |
Static Public Attributes | |
static daeStringRefArrayArray | _NameBindings |
Lists the type names that can be created by factories and indicates which _FactoryTemplates to use for each type. | |
static daeMetaAttributeRefArray | _FactoryTemplates |
Points to the factory objects used to construct various types of attributes, _NameBindings specifies which type names are bound to which factories. | |
Protected Attributes | |
daeStringRef | _name |
daeInt | _offset |
daeAtomicType * | _type |
daeMetaElement * | _container |
daeString | _default |
daeBool | _isValid |
daeBool | _isRequired |
Member Function Documentation
|
Clones the
Reimplemented in daeMetaElementAttribute, daeMetaElementArrayAttribute, and daeMetaArrayAttribute. |
|
Copys the value of this attribute from fromElement into toElement.
Reimplemented in daeMetaElementAttribute, daeMetaElementArrayAttribute, and daeMetaArrayAttribute. |
|
Gets a particle from containing element
Reimplemented in daeMetaElementAttribute, daeMetaElementArrayAttribute, and daeMetaArrayAttribute. |
|
Gets the alignment in bytes on the class of this meta attribute type.
|
|
Gets the containing
|
|
Gets the number of particles associated with this attribute in instance
Reimplemented in daeMetaElementAttribute, daeMetaElementArrayAttribute, and daeMetaArrayAttribute. |
|
Gets the default for this attribute via a string. The attribute's type is used to convert the string into a binary value inside of an element.
|
|
Determines if the schema indicates that this is a required attribute.
|
|
Determines if the value of this attribute was ever set.
This will be the case if
|
|
Gets the name of this attribute.
|
|
Gets the byte offset (from @ this) where this attribute's storage is found in its container element class.
|
|
Gets the number of bytes for this attribute.
|
|
Gets the
|
|
Gets the storage inside of It is very useful for performing generic processing on elements and attributes from external tools regardless of element and attribute type.
|
|
Resolves a reference (if there is one) in the attribute type; only useful for reference types.
|
|
Sets the value of this attribute on
Reimplemented in daeMetaElementAttribute, and daeMetaArrayAttribute. |
|
Sets the containing
|
|
Sets the default for this attribute via a string. The attribute's type is used to convert the string into a binary value inside of an element.
|
|
Sets the value that indicates that this attribute is required by the schema. If set, the attribute will always be exported by the API regardless of its value.
|
|
Sets the value that indicates if this attribute contains a valid value. If you don't set this on an optional attribute, that attribute will not be written.
|
|
Sets the name of the attribute.
|
|
Sets the byte offset (from
|
|
Sets the type of the attribute.
|
The documentation for this class was generated from the following files:
- C:/SVN_wf/COLLADA_DOM/include/dae/daeMetaAttribute.h
- C:/SVN_wf/COLLADA_DOM/src/dae/daeMetaAttribute.cpp
©2005 Sony Computer Entertainment Inc.. All Rights Reserved.
Generated on Fri Feb 10 16:47:03 2006 for COLLADA 1.4 DOM by
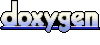