daeURI Class Reference
#include <daeURI.h>
Collaboration diagram for daeURI:

Detailed Description
ThedaeURI
is a simple class designed to aid in the parsing and resolution of URI references inside COLLADA elements.
A daeURI
is created for every anyURL
and IDREF
in the COLLADA schema. For example, the <instance> element has the url= attribute of type anyURL
, and the <controller> element has the target= attribute of type IDREF
. The daeURI
class contains a URI string; the setURI()
method breaks the string into its components including protocol, authority, path (directory), and ID. It also has the capability to attempt to resolve this reference into a daeElement
, through the method resolveElement()
. If a daeURI
is stored within a daeElement
, it fills its container field to point to the containing element.
The main API on the daeURI
, resolveElement()
, uses a daeURIResolver
to search for the daeElement
inside a daeDatabase
.
URIs are resolved hierarchically, where each URI is resolved based on the following criteria via itself and its element's base URI, which represents the URI of the collection that contains the element, retrieved by daeElement::getBaseURI().
If no base URI is provided, then the application URI is used as a base.
The URI resolution order for the COLLADA DOM is as follows:
- Absolute URI is specified (see definition below): The URI ignores its parent/base URI when validating.
- Relative URI is specified: The URI uses the base URI to provide the protocol, authority, and base path. This URI's path is appended to the path given the the base URI. This URI's file and ID are used.
- Each level of URI is resolved in this way against the base URI of the containing file until the top level is reached. Then the application URI is used as the default.
Definition of Absolute URI: For the purposes of the COLLADA DOM, a URI is considered absolute if it starts by specifying a protocol. For example,
- file://c:/data/foo.dae#myScene is an absolute URI.
- foo.dae::myScene is relative.
- foo.dae is a top-level file reference and is relative. If the URI does not include a pound sign (#), the
id
is empty.
Public Types | |
enum | ResolveState { uri_empty, uri_loaded, uri_pending, uri_success, uri_failed_unsupported_protocol, uri_failed_file_not_found, uri_failed_id_not_found, uri_failed_invalid_id, uri_resolve_local, uri_resolve_relative, uri_resolve_absolute, uri_failed_invalid_reference, uri_failed_externalization, uri_failed_missing_container } |
An enum describing the status of the URI resolution process. More... | |
Public Member Functions | |
daeURI () | |
Constructs a daeURI object that contains no URI reference. | |
~daeURI () | |
Destructor. | |
daeURI (int dummy) | |
Constructs a daeURI object that points to the application's current working directory. | |
daeURI (daeString URIString, daeBool nofrag=false) | |
Constructs a daeURI object from a URI passed in as a string. | |
daeURI (daeURI &baseURI, daeString URIString) | |
Constructs a daeURI object using a baseURI and a uriString. | |
daeURI (daeURI &constructFromURI) | |
Constructs a daeURI object based on a simple copy from an existing daeURI . | |
daeString | getID () |
Gets the ID string parsed from the URI. | |
daeString | getFile () |
Gets the file string parsed from the URI. | |
daeString | getFilepath () |
Gets the path string to the file, without the path name, parsed from the URI. | |
daeString | getProtocol () |
Gets the protocol string parsed from the URI. | |
daeString | getAuthority () |
Gets the authority string parsed from the URI. | |
daeString | getExtension () |
Gets the extension string parsed from the URI. | |
daeElementRef | getElement () |
Gets the element that this URI resolves to in memory. | |
void | setElement (daeElementRef newref) |
Sets the element that this URI resolves to in memory. | |
ResolveState | getState () |
Gets the resolve state of the URI. | |
void | setState (ResolveState newState) |
Sets the resolve state of the URI. | |
daeElement * | getContainer () |
Gets a pointer to the daeElement that contains this URI. | |
void | setContainer (daeElement *element) |
Sets the pointer to the daeElement that contains this URI. | |
void | setURI (daeString uri) |
Copies parameter uri into data member uriString, and then decomposes each of protocol, authority, filepath, file, and id. | |
daeString | getURI () const |
Gets the URI stored in the daeURI. | |
daeString | getOriginalURI () |
Gets the original URI String as originally set, not flattened against the base URI. | |
void | resolveElement () |
Uses the daeURIResolver static API to try to resolve this URI into a daeElement reference, placing the resolved element into element. | |
void | resolveURI () |
Configures the uriString for this daeURI based on the element set in element. | |
void | validate (daeURI *baseURI=NULL) |
Flattens this URI with base URI to obtain a useable complete URI for resolution. | |
void | copyFrom (daeURI &from) |
Copies the URI specified in from into this . | |
void | print () |
Outputs all components of this URI to stderr. | |
int | makeRelativeTo (daeURI *uri) |
Makes the "originalURI" in this URI relative to some other uri. | |
daeBool | getPath (daeChar *dest, daeInt size) |
Gets the path part of the URI, including everything from immediately after the authority up to and including the file name, but not the query or fragment. | |
Static Public Member Functions | |
static void | setBaseURI (daeURI &uri) |
Sets the application's default base URI. | |
static daeURI * | getBaseURI () |
Gets the application's default base URI. | |
static void | normalizeURIPath (char *path) |
Performs RFC2396 path normalization. |
Member Enumeration Documentation
|
Constructor & Destructor Documentation
|
Constructs a daeURI object that points to the application's current working directory.
|
|
Constructs a daeURI object from a URI passed in as a string.
|
|
Constructs a daeURI object using a
Calls setURI(URIString), and
|
|
Constructs a daeURI object based on a simple copy from an existing
|
Member Function Documentation
|
Copies the URI specified in Performs a simple copy without validating the URI.
|
|
Gets the authority string parsed from the URI.
|
|
Gets the application's default base URI.
|
|
Gets a pointer to the
|
|
Gets the element that this URI resolves to in memory.
|
|
Gets the extension string parsed from the URI.
|
|
Gets the file string parsed from the URI.
|
|
Gets the path string to the file, without the path name, parsed from the URI.
|
|
Gets the ID string parsed from the URI.
|
|
Gets the original URI String as originally set, not flattened against the base URI.
|
|
Gets the path part of the URI, including everything from immediately after the authority up to and including the file name, but not the query or fragment.
|
|
Gets the protocol string parsed from the URI.
|
|
Gets the resolve state of the URI.
|
|
Gets the URI stored in the daeURI.
|
|
Makes the "originalURI" in this URI relative to some other uri.
|
|
Performs RFC2396 path normalization.
|
|
Outputs all components of this URI to stderr. Useful for debugging URIs, this outputs each part of the URI separately. |
|
Uses the
This function can effectively force a load of a file, perform a database query, and so on, based on the |
|
Configures the Uses the element's base URI and ID information to configure the URI string. |
|
Sets the application's default base URI. This is effectively the default protocol, authority, and path in the case of top-level relative URIs.
|
|
Sets the pointer to the
|
|
Sets the element that this URI resolves to in memory.
|
|
Sets the resolve state of the URI.
|
|
Copies parameter
After
|
|
Flattens this URI with base URI to obtain a useable complete URI for resolution.
|
The documentation for this class was generated from the following files:
- C:/SVN_wf/COLLADA_DOM/include/dae/daeURI.h
- C:/SVN_wf/COLLADA_DOM/src/dae/daeURI.cpp
©2005 Sony Computer Entertainment Inc.. All Rights Reserved.
Generated on Fri Feb 10 16:47:04 2006 for COLLADA 1.4 DOM by
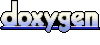