domAsset Class Reference
#include <domAsset.h>
Inheritance diagram for domAsset:

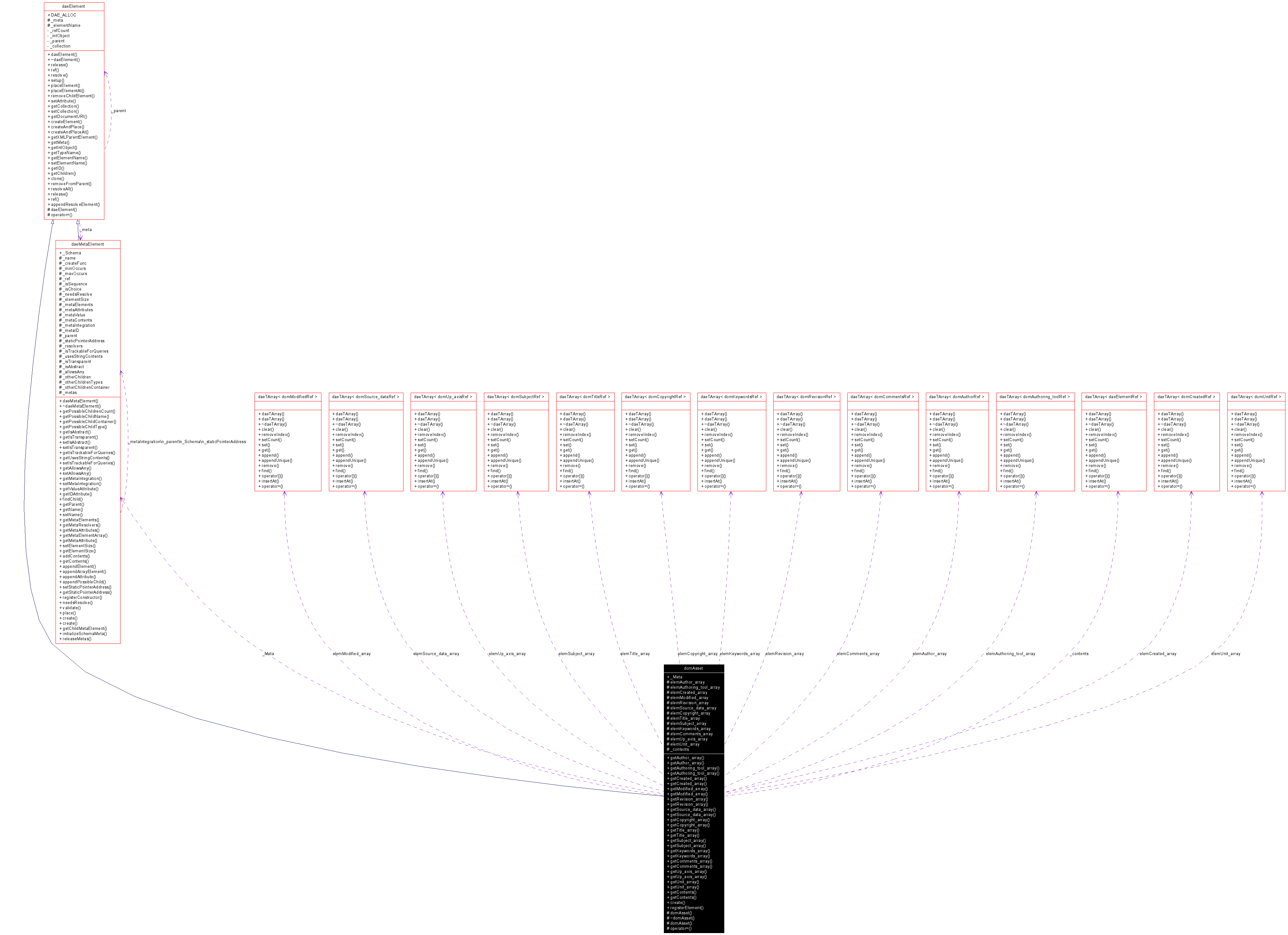
Detailed Description
The asset element defines asset management information regarding its parent element.Computers store vast amounts of information. An asset is a set of information that is organized into a distinct collection and managed as a unit. A wide range of attributes describes assets so that the information can be maintained and understood by software tools and humans.
Public Types | |
typedef daeSmartRef< domAuthor > | domAuthorRef |
typedef daeTArray< domAuthorRef > | domAuthor_Array |
typedef daeSmartRef< domAuthoring_tool > | domAuthoring_toolRef |
typedef daeTArray< domAuthoring_toolRef > | domAuthoring_tool_Array |
typedef daeSmartRef< domCreated > | domCreatedRef |
typedef daeTArray< domCreatedRef > | domCreated_Array |
typedef daeSmartRef< domModified > | domModifiedRef |
typedef daeTArray< domModifiedRef > | domModified_Array |
typedef daeSmartRef< domRevision > | domRevisionRef |
typedef daeTArray< domRevisionRef > | domRevision_Array |
typedef daeSmartRef< domSource_data > | domSource_dataRef |
typedef daeTArray< domSource_dataRef > | domSource_data_Array |
typedef daeSmartRef< domCopyright > | domCopyrightRef |
typedef daeTArray< domCopyrightRef > | domCopyright_Array |
typedef daeSmartRef< domTitle > | domTitleRef |
typedef daeTArray< domTitleRef > | domTitle_Array |
typedef daeSmartRef< domSubject > | domSubjectRef |
typedef daeTArray< domSubjectRef > | domSubject_Array |
typedef daeSmartRef< domKeywords > | domKeywordsRef |
typedef daeTArray< domKeywordsRef > | domKeywords_Array |
typedef daeSmartRef< domComments > | domCommentsRef |
typedef daeTArray< domCommentsRef > | domComments_Array |
typedef daeSmartRef< domUp_axis > | domUp_axisRef |
typedef daeTArray< domUp_axisRef > | domUp_axis_Array |
typedef daeSmartRef< domUnit > | domUnitRef |
typedef daeTArray< domUnitRef > | domUnit_Array |
Public Member Functions | |
domAuthor_Array & | getAuthor_array () |
Gets the author element array. | |
const domAuthor_Array & | getAuthor_array () const |
Gets the author element array. | |
domAuthoring_tool_Array & | getAuthoring_tool_array () |
Gets the authoring_tool element array. | |
const domAuthoring_tool_Array & | getAuthoring_tool_array () const |
Gets the authoring_tool element array. | |
domCreated_Array & | getCreated_array () |
Gets the created element array. | |
const domCreated_Array & | getCreated_array () const |
Gets the created element array. | |
domModified_Array & | getModified_array () |
Gets the modified element array. | |
const domModified_Array & | getModified_array () const |
Gets the modified element array. | |
domRevision_Array & | getRevision_array () |
Gets the revision element array. | |
const domRevision_Array & | getRevision_array () const |
Gets the revision element array. | |
domSource_data_Array & | getSource_data_array () |
Gets the source_data element array. | |
const domSource_data_Array & | getSource_data_array () const |
Gets the source_data element array. | |
domCopyright_Array & | getCopyright_array () |
Gets the copyright element array. | |
const domCopyright_Array & | getCopyright_array () const |
Gets the copyright element array. | |
domTitle_Array & | getTitle_array () |
Gets the title element array. | |
const domTitle_Array & | getTitle_array () const |
Gets the title element array. | |
domSubject_Array & | getSubject_array () |
Gets the subject element array. | |
const domSubject_Array & | getSubject_array () const |
Gets the subject element array. | |
domKeywords_Array & | getKeywords_array () |
Gets the keywords element array. | |
const domKeywords_Array & | getKeywords_array () const |
Gets the keywords element array. | |
domComments_Array & | getComments_array () |
Gets the comments element array. | |
const domComments_Array & | getComments_array () const |
Gets the comments element array. | |
domUp_axis_Array & | getUp_axis_array () |
Gets the up_axis element array. | |
const domUp_axis_Array & | getUp_axis_array () const |
Gets the up_axis element array. | |
domUnit_Array & | getUnit_array () |
Gets the unit element array. | |
const domUnit_Array & | getUnit_array () const |
Gets the unit element array. | |
daeElementRefArray & | getContents () |
Gets the _contents array. | |
const daeElementRefArray & | getContents () const |
Gets the _contents array. | |
Static Public Member Functions | |
static daeElementRef | create (daeInt bytes) |
Creates an instance of this class and returns a daeElementRef referencing it. | |
static daeMetaElement * | registerElement () |
Creates a daeMetaElement object that describes this element in the meta object reflection framework. | |
Static Public Attributes | |
static daeMetaElement * | _Meta = NULL |
The daeMetaElement that describes this element in the meta object reflection framework. | |
Protected Member Functions | |
domAsset () | |
Constructor. | |
virtual | ~domAsset () |
Destructor. | |
domAsset (const domAsset &cpy) | |
Copy Constructor. | |
virtual domAsset & | operator= (const domAsset &cpy) |
Overloaded assignment operator. | |
Protected Attributes | |
domAuthor_Array | elemAuthor_array |
The author element contains the name of an author of the parent element. | |
domAuthoring_tool_Array | elemAuthoring_tool_array |
The authoring_tool element contains the name of an application or tools used to create the parent element. | |
domCreated_Array | elemCreated_array |
The created element contains the date and time that the parent element was created and represented in an ISO 8601 format. | |
domModified_Array | elemModified_array |
The modified element contains the date and time that the parent element was last modified and represented in an ISO 8601 format. | |
domRevision_Array | elemRevision_array |
The revision element contains the revision information for the parent element. | |
domSource_data_Array | elemSource_data_array |
The source_data element contains the URI to the source data from which the parent element was created. | |
domCopyright_Array | elemCopyright_array |
The copyright element contains the author's copyright information applicable to the parent element. | |
domTitle_Array | elemTitle_array |
The title element contains the title information for the parent element. | |
domSubject_Array | elemSubject_array |
The subject element contains a description of the topical subject of the parent element. | |
domKeywords_Array | elemKeywords_array |
The keywords element contains a list of words used as search criteria for the parent element. | |
domComments_Array | elemComments_array |
The comments element contains descriptive information about the parent element. | |
domUp_axis_Array | elemUp_axis_array |
The up_axis element contains descriptive information about coordinate system of the geometric data. | |
domUnit_Array | elemUnit_array |
The unit element contains descriptive information about unit of measure. | |
daeElementRefArray | _contents |
Used to preserve order in elements that do not specify strict sequencing of sub-elements. | |
Classes | |
class | domAuthor |
The author element contains the name of an author of the parent element. More... | |
class | domAuthoring_tool |
The authoring_tool element contains the name of an application or tools used to create the parent element. More... | |
class | domComments |
The comments element contains descriptive information about the parent element. More... | |
class | domCopyright |
The copyright element contains the author's copyright information applicable to the parent element. More... | |
class | domCreated |
The created element contains the date and time that the parent element was created and represented in an ISO 8601 format. More... | |
class | domKeywords |
The keywords element contains a list of words used as search criteria for the parent element. More... | |
class | domModified |
The modified element contains the date and time that the parent element was last modified and represented in an ISO 8601 format. More... | |
class | domRevision |
The revision element contains the revision information for the parent element. More... | |
class | domSource_data |
The source_data element contains the URI to the source data from which the parent element was created. More... | |
class | domSubject |
The subject element contains a description of the topical subject of the parent element. More... | |
class | domTitle |
The title element contains the title information for the parent element. More... | |
class | domUnit |
The unit element contains descriptive information about unit of measure. More... | |
class | domUp_axis |
The up_axis element contains descriptive information about coordinate system of the geometric data. More... |
Member Function Documentation
|
Creates an instance of this class and returns a daeElementRef referencing it.
|
|
Gets the author element array.
|
|
Gets the author element array.
|
|
Gets the authoring_tool element array.
|
|
Gets the authoring_tool element array.
|
|
Gets the comments element array.
|
|
Gets the comments element array.
|
|
Gets the _contents array.
|
|
Gets the _contents array.
|
|
Gets the copyright element array.
|
|
Gets the copyright element array.
|
|
Gets the created element array.
|
|
Gets the created element array.
|
|
Gets the keywords element array.
|
|
Gets the keywords element array.
|
|
Gets the modified element array.
|
|
Gets the modified element array.
|
|
Gets the revision element array.
|
|
Gets the revision element array.
|
|
Gets the source_data element array.
|
|
Gets the source_data element array.
|
|
Gets the subject element array.
|
|
Gets the subject element array.
|
|
Gets the title element array.
|
|
Gets the title element array.
|
|
Gets the unit element array.
|
|
Gets the unit element array.
|
|
Gets the up_axis element array.
|
|
Gets the up_axis element array.
|
|
Creates a daeMetaElement object that describes this element in the meta object reflection framework. If a daeMetaElement already exists it will return that instead of creating a new one.
|
Member Data Documentation
|
The author element contains the name of an author of the parent element. The author element may appear zero or more times.
|
|
The authoring_tool element contains the name of an application or tools used to create the parent element. The authoring_tool element may appear zero or one time.
|
|
The comments element contains descriptive information about the parent element. The comments element may appear zero or more times.
|
|
The copyright element contains the author's copyright information applicable to the parent element. The copyright element may appear zero or more times.
|
|
The created element contains the date and time that the parent element was created and represented in an ISO 8601 format. The created element may appear zero or one time.
|
|
The keywords element contains a list of words used as search criteria for the parent element. The keywords element may appear zero or more times.
|
|
The modified element contains the date and time that the parent element was last modified and represented in an ISO 8601 format. The modified element may appear zero or one time.
|
|
The revision element contains the revision information for the parent element. The revision element may appear zero or one time.
|
|
The source_data element contains the URI to the source data from which the parent element was created. The source_data element may appear zero or one time.
|
|
The subject element contains a description of the topical subject of the parent element. The subject element may appear zero or one time.
|
|
The title element contains the title information for the parent element. The title element may appear zero or one time.
|
|
The unit element contains descriptive information about unit of measure. It has attributes for the name of the unit and the measurement with respect to the meter. The unit element may appear zero or one time. The default value for the name attribute is 'meter'. The default value for the meter attribute is '1.0'.
|
|
The up_axis element contains descriptive information about coordinate system of the geometric data. All coordinates are right-handed by definition. This element specifies which axis is considered up. The up_axis element contains one of: X_UP, Y_UP, or Z_UP. The default is the Y-axis. The up_axis element may appear zero or one time.
|
The documentation for this class was generated from the following files:
- C:/SVN_wf/COLLADA_DOM/include/1.3/dom/domAsset.h
- C:/SVN_wf/COLLADA_DOM/src/1.3/dom/domAsset.cpp
©2005 Sony Computer Entertainment Inc.. All Rights Reserved.
Generated on Fri Feb 10 16:47:19 2006 for COLLADA 1.4 DOM by
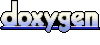