daeElement Class Reference
#include <daeElement.h>
Inheritance diagram for daeElement:

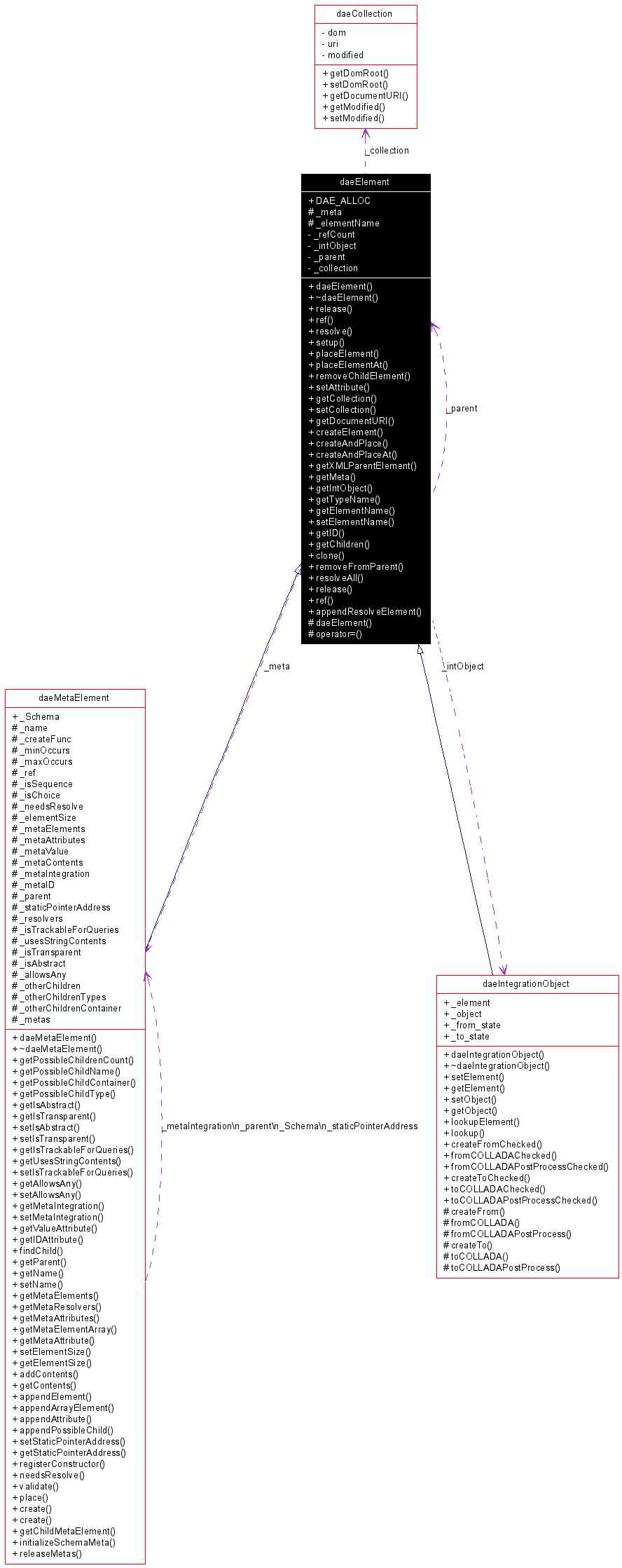
Detailed Description
ThedaeElement
class represents an instance of a COLLADA "Element"; it is the main base class for the COLLADA Dom.
Features of this class include:
- Uses factory concepts defined via daeMetaElement
- Composed of attributes, content elements and content values
- Reference counted via daeSmartRef
- Contains information for XML base URI, and XML containing element
Public Types | |
enum | IntegrationState { int_uninitialized, int_created, int_converted, int_finished } |
An enum that describes the state of user integration with this object. More... | |
Public Member Functions | |
daeElement () | |
Element Constructor. | |
virtual | ~daeElement () |
Element Destructor. | |
void | release () const |
Decrements the reference count and deletes the object if reference count is zero. | |
void | ref () const |
Increments the reference count of this element. | |
void | resolve () |
Resolves all fields of type daeURI. | |
void | setup (daeMetaElement *meta) |
Sets up a daeElement . | |
daeBool | placeElement (daeElement *element) |
Places an element as a child of this element. | |
daeBool | placeElementAt (daeInt index, daeElement *element) |
This function searches through the list of potential child elements (fields) checking for a matching element type where this element can be added. | |
daeBool | removeChildElement (daeElement *element) |
Removes the specified element from it parent, the this element. | |
virtual daeBool | setAttribute (daeString attrName, daeString attrValue) |
Looks up an attribute field via its meta name and assign its value as the attrValue String. | |
daeCollection * | getCollection () |
Finds the database collection associated with this element. | |
void | setCollection (daeCollection *c) |
Sets the database collection associated with this element. | |
daeURI * | getDocumentURI () |
Gets the URI of the document containing this element, note that this is NOT the URI of the element. | |
daeSmartRef< daeElement > | createElement (daeString className) |
Creates an element via the element factory system. | |
daeElement * | createAndPlace (daeString className) |
Creates a subelement via createElement() and places it via placeElement() . | |
daeElement * | createAndPlaceAt (daeInt index, daeString className) |
Create a sub-element via createElement and place it via placeElementAt This also automatically inserts the new element at the specified index in the _contents of it's parent, if the parent has one. | |
daeElement * | getXMLParentElement () |
Gets the container element for this element. | |
daeMetaElement * | getMeta () |
Gets the associated Meta information for this element. | |
daeIntegrationObject * | getIntObject (IntegrationState from_state=int_converted, IntegrationState to_state=int_uninitialized) |
Gets the integration object associated with this daeElement object. | |
daeString | getTypeName () const |
Gets the element type name for this element. | |
daeString | getElementName () const |
Gets this element's name. | |
void | setElementName (daeString nm) |
Sets this element's name. | |
daeString | getID () const |
Gets the element ID if it exists. | |
void | getChildren (daeTArray< daeSmartRef< daeElement > > &array) |
Gets the children/sub-elements of this element. | |
daeSmartRef< daeElement > | clone (daeString idSuffix=NULL, daeString nameSuffix=NULL) |
Clones/deep copies this daeElement and all of it's subtree. | |
Static Public Member Functions | |
static daeBool | removeFromParent (daeElement *element) |
Removes the specified element from its parent element. | |
static void | resolveAll () |
Resolves all daeURIs yet to be resolved in all daeElements that have been created. | |
static void | release (const daeElement *elem) |
Releases the element passed in. | |
static void | ref (const daeElement *elem) |
Increments the reference counter for the element passed in. | |
static void | appendResolveElement (daeElement *elem) |
Appends the passed in element to the list of elements that need to be resolved. | |
Public Attributes | |
DAE_ALLOC | |
Macro that defines new and delete overrides for this class. | |
Protected Member Functions | |
daeElement (const daeElement &cpy) | |
virtual daeElement & | operator= (const daeElement &cpy) |
Protected Attributes | |
daeMetaElement * | _meta |
daeString | _elementName |
Member Enumeration Documentation
|
An enum that describes the state of user integration with this object.
|
Constructor & Destructor Documentation
|
Element Constructor.
|
|
Element Destructor.
|
Member Function Documentation
|
Appends the passed in element to the list of elements that need to be resolved.
The elements in this list will be resolved during
|
|
Clones/deep copies this
|
|
Creates a subelement via
Automatically adds the new element to the
|
|
Create a sub-element via createElement and place it via placeElementAt This also automatically inserts the new element at the specified index in the _contents of it's parent, if the parent has one. This is useful when constructing the COLLADA dom hierarchy
|
|
Creates an element via the element factory system. This creation is based only on potential child elements of this element.
|
|
Gets the children/sub-elements of this element. This is a helper function used to easily access an element's children without the use of the _meta objects. This function adds the convenience of the _contents array to elements that do not contain a _contents array.
|
|
Finds the database collection associated with
|
|
Gets the URI of the document containing this element, note that this is NOT the URI of the element.
|
|
Gets this element's name.
|
|
Gets the element ID if it exists.
|
|
Gets the integration object associated with this
See
|
|
Gets the associated Meta information for this element.
This Meta also acts as a factory. See
|
|
Gets the element type name for this element.
|
|
Gets the container element for
If
|
|
Places an element as a child of
This function searches through the list of potential child element fields in
|
|
This function searches through the list of potential child elements (fields) checking for a matching element type where this element can be added. If a match of type is found, the element* is assigned or appended to that field (based on whether it is a single child or an array of children. If the parent element contains a _contents array, element will be placed at the specified index, otherwise element gets placed among elements of the same type.
|
|
Increments the reference counter for the element passed in.
This function is a static wrapper that invokes
|
|
Increments the reference count of this element.
|
|
Releases the element passed in.
This function is a static wrapper that invokes
|
|
Decrements the reference count and deletes the object if reference count is zero.
|
|
Removes the specified element from it parent, the
This function is the opposite of
|
|
Removes the specified element from its parent element.
This function is the opposite of
Use this function instead of
|
|
Resolves all fields of type daeURI. This is done via database query of the URI. |
|
Resolves all This is used as part of post-parsing process of a COLLADA instance document, which results in a new collection in the database. |
|
Looks up an attribute field via its meta name and assign its value as the
Reimplemented in domAny. |
|
Sets the database collection associated with this element.
|
|
Sets this element's name.
|
|
Sets up a
Called on all
|
The documentation for this class was generated from the following files:
- C:/SVN_wf/COLLADA_DOM/include/dae/daeElement.h
- C:/SVN_wf/COLLADA_DOM/src/dae/daeElement.cpp
©2005 Sony Computer Entertainment Inc.. All Rights Reserved.
Generated on Fri Feb 10 16:46:59 2006 for COLLADA 1.4 DOM by
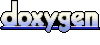