domMesh Class Reference
#include <domMesh.h>
Inheritance diagram for domMesh:

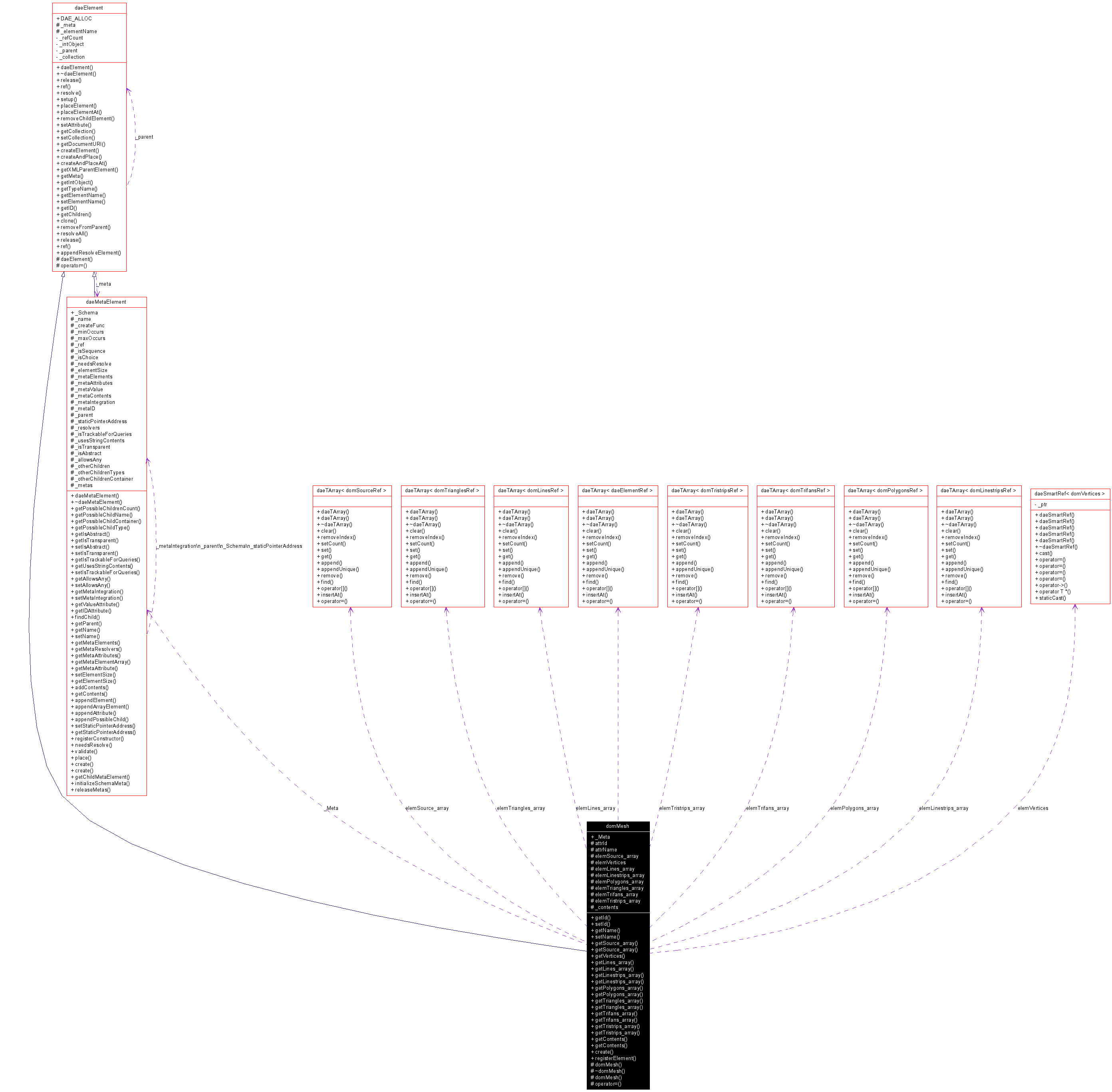
Detailed Description
The mesh element contains vertex and primitive information sufficient to describe basic geometric meshes.Meshes embody a general form of geometric description that primarily includes vertex and primitive information. Vertex information is the set of attributes associated with a point on the surface of the mesh. Each vertex includes data for attributes such as: * Vertex position * Vertex color * Vertex normal * Vertex texture coordinate The mesh also includes a description of how the vertices are organized to form the geometric shape of the mesh. The mesh vertices are collated into geometric primitives such as polygons, triangles, or lines.
Public Member Functions | |
xsID | getId () const |
Gets the id attribute. | |
void | setId (xsID atId) |
Sets the id attribute. | |
xsNCName | getName () const |
Gets the name attribute. | |
void | setName (xsNCName atName) |
Sets the name attribute. | |
domSource_Array & | getSource_array () |
Gets the source element array. | |
const domSource_Array & | getSource_array () const |
Gets the source element array. | |
const domVerticesRef | getVertices () const |
Gets the vertices element. | |
domLines_Array & | getLines_array () |
Gets the lines element array. | |
const domLines_Array & | getLines_array () const |
Gets the lines element array. | |
domLinestrips_Array & | getLinestrips_array () |
Gets the linestrips element array. | |
const domLinestrips_Array & | getLinestrips_array () const |
Gets the linestrips element array. | |
domPolygons_Array & | getPolygons_array () |
Gets the polygons element array. | |
const domPolygons_Array & | getPolygons_array () const |
Gets the polygons element array. | |
domTriangles_Array & | getTriangles_array () |
Gets the triangles element array. | |
const domTriangles_Array & | getTriangles_array () const |
Gets the triangles element array. | |
domTrifans_Array & | getTrifans_array () |
Gets the trifans element array. | |
const domTrifans_Array & | getTrifans_array () const |
Gets the trifans element array. | |
domTristrips_Array & | getTristrips_array () |
Gets the tristrips element array. | |
const domTristrips_Array & | getTristrips_array () const |
Gets the tristrips element array. | |
daeElementRefArray & | getContents () |
Gets the _contents array. | |
const daeElementRefArray & | getContents () const |
Gets the _contents array. | |
Static Public Member Functions | |
static daeElementRef | create (daeInt bytes) |
Creates an instance of this class and returns a daeElementRef referencing it. | |
static daeMetaElement * | registerElement () |
Creates a daeMetaElement object that describes this element in the meta object reflection framework. | |
Static Public Attributes | |
static daeMetaElement * | _Meta = NULL |
The daeMetaElement that describes this element in the meta object reflection framework. | |
Protected Member Functions | |
domMesh () | |
Constructor. | |
virtual | ~domMesh () |
Destructor. | |
domMesh (const domMesh &cpy) | |
Copy Constructor. | |
virtual domMesh & | operator= (const domMesh &cpy) |
Overloaded assignment operator. | |
Protected Attributes | |
xsID | attrId |
The id attribute is a text string containing the unique identifier of the mesh element. | |
xsNCName | attrName |
The name attribute is a text string containing the name of the mesh element. | |
domSource_Array | elemSource_array |
The source element must occur one or more times as the first elements of mesh. | |
domVerticesRef | elemVertices |
The vertices element must occur exactly one time. | |
domLines_Array | elemLines_array |
The lines element contains line primitives. | |
domLinestrips_Array | elemLinestrips_array |
The linestrips element contains line-strip primitives. | |
domPolygons_Array | elemPolygons_array |
The polygons element contains polygon primitives. | |
domTriangles_Array | elemTriangles_array |
The triangles element contains triangle primitives. | |
domTrifans_Array | elemTrifans_array |
The trifans element contains triangle-fan primitives. | |
domTristrips_Array | elemTristrips_array |
The tristrips element contains triangle-strip primitives. | |
daeElementRefArray | _contents |
Used to preserve order in elements that do not specify strict sequencing of sub-elements. |
Member Function Documentation
|
Creates an instance of this class and returns a daeElementRef referencing it.
|
|
Gets the _contents array.
|
|
Gets the _contents array.
|
|
Gets the id attribute.
|
|
Gets the lines element array.
|
|
Gets the lines element array.
|
|
Gets the linestrips element array.
|
|
Gets the linestrips element array.
|
|
Gets the name attribute.
|
|
Gets the polygons element array.
|
|
Gets the polygons element array.
|
|
Gets the source element array.
|
|
Gets the source element array.
|
|
Gets the triangles element array.
|
|
Gets the triangles element array.
|
|
Gets the trifans element array.
|
|
Gets the trifans element array.
|
|
Gets the tristrips element array.
|
|
Gets the tristrips element array.
|
|
Gets the vertices element.
|
|
Creates a daeMetaElement object that describes this element in the meta object reflection framework. If a daeMetaElement already exists it will return that instead of creating a new one.
|
|
Sets the id attribute.
|
|
Sets the name attribute.
|
Member Data Documentation
|
The id attribute is a text string containing the unique identifier of the mesh element. This value must be unique within the instance document. Optional attribute. |
|
The name attribute is a text string containing the name of the mesh element. Optional attribute. |
|
The lines element contains line primitives.
|
|
The linestrips element contains line-strip primitives.
|
|
The polygons element contains polygon primitives.
|
|
The source element must occur one or more times as the first elements of mesh. The source element may also occur 0 or more times after the vertices element but before the primitive elements. The source elements provide the bulk of the mesh's vertex data.
|
|
The triangles element contains triangle primitives.
|
|
The trifans element contains triangle-fan primitives.
|
|
The tristrips element contains triangle-strip primitives.
|
|
The vertices element must occur exactly one time. The vertices element describes the meshvertex attributes and establishes their topological identity.
|
The documentation for this class was generated from the following files:
- C:/SVN_wf/COLLADA_DOM/include/1.3/dom/domMesh.h
- C:/SVN_wf/COLLADA_DOM/src/1.3/dom/domMesh.cpp
©2005 Sony Computer Entertainment Inc.. All Rights Reserved.
Generated on Fri Feb 10 16:49:07 2006 for COLLADA 1.4 DOM by
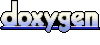