domArray Class Reference
#include <domArray.h>
Inheritance diagram for domArray:

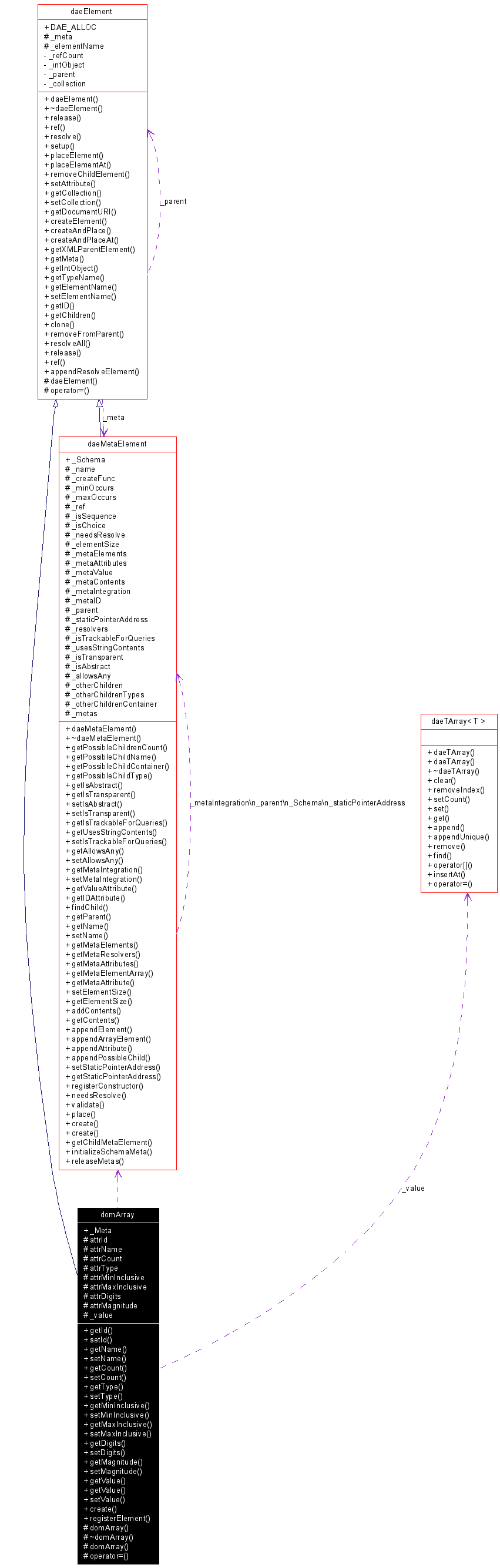
Detailed Description
The array element declares the storage for a homogenous array of generic data values.The array element is deprecated in this version of COLLADA and may be removed in the future.
Public Member Functions | |
xsID | getId () const |
Gets the id attribute. | |
void | setId (xsID atId) |
Sets the id attribute. | |
xsNCName | getName () const |
Gets the name attribute. | |
void | setName (xsNCName atName) |
Sets the name attribute. | |
xsNonNegativeInteger | getCount () const |
Gets the count attribute. | |
void | setCount (xsNonNegativeInteger atCount) |
Sets the count attribute. | |
domArrayTypes | getType () const |
Gets the type attribute. | |
void | setType (domArrayTypes atType) |
Sets the type attribute. | |
xsInteger | getMinInclusive () const |
Gets the minInclusive attribute. | |
void | setMinInclusive (xsInteger atMinInclusive) |
Sets the minInclusive attribute. | |
xsInteger | getMaxInclusive () const |
Gets the maxInclusive attribute. | |
void | setMaxInclusive (xsInteger atMaxInclusive) |
Sets the maxInclusive attribute. | |
xsShort | getDigits () const |
Gets the digits attribute. | |
void | setDigits (xsShort atDigits) |
Sets the digits attribute. | |
xsShort | getMagnitude () const |
Gets the magnitude attribute. | |
void | setMagnitude (xsShort atMagnitude) |
Sets the magnitude attribute. | |
domListOfTokens & | getValue () |
Gets the _value array. | |
const domListOfTokens & | getValue () const |
Gets the _value array. | |
void | setValue (const domListOfTokens &val) |
Sets the _value array. | |
Static Public Member Functions | |
static daeElementRef | create (daeInt bytes) |
Creates an instance of this class and returns a daeElementRef referencing it. | |
static daeMetaElement * | registerElement () |
Creates a daeMetaElement object that describes this element in the meta object reflection framework. | |
Static Public Attributes | |
static daeMetaElement * | _Meta = NULL |
The daeMetaElement that describes this element in the meta object reflection framework. | |
Protected Member Functions | |
domArray () | |
Constructor. | |
virtual | ~domArray () |
Destructor. | |
domArray (const domArray &cpy) | |
Copy Constructor. | |
virtual domArray & | operator= (const domArray &cpy) |
Overloaded assignment operator. | |
Protected Attributes | |
xsID | attrId |
The id attribute is a text string containing the unique identifier of the array element. | |
xsNCName | attrName |
The name attribute is the text string name of this element. | |
xsNonNegativeInteger | attrCount |
The count attribute indicates the number of values in the array. | |
domArrayTypes | attrType |
The type attribute indicates the data type, such as floating-point or integer numbers of the values contained in the array. | |
xsInteger | attrMinInclusive |
The minInclusive attribute indicates the smallest integer value that can be contained in the array. | |
xsInteger | attrMaxInclusive |
The maxInclusive attribute indicates the largest integer value that can be contained in the array. | |
xsShort | attrDigits |
The digits attribute indicates the number of significant decimal digits of the float values that can be contained in the array. | |
xsShort | attrMagnitude |
The magnitude attribute indicates the largest exponent of the float values that can be contained in the array. | |
domListOfTokens | _value |
The domListOfTokens value of the text data of this element. |
Member Function Documentation
|
Creates an instance of this class and returns a daeElementRef referencing it.
|
|
Gets the count attribute.
|
|
Gets the digits attribute.
|
|
Gets the id attribute.
|
|
Gets the magnitude attribute.
|
|
Gets the maxInclusive attribute.
|
|
Gets the minInclusive attribute.
|
|
Gets the name attribute.
|
|
Gets the type attribute.
|
|
Gets the _value array.
|
|
Gets the _value array.
|
|
Creates a daeMetaElement object that describes this element in the meta object reflection framework. If a daeMetaElement already exists it will return that instead of creating a new one.
|
|
Sets the count attribute.
|
|
Sets the digits attribute.
|
|
Sets the id attribute.
|
|
Sets the magnitude attribute.
|
|
Sets the maxInclusive attribute.
|
|
Sets the minInclusive attribute.
|
|
Sets the name attribute.
|
|
Sets the type attribute.
|
|
Sets the _value array.
|
Member Data Documentation
|
The count attribute indicates the number of values in the array. Required attribute. |
|
The digits attribute indicates the number of significant decimal digits of the float values that can be contained in the array. The default value is 6. Optional attribute. |
|
The id attribute is a text string containing the unique identifier of the array element. This value must be unique within the instance document. Optional attribute. |
|
The magnitude attribute indicates the largest exponent of the float values that can be contained in the array. The default value is 38. Optional attribute. |
|
The maxInclusive attribute indicates the largest integer value that can be contained in the array. The default value is 2147483647. Optional attribute. |
|
The minInclusive attribute indicates the smallest integer value that can be contained in the array. The default value is -2147483648. Optional attribute. |
|
The name attribute is the text string name of this element. Optional attribute. |
|
The type attribute indicates the data type, such as floating-point or integer numbers of the values contained in the array. The array may also contain a list of names or tokens conforming to the XML Schema Language derived types xs:Name and xs:token, respectively. Required attribute. |
The documentation for this class was generated from the following files:
- C:/SVN_wf/COLLADA_DOM/include/1.3/dom/domArray.h
- C:/SVN_wf/COLLADA_DOM/src/1.3/dom/domArray.cpp
©2005 Sony Computer Entertainment Inc.. All Rights Reserved.
Generated on Fri Feb 10 16:47:14 2006 for COLLADA 1.4 DOM by
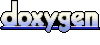