daeAtomicType Class Reference
#include <daeAtomicType.h>
Inheritance diagram for daeAtomicType:
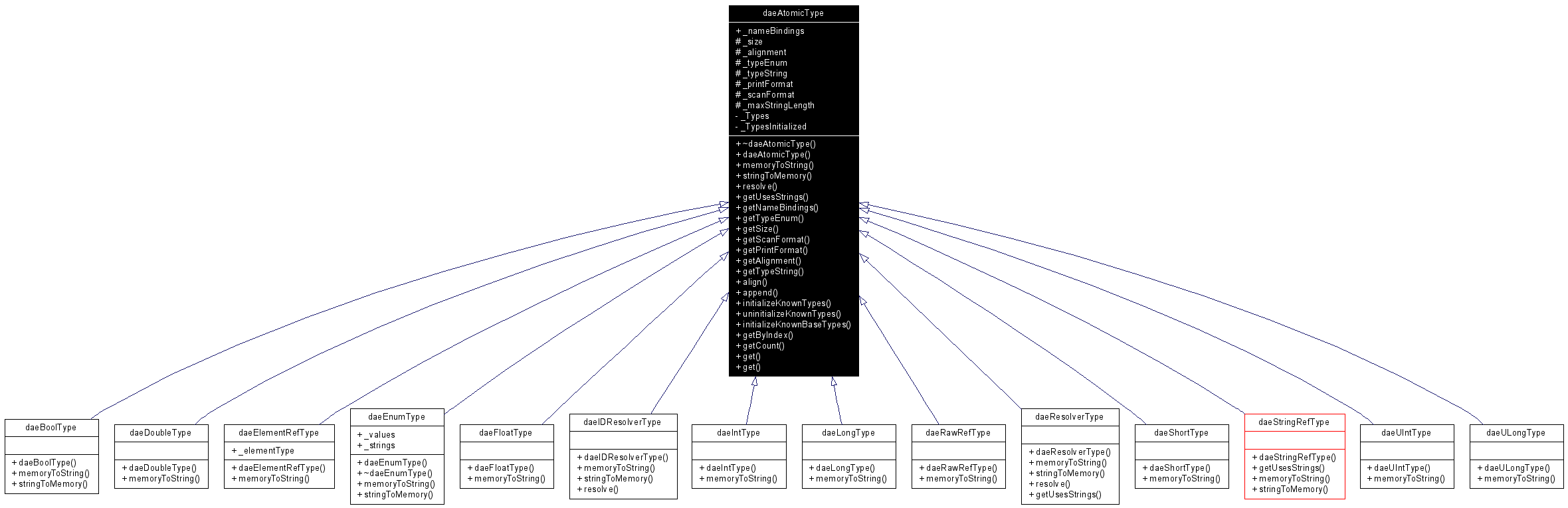
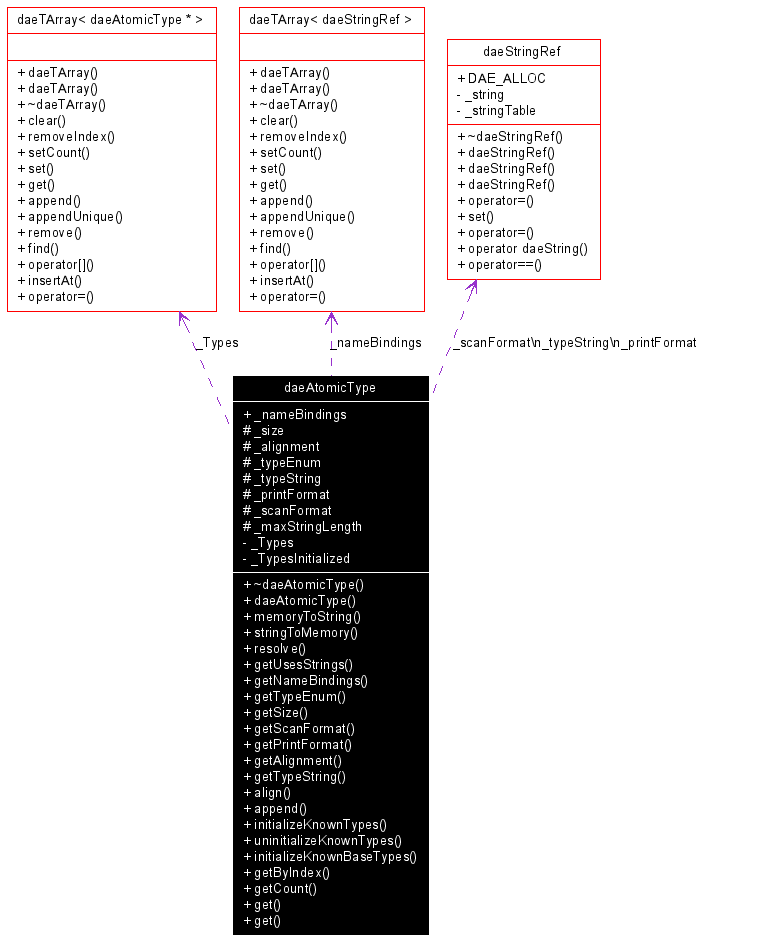
Detailed Description
ThedaeAtomicType
class implements a standard interface for data elements in the reflective object system.
daeAtomicType
provides a central virtual interface that can be used by the rest of the reflective object system.
The atomic type system if very useful for file IO and building automatic tools for data inspection and manipulation, such as hierarchy examiners and object editors.
Types provide the following symantic operations:
Types are also able to align data pointers appropriately.
Public Types | |
enum | daeAtomicTypes { BoolType, EnumType, CharType, ShortType, IntType, UIntType, LongType, ULongType, FloatType, DoubleType, StringRefType, ElementRefType, MemoryRefType, RawRefType, ResolverType, IDResolverType, TokenType, ExtensionType } |
An enum for identifying the different atomic types. More... | |
Public Member Functions | |
virtual | ~daeAtomicType () |
destructor | |
daeAtomicType () | |
constructor | |
virtual daeBool | memoryToString (daeChar *src, daeChar *dst, daeInt dstSize) |
Prints an atomic typed element into a destination string. | |
virtual daeBool | stringToMemory (daeChar *src, daeChar *dst) |
Reads an atomic typed item into the destination runtime memory. | |
virtual void | resolve (daeElementRef element, daeMetaAttributeRef ma) |
Resolves a reference, if indeed this type is a reference type. | |
virtual daeBool | getUsesStrings () |
Determines if this atomic type requires a special string-based parse state. | |
daeStringRefArray & | getNameBindings () |
Gets the array of strings as name bindings for this type. | |
daeEnum | getTypeEnum () |
Gets the enum associated with this atomic type. | |
daeInt | getSize () |
Gets the size in bytes for this atomic type. | |
daeStringRef | getScanFormat () |
Gets the scanf format used for this type. | |
daeStringRef | getPrintFormat () |
Gets the printf format used for this type. | |
daeInt | getAlignment () |
Gets the alignment in bytes necessary for this type on this platform. | |
daeStringRef | getTypeString () |
Gets the string associated with this type. | |
daeChar * | align (daeChar *ptr) |
Performs an alignment based on the alignment for this type. | |
Static Public Member Functions | |
static daeInt | append (daeAtomicType *t) |
Appends a new type to the global list of types. | |
static void | initializeKnownTypes () |
Performs a static initialization of all known atomic types. | |
static void | uninitializeKnownTypes () |
Performs an uninitialization for all known types, freeing associated memory. | |
static void | initializeKnownBaseTypes () |
Performs a static initialization of all known base atomic types. | |
static const daeAtomicType * | getByIndex (daeInt index) |
Gets a type from the list of types, based on its index. | |
static daeInt | getCount () |
Gets the number of known atomic types. | |
static daeAtomicType * | get (daeStringRef type) |
Finds a type by its string name. | |
static daeAtomicType * | get (daeEnum type) |
Finds a type by its enum. | |
Public Attributes | |
daeStringRefArray | _nameBindings |
An array of strings as name bindings for this type. | |
Protected Attributes | |
daeInt | _size |
daeInt | _alignment |
daeEnum | _typeEnum |
daeStringRef | _typeString |
daeStringRef | _printFormat |
daeStringRef | _scanFormat |
daeInt | _maxStringLength |
Member Enumeration Documentation
|
Member Function Documentation
|
Performs an alignment based on the alignment for this type.
|
|
Appends a new type to the global list of types.
|
|
Finds a type by its enum.
|
|
Finds a type by its string name.
|
|
Gets the alignment in bytes necessary for this type on this platform.
|
|
Gets a type from the list of types, based on its index.
|
|
Gets the number of known atomic types.
|
|
Gets the array of strings as name bindings for this type.
|
|
Gets the printf format used for this type.
|
|
Gets the scanf format used for this type.
|
|
Gets the size in bytes for this atomic type.
|
|
Gets the enum associated with this atomic type. This is not scalable and only works for base types, otherwise 'extension' is used.
|
|
Gets the string associated with this type.
|
|
Determines if this atomic type requires a special string-based parse state.
Reimplemented in daeStringRefType, daeTokenType, and daeResolverType. |
|
Prints an atomic typed element into a destination string.
Reimplemented in daeBoolType, daeIntType, daeLongType, daeUIntType, daeULongType, daeShortType, daeFloatType, daeDoubleType, daeStringRefType, daeElementRefType, daeEnumType, daeRawRefType, daeResolverType, and daeIDResolverType. |
|
Resolves a reference, if indeed this type is a reference type.
Reimplemented in daeResolverType, and daeIDResolverType. |
|
Reads an atomic typed item into the destination runtime memory.
Reimplemented in daeBoolType, daeStringRefType, daeEnumType, daeResolverType, and daeIDResolverType. |
The documentation for this class was generated from the following files:
- C:/SVN_wf/COLLADA_DOM/include/dae/daeAtomicType.h
- C:/SVN_wf/COLLADA_DOM/src/dae/daeAtomicType.cpp
©2005 Sony Computer Entertainment Inc.. All Rights Reserved.
Generated on Fri Feb 10 16:46:45 2006 for COLLADA 1.4 DOM by
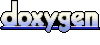