Example Program to Debug
We're going to explain the debug process by working through an example. Although we are limiting our discussion to the gdb debugger, the same example could be used for other debuggers.To create our example debug project, go to the File menu and select New and then Project. The new project dialog will be displayed.
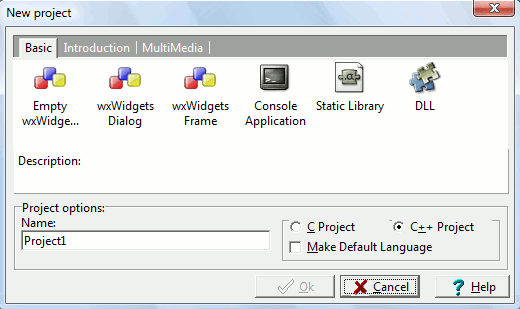
Select the Console Application from the window. We'll name our project "sampleDebug". You'll notice that a new project will be created with a skeleton C++ code called main.cpp. Replace the C++ code in main.cpp with the following code:
main.cpp
|
#include <cstdlib> |
Remember to save the project after you've replaced the contents of main.cpp.
This sample project will demonstrate the concepts of functions, local and global variables, breakpoints, and backtraces. Note that lines 24-27 are currently commented.
/* |
These lines will not cause an error during compile-time (i.e. the Mingw gcc compiler will create an executable with no reported compile errors), but it will cause a core dump at runtime due to the use of the uninitialized variable
iTestb
.In the next few sections, we'll put our sampleDebug project to good use explaining how to use the debugger...