TrueSync
|
TrueSyncConfig.cs
bool GetIgnoreLayerCollision(int layerA, int layerB)
Returns true if the collision between layerA and layerB should be ignored.
Definition: TrueSyncConfig.cs:85
bool[] physics3DIgnoreMatrix
Holds which layers should be ingnored in 3D collisions.
Definition: TrueSyncConfig.cs:57
bool speculativeContacts2D
If true enables a deeper collision detection system.
Definition: TrueSyncConfig.cs:47
bool physics3DEnabled
Indicates if the 3D physics engine should be enabled.
Definition: TrueSyncConfig.cs:52
Represents a set of configurations for TrueSync.
Definition: TrueSyncConfig.cs:8
int panicWindow
Maximum number of ticks to wait until all other players inputs arrive.
Definition: TrueSyncConfig.cs:27
bool speculativeContacts3D
If true enables a deeper collision detection system.
Definition: TrueSyncConfig.cs:67
bool showStats
When true shows a debug interface with a few information.
Definition: TrueSyncConfig.cs:72
Definition: LayerCollisionMatrix.cs:3
bool[] physics2DIgnoreMatrix
Holds which layers should be ingnored in 2D collisions.
Definition: TrueSyncConfig.cs:37
bool physics2DEnabled
Indicates if the 2D physics engine should be enabled.
Definition: TrueSyncConfig.cs:32
Generated by
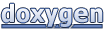