TrueSync
|
TSRigidBody.cs
249 return TSVector.Cross(tsCollider.Body.TSAngularVelocity, directionPoint) + tsCollider.Body.TSLinearVelocity;
void MoveRotation(TSQuaternion rot)
Rotates the body to a provided rotation.
Definition: TSRigidBody.cs:281
TSVector GetPointVelocity(TSVector worldPoint)
Returns the velocity of the body at some position in world space.
Definition: TSRigidBody.cs:247
void MovePosition(TSVector position)
Moves the body to a new position.
Definition: TSRigidBody.cs:274
A deterministic version of Unity's Transform component for 3D physics.
Definition: TSTransform.cs:9
bool isKinematic
If true it doesn't get influences from external forces.
Definition: TSRigidBody.cs:71
TSRigidBodyConstraints constraints
Freeze constraints applied to this body.
Definition: TSRigidBody.cs:149
void LookAt(TSVector target)
Changes orientation to look at target position.
Definition: TSRigidBody.cs:267
void AddForce(TSVector force, ForceMode mode)
Applies the provided force in the body.
Definition: TSRigidBody.cs:212
bool IsBodyInitialized
Returns true if the body was already initialized.
Definition: TSCollider.cs:240
void AddForceAtPosition(TSVector force, TSVector position)
Applies the provided force in the body.
Definition: TSRigidBody.cs:226
Definition: LayerCollisionMatrix.cs:3
void AddForceAtPosition(TSVector force, TSVector position, ForceMode mode)
Applies the provided force in the body.
Definition: TSRigidBody.cs:236
void AddTorque(TSVector torque)
Simulates the provided tourque in the body.
Definition: TSRigidBody.cs:257
Generated by
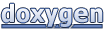