TrueSync
|
TSRigidBody2D.cs
221 return TSVector.Cross(new TSVector(0, 0, tsCollider.Body.TSAngularVelocity), directionPoint).ToTSVector2() + tsCollider.Body.TSLinearVelocity;
void MovePosition(TSVector2 position)
Moves the body to a new position.
Definition: TSRigidBody2D.cs:236
void AddForceAtPosition(TSVector2 force, TSVector2 position)
Applies the provided force in the body.
Definition: TSRigidBody2D.cs:198
InterpolateMode interpolation
Interpolation mode that should be used.
Definition: TSRigidBody2D.cs:138
void AddTorque(TSVector2 torque)
Simulates the provided tourque in the body.
Definition: TSRigidBody2D.cs:229
void AddForce(TSVector2 force)
Applies the provided force in the body.
Definition: TSRigidBody2D.cs:174
A deterministic version of Unity's Transform component for 2D physics.
Definition: TSTransform2D.cs:9
bool IsBodyInitialized
Returns true if the body was already initialized.
Definition: TSCollider2D.cs:252
TSVector2 GetPointVelocity(TSVector2 worldPoint)
Returns the velocity of the body at some position in world space.
Definition: TSRigidBody2D.cs:219
void AddForceAtPosition(TSVector2 force, TSVector2 position, ForceMode mode)
Applies the provided force in the body.
Definition: TSRigidBody2D.cs:208
void AddForce(TSVector2 force, ForceMode mode)
Applies the provided force in the body.
Definition: TSRigidBody2D.cs:184
bool freezeZAxis
If true it freezes Z rotation of the RigidBody (it only appears when in 2D Physics).
Definition: TSRigidBody2D.cs:143
bool isKinematic
If true it doesn't get influences from external forces.
Definition: TSRigidBody2D.cs:69
Definition: LayerCollisionMatrix.cs:3
Generated by
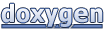