![]() |
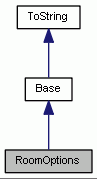
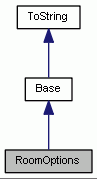
Public Member Functions | |
RoomOptions (bool isVisible=true, bool isOpen=true, nByte maxPlayers=0, const Common::Hashtable &customRoomProperties=Common::Hashtable(), const Common::JVector< Common::JString > &propsListedInLobby=Common::JVector< Common::JString >(), const Common::JString &lobbyName=Common::JString(), nByte lobbyType=LobbyType::DEFAULT, int playerTtl=0, int emptyRoomTtl=0, bool suppressRoomEvents=false, const Common::JVector< Common::JString > *pPlugins=NULL, bool publishUserID=false, nByte directMode=DirectMode::NONE) | |
~RoomOptions (void) | |
RoomOptions (const RoomOptions &toCopy) | |
RoomOptions & | operator= (const RoomOptions &toCopy) |
bool | getIsVisible (void) const |
RoomOptions & | setIsVisible (bool isVisible) |
bool | getIsOpen (void) const |
RoomOptions & | setIsOpen (bool isOpen) |
nByte | getMaxPlayers (void) const |
RoomOptions & | setMaxPlayers (nByte maxPlayers) |
const Common::Hashtable & | getCustomRoomProperties (void) const |
RoomOptions & | setCustomRoomProperties (const Common::Hashtable &customRoomProperties) |
const Common::JVector< Common::JString > & | getPropsListedInLobby (void) const |
RoomOptions & | setPropsListedInLobby (const Common::JVector< Common::JString > &propsListedInLobby) |
const Common::JString & | getLobbyName (void) const |
RoomOptions & | setLobbyName (const Common::JString &lobbyName) |
nByte | getLobbyType (void) const |
RoomOptions & | setLobbyType (nByte lobbyType) |
int | getPlayerTtl (void) const |
RoomOptions & | setPlayerTtl (int playerTtl) |
int | getEmptyRoomTtl (void) const |
RoomOptions & | setEmptyRoomTtl (int emptyRoomTtl) |
bool | getSuppressRoomEvents (void) const |
RoomOptions & | setSuppressRoomEvents (bool suppressRoomEvents) |
const Common::JVector< Common::JString > * | getPlugins (void) const |
RoomOptions & | setPlugins (const Common::JVector< Common::JString > *pPlugins) |
bool | getPublishUserID (void) const |
RoomOptions & | setPublishUserID (bool publishUserID) |
nByte | getDirectMode (void) const |
RoomOptions & | setDirectMode (nByte directMode) |
virtual Common::JString & | toString (Common::JString &retStr, bool withTypes=false) const |
![]() | |
virtual | ~Base (void) |
![]() | |
virtual | ~ToString (void) |
virtual JString | typeToString (void) const |
JString | toString (bool withTypes=false) const |
Additional Inherited Members | |
![]() | |
static void | setListener (const BaseListener *baseListener) |
static int | getDebugOutputLevel (void) |
static bool | setDebugOutputLevel (int debugLevel) |
static const LogFormatOptions & | getLogFormatOptions (void) |
static void | setLogFormatOptions (const LogFormatOptions &options) |
Detailed Description
This class aggregates the various optional parameters that can be specified on room creation.
Constructor & Destructor Documentation
§ RoomOptions() [1/2]
RoomOptions | ( | bool | isVisible = true , |
bool | isOpen = true , |
||
nByte | maxPlayers = 0 , |
||
const Common::Hashtable & | customRoomProperties = Common::Hashtable() , |
||
const Common::JVector< Common::JString > & | propsListedInLobby = Common::JVector<Common::JString>() , |
||
const Common::JString & | lobbyName = Common::JString() , |
||
nByte | lobbyType = LobbyType::DEFAULT , |
||
int | playerTtl = 0 , |
||
int | emptyRoomTtl = 0 , |
||
bool | suppressRoomEvents = false , |
||
const Common::JVector< Common::JString > * | pPlugins = NULL , |
||
bool | publishUserID = false , |
||
nByte | directMode = DirectMode::NONE |
||
) |
Constructor: Creates a new instance with the specified parameters.
- Parameters
-
isVisible see setIsVisible() - optional, defaults to true. isOpen see setIsOpen() - optional, defaults to true. maxPlayers see setMaxPlayers() - optional, defaults to 0. customRoomProperties see setCustomRoomProperties() - optional, defaults to an empty Hashtable instance. propsListedInLobby see setPropsListedInLobby() - optional, defaults to an empty JVector instance. lobbyName see setLobbyName() - optional, defaults to an empty JString instance. lobbyType see setLobbyType() - optional, defaults to LobbyType::DEFAULT. playerTtl see setPlayerTtl() - optional, defaults to 0. emptyRoomTtl see setEmptyRoomTtl() - optional, defaults to 0. suppressRoomEvents see setSuppressRoomEvents() - optional, defaults to false. pPlugins see setPlugins() - optional, defaults to NULL. publishUserID see setPublishUserID() - optional, defaults to false. directMode see setDirectMode() - optional, defaults to DirectMode::NONE.
§ ~RoomOptions()
~RoomOptions | ( | void | ) |
Destructor.
§ RoomOptions() [2/2]
RoomOptions | ( | const RoomOptions & | toCopy | ) |
Copy-Constructor: Creates a new instance that is a deep copy of the argument instance.
- Parameters
-
toCopy The instance to copy.
Member Function Documentation
§ operator=()
RoomOptions & operator= | ( | const RoomOptions & | toCopy | ) |
operator=.
Makes a deep copy of its right operand into its left operand.
This overwrites old data in the left operand.
§ getIsVisible()
bool getIsVisible | ( | void | ) | const |
- Returns
- the currently set value for the isVisible flag
- See also
- setIsVisible()
§ setIsVisible()
RoomOptions & setIsVisible | ( | bool | isVisible | ) |
Sets the initial state of the rooms visibility flag.
A room that is not visible is excluded from the room lists that are sent to the clients in lobbies. An invisible room can be joined by name but is excluded from random matchmaking.
Use this to "hide" a room and simulate "private rooms". Players can exchange a room name and create the room as invisible to avoid anyone else joining it.
- Remarks
- This function sets the initial value that is used for room creation. To change the value of the flag for an already existing room, see MutableRoom::setIsVisible().
- Parameters
-
isVisible the new value to which the flag will be set
- Returns
- a reference to the instance on which it was called to allow for chaining multiple setter calls
- See also
- getIsVisible()
§ getIsOpen()
bool getIsOpen | ( | void | ) | const |
- Returns
- the currently set value for the isOpen flag
- See also
- setIsOpen()
§ setIsOpen()
RoomOptions & setIsOpen | ( | bool | isOpen | ) |
Sets the initial state of the rooms isOpen flag.
If a room is closed, then no further player can join it until the room gets reopened again. A closed room can still be listed in the lobby (use setIsVisible() to control lobby-visibility).
- Remarks
- This function sets the initial value that is used for room creation. To change the value of the flag for an already existing room, see MutableRoom::setIsOpen().
- Parameters
-
isOpen the new value to which the flag will be set
- Returns
- a reference to the instance on which it was called to allow for chaining multiple setter calls
- See also
- getIsOpen()
§ getMaxPlayers()
nByte getMaxPlayers | ( | void | ) | const |
- Returns
- the currently set max players
- See also
- setmaxPlayers()
§ setMaxPlayers()
RoomOptions & setMaxPlayers | ( | nByte | maxPlayers | ) |
Sets the initial value for the max players setting of the room.
This function sets the maximum number of players that can be inside the room at the same time, including inactive players. 0 means "no limit".
- Remarks
- This function sets the initial value that is used for room creation. To change the max players setting of an already existing room, see MutableRoom::setMaxPlayers().
- Parameters
-
maxPlayers the new maximum amount of players that can be inside the room at the same time
- Returns
- a reference to the instance on which it was called to allow for chaining multiple setter calls
- See also
- getMaxPlayers()
§ getCustomRoomProperties()
const Hashtable & getCustomRoomProperties | ( | void | ) | const |
- Returns
- the currently set custom room properties
- See also
- setCustomRoomProperties()
§ setCustomRoomProperties()
RoomOptions & setCustomRoomProperties | ( | const Common::Hashtable & | customRoomProperties | ) |
Sets the initial custom properties of a room.
Custom room properties are any key-value pairs that you need to define the game's setup. The shorter your key strings are, the better. Example: Map, Mode (could be L"m" when used with L"Map"), TileSet (could be L"t").
- Note
- JString is the only supported type for custom property keys. For custom property values you can use any type that is listed in the Table of Datatypes.
- Remarks
- This function sets the initial custom properties that are used for room creation. To change the custom properties of an already existing room, see MutableRoom::mergeCustomProperties(), MutableRoom::addCustomProperty(), MutableRoom::addCustomProperties(), MutableRoom::removeCustomProperty() and MutableRoom::removeCustomProperties().
- Parameters
-
customRoomProperties a Hashtable of custom property key-value pairs
- Returns
- a reference to the instance on which it was called to allow for chaining multiple setter calls
§ getPropsListedInLobby()
- Returns
- the currently set list of properties to show in the lobby
- See also
- setPropsListedInLobby()
§ setPropsListedInLobby()
RoomOptions & setPropsListedInLobby | ( | const Common::JVector< Common::JString > & | propsListedInLobby | ) |
Sets the initial list of custom properties of the room that should be shown in the lobby.
List the keys of all the custom room properties that should be available to clients that are in a lobby. Use with care. Unless a custom property is essential for matchmaking or user info, it should not be sent to the lobby, which causes traffic and delays for clients in the lobby. Default: No custom properties are sent to the lobby.
- Note
- Properties that are intended to be shown in the lobby should be as compact as possible. Literally every single byte counts here as this info needs to be sent to every client in the lobby for every single visible room, so that with lots of users online and games running this quickly adds up to a lot of data.
- Remarks
- This function sets the initial list of property keys. To change which properties are shown to the lobby for an already existing room see MutableRoom::setPropsListedInLobby().
- Parameters
-
propsListedInLobby the keys of the custom room properties that should be shown in the lobby
- Returns
- a reference to the instance on which it was called to allow for chaining multiple setter calls
§ getLobbyName()
const JString & getLobbyName | ( | void | ) | const |
- Returns
- the currently set lobby name
- See also
- setLobbyName()
§ setLobbyName()
RoomOptions & setLobbyName | ( | const Common::JString & | lobbyName | ) |
Sets the name of the lobby to which the room gets added to.
Rooms can be assigned to different lobbies on room creation. Client::opJoinRandomRoom() only uses those room for matchmaking that are assigned to the lobby in which it is told to be looking for rooms. A lobby can be joined by a call to Client::opJoinLobby() and inside lobbies of certain types clients can receive room lists that contain all visible rooms that are assigned to that lobby.
- Remarks
- If you don't set a lobby name or if you set it to an empty string, then any value that is passed for setLobbyType() gets ignored and the room gets added to the default lobby.
- Lobbies are unique per lobbyName plus lobbyType, so multiple different lobbies may have the same name, as long as they are of a different type.
- Parameters
-
lobbyName identifies for the lobby and needs to be unique within the scope of the lobbyType
- Returns
- a reference to the instance on which it was called to allow for chaining multiple setter calls
- See also
- getLobbyName(), setLobbyType(), Matchmaking Guide
§ getLobbyType()
nByte getLobbyType | ( | void | ) | const |
- Returns
- the currently set lobby type
- See also
- setLobbyType()
§ setLobbyType()
RoomOptions & setLobbyType | ( | nByte | lobbyType | ) |
Sets the type of the lobby to which the room gets added to. Must be one of the values in LobbyType
Please see Matchmaking Guide regarding the differences between the various lobby types.
- Note
- This option gets ignored and the room gets added to the default lobby, if you don't also set the lobby name to a non-empty string via a call to setLobbyName().
- Parameters
-
lobbyType one of the values in LobbyType
- Returns
- a reference to the instance on which it was called to allow for chaining multiple setter calls
- See also
- getLobbyType(), setLobbyName(), LobbyType, Matchmaking Guide
§ getPlayerTtl()
int getPlayerTtl | ( | void | ) | const |
- Returns
- the currently set player time to live in milliseconds
- See also
- setPlayerTtl()
§ setPlayerTtl()
RoomOptions & setPlayerTtl | ( | int | playerTtl | ) |
Sets the player time to live in milliseconds.
If a client disconnects or if it leaves a room with the 'willComeBack' flag set to true, its player becomes inactive first and only gets removed from the room after this timeout.
- -1 and INT_MAX set the inactivity time to 'infinite'.
- 0 (default) deactivates player inactivity.
- All other positive values set the inactivity time to their value in milliseconds.
- All other negative values get ignored and the behavior for them is as if the default value was used.
- Note
- A player is only able to rejoin a room in its existing player slot while it is still inactive. Once it has left for good it will be treated as a completely new player.
- Parameters
-
playerTtl a value between -1 and INT_MAX
- Returns
- a reference to the instance on which it was called to allow for chaining multiple setter calls
§ getEmptyRoomTtl()
int getEmptyRoomTtl | ( | void | ) | const |
- Returns
- the currently set empty room time to live in milliseconds
- See also
- setEmptyRoomTtl()
§ setEmptyRoomTtl()
RoomOptions & setEmptyRoomTtl | ( | int | emptyRoomTtl | ) |
Sets the room time to live in milliseconds.
The amount of time in milliseconds that Photon servers should wait before disposing an empty room. A room is considered empty when there is no active player joined to it. So the room disposal timer starts when the last active player leaves. When a player joins or rejoins the room, then the countdown is reset.
By default, the maximum value allowed is:
- 300000ms (5 minutes) on Photon Cloud
- 60000ms (1minute) on Photon Server
- 0 (default) means that an empty room gets instantly disposed.
- All positive values set the keep-alive time to their value in milliseconds.
- All negative values get ignored and the behavior for them is as if the default value was used.
- Note
- The disposal of a room means that the room gets removed from memory on the server side. Without accordingly configured Webhooks this also means that the room will be destroyed and all data related to it (like room and player properties, event caches, inactive players, etc.) gets deleted. When Webhooks for the used appID have been been setup for room persistence, then disposed rooms get stored for later retrieval. Stored rooms get reconstructed in memory when a player joins or rejoins them
- Parameters
-
emptyRoomTtl a value between 0 and INT_MAX
- Returns
- a reference to the instance on which it was called to allow for chaining multiple setter calls
- See also
- getEmptyRoomTtl(), Persistence Guide, Webhooks FAQ
§ getSuppressRoomEvents()
bool getSuppressRoomEvents | ( | void | ) | const |
- Returns
- the currently set value for the suppressRoomEvents flag
- See also
- setSuppressRoomEvents()
§ setSuppressRoomEvents()
RoomOptions & setSuppressRoomEvents | ( | bool | suppressRoomEvents | ) |
Sets the value of the suppressRoomEvents flag which determines if the server should skip room events for joining and leaving players.
Setting this flag to true makes the client unaware of the other players in a room. That can save some traffic if you have some server logic that updates players, but it can also limit the client's usability.
- Parameters
-
suppressRoomEvents the new value to which the flag will be set
- Returns
- a reference to the instance on which it was called to allow for chaining multiple setter calls
- See also
- getSuppressRoomEvents()
§ getPlugins()
- Returns
- the currently set list of plugins
- See also
- setPlugins()
§ setPlugins()
RoomOptions & setPlugins | ( | const Common::JVector< Common::JString > * | pPlugins | ) |
Informs the server of the expected plugin setup.
The operation will fail in case of a plugin mismatch returning ErrorCode::PLUGIN_MISMATCH. Setting an empty JVector means that the client expects no plugin to be setup. Note: for backwards compatibility setting NULL (the default value) omits any check.
- Parameters
-
pPlugins the expected plugins
- Returns
- a reference to the instance on which it was called to allow for chaining multiple setter calls
- See also
- getPlugins()
§ getPublishUserID()
bool getPublishUserID | ( | void | ) | const |
- Returns
- the currently set value for the publishUserID flag
- See also
- setPublishUserID()
§ setPublishUserID()
RoomOptions & setPublishUserID | ( | bool | publishUserID | ) |
Defines if the UserIds of players get "published" in the room. Useful for Client::opFindFriends(), if players want to play another game together.
When you set this to true, Photon will publish the UserIds of the players in that room. In that case, you can use Player::getUserID(), to access any player's userID. This is useful for FindFriends and to set "expected users" to reserve slots in a room (see Client::opCreateRoom(), Client::opJoinOrCreateRoom() and Client::opJoinRoom()).
- Parameters
-
publishUserID true, if userIDs should be published, false otherwise
- Returns
- a reference to the instance on which it was called to allow for chaining multiple setter calls
- See also
- getPublishUserID()
§ getDirectMode()
nByte getDirectMode | ( | void | ) | const |
- Returns
- the currently set value for the DirectMode flag
- See also
- setDirectMode()
§ setDirectMode()
RoomOptions & setDirectMode | ( | nByte | directMode | ) |
Sets the DirectMode that should be used for this room.
The value of this option determines if clients establish direct peer to peer connections with other clients that can then be used to send them direct peer to peer messages with Client::sendDirect().
- Parameters
-
directMode one of the values in DirectMode
- Returns
- a reference to the instance on which it was called to allow for chaining multiple setter calls
- See also
- getDirectMode()
§ toString()
|
virtual |
- Remarks
- The cost of this function depends a lot on implementation details of the implementing subclasses, but for container classes this function can become quite expensive, if the instance contains huge amounts of data, as its cost for many container class implementations increases disproportionately high to the size of the payload.
- Parameters
-
retStr reference to a string, to store the return-value in; the information, which is generated by this function, will be attached at the end of any eventually existing previous content of the string withTypes set to true, to include type information in the generated string
- Returns
- a JString representation of the instance and its contents for debugging purposes.
Implements ToString.