![]() |

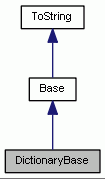
Public Member Functions | |
virtual | ~DictionaryBase (void) |
DictionaryBase (const DictionaryBase &toCopy) | |
DictionaryBase & | operator= (const DictionaryBase &toCopy) |
bool | operator== (const DictionaryBase &toCompare) const |
bool | operator!= (const DictionaryBase &toCompare) const |
template<typename FKeyType > | |
void | remove (const FKeyType &key) |
template<typename FKeyType > | |
bool | contains (const FKeyType &key) const |
void | removeAllElements (void) |
JString | typeToString (void) const |
JString & | toString (JString &retStr, bool withTypes=false) const |
const Hashtable & | getHashtable (void) const |
unsigned int | getSize (void) const |
virtual const nByte * | getKeyTypes (void) const |
virtual const nByte * | getValueTypes (void) const |
template<typename FKeyType > | |
const short * | getValueSizes (const FKeyType &key) const |
virtual const unsigned int * | getValueDimensions (void) const |
template<typename FKeyType , typename FValueType > | |
const FValueType * | getValue (const FKeyType &key, const FValueType *) const |
template<typename FKeyType > | |
const Object * | getValue (const FKeyType &key, const Object *) const |
template<typename FKeyType > | |
JVector< FKeyType > | getKeys (const FKeyType *) const |
JVector< Object > | getKeys (const Object *) const |
![]() | |
virtual | ~Base (void) |
![]() | |
virtual | ~ToString (void) |
JString | toString (bool withTypes=false) const |
Additional Inherited Members | |
![]() | |
static void | setListener (const BaseListener *baseListener) |
static int | getDebugOutputLevel (void) |
static bool | setDebugOutputLevel (int debugLevel) |
static const LogFormatOptions & | getLogFormatOptions (void) |
static void | setLogFormatOptions (const LogFormatOptions &options) |
Detailed Description
The DictionaryBase class is the base class for the Dictionary class template and intended to be used instead of Dictionary in cases, when the key type and/or value type of a Dictionary instance can't be known at compile time, but only at runtime.
Whenever possible you should use the class template Dictionary instead of DictionaryBase to enable compile time type safety and optimizations that need compile time type identification. However, when for example receiving unknown data over the network at runtime, the type of that data can't be non at compile time. In those cases DictionaryBase instances are used.
DictionaryBase instances only offer read only API: They can't be modified with the exception of replacing the complete instance with the content of another one. No single entries can be added, removed, or changed. Use the Dictionary sub class template for modifiable Dictionary instances.
Please have a look at the Table of Datatypes for a list of types, that are supported as keys and as values.
Please refer to the documentation for put() and getValue() to see how to store and access data in a Dictionary.
- See also
- getValue(), Dictionary
Constructor & Destructor Documentation
§ ~DictionaryBase()
|
virtual |
Destructor.
§ DictionaryBase()
DictionaryBase | ( | const DictionaryBase & | toCopy | ) |
Copy-Constructor: Creates a deep copy of the argument.
- Parameters
-
toCopy The object to copy.
Member Function Documentation
§ operator=()
DictionaryBase & operator= | ( | const DictionaryBase & | toCopy | ) |
operator=. Makes a deep copy of its right operand into its left operand. This overwrites old data in the left operand.
§ operator==()
bool operator== | ( | const DictionaryBase & | toCompare | ) | const |
operator==.
- Returns
- true, if both operands are equal, false otherwise.
Two instances are considered equal if they each hold the same number of entries and, for a given key, the corresponding values equal each other.
Two values are considered equal to each other, if instances of class Object, that are holding them as payloads, equal each other.
- See also
- Object::operator==()
§ operator!=()
bool operator!= | ( | const DictionaryBase & | toCompare | ) | const |
operator!=.
- Returns
- false, if operator==() would return true, true otherwise.
§ remove()
void remove | ( | const FKeyType & | key | ) |
Deletes the specified key and the corresponding value, if found in the Hashtable.
- Parameters
-
key Pointer to the key of the key/value-pair to remove.
- Returns
- nothing.
- See also
- removeAllElements()
§ contains()
bool contains | ( | const FKeyType & | key | ) | const |
Checks, whether the Hashtable contains a certain key.
- Parameters
-
key Pointer to the key to look up.
- Returns
- true if the specified key was found, false otherwise.
§ removeAllElements()
void removeAllElements | ( | void | ) |
§ typeToString()
|
virtual |
- Remarks
- This function is intended for debugging purposes. For runtime type checking you should use RTTI's typeid() instead. Demangling and cutting off of namespaces will only happen on platforms, which offer a system functionality for demangling.
- Returns
- a string representation of the class name of the polymorphically correct runtime class of the instance, on which it is called on, after this class name has been demangled and eventual namespaces have been removed.
Reimplemented from ToString.
Reimplemented in Dictionary< nByte, Common::ExitGames::Common::Object >, and Dictionary< nByte, Common::Object >.
§ toString()
- Remarks
- The cost of this function depends a lot on implementation details of the implementing subclasses, but for container classes this function can become quite expensive, if the instance contains huge amounts of data, as its cost for many container class implementations increases disproportionately high to the size of the payload.
- Parameters
-
retStr reference to a string, to store the return-value in; the information, which is generated by this function, will be attached at the end of any eventually existing previous content of the string withTypes set to true, to include type information in the generated string
- Returns
- a JString representation of the instance and its contents for debugging purposes.
Implements ToString.
Reimplemented in Dictionary< nByte, Common::ExitGames::Common::Object >, and Dictionary< nByte, Common::Object >.
§ getHashtable()
const Hashtable & getHashtable | ( | void | ) | const |
- Returns
- a readonly reference to a Hashtable representation of the Dictionary instance. The returned reference refers to the original payload data of the Dictionary, so its payload will change, if the payload of the according Dictionary instance changes.
§ getSize()
unsigned int getSize | ( | void | ) | const |
- Returns
- the number of key/value pairs stored in the Hashtable.
§ getKeyTypes()
|
virtual |
- Returns
- an array, holding the type code for the key type of the Dictionary and type codes for the key types of potential nested Dictionaries.
Only index 0 of the returned array is guaranteed to be valid. The existence of elements at other indices depends on the value of the element in the array returned by getValueTypes() at the previous index in the following way: Only when getValueTypes()[i] == TypeCode::DICTIONARY, then getKeyTypes()[i+1] will be valid.
Type information for nested Dictionaries will be stored like in the following example: Dictionary<int, Dictionary<short, float**>*> This is a Dictionary, with the key type being int and the value type being a 1D array of type Dictionary<short, float**>, so that all values are Dictionaries, which keys are shorts and which values are 2D arrays of float. This function's return value in this example will hold the values TypeCode::INTEGER at index 0 and TypeCode::SHORT at index 1.
The codes returned by this function match the ones, that are stored in member variable "typename" of class template Helpers::ConfirmAllowedKey's specializations. Only the types, for which specializations of that template exist, are valid Dictionary keys.
Reimplemented in Dictionary< EKeyType, EValueType >, Dictionary< nByte, Common::ExitGames::Common::Object >, and Dictionary< nByte, Common::Object >.
§ getValueTypes()
|
virtual |
- Returns
- an array, holding the type code for the value type of the Dictionary and type codes for the value types of potential nested Dictionaries.
Only index 0 of the returned array is guaranteed to be valid. The existence of elements at other indices depends on the value of the element at the previous index in the following way: Only when getValueTypes()[i] == TypeCode::DICTIONARY, then getValueTypes()[i+1] will be valid.
Type information for nested Dictionaries will be stored like in the following example: Dictionary<int, Dictionary<short, float**>*> This is a Dictionary, with the key type being int and the value type being a 1D array of type Dictionary<short, float**>, so that all values are Dictionaries, which keys are shorts and which values are 2D arrays of float. This function's return value in this example will hold the values TypeCode::DICTIONARY at index 0 and TypeCode::FLOAT at index 1.
The codes returned by this function match the ones, that are stored in member variable "typename" of class template Helpers::ConfirmAllowed's specializations. Only the types, for which specializations of that template exist, are valid Dictionary values.
Reimplemented in Dictionary< EKeyType, EValueType >, Dictionary< nByte, Common::ExitGames::Common::Object >, and Dictionary< nByte, Common::Object >.
§ getValueSizes()
const short * getValueSizes | ( | const FKeyType & | key | ) | const |
- Returns
- Object::getSizes() of the value, that corresponds to the passed key.
- Parameters
-
key Reference to the key to return the corresponding value sizes for
§ getValueDimensions()
|
virtual |
- Returns
- an array, holding the amount of array dimensions for the value type of the Dictionary and for the value types of potential nested Dictionaries.
Only index 0 of the returned array is guaranteed to be valid. The existence of elements at other indices depends on the value of the element in the array returned by getValueTypes() at the previous index in the following way: Only when getValueTypes()[i] == TypeCode::DICTIONARY, then getValueDimensions()[i+1] will be valid.
Type information for nested Dictionaries will be stored like in the following example: Dictionary<int, Dictionary<short, float**>*> This is a Dictionary, with the key type being int and the value type being a 1D array of type Dictionary<short, float**>, so that all values are Dictionaries, which keys are shorts and which values are 2D arrays of float. This function's return value in this example will hold the value 1 (for 1D array) at index 0 and 2 (for 2D) at index 1. If a value type is no array, then this functions return value will contain 0 at the corresponding index.
Reimplemented in Dictionary< EKeyType, EValueType >, Dictionary< nByte, Common::ExitGames::Common::Object >, and Dictionary< nByte, Common::Object >.
§ getValue() [1/2]
const FValueType * getValue | ( | const FKeyType & | key, |
const FValueType * | |||
) | const |
Returns the corresponding value for a specified key.
- Parameters
-
key Reference to the key to return the corresponding value for.
- Returns
- a pointer to the corresponding value if the Hashtable contains the specified key, NULL otherwise.
- See also
- put()
§ getValue() [2/2]
Returns the corresponding value for a specified key.
- Parameters
-
key Reference to the key to return the corresponding value for.
- Returns
- a pointer to the corresponding value if the Hashtable contains the specified key, NULL otherwise.
- See also
- put()
§ getKeys() [1/2]
JVector< FKeyType > getKeys | ( | const FKeyType * | ) | const |