![]() |
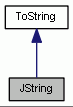
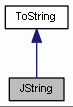
Public Member Functions | |
JString (unsigned int bufferlen=0) | |
JString (const char *Value) | |
JString (const EG_CHAR *Value) | |
JString (const JString &Value) | |
JString (const UTF8String &Value) | |
JString (const ANSIString &Value) | |
~JString (void) | |
JString & | operator= (const JString &Rhs) |
JString & | operator= (const char *Rhs) |
JString & | operator= (const EG_CHAR *Rhs) |
JString & | operator= (const UTF8String &Rhs) |
JString & | operator= (const ANSIString &Rhs) |
JString & | operator= (char Rhs) |
JString & | operator= (signed char Rhs) |
JString & | operator= (unsigned char Rhs) |
JString & | operator= (EG_CHAR Rhs) |
JString & | operator= (short aNum) |
JString & | operator= (unsigned short aNum) |
JString & | operator= (int aNum) |
JString & | operator= (unsigned int aNum) |
JString & | operator= (long aNum) |
JString & | operator= (unsigned long aNum) |
JString & | operator= (long long aNum) |
JString & | operator= (unsigned long long aNum) |
JString & | operator= (float aNum) |
JString & | operator= (double aNum) |
JString & | operator= (long double aNum) |
JString & | operator= (bool aBool) |
operator const EG_CHAR * (void) const | |
JString & | operator+= (const JString &Rhs) |
template<typename Etype > | |
JString & | operator+= (const Etype &Rhs) |
bool | operator== (const JString &Rhs) const |
bool | operator!= (const JString &Rhs) const |
bool | operator< (const JString &Rhs) const |
bool | operator> (const JString &Rhs) const |
bool | operator<= (const JString &Rhs) const |
bool | operator>= (const JString &Rhs) const |
EG_CHAR | operator[] (unsigned int Index) const |
EG_CHAR & | operator[] (unsigned int Index) |
unsigned int | capacity (void) const |
EG_CHAR | charAt (unsigned int index) const |
int | compareTo (const JString &anotherString) const |
const JString & | concat (const JString &str) |
const EG_CHAR * | cstr (void) const |
JString | deleteChars (unsigned int start, unsigned int length) const |
bool | endsWith (const JString &suffix) const |
void | ensureCapacity (unsigned int minCapacity) |
bool | equals (const JString &anotherString) const |
bool | equalsIgnoreCase (const JString &anotherString) const |
int | indexOf (char ch) const |
int | indexOf (char ch, unsigned int fromIndex) const |
int | indexOf (EG_CHAR ch) const |
int | indexOf (EG_CHAR ch, unsigned int fromIndex) const |
int | indexOf (const JString &str) const |
int | indexOf (const JString &str, unsigned int fromIndex) const |
int | lastIndexOf (char ch) const |
int | lastIndexOf (char ch, unsigned int fromIndex) const |
int | lastIndexOf (EG_CHAR ch) const |
int | lastIndexOf (EG_CHAR ch, unsigned int fromIndex) const |
int | lastIndexOf (const JString &str) const |
int | lastIndexOf (const JString &str, unsigned int fromIndex) const |
unsigned int | length (void) const |
JString | replace (char oldChar, char newChar) const |
JString | replace (EG_CHAR oldChar, EG_CHAR newChar) const |
JString | replace (const JString &match, const JString &replacement) const |
bool | startsWith (const JString &prefix) const |
bool | startsWith (const JString &prefix, unsigned int offset) const |
JString | substring (unsigned int beginIndex) const |
JString | substring (unsigned int beginIndex, unsigned int endIndex) const |
JString | toLowerCase (void) const |
JString | toUpperCase (void) const |
int | toInt (void) const |
JString | trim (void) |
UTF8String | UTF8Representation (void) const |
ANSIString | ANSIRepresentation (void) const |
JString & | toString (JString &retStr, bool withTypes=false) const |
![]() | |
virtual | ~ToString (void) |
virtual JString | typeToString (void) const |
JString | toString (bool withTypes=false) const |
Related Functions | |
(Note that these are not member functions.) | |
template<typename _Elem , typename _Traits > | |
std::basic_ostream< _Elem, _Traits > & | operator<< (::std::basic_ostream< _Elem, _Traits > &stream, const JString &string) |
template<typename Etype > | |
JString | operator+ (const JString &Lsh, const Etype &Rsh) |
template<typename Etype > | |
JString | operator+ (const Etype &Lsh, const JString &Rsh) |
template<typename Etype > | |
bool | operator== (const JString &Lsh, const Etype &Rsh) |
template<typename Etype > | |
bool | operator== (const Etype &Lsh, const JString &Rsh) |
template<typename Etype > | |
bool | operator!= (const JString &Lsh, const Etype &Rsh) |
template<typename Etype > | |
bool | operator!= (const Etype &Lsh, const JString &Rsh) |
template<typename Etype > | |
bool | operator< (const JString &Lsh, const Etype &Rsh) |
template<typename Etype > | |
bool | operator< (const Etype &Lsh, const JString &Rsh) |
template<typename Etype > | |
bool | operator> (const JString &Lsh, const Etype &Rsh) |
template<typename Etype > | |
bool | operator> (const Etype &Lsh, const JString &Rsh) |
template<typename Etype > | |
bool | operator<= (const JString &Lsh, const Etype &Rsh) |
template<typename Etype > | |
bool | operator<= (const Etype &Lsh, const JString &Rsh) |
template<typename Etype > | |
bool | operator>= (const JString &Lsh, const Etype &Rsh) |
template<typename Etype > | |
bool | operator>= (const Etype &Lsh, const JString &Rsh) |
JString | operator+ (const JString &Lsh, const JString &Rsh) |
Detailed Description
The JString class is a representation of Text strings, based on the String class from Sun Java.
This class is used to avoid dealing with char pointers/arrays directly, while staying independent from the String class in the Standard Template Library of C++, as some compilers do not implement the STL.
Constructor & Destructor Documentation
§ JString() [1/6]
|
explicit |
Constructor: Creates an empty JString.
- Remarks
- By default no memory is allocated for the internal buffer. You can however pass the number of characters to allocate memory for. If that number is too big, then you will waste memory, but with a reasonable bufferlen you can avoid expensive later reallocations, when appending to the string.
- Parameters
-
bufferlen optional, let the string allocate memory for x characters
§ JString() [2/6]
JString | ( | const char * | Value | ) |
Copy-Constructor: Creates a new JString from a deep copy of the argument string.
- Parameters
-
Value The UTF8 string to copy.
§ JString() [3/6]
JString | ( | const EG_CHAR * | Value | ) |
Copy-Constructor: Creates a new JString from a deep copy of the argument string.
- Parameters
-
Value The UTF16 string to copy.
§ JString() [4/6]
§ JString() [5/6]
JString | ( | const UTF8String & | Value | ) |
Copy-Constructor: Creates a new JString from a deep copy of the argument string.
- Parameters
-
Value The UTF8String to copy.
§ JString() [6/6]
JString | ( | const ANSIString & | Value | ) |
Copy-Constructor: Creates a new JString from a deep copy of the argument string.
- Parameters
-
Value :The ANSIString to copy.
§ ~JString()
~JString | ( | void | ) |
Destructor.
Member Function Documentation
§ operator=() [1/21]
operator=.
Makes a deep copy of its right operand into its left operand.
This overwrites old data in the left operand.
§ operator=() [2/21]
JString & operator= | ( | const char * | Rhs | ) |
operator=.
Makes a deep copy of its right operand (which is assumed to be encoded as UTF8) into its left operand.
This overwrites old data in the left operand.
§ operator=() [3/21]
JString & operator= | ( | const EG_CHAR * | Rhs | ) |
operator=.
Makes a deep copy of its right operand into its left operand.
This overwrites old data in the left operand.
§ operator=() [4/21]
JString & operator= | ( | const UTF8String & | Rhs | ) |
operator=.
Makes a deep copy of its right operand into its left operand.
This overwrites old data in the left operand.
§ operator=() [5/21]
JString & operator= | ( | const ANSIString & | Rhs | ) |
operator=.
Makes a deep copy of its right operand into its left operand.
This overwrites old data in the left operand.
§ operator=() [6/21]
JString & operator= | ( | char | aChar | ) |
operator=.
saves a string representation of its right operand in its left operand.
This overwrites old data in the left operand.
§ operator=() [7/21]
JString & operator= | ( | signed char | aChar | ) |
operator=.
saves a string representation of its right operand in its left operand.
This overwrites old data in the left operand.
§ operator=() [8/21]
JString & operator= | ( | unsigned char | aChar | ) |
operator=.
saves a string representation of its right operand in its left operand.
This overwrites old data in the left operand.
§ operator=() [9/21]
JString & operator= | ( | EG_CHAR | aWideChar | ) |
operator=.
saves a string representation of its right operand in its left operand.
This overwrites old data in the left operand.
§ operator=() [10/21]
JString & operator= | ( | short | aNum | ) |
operator=.
saves a string representation of its right operand in its left operand.
This overwrites old data in the left operand.
§ operator=() [11/21]
JString & operator= | ( | unsigned short | aNum | ) |
operator=.
saves a string representation of its right operand in its left operand.
This overwrites old data in the left operand.
§ operator=() [12/21]
JString & operator= | ( | int | aNum | ) |
operator=.
saves a string representation of its right operand in its left operand.
This overwrites old data in the left operand.
§ operator=() [13/21]
JString & operator= | ( | unsigned int | aNum | ) |
operator=.
saves a string representation of its right operand in its left operand.
This overwrites old data in the left operand.
§ operator=() [14/21]
JString & operator= | ( | long | aNum | ) |
operator=.
saves a string representation of its right operand in its left operand.
This overwrites old data in the left operand.
§ operator=() [15/21]
JString & operator= | ( | unsigned long | aNum | ) |
operator=.
saves a string representation of its right operand in its left operand.
This overwrites old data in the left operand.
§ operator=() [16/21]
JString & operator= | ( | long long | aNum | ) |
operator=.
saves a string representation of its right operand in its left operand.
This overwrites old data in the left operand.
§ operator=() [17/21]
JString & operator= | ( | unsigned long long | aNum | ) |
operator=.
saves a string representation of its right operand in its left operand.
This overwrites old data in the left operand.
§ operator=() [18/21]
JString & operator= | ( | float | aNum | ) |
operator=.
saves a string representation of its right operand in its left operand.
This overwrites old data in the left operand.
§ operator=() [19/21]
JString & operator= | ( | double | aNum | ) |
operator=.
saves a string representation of its right operand in its left operand.
This overwrites old data in the left operand.
§ operator=() [20/21]
JString & operator= | ( | long double | aNum | ) |
operator=.
saves a string representation of its right operand in its left operand.
This overwrites old data in the left operand.
§ operator=() [21/21]
JString & operator= | ( | bool | aBool | ) |
operator=.
saves a string representation of its right operand in its left operand.
This overwrites old data in the left operand.
§ operator const EG_CHAR *()
operator const EG_CHAR * | ( | void | ) | const |
operator const EG_CHAR*.
Copies a pointer to the content of its right operand into its left operand.
This overwrites old data in the left operand.
§ operator+=() [1/2]
operator+=.
Attaches its right operand to its left operand.
§ operator+=() [2/2]
JString & operator+= | ( | const Etype & | Rhs | ) |
operator+=.
Attaches its right operand to its left operand.
§ operator==()
bool operator== | ( | const JString & | Rhs | ) | const |
operator==.
- Returns
- true, if both operands are equal, false otherwise.
§ operator!=()
bool operator!= | ( | const JString & | Rhs | ) | const |
operator!=.
- Returns
- false, if both operands are equal, true otherwise.
§ operator<()
bool operator< | ( | const JString & | Rhs | ) | const |
operator<. The return value indicates the lexicographic relation between the operands.
- Returns
- true, if left operand is less than right operand, false otherwise.
§ operator>()
bool operator> | ( | const JString & | Rhs | ) | const |
operator>. The return value indicates the lexicographic relation between the operands.
- Returns
- true, if left operand is greater than right operand, false otherwise.
§ operator<=()
bool operator<= | ( | const JString & | Rhs | ) | const |
operator<=. The return value indicates the lexicographic relation between the operands.
- Returns
- true, if the left operand is less than or equal to the right operand, false otherwise.
§ operator>=()
bool operator>= | ( | const JString & | Rhs | ) | const |
operator>=. The return value indicates the lexicographic relation between the operands.
- Returns
- true, if the left operand is greater than or equal to the right operand, false otherwise.
§ operator[]() [1/2]
EG_CHAR operator[] | ( | unsigned int | index | ) | const |
operator[]. Accesses the character of the string at the given index like in an array. This does not check for valid indexes and shows undefined behavior for invalid indexes
§ operator[]() [2/2]
EG_CHAR & operator[] | ( | unsigned int | index | ) |
operator[]. Accesses the character of the string at the given index like in an array. This does not check for valid indexes and shows undefined behavior for invalid indexes
§ capacity()
unsigned int capacity | ( | void | ) | const |
Returns the current capacity of the JString.
- Returns
- the current capacity in characters.
§ charAt()
EG_CHAR charAt | ( | unsigned int | index | ) | const |
Returns the character of the JString at the passed index. This does not check for valid indexes and shows undefined behavior for invalid indexes!
- Parameters
-
index the index of the element, that should be returned. Must not be bigger than the current size of the string!
- Returns
- the character at the passed index.
§ compareTo()
int compareTo | ( | const JString & | anotherString | ) | const |
§ concat()
Attaches the passed string to the string, the function is called for.
- Parameters
-
str the string to attach
- Returns
- the string, the function was called for, after the parameter string was attached to it.
§ cstr()
const EG_CHAR * cstr | ( | void | ) | const |
§ deleteChars()
JString deleteChars | ( | unsigned int | start, |
unsigned int | length | ||
) | const |
Deletes a substring inside a returned copy of the string. This does not affect the original string.
- Parameters
-
start start of the substring length length of the substring
- Returns
- a copy of the string, after deleting the specified substring from the copy, or an empty string for invalid parameters
§ endsWith()
bool endsWith | ( | const JString & | suffix | ) | const |
Checks, if the JString, this function is called for, ends with the passed string.
- Parameters
-
suffix the string to check for, if the other one ends with it
- Returns
- true, if the string, the function is called for, ends with the passed string, false otherwise.
§ ensureCapacity()
void ensureCapacity | ( | unsigned int | minCapacity | ) |
Resizes the JString to the passed capacity, if its old capacity has been smaller. Most likely the whole JString has to be copied into new memory, so this is an expensive operation for huge JStrings. Call this function first, before you use concat()-function and/or +=operators a lot of times on this JString-instance, to avoid multiple expensive resizes through appending.
- Parameters
-
minCapacity the new capacity for the JString in number of characters.
- Returns
- nothing.
§ equals()
bool equals | ( | const JString & | anotherString | ) | const |
Checks, if the JString, this function is called for, is equal to the passed string. This function is case-sensitive.
- Parameters
-
anotherString the string to check for, if it is equal to other one
- Returns
- true, if both strings are equal to each other, false otherwise.
§ equalsIgnoreCase()
bool equalsIgnoreCase | ( | const JString & | anotherString | ) | const |
Checks, if the JString, this function is called for, is, equal to the passed string. This function is not case-sensitive.
- Parameters
-
anotherString the string to check for, if it is equal to other one
- Returns
- true, if both strings are equal to each other, false otherwise.
§ indexOf() [1/6]
int indexOf | ( | char | ch | ) | const |
Returns the index of the first occurrence of the parameter in the string, the function is called for. Searching begins at the first character of the string and goes forward, until the end of the string is reached.
- Parameters
-
ch the character to search for
- Returns
- the index of the first occurrence of the parameter or -1 if it could not be found at all
§ indexOf() [2/6]
int indexOf | ( | char | ch, |
unsigned int | fromIndex | ||
) | const |
Returns the index of the first occurrence of the parameter in the string, the function is called for. Searching begins at the passed index and goes forward, until the end of the string is reached.
- Parameters
-
ch the character to search for fromIndex the index, to begin the search from
- Returns
- the index of the first occurrence of the first parameter or -1 if it could not be found at all
§ indexOf() [3/6]
int indexOf | ( | EG_CHAR | ch | ) | const |
Returns the index of the first occurrence of the parameter in the string, the function is called for. Searching begins at the first character of the string and goes forward, until the end of the string is reached.
- Parameters
-
ch the character to search for
- Returns
- the index of the first occurrence of the parameter or -1 if it could not be found at all
§ indexOf() [4/6]
int indexOf | ( | EG_CHAR | ch, |
unsigned int | fromIndex | ||
) | const |
Returns the index of the first occurrence of the parameter in the string, the function is called for. Searching begins at the passed index and goes forward, until the end of the string is reached.
- Parameters
-
ch the character to search for fromIndex the index, to begin the search from
- Returns
- the index of the first occurrence of the first parameter or -1 if it could not be found at all
§ indexOf() [5/6]
int indexOf | ( | const JString & | str | ) | const |
Returns the index of the first occurrence of the parameter in the string, the function is called for. Searching begins at the first character of the string and goes forward, until the end of the string is reached.
- Parameters
-
str the string to search for
- Returns
- the index of the first occurrence of the parameter or -1 if it could not be found at all
§ indexOf() [6/6]
int indexOf | ( | const JString & | str, |
unsigned int | fromIndex | ||
) | const |
Returns the index of the first occurrence of the parameter in the string, the function is called for. Searching begins at the passed index and goes forward, until the end of the string is reached.
- Parameters
-
str the string to search for fromIndex the index, to begin the search from
- Returns
- the index of the first occurrence of the first parameter or -1 if it could not be found at all
§ lastIndexOf() [1/6]
int lastIndexOf | ( | char | ch | ) | const |
Returns the index of the last occurrence of the parameter in the string, the function is called on. Searching begins at the last character of the string and goes forward, until the start of the string is reached.
- Parameters
-
ch the character to search for
- Returns
- the index of the last occurrence of the parameter or -1 if it could not be found at all
§ lastIndexOf() [2/6]
int lastIndexOf | ( | char | ch, |
unsigned int | fromIndex | ||
) | const |
Returns the index of the last occurrence of the parameter in the string, the function is called on. Searching begins at the passed index and goes backward, until the start of the string is reached.
- Parameters
-
ch the character to search for fromIndex the index, to begin the search from
- Returns
- the index of the last occurrence of the first parameter or -1 if it could not be found at all
§ lastIndexOf() [3/6]
int lastIndexOf | ( | EG_CHAR | ch | ) | const |
Returns the index of the last occurrence of the parameter in the string, the function is called on. Searching begins at the last character of the string and goes forward, until the start of the string is reached.
- Parameters
-
ch the character to search for
- Returns
- the index of the last occurrence of the parameter or -1 if it could not be found at all
§ lastIndexOf() [4/6]
int lastIndexOf | ( | EG_CHAR | ch, |
unsigned int | fromIndex | ||
) | const |
Returns the index of the last occurrence of the parameter in the string, the function is called on. Searching begins at the passed index and goes backward, until the start of the string is reached.
- Parameters
-
ch the character to search for fromIndex the index, to begin the search from
- Returns
- the index of the last occurrence of the first parameter or -1 if it could not be found at all
§ lastIndexOf() [5/6]
int lastIndexOf | ( | const JString & | str | ) | const |
Returns the index of the last occurrence of the parameter in the string, the function is called for. Searching begins at the last character of the string and goes forward, until the start of the string is reached.
- Parameters
-
str the string to search for
- Returns
- the index of the last occurrence of the parameter or -1 if it could not be found at all
§ lastIndexOf() [6/6]
int lastIndexOf | ( | const JString & | str, |
unsigned int | fromIndex | ||
) | const |
Returns the index of the last occurrence of the parameter in the string, the function is called for. Searching begins at the passed index and goes backward, until the start of the string is reached.
- Parameters
-
str the string to search for fromIndex the index, to begin the search from
- Returns
- the index of the last occurrence of the first parameter or -1 if it could not be found at all
§ length()
unsigned int length | ( | void | ) | const |
- Returns
- the length of the string in characters
§ replace() [1/3]
JString replace | ( | char | oldChar, |
char | newChar | ||
) | const |
Searches the string for all occurrences of parameter 1 and replaces them with parameter 2. The result of the replacements is returned as a new instance, while the original string stays unchanged.
- Parameters
-
oldChar the character to search for newChar the character to replace the other one with
- Returns
- a copy of the string, the function was called for, in which all occurrences of parameter 1 have been replaced with parameter 2.
§ replace() [2/3]
JString replace | ( | EG_CHAR | oldChar, |
EG_CHAR | newChar | ||
) | const |
Searches the string for all occurrences of parameter 1 and replaces them with parameter 2. The result of the replacements is returned as a new instance, while the original string stays unchanged.
- Parameters
-
oldChar the character to search for newChar the character to replace oldChar with
- Returns
- a copy of the string, the function was called for, in which all occurrences of parameter 1 have been replaced with parameter 2.
§ replace() [3/3]
Searches the string for all occurrences of parameter 1 and replaces them with parameter 2. The result of the replacements is returned as a new instance, while the original string stays unchanged.
- Parameters
-
match the substring to search for replacement the string to replace match with
- Returns
- a copy of the string, the function was called for, in which all occurrences of parameter 1 have been replaced with parameter 2.
§ startsWith() [1/2]
bool startsWith | ( | const JString & | prefix | ) | const |
Checks, if the string, begins with the passed prefix
- Parameters
-
prefix the prefix to search for
- Returns
- true, if the string begins with the prefix, false otherwise
§ startsWith() [2/2]
bool startsWith | ( | const JString & | prefix, |
unsigned int | offset | ||
) | const |
Checks, if the substring of the string, starting at the passed index, begins with the passed prefix
- Parameters
-
prefix the prefix to search for offset start of the substring to check for
- Returns
- true, if the substring begins with the prefix, false otherwise
§ substring() [1/2]
JString substring | ( | unsigned int | beginIndex | ) | const |
Returns a substring of the string, beginning at the passed index
- Parameters
-
beginIndex start of the substring to return
- Returns
- a substring, beginning at the passed index
§ substring() [2/2]
JString substring | ( | unsigned int | beginIndex, |
unsigned int | endIndex | ||
) | const |
Returns a substring of the string, beginning at the first passed index and ending at the second one
- Parameters
-
beginIndex index of the first character of the substring to return endIndex index of the last character of the substring to return + 1
- Remarks
- This function will treat the second index as first one and vice versa, if the first is bigger than the second one.
- Returns
- a substring, beginning at the first passed index and ending at the second one
§ toLowerCase()
JString toLowerCase | ( | void | ) | const |
Copies the string and changes all upper case characters in the copy to lower case. This does not affect the original string.
- Returns
- a lowercase copy of the string
§ toUpperCase()
JString toUpperCase | ( | void | ) | const |
Copies the string and changes all lower case characters in the copy to upper case. This does not affect the original string.
- Returns
- an uppercase copy of the string
§ toInt()
int toInt | ( | void | ) | const |
Converts the string into an integer. Conversion ends at the first character, that can not be interpreted as a number.
- Returns
- the integer value produced by interpreting the string as a number or 0 if it could not be interpreted
§ trim()
JString trim | ( | void | ) |
Removes all whitespaces at the start and end of the string. e.g.: L" Hello World! " -> "Hello World!"
- Returns
- the string without any whitespaces at its start or end.
§ UTF8Representation()
UTF8String UTF8Representation | ( | void | ) | const |
Converts the string to UTF8 and returns the converted string. Use this, if you need to pass the JString to an API, which does not support wide strings. This is a non-lossy conversion.
§ ANSIRepresentation()
ANSIString ANSIRepresentation | ( | void | ) | const |
Converts the string to ANSI, using the current locale, and returns the converted string. Use this, if you need to pass the JString to an API, which does not support Unicode. Attention: This is a lossy conversion, if any characters in the string are not supported by the current locale (which is most likely for characters not common in western languages)!
§ toString()
- Remarks
- The cost of this function depends a lot on implementation details of the implementing subclasses, but for container classes this function can become quite expensive, if the instance contains huge amounts of data, as its cost for many container class implementations increases disproportionately high to the size of the payload.
- Parameters
-
retStr reference to a string, to store the return-value in; the information, which is generated by this function, will be attached at the end of any eventually existing previous content of the string withTypes set to true, to include type information in the generated string
- Returns
- a JString representation of the instance and its contents for debugging purposes.
Implements ToString.
Friends And Related Function Documentation
§ operator<<()
|
related |
operator<<.
Used to print the JString to a std::wostream.
§ operator+() [1/3]
operator+.
Adds its right operand to its left operand and returns the result as a new JString.
§ operator+() [2/3]
operator+.
Adds its right operand to its left operand and returns the result as a new JString.
§ operator==() [1/2]
|
related |
operator==.
- Returns
- true, if both operands are equal, false otherwise.
§ operator==() [2/2]
|
related |
operator==.
- Returns
- true, if both operands are equal, false otherwise.
§ operator!=() [1/2]
|
related |
operator!=.
- Returns
- false, if both operands are equal, true otherwise.
§ operator!=() [2/2]
|
related |
operator!=.
- Returns
- false, if both operands are equal, true otherwise.
§ operator<() [1/2]
|
related |
operator<. The return value indicates the lexicographic relation between the operands.
- Returns
- true, if left operand is less than right operand, false otherwise.
§ operator<() [2/2]
|
related |
operator<. The return value indicates the lexicographic relation between the operands.
- Returns
- true, if left operand is less than right operand, false otherwise.
§ operator>() [1/2]
|
related |
operator>. The return value indicates the lexicographic relation between the operands.
- Returns
- true, if left operand is greater than right operand, false otherwise.
§ operator>() [2/2]
|
related |
operator>. The return value indicates the lexicographic relation between the operands.
- Returns
- true, if left operand is greater than right operand, false otherwise.
§ operator<=() [1/2]
|
related |
operator<=. The return value indicates the lexicographic relation between the operands.
- Returns
- true, if the left operand is less than or equal to the right operand, false otherwise.
§ operator<=() [2/2]
|
related |
operator<=. The return value indicates the lexicographic relation between the operands.
- Returns
- true, if the left operand is less than or equal to the right operand, false otherwise.
§ operator>=() [1/2]
|
related |
operator>=. The return value indicates the lexicographic relation between the operands.
- Returns
- true, if the left operand is greater than or equal to the right operand, false otherwise.
§ operator>=() [2/2]
|
related |
operator>=. The return value indicates the lexicographic relation between the operands.
- Returns
- true, if the left operand is greater than or equal to the right operand, false otherwise.