![]() |
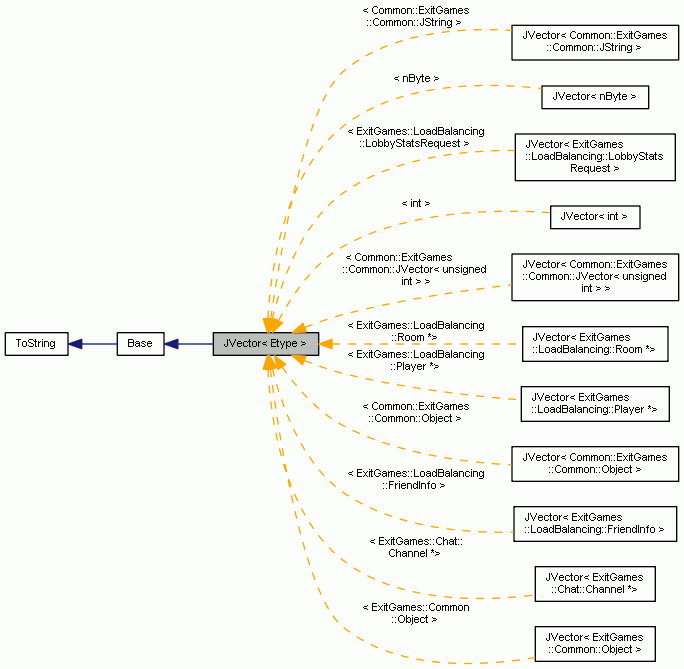
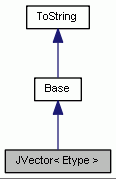
Public Member Functions | |
JVector (unsigned int initialCapacity=0, unsigned int capacityIncrement=1) | |
JVector (const Etype *carray, unsigned int elementCount, unsigned int initialCapacity=0, unsigned int capacityIncrement=1) | |
virtual | ~JVector (void) |
JVector (const JVector< Etype > &rhv) | |
JVector & | operator= (const JVector< Etype > &rhv) |
bool | operator== (const JVector< Etype > &toCompare) const |
bool | operator!= (const JVector< Etype > &toCompare) const |
const Etype & | operator[] (unsigned int index) const |
Etype & | operator[] (unsigned int index) |
unsigned int | getCapacity (void) const |
bool | contains (const Etype &elem) const |
const Etype & | getFirstElement (void) const |
int | getIndexOf (const Etype &elem) const |
bool | getIsEmpty (void) const |
const Etype & | getLastElement (void) const |
int | getLastIndexOf (const Etype &elem) const |
unsigned int | getSize (void) const |
const Etype * | getCArray (void) const |
void | copyInto (Etype *array) const |
void | addElement (const Etype &obj) |
void | addElements (const JVector< Etype > &vector) |
void | addElements (const Etype *carray, unsigned int elementCount) |
void | ensureCapacity (unsigned int minCapacity) |
void | removeAllElements (void) |
bool | removeElement (const Etype &obj) |
void | trimToSize (void) |
const Etype & | getElementAt (unsigned int index) const |
void | insertElementAt (const Etype &obj, unsigned int index) |
void | removeElementAt (unsigned int index) |
void | setElementAt (const Etype &obj, unsigned int index) |
JString & | toString (JString &retStr, bool withTypes=false) const |
![]() | |
virtual | ~Base (void) |
![]() | |
virtual | ~ToString (void) |
virtual JString | typeToString (void) const |
JString | toString (bool withTypes=false) const |
Additional Inherited Members | |
![]() | |
static void | setListener (const BaseListener *baseListener) |
static int | getDebugOutputLevel (void) |
static bool | setDebugOutputLevel (int debugLevel) |
static const LogFormatOptions & | getLogFormatOptions (void) |
static void | setLogFormatOptions (const LogFormatOptions &options) |
Detailed Description
template<typename Etype>
class ExitGames::Common::JVector< Etype >
This is a C++ implementation of the Vector Container class from Sun Java.
This class is based on the Java Vector class and as such contains all the public member functions of its Java equivalent. Unlike Java, typecasts are not necessary since C++ allows template instantiation of types at compile time. In addition to the Java public member functions, some operators were also added in order to take advantage of the operator overloading feature available in C++.
Constructor & Destructor Documentation
§ JVector() [1/3]
JVector | ( | unsigned int | initialCapacity = 0 , |
unsigned int | capacityIncrement = 1 |
||
) |
Constructor.
Creates an empty JVector of elements of the type of the template parameter.
- Parameters
-
initialCapacity the amount of elements, the JVector can take without need for resize. If you choose this too small, the JVector needs expensive resizes later (it's most likely, that the complete memory has to be copied to a new location on resize), if you choose it too big, you will waste much memory. The default is 40. capacityIncrement Every time, when one adds an element to the Vector and it has no capacity left anymore, it's capacity will grow with this amount of elements on automatic resize. If you pass a too small value here, expensive resize will be needed more often, if you choose a too big one, possibly memory is wasted. The default is 10.
§ JVector() [2/3]
JVector | ( | const Etype * | carray, |
unsigned int | elementCount, | ||
unsigned int | initialCapacity = 0 , |
||
unsigned int | capacityIncrement = 1 |
||
) |
Constructor.
Creates a JVector, initialized with the passed carray the template parameter.
- Parameters
-
carray all elements of this array up to elementCount will get copied into the constructed instance elementCount shall not be greater than the actual element count of carray or undefined behavior will occur initialCapacity the amount of elements, the JVector can take without need for resize. Defaults to the value that gets passed for elementCount. If you choose this too small, the JVector needs expensive resizes later (it's most likely, that the complete memory has to be copied to a new location on resize), if you choose it too big, you will waste much memory. capacityIncrement Every time, when one adds an element to the Vector and it has no capacity left anymore, it's capacity will grow with this amount of elements on automatic resize. If you pass a too small value here, expensive resize will be needed more often, if you choose a too big one, possibly memory is wasted. The default is 10.
§ ~JVector()
|
virtual |
Destructor.
§ JVector() [3/3]
Copy-Constructor.
Creates an object out of a deep copy of its parameter.
The parameter has to be of the same template overload as the object, you want to create.
- Parameters
-
toCopy The object to copy.
Member Function Documentation
§ operator=()
operator=.
Makes a deep copy of its right operand into its left operand. Both operands have to be of the same template overload.
This overwrites old data in the left operand.
§ operator==()
bool operator== | ( | const JVector< Etype > & | toCompare | ) | const |
operator==.
- Returns
- true, if both operands are equal, false otherwise. Two instances are treated as equal, if they both contain the the same amount of elements and every element of one instance equals the other instance's element at the same index. If the element type is a pointer type, then the pointers are checked for equality, not the values, to which they point to.
§ operator!=()
bool operator!= | ( | const JVector< Etype > & | toCompare | ) | const |
operator!=.
- Returns
- false, if operator==() would return true, true otherwise.
§ operator[]() [1/2]
const Etype & operator[] | ( | unsigned int | index | ) | const |
operator[]. Wraps the function getElementAt(), so you have the same syntax like for arrays.
§ operator[]() [2/2]
Etype & operator[] | ( | unsigned int | index | ) |
operator[]. Wraps the function getElementAt(), so you have the same syntax like for arrays.
§ getCapacity()
unsigned int getCapacity | ( | void | ) | const |
Returns the current capacity of the JVector.
- Returns
- the current capacity.
§ contains()
bool contains | ( | const Etype & | elem | ) | const |
Checks, if the JVector contains the passed data as an element.
- Parameters
-
elem a reference to the data, you want to check. Needs to be either a primitive type or an object of a class with an overloaded == operator.
- Returns
- true, if the element was found, false otherwise.
§ getFirstElement()
const Etype & getFirstElement | ( | void | ) | const |
Returns the first element of the JVector. Shows undefined behavior for empty vectors.
- Returns
- the first element.
§ getIndexOf()
int getIndexOf | ( | const Etype & | elem | ) | const |
Searches the JVector from the first element in forward direction for the passed element and returns the first index, where it was found.
- Parameters
-
elem the element, to search for.
- Returns
- the index of the first found of the passed element or -1, if the element could not be found at all.
§ getIsEmpty()
bool getIsEmpty | ( | void | ) | const |
§ getLastElement()
const Etype & getLastElement | ( | void | ) | const |
Returns the last element of the JVector. Shows undefined behavior for empty vectors.
- Returns
- the last element.
§ getLastIndexOf()
int getLastIndexOf | ( | const Etype & | elem | ) | const |
Searches the JVector from the last element in backward direction for the passed element and returns the first index, where it was found.
- Parameters
-
elem the element, to search for.
- Returns
- the index of the first found of the passed element or -1, if the element could not be found at all.
§ getSize()
unsigned int getSize | ( | void | ) | const |
Returns the size of the JVector.
- Returns
- the size.
§ getCArray()
const Etype * getCArray | ( | void | ) | const |
- Remarks
- For a deep-copy copyInto() should be used. Use getSize() to find out the element count of the returned array.
- Returns
- a read-only pointer copy of the Etype*, that is internally used to store the elements.
§ copyInto()
void copyInto | ( | Etype * | array | ) | const |
Copies all elements of the JVector into the passed array. The caller has to make sure, that the array is big enough to take all elements of the vector, otherwise calling this function produces a buffer overflow.
- Parameters
-
array an array of variables of the type of the template overload.
- Returns
- nothing.
§ addElement()
void addElement | ( | const Etype & | elem | ) |
Adds an element to the JVector. This automatically resizes the JVectors capacity to it's old size + the capacityIncrement, that you passed, when creating the vector (if you passed no value for capacityIncrement, then it was set to it's default value (see constructor doc)), if the size of the JVector has already reached it's capacity. When resizing occurs, then most likely the whole vector has to be copied to new memory. So this can be an expensive operation for huge vectors.
- Note
- When this function needs to increase the capacity, then all references/pointers to elements, that have been acquired before this function has been called, become invalid!
- Parameters
-
elem the element to add.
- Returns
- nothing.
§ addElements() [1/2]
void addElements | ( | const JVector< Etype > & | vector | ) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. Calls the above function with vector.getCArray() and vector.getSize() as parameters
- Parameters
-
vector the vector from which to copy the elements
§ addElements() [2/2]
void addElements | ( | const Etype * | carray, |
unsigned int | elementCount | ||
) |
Adds the first 'elementCount' elements of the provided array to the JVector. This automatically resizes the JVectors capacity to it's old size + 'elementCount', if the new size of the JVector is bigger than it's old capacity. When resizing occurs, then most likely the whole vector has to be copied to new memory. So this can be an expensive operation for huge vectors.
- Note
- When this function needs to increase the capacity, then all references/pointers to elements, that have been acquired before this function has been called, become invalid!
- Parameters
-
carray the elements to add. elementCount the number of elements to add - must not be greater than the size of carray.
- Returns
- nothing.
§ ensureCapacity()
void ensureCapacity | ( | unsigned int | minCapacity | ) |
Resizes the JVector to the passed capacity, if it's old capacity has been smaller. If resizing is needed, then the whole JVector has to be copied into new memory, so in that case this is an expensive operation for huge JVectors. Call this function, before you add a lot of elements to the vector, to avoid multiple expensive resizes through adding.
- Note
- When this function needs to increase the capacity, then all references/pointers to elements, that have been acquired before this function has been called, will get invalid!
- Parameters
-
minCapacity the new capacity for the JVector.
- Returns
- nothing.
§ removeAllElements()
void removeAllElements | ( | void | ) |
Clears the JVector.
- Returns
- nothing.
§ removeElement()
bool removeElement | ( | const Etype & | obj | ) |
Removes the passed element from the JVector.
- Parameters
-
obj the element, to remove.
- Returns
- true, if the element has been removed, false, if it could not be found.
§ trimToSize()
void trimToSize | ( | void | ) |
Trims the capacity of the JVector to the size, it currently uses. Call this function for a JVector with huge unused capacity, if you do not want to add further elements to it and if you are short on memory. This function copies the whole vector to new memory, so it is expensive for huge vectors. If you only add one element to the JVector later, it's copied again.
- Note
- Trimming a JVector instance (that isn't already optimally trimmed) will make all references/pointers to elements, that have been acquired before this function has been called, invalid!
§ getElementAt()
const Etype & getElementAt | ( | unsigned int | index | ) | const |
Returns the element of the JVector at the passed index. This does not check for valid indexes and shows undefined behavior for invalid indexes!
- Parameters
-
index the index of the element, that should be returned. Must not be bigger than the current size of the vector!
- Returns
- the element at the passed index.
§ insertElementAt()
void insertElementAt | ( | const Etype & | obj, |
unsigned int | index | ||
) |
Inserts parameter one into the JVector at the index, passed as parameter two. Because all elements above or at the passed index have to be moved one position up, it is expensive, to insert an element at an low index into a huge JVector.
- Parameters
-
obj the element, to insert. index the position in the JVector, the element is inserted at.
- Returns
- nothing.
§ removeElementAt()
void removeElementAt | ( | unsigned int | index | ) |
Removes the element at the passed index from the JVector. Shows undefined behavior for invalid indexes.
- Parameters
-
index the index of the element to remove.
- Returns
- nothing.
§ setElementAt()
void setElementAt | ( | const Etype & | obj, |
unsigned int | index | ||
) |
Sets the element at the passed index of the JVector to the passed new value. Shows undefined behavior for invalid indexes.
- Parameters
-
obj the new value. index the index of the element, which is set to the new value.
- Returns
- nothing.
§ toString()
- Remarks
- The cost of this function depends a lot on implementation details of the implementing subclasses, but for container classes this function can become quite expensive, if the instance contains huge amounts of data, as its cost for many container class implementations increases disproportionately high to the size of the payload.
- Parameters
-
retStr reference to a string, to store the return-value in; the information, which is generated by this function, will be attached at the end of any eventually existing previous content of the string withTypes set to true, to include type information in the generated string
- Returns
- a JString representation of the instance and its contents for debugging purposes.
Implements ToString.