![]() |
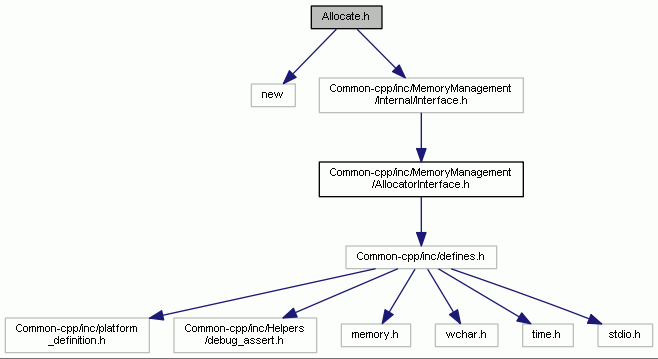
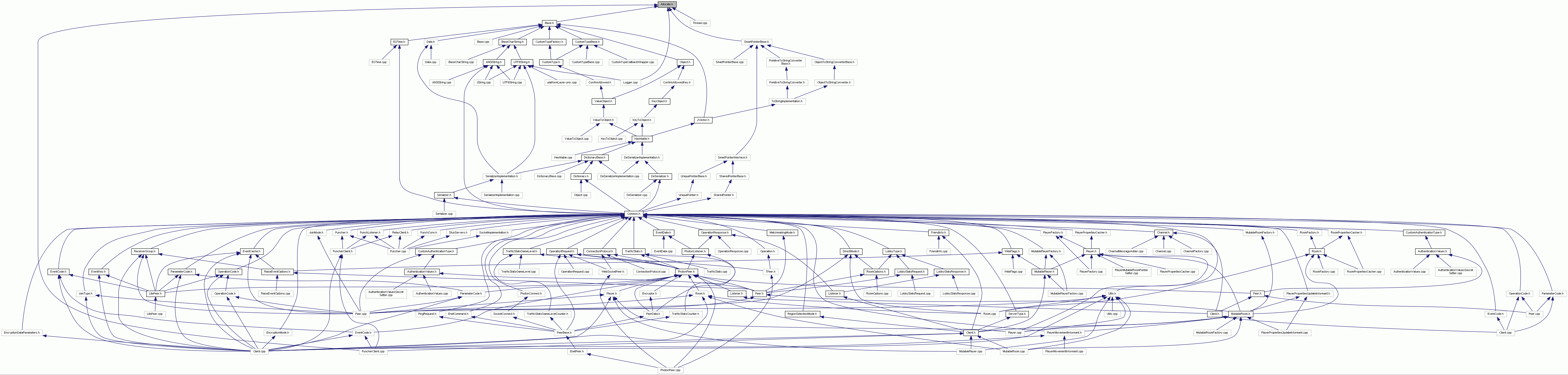
Namespaces | |
ExitGames | |
ExitGames::Common | |
ExitGames::Common::MemoryManagement | |
Macros | |
#define | EG_SIZE_T |
LowLevelMemoryManagement | |
The macros in this section are an alternative for the C dynamic memory management functions malloc(), free(), realloc() and calloc(). They only work in C++, not in C, but same as the standard c library functions they don't call constructors/destructors, but only (de-)allocate the raw memory. This might be desired for high performance container implementations: it makes it possible to allocate storage for many elements at once, while still giving the option to wait with the construction until an element is used. However in most scenarios it makes more sense to use the High Level memory management functions The Memory Management API is optimized for frequent small-sized general purpose dynamic allocations (all dynamic memory allocations that are smaller than several megabytes per allocation and that can happen inside the main loop). The concrete implementation may vary between platforms and configurations, but will usually be a lot faster for this purpose than the standard C functions malloc(), free(), realloc() and calloc() and the standard C++ operators new, new[], delete and delete[]. | |
#define | EG_MALLOC |
#define | EG_FREE |
#define | EG_REALLOC |
#define | EG_CALLOC |
HighLevelMemoryManagement | |
The template functions in this section are an alternative for the C++ dynamic memory management operators new, new[], delete and delete[]. They are implemented in terms of enhancing the Low Level Memory Management macros and for this reason offer similar advantages over new and co like those macros offer over malloc and co. However same as new and co they also construct and destruct the objects that they allocate and deallocate. | |
#define | ALLOCATE(type, p, ...) |
#define | ALLOCATE_ARRAY(type, p, count, ...) |
#define | REALLOCATE_ARRAY(type, p, count, ...) |
#define | DEALLOCATE(type, p) |
#define | DEALLOCATE_ARRAY(type, p) |
void | setMaxAllocSize (size_t maxAllocSize) |
void | setMaxSizeForAllocatorUsage (size_t maxSizeForAllocatorUsage) |
void | setAllocator (ExitGames::Common::MemoryManagement::AllocatorInterface &allocator) |
void | setAllocatorToDefault (void) |
template<typename Ftype > | |
Ftype * | allocate (void) |
template<typename Ftype > | |
Ftype * | allocateArray (size_t count) |
template<typename Ftype > | |
Ftype * | reallocateArray (Ftype *p, size_t count) |
template<typename Ftype > | |
void | deallocate (const Ftype *p) |
template<typename Ftype > | |
void | deallocateArray (const Ftype *p) |
Macro Definition Documentation
§ EG_MALLOC
#define EG_MALLOC |
This macro allocates the requested amount of bytes as a single continuous block from dynamic memory and returns the address of the first byte of that block.
Blocks of memory that have been allocated with EG_MALLOC(), have to be deallocated with EG_FREE(), when they are no longer needed.
If the requested amount of bytes is 0, then this macro will do nothing and return a NULL pointer.
§ EG_FREE
#define EG_FREE |
Pass the address of memory, that has previously been returned by EG_MALLOC(), EG_REALLOC() or EG_CALLOC() to this function, to deallocate it.
If the passed address is NULL, then this macro will do nothing.
If a passed non-NULL address was not previously returned by EG_MALLOC(), EG_REALLOC() or EG_CALLOC(), then the behavior is undefined.
§ EG_REALLOC
#define EG_REALLOC |
This macro resizes the block of memory at the passed address to the passed size and returns the new address of this block of memory.
The returned address isn't guaranteed to match the passed one. Depending on the old and new size of the memory block, resizing the block may include moving it to a new location. When a block gets moved, is an implementation detail, that could be different between implementations on different platforms and can change without notice. Notably block-movements might happen in the case of an increase as well as of a decrease of the block size.
If a block of memory gets moved to a new location, then the content of all bytes that fit in both, the old and the new block size, is copied from the old to the new location by a call to memcpy(). For this reason calls to EG_REALLOC() can be expensive for huge blocks of memory.
If the new block size is smaller than the old one, then all content at the surplus bytes will get lost.
If the passed address is NULL, then this macro will behave just like EG_MALLOC().
If a passed non-NULL address was not previously returned by EG_MALLOC(), EG_REALLOC() or EG_CALLOC(), then the behavior is undefined.
§ EG_CALLOC
#define EG_CALLOC |
This macro allocates memory for the requested amount of array elements of the specified element size as a single continuous block from dynamic memory, initializes all its bytes to 0 and returns the address of the first byte of that block.
Blocks of memory that have been allocated with EG_CALLOC(), have to be deallocated with EG_FREE(), when they are no longer needed.
If the requested amount of bytes is 0, then this macro will do nothing and return a NULL pointer.
§ ALLOCATE
#define ALLOCATE | ( | type, | |
p, | |||
... | |||
) |
This is the macro version of the allocate() template function.
Normally the template version should be preferred, but using the macro instead can be needed, if you want to pass more than 10 parameters to the constructor or if you want to call a private or protected constructor to which your class has (friend-/subclass-)access.
- Parameters
-
type the data type of the instance to create p a pointer, in which the macro will store the address of the freshly created instance ... optional arguments to pass to the constructor
§ ALLOCATE_ARRAY
#define ALLOCATE_ARRAY | ( | type, | |
p, | |||
count, | |||
... | |||
) |
This is the macro version of the allocateArray() template function.
Normally the template version should be preferred, but using the macro instead can be needed, if you want to pass more than 10 parameters to the constructor or if you want to call a private or protected constructor to which your class has (friend-/subclass-)access.
- Parameters
-
type the data type of the instance to create p a pointer, in which the macro will store the address of the freshly created instance count the number of the elements to create ... optional arguments to pass to the constructor
§ REALLOCATE_ARRAY
#define REALLOCATE_ARRAY | ( | type, | |
p, | |||
count, | |||
... | |||
) |
This is the macro version of the reallocateArray() template function.
Normally the template version should be preferred, but using the macro instead can be needed, if you want to pass more than 10 parameters to the constructor or if you want to call a private or protected constructor to which your class has (friend-/subclass-)access.
- Parameters
-
type the data type of the instance to create p a pointer, in which the macro will store the address of the freshly created instance count the number of the elements to create ... optional arguments to pass to the constructor
§ DEALLOCATE
#define DEALLOCATE | ( | type, | |
p | |||
) |
This is the macro version of the deallocate() template function.
Normally the template version should be preferred, but using the macro instead can make sense for consistency reasons when the macro version has been used for allocation.
- Parameters
-
type the data type of the instance, to which p points p a pointer to the instance to destroy
§ DEALLOCATE_ARRAY
#define DEALLOCATE_ARRAY | ( | type, | |
p | |||
) |
This is the macro version of the deallocateArray() template function.
Normally the template version should be preferred, but using the macro instead can make sense for consistency reasons when the macro version has been used for allocation.
- Parameters
-
type the data type of the instance, to which p points p a pointer to the instance to destroy