![]() |
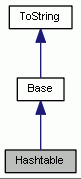
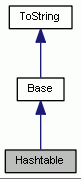
Public Member Functions | |
Hashtable (void) | |
~Hashtable (void) | |
Hashtable (const Hashtable &toCopy) | |
Hashtable & | operator= (const Hashtable &toCopy) |
bool | operator== (const Hashtable &toCompare) const |
bool | operator!= (const Hashtable &toCompare) const |
const Object & | operator[] (unsigned int index) const |
Object & | operator[] (unsigned int index) |
void | put (const Hashtable &src) |
template<typename FKeyType , typename FValueType > | |
void | put (const FKeyType &key, const FValueType &val) |
template<typename FKeyType > | |
void | put (const FKeyType &key) |
template<typename FKeyType , typename FValueType > | |
void | put (const FKeyType &key, const FValueType pVal, typename Common::Helpers::ArrayLengthType< FValueType >::type size) |
template<typename FKeyType , typename FValueType > | |
void | put (const FKeyType &key, const FValueType pVal, const short *sizes) |
template<typename FKeyType > | |
const Object * | getValue (const FKeyType &key) const |
unsigned int | getSize (void) const |
const JVector< Object > & | getKeys (void) const |
template<typename FKeyType > | |
void | remove (const FKeyType &key) |
template<typename FKeyType > | |
bool | contains (const FKeyType &key) const |
void | removeAllElements (void) |
JString & | toString (JString &retStr, bool withTypes=false) const |
![]() | |
virtual | ~Base (void) |
![]() | |
virtual | ~ToString (void) |
virtual JString | typeToString (void) const |
JString | toString (bool withTypes=false) const |
Additional Inherited Members | |
![]() | |
static void | setListener (const BaseListener *baseListener) |
static int | getDebugOutputLevel (void) |
static bool | setDebugOutputLevel (int debugLevel) |
static const LogFormatOptions & | getLogFormatOptions (void) |
static void | setLogFormatOptions (const LogFormatOptions &options) |
Detailed Description
The Hashtable class together with the Dictionary class template is one of the two main container classes for objects to be transmitted over Photon when using the C++ Client.
This class implements the well-known concept of a container structure storing an arbitrary number of key/value-pairs.
In contrast to a Dictionary, the types of both the keys and also the values in a Hashtable can differ for every entry. This adds flexibility, but it also reduces type safety and means, that the type infos have to be stored twice (once for the key and once for the value) per entry in a Hashtable, while in a Dictionary it only has to be stored twice for the whole Dictionary, no matter how many entries are in there. Therefor with Dictionaries transferring the same amount of key-value pairs will cause less traffic than with Hashtables.
Please have a look at the Table of Datatypes for a list of types, that are supported as keys and as values.
Please refer to the documentation for put() and getValue() to see how to store and access data in a Hashtable.
- See also
- put(), getValue(), KeyObject, ValueObject, Dictionary
Constructor & Destructor Documentation
§ Hashtable() [1/2]
Hashtable | ( | void | ) |
Constructor: Creates an empty instance.
§ ~Hashtable()
~Hashtable | ( | void | ) |
Destructor.
§ Hashtable() [2/2]
Copy-Constructor: Creates a deep copy of the argument.
- Parameters
-
toCopy The object to copy.
Member Function Documentation
§ operator=()
operator=. Makes a deep copy of its right operand into its left operand. This overwrites old data in the left operand.
§ operator==()
bool operator== | ( | const Hashtable & | toCompare | ) | const |
operator==.
- Returns
- true, if both operands are equal, false otherwise.
Two instances are considered equal if they each hold the same number of entries and, for a given key, the corresponding values equal each other.
Two values are considered equal to each other, if instances of class Object, that are holding them as payloads, equal each other.
- See also
- Object::operator==()
§ operator!=()
bool operator!= | ( | const Hashtable & | toCompare | ) | const |
operator!=.
- Returns
- false, if operator==() would return true, true otherwise.
§ operator[]() [1/2]
const Object & operator[] | ( | unsigned int | index | ) | const |
operator[].
Accesses the value at the given index like in an array. This does not check for valid indexes and shows undefined behavior for invalid indexes
§ operator[]() [2/2]
Object & operator[] | ( | unsigned int | index | ) |
operator[].
Accesses the value at the given index like in an array. This does not check for valid indexes and shows undefined behavior for invalid indexes
§ put() [1/5]
void put | ( | const Hashtable & | src | ) |
Adds all pairs of a key and a corresponding value from the passed instance to the instance, on which it is called on. If a key is already existing, then its old value will be replaced with the new one.
- Parameters
-
src instance, from which to add the content
- Returns
- nothing.
§ put() [2/5]
void put | ( | const FKeyType & | key, |
const FValueType & | val | ||
) |
Adds a pair of a key and a corresponding value to the instance.
If the key is already existing, then it's old value will be replaced with the new one. Please have a look at the table of datatypes for a list of supported types for keys and values
- Parameters
-
key the key to add val the value to add
- Returns
- nothing.
§ put() [3/5]
void put | ( | const FKeyType & | key | ) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
This overload adds an empty object as value for the provided key.
§ put() [4/5]
void put | ( | const FKeyType & | key, |
const FValueType | pVal, | ||
typename Common::Helpers::ArrayLengthType< FValueType >::type | size | ||
) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
This overload accepts singledimensional arrays and NULL-pointers passed for parameter pVal. NULL pointers are only legal input, if size is 0
- Parameters
-
key the key to add pVal the value array to add size the size of the value array
§ put() [5/5]
void put | ( | const FKeyType & | key, |
const FValueType | pVal, | ||
const short * | sizes | ||
) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
This overload accepts multidimensional arrays and NULL-pointers passed for parameter pVal. The array that is passed for parameter pVal has to be a pointer of the correct abstraction level, meaning a normal pointer for a singledimensional array, a doublepointer for a twodimensional array, a triplepointer for a threedimensional array and so on. For pVal NULL pointers are only legal input, if sizes[0] is 0. For sizes NULL is no valid input.
- Parameters
-
key the key to add pVal the value array to add sizes the sizes for every dimension of the value array - the length of this array has to match the dimensions of pVal
§ getValue()
const Object * getValue | ( | const FKeyType & | key | ) | const |
§ getSize()
unsigned int getSize | ( | void | ) | const |
- Returns
- the number of key/value pairs stored in the Hashtable.
§ getKeys()
§ remove()
void remove | ( | const FKeyType & | key | ) |
Deletes the specified key and the corresponding value, if found in the Hashtable.
- Parameters
-
key Pointer to the key of the key/value-pair to remove.
- Returns
- nothing.
- See also
- removeAllElements()
§ contains()
bool contains | ( | const FKeyType & | key | ) | const |
Checks, whether the Hashtable contains a certain key.
- Parameters
-
key Pointer to the key to look up.
- Returns
- true if the specified key was found, false otherwise.
§ removeAllElements()
void removeAllElements | ( | void | ) |
§ toString()
- Remarks
- The cost of this function depends a lot on implementation details of the implementing subclasses, but for container classes this function can become quite expensive, if the instance contains huge amounts of data, as its cost for many container class implementations increases disproportionately high to the size of the payload.
- Parameters
-
retStr reference to a string, to store the return-value in; the information, which is generated by this function, will be attached at the end of any eventually existing previous content of the string withTypes set to true, to include type information in the generated string
- Returns
- a JString representation of the instance and its contents for debugging purposes.
Implements ToString.