![]() |
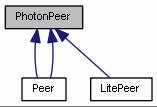
Public Member Functions | |
PhotonPeer (PhotonListener &listener, nByte connectionProtocol=ConnectionProtocol::DEFAULT) | |
virtual | ~PhotonPeer (void) |
virtual bool | connect (const Common::JString &ipAddr, const Common::JString &appID=Common::JString()) |
template<typename Ftype > | |
bool | connect (const Common::JString &ipAddr, const Common::JString &appID, const Ftype &customData) |
template<typename Ftype > | |
bool | connect (const Common::JString &ipAddr, const Common::JString &appID, const Ftype pCustomDataArray, typename Common::Helpers::ArrayLengthType< Ftype >::type arrSize) |
template<typename Ftype > | |
bool | connect (const Common::JString &ipAddr, const Common::JString &appID, const Ftype pCustomDataArray, const short *pArrSizes) |
virtual void | disconnect (void) |
virtual void | service (bool dispatchIncomingCommands=true) |
virtual void | serviceBasic (void) |
virtual bool | opCustom (const OperationRequest &operationRequest, bool sendReliable, nByte channelID=0, bool encrypt=false) |
virtual bool | sendOutgoingCommands (void) |
virtual bool | sendAcksOnly (void) |
virtual bool | dispatchIncomingCommands (void) |
virtual bool | establishEncryption (void) |
virtual void | fetchServerTimestamp (void) |
virtual void | resetTrafficStats (void) |
virtual void | resetTrafficStatsMaximumCounters (void) |
virtual Common::JString | vitalStatsToString (bool all) const |
virtual void | pingServer (const Common::JString &address, unsigned int pingAttempts) |
virtual void | initUserDataEncryption (const Common::JVector< nByte > &secret) |
virtual void | initUDPEncryption (const Common::JVector< nByte > &encryptSecret, const Common::JVector< nByte > &HMACSecret) |
PhotonListener * | getListener (void) |
int | getServerTimeOffset (void) const |
int | getServerTime (void) const |
int | getBytesOut (void) const |
int | getBytesIn (void) const |
int | getByteCountCurrentDispatch (void) const |
int | getByteCountLastOperation (void) const |
int | getPeerState (void) const |
int | getSentCountAllowance (void) const |
void | setSentCountAllowance (int sentCountAllowance) |
int | getTimePingInterval (void) const |
void | setTimePingInterval (int timePingInterval) |
int | getRoundTripTime (void) const |
int | getRoundTripTimeVariance (void) const |
int | getTimestampOfLastSocketReceive (void) const |
int | getDebugOutputLevel (void) const |
bool | setDebugOutputLevel (int debugLevel) |
const Common::LogFormatOptions & | getLogFormatOptions (void) const |
void | setLogFormatOptions (const Common::LogFormatOptions &formatOptions) |
int | getIncomingReliableCommandsCount (void) const |
short | getPeerID (void) const |
int | getDisconnectTimeout (void) const |
void | setDisconnectTimeout (int disconnectTimeout) |
int | getQueuedIncomingCommands (void) const |
int | getQueuedOutgoingCommands (void) const |
Common::JString | getServerAddress (void) const |
bool | getIsPayloadEncryptionAvailable (void) const |
bool | getIsEncryptionAvailable (void) const |
int | getResentReliableCommands (void) const |
int | getLimitOfUnreliableCommands (void) const |
void | setLimitOfUnreliableCommands (int value) |
bool | getCRCEnabled (void) const |
void | setCRCEnabled (bool crcEnabled) |
int | getPacketLossByCRC (void) const |
bool | getTrafficStatsEnabled (void) const |
void | setTrafficStatsEnabled (bool trafficStasEnabled) |
int | getTrafficStatsElapsedMs (void) const |
const TrafficStats & | getTrafficStatsIncoming (void) const |
const TrafficStats & | getTrafficStatsOutgoing (void) const |
const TrafficStatsGameLevel & | getTrafficStatsGameLevel (void) const |
nByte | getQuickResendAttempts (void) const |
void | setQuickResendAttempts (nByte quickResendAttempts) |
nByte | getConnectionProtocol (void) const |
void | setConnectionProtocol (nByte connectionProtocol) |
nByte | getChannelCountUserChannels (void) const |
Static Public Member Functions | |
static short | getPeerCount (void) |
static unsigned int | getMaxAppIDLength (void) |
Detailed Description
The PhotonPeer class provides an API for reliable and unreliable realtime communication.
PhotonPeer uses the callback interface PhotonListener that needs to be implemented by your application, to receive results and events from the Photon Server.
Constructor & Destructor Documentation
§ PhotonPeer()
PhotonPeer | ( | PhotonListener & | listener, |
nByte | connectionProtocol = ConnectionProtocol::DEFAULT |
||
) |
Constructor.
- Parameters
-
listener Reference to the application's implementation of the Listener callback interface. Has to be valid for at least the lifetime of the PhotonPeer instance, which is created by this constructor. connectionProtocol The protocol to use to connect to Photon. Must match one of the constants specified in ConnectionProtocol.
- See also
- PhotonListener, ConnectionProtocol
§ ~PhotonPeer()
|
virtual |
Destructor.
Member Function Documentation
§ connect() [1/4]
|
virtual |
This function starts establishing a connection to a Photon server. The servers response will arrive in PhotonListener::onStatusChanged().
The connection is successfully established when the Photon client received a valid response from the server. The connect-attempt fails when a network error occurs or when server is not responding. A call to this function starts an asynchronous operation. The result of this operation gets returned through the PhotonListener::onStatusChanged() callback function.
- Parameters
-
ipAddr A null terminated string containing the IP address or domain name and optionally the port number to connect to. IP addresses can be in IPv4 or IPv6 format, examples: "192.168.0.1", "192.168.0.1:5055", "udp.gameserver.com", "udp.gameserver.com:5055", "[2002:C0A8:1::]", "[2002:C0A8:1::]:5055". Note that IPv6 addresses must include square brackets to indicate where the address itself end and the port begins. If no port is given, then the default port for the chosen protocol and server type will be used. appID the appID (default: an empty string)
- Returns
- true, if it could successfully start establishing a connection (the result will be passed in the callback function in this case) or false, if an error occurred and the connection could not be established (the callback function will not be called then).
- See also
- disconnect(), NetworkPort
§ connect() [2/4]
bool connect | ( | const Common::JString & | ipAddr, |
const Common::JString & | appID, | ||
const Ftype & | customData | ||
) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
- Parameters
-
ipAddr Null terminated string containing IP address or domain name and optionally a port of server to connect. Should be in usual format\: "address[\:port]", for example\: "192.168.0.1\:5055" or "udp.gameserver.com". If no port is given, port 5055 will be used by default. appID the appID (default\: an empty string) customData custom data to send to the server when initializing the connection - has to be provided in the form of one of the supported data types, specified at Table of Datatypes
§ connect() [3/4]
bool connect | ( | const Common::JString & | ipAddr, |
const Common::JString & | appID, | ||
const Ftype | pCustomDataArray, | ||
typename Common::Helpers::ArrayLengthType< Ftype >::type | arrSize | ||
) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
This overload accepts singledimensional arrays and NULL-pointers passed for parameter pCustomDataArray. NULL pointers are only legal input, if arrSize is 0
- Parameters
-
ipAddr Null terminated string containing IP address or domain name and optionally a port of server to connect. Should be in usual format\: "address[\:port]", for example\: "192.168.0.1\:5055" or "udp.gameserver.com". If no port is given, port 5055 will be used by default. appID the appID (default\: an empty string) pCustomDataArray custom data to send to the server when initializing the connection - has to be provided in the form of a 1D array of one of the supported data types, specified at Table of Datatypes arrSize the element count of the customData array
§ connect() [4/4]
bool connect | ( | const Common::JString & | ipAddr, |
const Common::JString & | appID, | ||
const Ftype | pCustomDataArray, | ||
const short * | pArrSizes | ||
) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
This overload accepts multidimensional arrays and NULL-pointers passed for parameter pCustomDataArray. The array that is passed for parameter pCustomDataArray has to be a pointer of the correct abstraction level, meaning a normal pointer for a singledimensional array, a doublepointer for a twodimensional array, a triplepointer for a threedimensional array and so on. For pCustomDataArray NULL pointers are only legal input, if pArrSizes[0] is 0. For pArrSizes NULL is no valid input.
- Parameters
-
ipAddr Null terminated string containing IP address or domain name and optionally a port of server to connect. Should be in usual format\: "address[\:port]", for example\: "192.168.0.1\:5055" or "udp.gameserver.com". If no port is given, port 5055 will be used by default. appID the appID (default\: an empty string) pCustomDataArray custom data to send to the server when initializing the connection - has to be provided in the form of an array of one of the supported data types, specified at Table of Datatypes pArrSizes the element counts for every dimension of the custom data array - the element count of this array has to match the dimensions of the custom data array
§ disconnect()
|
virtual |
Initiates the disconnection from the Photon server. The servers response will arrive in PhotonListener::onStatusChanged().
This function generates a disconnection request that will be sent to the Photon server. If the disconnection is completed successfully, then the PhotonListener::onStatusChanged() callback will be called, with a statusCode of StatusCode::DISCONNECT.
- See also
- connect()
Reimplemented in Peer.
§ service()
|
virtual |
This function executes the PhotonPeer internal processes. Call this regularly!
This function is meant to be called frequently, like once per game loop. It handles the internal calls for keeping the PhotonPeer communication alive, and will take care of sending all local outgoing acknowledgements and messages, as well as dispatching incoming messages to the application and firing the corresponding callbacks. Internally service() calls the following functions:
- serviceBasic()
- dispatchIncomingCommands() (called withing a loop until all incoming commands have been dispatched.)
- sendOutgoingCommands() (called withing a loop until everything queued for sending has been sent.)
service() is provided for convenience. If you need to tweak the performance, you can ignore service() and call its three subfunctions directly with individual time intervals, to gain more control over the internal communication process. For instance, calling sendOutgoingCommands() more rarely will result in less packets to be generated, as more commands will be accumulated into a single packet. See sendOutgoingCommands() for more information on efficiency.
For situations where you want to keep the connection alive, but can't process incoming messages (e.g. when loading a level), you can temporarily pass false for dispatchIncomingCommands to skip the calls to dispatchIncomingCommands(). Incoming commands will be stored in the incoming queue until they are dispatched again.
- Parameters
-
dispatchIncomingCommands true = dispatchIncomingCommands() will be called; false = dispatchIncomingCommands() won't be called, default is true
§ serviceBasic()
|
virtual |
This function takes care of exchanging data with the system's network layer.
You only need to call this function in case you choose not to use service(), but call the subfunctions of service() directly. Please see the documentation of service() for more information.
serviceBasic() is called from within service(). If you decide not to use service(), then serviceBasic() needs to be called frequently, like once per game loop.
- See also
- service()
§ opCustom()
|
virtual |
Sends a custom operation to a custom Server, using reliable or unreliable Photon transmission.
Allows the client to send a custom operation to the Photon server (which has to be modified accordingly). The Server can be extended and modified for special purposes like server side collision detection or a consistent world.
You need to be connected (see connect()) prior to calling opCustom().
- Parameters
-
operationRequest holds the payload of the operation sendReliable = operation will be sent reliably; false = no resend in case of packet loss - will be ignored, when not using udp as protocol channelID the logical channel, default is 0. See Fragmentation and Channels for more information. encrypt true = encrypt message; false = no encryption
- Returns
- true, if successful, false otherwise
§ sendOutgoingCommands()
|
virtual |
This function initiates the transmission of outgoing commands.
Any Photon function that generates messages will store these messages as a "command" in an outgoing queue for later transmission. Commands can either be explicitly created operations generated for example by opCustom() or internally generated messages like acknowledgements for reliable messages from other players. sendOutgoingCommands() will initiate the data transmission by passing the outgoing commands to the system's sockets for immediate transmission.
In case of UDP sendOutgoingCommands() will also split the commands into multiple packets if needed and/of aggregate multiple commands together into one packet, if possible. Because of the latter calling sendOutgoingcommands() more rarely will result in less overhead, as there will be fewer packets for the clients to be sent and processed. The underlying platform can also limit the frequency in which outgoing packets can be sent and received. The downside of lower sending frequencies is a higher latency, until messages are exchanged and acknowledged, which may lead to a jerky gameplay.
To help you keeping track of the incoming and outgoing queues at development time and adjust your sending frequency, there will be a warning message sent to your debugReturn callback if a queue has exceeded the warning threshold.
- Note
- While service() is calling serviceBasic() implicitly, you will have to regularly call it yourself explictly , when you use sendOutgoingCommands() and dispatchIncomingCommands() directly instead.
Usually you don't have to call sendOutgoingCommands() this explicitly, as this is done within service().
- See also
- service()
§ sendAcksOnly()
|
virtual |
Sends only ACKs (UDP) or Ping (TCP) instead of queued outgoing commands. Useful to pause sending actual data.
- Note
- While service() is calling serviceBasic() implicitly, you will have to regularly call it yourself explictly , when you use sendAcksOnly() and dispatchIncomingCommands() instead.
§ dispatchIncomingCommands()
|
virtual |
Checks for incoming commands waiting in the queue, and dispatches a single command to the application.
Dispatching means, that if the command is an operation response or an event, the appropriate callback function will be called). dispatchIncomingCommands() will also take care of generating and queuing acknowledgments for incoming reliable commands. Please note that this function will only dispatch one command per all. If you want to dispatch every single command which is waiting in the queue, call dipatchIncomingCommands() within a while loop, until its return code is false.
- Note
- While service() is calling serviceBasic() implicitly, you will have to regularly call it yourself explictly, when you use sendOutgoingCommands() and dispatchIncomingCommands() directly instead.
- Returns
- true if it has successfully dispatched a command, false otherwise (for example, when there has not been any command left in the queue, waiting for dispatching).
- See also
- service()
§ establishEncryption()
|
virtual |
This function creates a public key for this client and exchanges it with the server.
If establishEncryption() returns true, then Photon will inform you about the successfull establishment or a failure by calling PhotonListener::onStatusChanged() with the statusCode beeing either StatusCode::ENCRYPTION_ESTABLISHED or StatusCode::ENCRYPTION_FAILED_TO_ESTABLISH
- Returns
- true if encryption has been successfully initiated, false otherwise.
§ fetchServerTimestamp()
|
virtual |
This will fetch the server's timestamp and update the approximation for getServerTime() and getServerTimeOffset().
The server time approximation will NOT become more accurate by repeated calls. Accuracy currently depends on a single roundtrip which is done as fast as possible.
The command used for this is immediately acknowledged by the server. This makes sure the roundtriptime is low and the timestamp + roundtriptime / 2 is close to the original value.
§ resetTrafficStats()
|
virtual |
Creates new instances of TrafficStats and starts a new timer for those.
§ resetTrafficStatsMaximumCounters()
|
virtual |
Resets traffic stats values that can be maxed out.
§ vitalStatsToString()
|
virtual |
Returns a string of the most interesting connection statistics. When you have issues on the client side, these might contain hints about the issue's cause.
- Parameters
-
all If true, Incoming and Outgoing low-level stats are included in the string.
- Returns
- stats as a string.
§ pingServer()
|
virtual |
Sends a ping signal to the specified address.
Each call to this function results in a number of calls to PhotonListener::onPingResponse() that equals the value which has been passed for parameter pingAttempts.
This function can be used to ping multiple Photon servers and determine the one with the lowest latency.
As the latency of the same server may vary it can make sense to send multiple ping attempts. In that case the next attempt gets sent when either the servers response for the previous attempt has been received or when that previous attempt has timed out.
Multiple calls to this function do not get queued, but run in parallel.
A valid Photon server must run at the specified address.
- Note
- This function is not available on platforms that do not support those parts of the stdlib that have been introduced with C++ 11.
- This function is not available on platforms that do not support multi-threading.
- Parameters
-
address the address, which should be pinged pingAttempts the amount of ping signals to send
- See also
- PhotonListener::onPingResponse()
§ initUserDataEncryption()
|
virtual |
Initializes userData encryption with the provided key.
- Note
- You must also provide the same key to the server to which you want to connect. It needs to be an aes256 key and must not have been received through an unsecured connection.
- Remarks
- If you don't already have generated a key that you can access securely on both, the client and the server, you may want to consider to use establishEncryption() instead, which also initializes userData encryption, but does generate suitable keys on client and server side itself.
- Parameters
-
secret an aes256 key
§ initUDPEncryption()
|
virtual |
Initializes UDP packet Data encryption with the provided keys.
This function has no effect for non-UDP connections, but you may still call it while having an active connection that uses a different protocol. In that case they keys will be stored in case that you switch the protocol at the time of a later re-connect. For XB1 UDP connections UDP packet encryption is a mandatory requirement by Microsoft. On other platforms you may also consider to use establishEncryption() or initUserDataEncryption(), which provide alternative encryption implementations that do also work with other connection protocols.
- Note
- You must also provide the same keys to the server to which you want to connect. They need to be aes256 keys and must not have been received through an unsecured connection.
- This function is only available on Windows Desktop, Windows Store and XBox 1.
- Parameters
-
encryptSecret an aes256 key used for packet encryption HMACSecret an aes256 key used for packet authentication
§ getListener()
PhotonListener * getListener | ( | void | ) |
- Returns
- a pointer to the application's implementation of the Listener callback interface, as passed to the constructor of PhotonPeer.
§ getServerTimeOffset()
int getServerTimeOffset | ( | void | ) | const |
- Returns
- the difference between the local uptime and the Photon Server's system time in ms.
In real-time games it's often useful to relate game events to a global common timeline, that's valid for all players and independent from derivations throughout the clients' system times. The Photon Server's System Time can serve as this reference time. The serverTimeOffset represents the difference between the client's local system time and the Photon server's system time.
ServerTime = serverTimeOffset + GETTIMEMS()
The serverTimeOffset is fetched shortly after connect by Photon. Use GETTIMEMS() to get your local time in ms. You can let Photon refetch the offset by calling fetchServerTimestamp(). The ServerTimeOffset will be 0 until shortly after initial connect.
§ getServerTime()
int getServerTime | ( | void | ) | const |
- Returns
- the Photon Server's system time ins ms.
§ getBytesOut()
int getBytesOut | ( | void | ) | const |
- Returns
- the total number of outgoing bytes transmitted by this PhotonPeer object.
- See also
- getBytesIn()
§ getBytesIn()
int getBytesIn | ( | void | ) | const |
- Returns
- the total number of incoming bytes received by this PhotonPeer object.
- See also
- getBytesOut()
§ getByteCountCurrentDispatch()
int getByteCountCurrentDispatch | ( | void | ) | const |
- Returns
- the size of the dispatched event or operation-result in bytes. This value is set before onEvent() or onOperationResponse() is called (within dispatchIncomingCommands()). Get this value directly in onEvent() or onOperationResponse().
§ getByteCountLastOperation()
int getByteCountLastOperation | ( | void | ) | const |
- Returns
- the size of the last serialized operation call in bytes. The value includes all headers for this single operation but excludes those of UDP, Enet Package Headers and TCP. Get this value immediately after calling an operation.
§ getPeerState()
int getPeerState | ( | void | ) | const |
- Returns
- the current state of the PhotonPeer object
The state of the PhotonPeer object is changed internally upon connection and disconnection, and will be one of the values of the PeerState enum.
- See also
- connect(), disconnect()
§ getSentCountAllowance()
int getSentCountAllowance | ( | void | ) | const |
- Returns
- the number of resend retries before a peer is considered lost/disconnected.
This is udp specific and will always return 0 for other protocols.
§ setSentCountAllowance()
void setSentCountAllowance | ( | int | sentCountAllowance | ) |
Sets the number of re-send retries before a peer is considered lost/disconnected.
This is udp specific and will do nothing at all for other protocols.
- Parameters
-
sentCountAllowance the new number of re/-send retries before a peer is considered lost/disconnected.
§ getTimePingInterval()
int getTimePingInterval | ( | void | ) | const |
- Returns
- the time threshold in milliseconds since the last reliable command, before a ping will be sent.
- See also
- setTimePingInterval()
§ setTimePingInterval()
void setTimePingInterval | ( | int | timePingInterval | ) |
Sets the time threshold in milliseconds since the last reliable command, before a ping will be sent.
- Parameters
-
timePingInterval time threshold in milliseconds since the last reliable command, before a ping will be sent.
- See also
- getTimePingInterval()
§ getRoundTripTime()
int getRoundTripTime | ( | void | ) | const |
- Returns
- the time in milliseconds until a reliable command is acknowledged by the server.
This is, what is commonly called a ping time or just a ping.
- See also
- getRoundTripTimeVariance()
§ getRoundTripTimeVariance()
int getRoundTripTimeVariance | ( | void | ) | const |
- Returns
- the variance of the roundtrip time in milliseconds. Gives a hint about how much the net latency is varying.
- See also
- getRoundTripTime()
§ getTimestampOfLastSocketReceive()
int getTimestampOfLastSocketReceive | ( | void | ) | const |
- Returns
- timestamp of the last time anything (!) was received from the server (including low level Ping and ACKs but also events and operation-returns). This is not the time when something was dispatched.
§ getDebugOutputLevel()
int getDebugOutputLevel | ( | void | ) | const |
Returns the current level of debug information that's passed on to BaseListener::debugReturn().
- Returns
- one of the values in DebugLevel
- See also
- setDebugOutputLevel()
§ setDebugOutputLevel()
bool setDebugOutputLevel | ( | int | debugLevel | ) |
Sets the current level of debug information that's passed on to BaseListener::debugReturn().
- Parameters
-
debugLevel one of the values in DebugLevel
- Returns
- true if the new debug level has been set correctly, false otherwise.
- See also
- getDebugOutputLevel()
§ getLogFormatOptions()
const LogFormatOptions & getLogFormatOptions | ( | void | ) | const |
- Returns
- the LogFormatOptions that are used by this instance.
- See also
- setFormatOptions()
§ setLogFormatOptions()
void setLogFormatOptions | ( | const Common::LogFormatOptions & | formatOptions | ) |
Sets the log format options to the supplied value.
- Parameters
-
formatOptions the new value to which the log format options will be set
- See also
- getFormatOptions()
§ getIncomingReliableCommandsCount()
int getIncomingReliableCommandsCount | ( | void | ) | const |
- Returns
- the total number of reliable commands currently waiting in the incoming queues of all channels or -1 if not connected.
§ getPeerID()
short getPeerID | ( | void | ) | const |
- Returns
- this peer's ID as assigned by the server. Will be -1, if not connected.
§ getDisconnectTimeout()
int getDisconnectTimeout | ( | void | ) | const |
- Returns
- the maximum time interval in milliseconds for doing resend retries before a peer is considered lost/disconnected.
§ setDisconnectTimeout()
void setDisconnectTimeout | ( | int | disconnectTimeout | ) |
Sets the maximum time ins milliseconds for making re-send retries before a peer is considered lost/disconnected.
- Parameters
-
disconnectTimeout resend max time in ms before a peer is considered lost/disconnected
§ getQueuedIncomingCommands()
int getQueuedIncomingCommands | ( | void | ) | const |
- Returns
- the number of queued incoming commands in all channels or -1 if not connected
§ getQueuedOutgoingCommands()
int getQueuedOutgoingCommands | ( | void | ) | const |
- Returns
- the number of queued outgoing commands in all channels or -1 if not connected
§ getServerAddress()
JString getServerAddress | ( | void | ) | const |
- Returns
- the IP or url of the server, to which the peer is connected to
§ getIsPayloadEncryptionAvailable()
bool getIsPayloadEncryptionAvailable | ( | void | ) | const |
- Returns
- this peer's payload encryption availability status. True if payload encryption is available, false otherwise.
§ getIsEncryptionAvailable()
bool getIsEncryptionAvailable | ( | void | ) | const |
- Returns
- this peer's encryption availability status. True if either payload encryption is available or if the connection protocol is UDP and UDP encryption is available or if the connection protocol is already secure on its own, false otherwise.
§ getResentReliableCommands()
int getResentReliableCommands | ( | void | ) | const |
- Returns
- the count of commands that got repeated (due to local repeat-timing before an ACK was received).
§ getLimitOfUnreliableCommands()
int getLimitOfUnreliableCommands | ( | void | ) | const |
- Returns
- the limit for the queue of received unreliable commands.
- See also
- setLimitOfUnreliableCommands()
§ setLimitOfUnreliableCommands()
void setLimitOfUnreliableCommands | ( | int | value | ) |
Sets the limit for the queue of received unreliable commands. This works only in UDP. This limit is applied when you call dispatchIncomingCommands. If this client (already) received more than this limit, it will throw away the older ones instead of dispatching them. This can produce bigger gaps for unreliable commands but your client catches up faster. This can be useful when the client couldn't dispatch anything for some time (cause it was in a room but loading a level). If set to 20, the incoming unreliable queues are truncated to 20. If 0, all received unreliable commands will be dispatched. This is a "per channel" value, so each channel can hold commands up to specified limit. This value interacts with dispatchIncomingCommands(): If that is called less often, more commands get skipped.
- See also
- getLimitOfUnreliableCommands()
§ getCRCEnabled()
bool getCRCEnabled | ( | void | ) | const |
- Returns
- true if CRC enabled
- See also
- setCRCEnabled
§ setCRCEnabled()
void setCRCEnabled | ( | bool | crcEnabled | ) |
Enables or disables CRC. While not connected, this controls if the next connection(s) should use a per-package CRC checksum. If the client is in another state than 'connected', then this function has no effect except for logging an error.
While turned on, the client and server will add a CRC checksum to every sent package. The checksum enables both sides to detect and ignore packages that were corrupted during transfer. Corrupted packages have the same impact as lost packages: They require a re-send, adding a delay and could lead to timeouts. Building the checksum has a low processing overhead but increases integrity of sent and received data. Packages discarded due to failed CRC checks are counted in PhotonPeer.PacketLossByCRC.
- Note
- This only has effect for UDP connections.
- This does not have any effect for connections that use UDP datagram encryption (which always use a built-in checksum).
- See also
- getCRCEnabled
§ getPacketLossByCRC()
int getPacketLossByCRC | ( | void | ) | const |
- Returns
- the count of packages dropped due to failed CRC checks for this connection.
- See also
- setCRCEnabled
§ getTrafficStatsEnabled()
bool getTrafficStatsEnabled | ( | void | ) | const |
- Returns
- true if traffic statistics of a peer are enabled. Default trafficStatsEnabled: false (disabled).
§ setTrafficStatsEnabled()
void setTrafficStatsEnabled | ( | bool | trafficStatsEnabled | ) |
Enables or disables the traffic statistics of a peer. Default trafficStatsEnabled: false (disabled).
§ getTrafficStatsElapsedMs()
int getTrafficStatsElapsedMs | ( | void | ) | const |
- Returns
- the count of milliseconds the stats are enabled for tracking.
§ getTrafficStatsIncoming()
const TrafficStats & getTrafficStatsIncoming | ( | void | ) | const |
- Returns
- the byte-count of incoming "low level" messages, which are either Enet Commands or TCP Messages. These include all headers, except those of the underlying internet protocol UDP or TCP.
§ getTrafficStatsOutgoing()
const TrafficStats & getTrafficStatsOutgoing | ( | void | ) | const |
- Returns
- the byte-count of outgoing "low level" messages, which are either Enet Commands or TCP Messages. These include all headers, except those of the underlying internet protocol UDP or TCP.
§ getTrafficStatsGameLevel()
const TrafficStatsGameLevel & getTrafficStatsGameLevel | ( | void | ) | const |
- Returns
- a statistic of incoming and outgoing traffic, split by operation, operation-result and event. Operations are outgoing traffic, results and events are incoming. Includes the per-command header sizes (UDP: Enet Command Header or TCP: Message Header).
§ getQuickResendAttempts()
nByte getQuickResendAttempts | ( | void | ) | const |
- Returns
- the number of resend attempts for a reliable command that are done in quick succession (after RoundTripTime+4*RoundTripTimeVariance).
§ setQuickResendAttempts()
void setQuickResendAttempts | ( | nByte | quickResendAttempts | ) |
Sets the number of resend attempts for a reliable command can be done in quick succession (after RoundTripTime+4*RoundTripTimeVariance).
- Remarks
- The default value is 0. Any later resend attempt will then double the time before the next resend takes place. The max value is 4. Make sure to set SentCountAllowance to a slightly higher value, as more repeats will get done.
§ getConnectionProtocol()
nByte getConnectionProtocol | ( | void | ) | const |
- Returns
- the currently set connection protocol.
- Note
- The value returned is not guaranteed to be the value used for the currently active connection, but only the value that has last been passed to setConnectionProtocol(). The reason therefor is that whatever you pass to setConnectionProtocol() won't take effect until you re-connect.
§ setConnectionProtocol()
void setConnectionProtocol | ( | nByte | connectionProtocol | ) |
Sets the connection protocol to be used with the next connect() call.
- Note
- This does not have any effect on the protocol that is used for an already active connection. So you need to re-connect after setting a different connection protocol for the changes to actually take effect.
§ getChannelCountUserChannels()
nByte getChannelCountUserChannels | ( | void | ) | const |
The IDs from 0 to getChannelCountUserChannels()-1 can be passed as channelID to operations that offer this parameter.
- Returns
- the number of different channels that are available for sending operations on.
§ getPeerCount()
|
static |
- Returns
- the count of peers, which have been initialized since the start of the application. Interesting mainly for debugging purposes.
§ getMaxAppIDLength()
|
static |
- Returns
- the maximum allowed length for the appID that gets passed to connect() in characters