![]() |
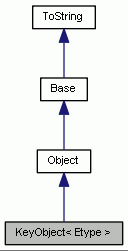
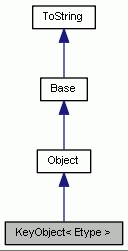
Public Member Functions | |
KeyObject (const KeyObject< Etype > &toCopy) | |
KeyObject (const Object &obj) | |
KeyObject (const Object *obj) | |
KeyObject (const typename Helpers::ConfirmAllowedKey< Etype >::type &data) | |
virtual | ~KeyObject (void) |
virtual KeyObject< Etype > & | operator= (const KeyObject< Etype > &toCopy) |
virtual KeyObject< Etype > & | operator= (const Object &toCopy) |
Etype | getDataCopy (void) const |
Etype * | getDataAddress (void) const |
![]() | |
Object (void) | |
virtual | ~Object (void) |
Object (const Object &toCopy) | |
bool | operator== (const Object &toCompare) const |
bool | operator!= (const Object &toCompare) const |
nByte | getType (void) const |
nByte | getCustomType (void) const |
const short * | getSizes (void) const |
unsigned int | getDimensions (void) const |
JString & | toString (JString &retStr, bool withTypes=false) const |
![]() | |
virtual | ~Base (void) |
![]() | |
virtual | ~ToString (void) |
virtual JString | typeToString (void) const |
JString | toString (bool withTypes=false) const |
Additional Inherited Members | |
![]() | |
static void | setListener (const BaseListener *baseListener) |
static int | getDebugOutputLevel (void) |
static bool | setDebugOutputLevel (int debugLevel) |
static const LogFormatOptions & | getLogFormatOptions (void) |
static void | setLogFormatOptions (const LogFormatOptions &options) |
Detailed Description
template<typename Etype>
class ExitGames::Common::KeyObject< Etype >
Container class template for objects to be stored as keys in a Hashtable or Dictionary.
- Remarks
- In most cases the library will do the work of storing a key in a KeyObject for you, so for example you don't have to explicitly create an instance of this class, when storing a key-value pair in a Dictionary or Hashtable instance. However there are some situations, where you will receive instances of class Object or want to create them (for example Hashtable::getKeys() will return a JVector<Object>) and in that case casting those instances into KeyObject-instances can be a convenient way of assuring a type-safe access to their payloads.
Constructor & Destructor Documentation
§ KeyObject() [1/4]
Copy-Constructor.
Creates an object out of a deep copy of its parameter.
The parameter has to be of the same template overload as the object, you want to create.
- Parameters
-
toCopy The object to copy.
§ KeyObject() [2/4]
Constructor.
Creates an object out of a deep copy of the passed Object&.
If the type of the content of the passed object does not match the template overload of the object to create, an empty object is created instead of a copy of the passed object, which leads to getDataCopy() and getDataAddress() return 0.
- Parameters
-
obj The Object& to copy.
§ KeyObject() [3/4]
Constructor.
Creates an object out of a deep copy of the passed Object*.
If the type of the content of the passed object does not match the template overload of the object to create, an empty object is created instead of a copy of the passed object, which leads to getDataCopy() and getDataAddress() return 0.
- Parameters
-
obj The Object* to copy.
§ KeyObject() [4/4]
KeyObject | ( | const typename Helpers::ConfirmAllowedKey< Etype >::type & | data | ) |
Constructor.
Creates an object out of a deep copy of the passed Etype.
- Parameters
-
data The value to copy. Has to be of a supported type.
§ ~KeyObject()
|
virtual |
Destructor.
Member Function Documentation
§ operator=() [1/2]
operator= : Makes a deep copy of its right operand into its left operand. This overwrites old data in the left operand.
§ operator=() [2/2]
operator= : Makes a deep copy of its right operand into its left operand. This overwrites old data in the left operand.
If the type of the content of the right operand does not match the template overload of the left operand, then the left operand stays unchanged.
Reimplemented from Object.
§ getDataCopy()
Etype getDataCopy | ( | void | ) | const |
Returns a deep copy of the content of the object. If you only need access to the content, while the object still exists, you can use getDataAddress() instead to avoid the deep copy. That is especially interesting for large content, of course.
If successful, the template overloads for array types of this function allocate the data for the copy, so you have to free (for arrays of primitive types) or delete (for arrays of class objects) it, as soon, as you do not need the array anymore. All non-array copies free there memory automatically, as soon as they leave their scope, same as the single indices of the array, as soon, as the array is freed.
In case of an error this function returns 0 for primitive return types and empty objects for classes.
- Returns
- a deep copy of the content of the object if successful, 0 or an empty object otherwise.
§ getDataAddress()
Etype * getDataAddress | ( | void | ) | const |
Returns the address of the original content of the object. If you need access to the data above lifetime of the object, call getDataCopy().
The return type is a pointer to the data, so it is a double-pointer, of course, for template overloads, which data already is a pointer.
In case of an error, this function returns 0.
- Returns
- the address of the original content of the object, if successful, 0 otherwise.