![]() |

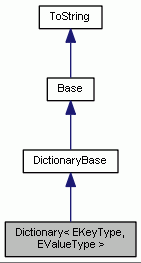
Public Member Functions | |
Dictionary (void) | |
~Dictionary (void) | |
Dictionary (const Dictionary< EKeyType, EValueType > &toCopy) | |
Dictionary & | operator= (const Dictionary< EKeyType, EValueType > &toCopy) |
bool | operator== (const Dictionary< EKeyType, EValueType > &toCompare) const |
bool | operator!= (const Dictionary< EKeyType, EValueType > &toCompare) const |
const EValueType & | operator[] (unsigned int index) const |
EValueType & | operator[] (unsigned int index) |
const nByte * | getKeyTypes (void) const |
const nByte * | getValueTypes (void) const |
const unsigned int * | getValueDimensions (void) const |
void | put (const Dictionary< EKeyType, EValueType > &src) |
void | put (const EKeyType &key, const EValueType &val) |
void | put (const EKeyType &key) |
void | put (const EKeyType &key, const EValueType pVal, typename Common::Helpers::ArrayLengthType< EValueType >::type size) |
void | put (const EKeyType &key, const EValueType pVal, const short *sizes) |
const EValueType * | getValue (const EKeyType &key) const |
JVector< EKeyType > | getKeys (void) const |
void | remove (const EKeyType &key) |
bool | contains (const EKeyType &key) const |
JString | typeToString (void) const |
JString & | toString (JString &retStr, bool withTypes=false) const |
![]() | |
virtual | ~DictionaryBase (void) |
DictionaryBase (const DictionaryBase &toCopy) | |
DictionaryBase & | operator= (const DictionaryBase &toCopy) |
bool | operator== (const DictionaryBase &toCompare) const |
bool | operator!= (const DictionaryBase &toCompare) const |
template<typename FKeyType > | |
void | remove (const FKeyType &key) |
template<typename FKeyType > | |
bool | contains (const FKeyType &key) const |
void | removeAllElements (void) |
JString | typeToString (void) const |
JString & | toString (JString &retStr, bool withTypes=false) const |
const Hashtable & | getHashtable (void) const |
unsigned int | getSize (void) const |
template<typename FKeyType > | |
const short * | getValueSizes (const FKeyType &key) const |
template<typename FKeyType , typename FValueType > | |
const FValueType * | getValue (const FKeyType &key, const FValueType *) const |
template<typename FKeyType > | |
const Object * | getValue (const FKeyType &key, const Object *) const |
template<typename FKeyType > | |
JVector< FKeyType > | getKeys (const FKeyType *) const |
JVector< Object > | getKeys (const Object *) const |
![]() | |
virtual | ~Base (void) |
![]() | |
virtual | ~ToString (void) |
JString | toString (bool withTypes=false) const |
Additional Inherited Members | |
![]() | |
static void | setListener (const BaseListener *baseListener) |
static int | getDebugOutputLevel (void) |
static bool | setDebugOutputLevel (int debugLevel) |
static const LogFormatOptions & | getLogFormatOptions (void) |
static void | setLogFormatOptions (const LogFormatOptions &options) |
Detailed Description
template<typename EKeyType, typename EValueType>
class ExitGames::Common::Dictionary< EKeyType, EValueType >
The Dictionary class template together with the Hashtable class is one of the two main container classes for objects to be transmitted over Photon when using the C++ Client.
This class implements the well-known concept of a container structure storing an arbitrary number of key/value-pairs.
In contrast to a Hashtable, the types of both the keys and also the values in a Dictionary have to be the same for all entries. This takes flexibility, but it also improves type safety and means, that the type infos only have to be stored twice for the whole Dictionary (once for the key and once for the value), while in a Hashtable they have to be stored twice per entry. Therefor with Dictionaries transferring the same amount of key-value pairs will cause less traffic than with Hashtables.
Please have a look at the Table of Datatypes for a list of types, that are supported as keys and as values.
Please refer to the documentation for put() and getValue() to see how to store and access data in a Dictionary.
- See also
- put(), getValue(), KeyObject, ValueObject, Hashtable, DictionaryBase
Constructor & Destructor Documentation
§ Dictionary() [1/2]
Dictionary | ( | void | ) |
Constructor: Creates an empty instance.
§ ~Dictionary()
~Dictionary | ( | void | ) |
Destructor.
§ Dictionary() [2/2]
Dictionary | ( | const Dictionary< EKeyType, EValueType > & | toCopy | ) |
Copy-Constructor: Creates a deep copy of the argument.
- Parameters
-
toCopy The object to copy.
Member Function Documentation
§ operator=()
Dictionary< EKeyType, EValueType > & operator= | ( | const Dictionary< EKeyType, EValueType > & | toCopy | ) |
operator=. Makes a deep copy of its right operand into its left operand. This overwrites old data in the left operand.
§ operator==()
bool operator== | ( | const Dictionary< EKeyType, EValueType > & | toCompare | ) | const |
operator==.
- Returns
- true, if both operands are equal, false otherwise.
Two instances are considered equal if they each hold the same number of entries and, for a given key, the corresponding values equal each other.
Two values are considered equal to each other, if instances of class Object, that are holding them as payloads, equal each other.
- See also
- Object::operator==()
§ operator!=()
bool operator!= | ( | const Dictionary< EKeyType, EValueType > & | toCompare | ) | const |
operator!=.
- Returns
- false, if operator==() would return true, true otherwise.
§ operator[]() [1/2]
const EValueType & operator[] | ( | unsigned int | index | ) | const |
operator[].
Accesses the value at the given index like in an array. This does not check for valid indexes and shows undefined behavior for invalid indexes
§ operator[]() [2/2]
EValueType & operator[] | ( | unsigned int | index | ) |
operator[].
Accesses the value at the given index like in an array. This does not check for valid indexes and shows undefined behavior for invalid indexes
§ getKeyTypes()
|
virtual |
- Returns
- an array, holding the type code for the key type of the Dictionary and type codes for the key types of potential nested Dictionaries.
Only index 0 of the returned array is guaranteed to be valid. The existence of elements at other indices depends on the value of the element in the array returned by getValueTypes() at the previous index in the following way: Only when getValueTypes()[i] == TypeCode::DICTIONARY, then getKeyTypes()[i+1] will be valid.
Type information for nested Dictionaries will be stored like in the following example: Dictionary<int, Dictionary<short, float**>*> This is a Dictionary, with the key type being int and the value type being a 1D array of type Dictionary<short, float**>, so that all values are Dictionaries, which keys are shorts and which values are 2D arrays of float. This function's return value in this example will hold the values TypeCode::INTEGER at index 0 and TypeCode::SHORT at index 1.
The codes returned by this function match the ones, that are stored in member variable "typename" of class template Helpers::ConfirmAllowedKey's specializations. Only the types, for which specializations of that template exist, are valid Dictionary keys.
Reimplemented from DictionaryBase.
§ getValueTypes()
|
virtual |
- Returns
- an array, holding the type code for the value type of the Dictionary and type codes for the value types of potential nested Dictionaries.
Only index 0 of the returned array is guaranteed to be valid. The existence of elements at other indices depends on the value of the element at the previous index in the following way: Only when getValueTypes()[i] == TypeCode::DICTIONARY, then getValueTypes()[i+1] will be valid.
Type information for nested Dictionaries will be stored like in the following example: Dictionary<int, Dictionary<short, float**>*> This is a Dictionary, with the key type being int and the value type being a 1D array of type Dictionary<short, float**>, so that all values are Dictionaries, which keys are shorts and which values are 2D arrays of float. This function's return value in this example will hold the values TypeCode::DICTIONARY at index 0 and TypeCode::FLOAT at index 1.
The codes returned by this function match the ones, that are stored in member variable "typename" of class template Helpers::ConfirmAllowed's specializations. Only the types, for which specializations of that template exist, are valid Dictionary values.
Reimplemented from DictionaryBase.
§ getValueDimensions()
|
virtual |
- Returns
- an array, holding the amount of array dimensions for the value type of the Dictionary and for the value types of potential nested Dictionaries.
Only index 0 of the returned array is guaranteed to be valid. The existence of elements at other indices depends on the value of the element in the array returned by getValueTypes() at the previous index in the following way: Only when getValueTypes()[i] == TypeCode::DICTIONARY, then getValueDimensions()[i+1] will be valid.
Type information for nested Dictionaries will be stored like in the following example: Dictionary<int, Dictionary<short, float**>*> This is a Dictionary, with the key type being int and the value type being a 1D array of type Dictionary<short, float**>, so that all values are Dictionaries, which keys are shorts and which values are 2D arrays of float. This function's return value in this example will hold the value 1 (for 1D array) at index 0 and 2 (for 2D) at index 1. If a value type is no array, then this functions return value will contain 0 at the corresponding index.
Reimplemented from DictionaryBase.
§ put() [1/5]
void put | ( | const Dictionary< EKeyType, EValueType > & | src | ) |
Adds all pairs of a key and a corresponding value from the passed instance to the instance, on which it is called on. If a key is already existing, then its old value will be replaced with the new one.
- Parameters
-
src instance, from which to add the content
- Returns
- nothing.
§ put() [2/5]
void put | ( | const EKeyType & | key, |
const EValueType & | val | ||
) |
Adds a pair of a key and a corresponding value to the instance.
If the key is already existing, then it's old value will be replaced with the new one. Please have a look at the table of datatypes for a list of supported types for keys and values
- Parameters
-
key the key to add val the value to add
- Returns
- nothing.
§ put() [3/5]
void put | ( | const EKeyType & | key | ) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
This overload adds an empty object as value for the provided key.
§ put() [4/5]
void put | ( | const EKeyType & | key, |
const EValueType | pVal, | ||
typename Common::Helpers::ArrayLengthType< EValueType >::type | size | ||
) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
This overload accepts singledimensional arrays and NULL-pointers passed for parameter pVal. NULL pointers are only legal input, if size is 0
- Parameters
-
key the key to add pVal the value array to add size the size of the value array
§ put() [5/5]
void put | ( | const EKeyType & | key, |
const EValueType | pVal, | ||
const short * | sizes | ||
) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
This overload accepts multidimensional arrays and NULL-pointers passed for parameter pVal. The array that is passed for parameter pVal has to be a pointer of the correct abstraction level, meaning a normal pointer for a singledimensional array, a doublepointer for a twodimensional array, a triplepointer for a threedimensional array and so on. For pVal NULL pointers are only legal input, if sizes[0] is 0. For sizes NULL is no valid input.
- Parameters
-
key the key to add pVal the value array to add sizes the sizes for every dimension of the value array - the length of this array has to match the dimensions of pVal
§ getValue()
const EValueType * getValue | ( | const EKeyType & | key | ) | const |
§ getKeys()
JVector< EKeyType > getKeys | ( | void | ) | const |
§ remove()
void remove | ( | const EKeyType & | key | ) |
Deletes the specified key and the corresponding value, if found in the Hashtable.
- Parameters
-
key Pointer to the key of the key/value-pair to remove.
- Returns
- nothing.
- See also
- removeAllElements()
§ contains()
bool contains | ( | const EKeyType & | key | ) | const |
Checks, whether the Hashtable contains a certain key.
- Parameters
-
key Pointer to the key to look up.
- Returns
- true if the specified key was found, false otherwise.
§ typeToString()
|
virtual |
- Remarks
- This function is intended for debugging purposes. For runtime type checking you should use RTTI's typeid() instead. Demangling and cutting off of namespaces will only happen on platforms, which offer a system functionality for demangling.
- Returns
- a string representation of the class name of the polymorphically correct runtime class of the instance, on which it is called on, after this class name has been demangled and eventual namespaces have been removed.
Reimplemented from ToString.
§ toString()
- Remarks
- The cost of this function depends a lot on implementation details of the implementing subclasses, but for container classes this function can become quite expensive, if the instance contains huge amounts of data, as its cost for many container class implementations increases disproportionately high to the size of the payload.
- Parameters
-
retStr reference to a string, to store the return-value in; the information, which is generated by this function, will be attached at the end of any eventually existing previous content of the string withTypes set to true, to include type information in the generated string
- Returns
- a JString representation of the instance and its contents for debugging purposes.
Implements ToString.