Socket APIs
|
wizchip_conf.c
Go to the documentation of this file.
58 //M20150401 : Remove ; in the default callback function such as wizchip_cris_enter(), wizchip_cs_select() and etc.
99 //uint8_t wizchip_bus_readbyte(uint32_t AddrSel) { return * ((volatile uint8_t *)((ptrdiff_t) AddrSel)); }
100 iodata_t wizchip_bus_readdata(uint32_t AddrSel) { return * ((volatile iodata_t *)((ptrdiff_t) AddrSel)); }
108 //void wizchip_bus_writebyte(uint32_t AddrSel, uint8_t wb) { *((volatile uint8_t*)((ptrdiff_t)AddrSel)) = wb; }
109 void wizchip_bus_writedata(uint32_t AddrSel, iodata_t wb) { *((volatile iodata_t*)((ptrdiff_t)AddrSel)) = wb; }
215 //void reg_wizchip_bus_cbfunc(uint8_t(*bus_rb)(uint32_t addr), void (*bus_wb)(uint32_t addr, uint8_t wb))
216 void reg_wizchip_bus_cbfunc(iodata_t(*bus_rb)(uint32_t addr), void (*bus_wb)(uint32_t addr, iodata_t wb))
261 void reg_wizchip_spiburst_cbfunc(void (*spi_rb)(uint8_t* pBuf, uint16_t len), void (*spi_wb)(uint8_t* pBuf, uint16_t len))
netmode_type wizchip_getnetmode(void)
Get the network mode such WOL, PPPoE, Ping Block, and etc.
Definition: wizchip_conf.c:769
void wizchip_gettimeout(wiz_NetTimeout *nettime)
Get retry time value(RTR) and retry count(RCR).
Definition: wizchip_conf.c:780
void wizphy_setphyconf(wiz_PhyConf *phyconf)
Set the phy information for WIZCHIP without power mode.
Definition: wizchip_conf.c:627
union __WIZCHIP::_IF IF
void(* _write_data)(uint32_t AddrSel, iodata_t wb)
Definition: wizchip_conf.h:238
Get network mode as WOL, PPPoE, Ping Block, and Force ARP mode.
Definition: wizchip_conf.h:298
void wizchip_spi_writeburst(uint8_t *pBuf, uint16_t len)
Default function to burst write in SPI interface.
Definition: wizchip_conf.c:141
void wizphy_getphyconf(wiz_PhyConf *phyconf)
Get phy configuration information.
Definition: wizchip_conf.c:657
void wizchip_spi_readburst(uint8_t *pBuf, uint16_t len)
Default function to burst read in SPI interface.
Definition: wizchip_conf.c:133
Get PHY operation mode in internal register. Valid Only W5500.
Definition: wizchip_conf.h:278
void reg_wizchip_bus_cbfunc(iodata_t(*bus_rb)(uint32_t addr), void(*bus_wb)(uint32_t addr, iodata_t wb))
Registers call back function for bus interface.
Definition: wizchip_conf.c:216
#define PHY_MODE_MANUAL
Configured PHY operation mode with user setting.
Definition: wizchip_conf.h:345
Get PHY Power mode as down or normal, Valid Only W5100, W5200.
Definition: wizchip_conf.h:284
Set network mode as WOL, PPPoE, Ping Block, and Force ARP mode.
Definition: wizchip_conf.h:297
void(* _read_burst)(uint8_t *pBuf, uint16_t len)
Definition: wizchip_conf.h:248
void reg_wizchip_cs_cbfunc(void(*cs_sel)(void), void(*cs_desel)(void))
Registers call back function for WIZCHIP select & deselect.
Definition: wizchip_conf.c:200
void wizchip_getnetinfo(wiz_NetInfo *pnetinfo)
Get the network information for WIZCHIP.
Definition: wizchip_conf.c:742
uint8_t wizchip_spi_readbyte(void)
Default function to read in SPI interface.
Definition: wizchip_conf.c:117
struct __WIZCHIP::_IF::@1 SPI
intr_kind wizchip_getinterruptmask(void)
Get Interrupt mask of WIZCHIP.
Definition: wizchip_conf.c:546
int8_t ctlnetwork(ctlnetwork_type cntype, void *arg)
Controls to network.
Definition: wizchip_conf.c:359
struct __WIZCHIP::_CRIS CRIS
#define PHY_MODE_AUTONEGO
Configured PHY operation mode with auto-negotiation.
Definition: wizchip_conf.h:346
Set interval time between the current and next interrupt.
Definition: wizchip_conf.h:271
iodata_t wizchip_bus_readdata(uint32_t AddrSel)
Default function to read in direct or indirect interface.
Definition: wizchip_conf.c:100
int8_t wizphy_getphypmode(void)
get the power mode of PHY in WIZCHIP. No use in W5100
Definition: wizchip_conf.c:596
Force to APP send whenever udp data is sent. Valid only in W5500.
Definition: wizchip_conf.h:406
void reg_wizchip_cris_cbfunc(void(*cris_en)(void), void(*cris_ex)(void))
Registers call back function for critical section of I/O functions such as WIZCHIP_READ, WIZCHIP_WRITE, WIZCHIP_READ_BUF and WIZCHIP_WRITE_BUF.
Definition: wizchip_conf.c:186
Initializes to WIZCHIP with SOCKET buffer size 2 or 1 dimension array typed uint8_t.
Definition: wizchip_conf.h:265
void wizchip_setinterruptmask(intr_kind intr)
Mask or Unmask Interrupt of WIZCHIP.
Definition: wizchip_conf.c:522
The set of callback functions for W5500:WIZCHIP I/O functions W5200:WIZCHIP I/O functions.
Definition: wizchip_conf.h:201
Definition: wizchip_conf.h:417
Set PHY power mode as normal and down when PHYSTATUS.OPMD == 1. Valid Only W5500. ...
Definition: wizchip_conf.h:280
int8_t wizphy_setphypmode(uint8_t pmode)
set the power mode of phy inside WIZCHIP. Refer to PHYCFGR in W5500, PHYSTATUS in W5200 ...
Definition: wizchip_conf.c:703
int8_t wizchip_init(uint8_t *txsize, uint8_t *rxsize)
Initializes WIZCHIP with socket buffer size.
Definition: wizchip_conf.c:412
void wizchip_settimeout(wiz_NetTimeout *nettime)
Set retry time value(RTR) and retry count(RCR).
Definition: wizchip_conf.c:774
#define _WIZCHIP_SOCK_NUM_
The count of independant socket of WIZCHIP.
Definition: wizchip_conf.h:188
void wizchip_setnetinfo(wiz_NetInfo *pnetinfo)
Set the network information for WIZCHIP.
Definition: wizchip_conf.c:729
void(* _write_burst)(uint8_t *pBuf, uint16_t len)
Definition: wizchip_conf.h:249
void wizchip_spi_writebyte(uint8_t wb)
Default function to write in SPI interface.
Definition: wizchip_conf.c:125
Set interval time between the current and next interrupt.
Definition: wizchip_conf.h:270
int8_t ctlwizchip(ctlwizchip_type cwtype, void *arg)
Controls to the WIZCHIP.
Definition: wizchip_conf.c:277
struct __WIZCHIP::_CS CS
int8_t wizchip_setnetmode(netmode_type netmode)
Set the network mode such WOL, PPPoE, Ping Block, and etc.
Definition: wizchip_conf.c:755
Definition: wizchip_conf.h:389
struct __WIZCHIP::_IF::@0 BUS
Definition: wizchip_conf.h:364
void reg_wizchip_spiburst_cbfunc(void(*spi_rb)(uint8_t *pBuf, uint16_t len), void(*spi_wb)(uint8_t *pBuf, uint16_t len))
Registers call back function for SPI interface.
Definition: wizchip_conf.c:261
When PHY configured by internal register, PHY operation mode (Manual/Auto, 10/100, Half/Full). Valid Only W5000.
Definition: wizchip_conf.h:277
void reg_wizchip_spi_cbfunc(uint8_t(*spi_rb)(void), void(*spi_wb)(uint8_t wb))
Registers call back function for SPI interface.
Definition: wizchip_conf.c:244
WIZCHIP Config Header File.
int8_t wizphy_getphylink(void)
get the link status of phy in WIZCHIP. No use in W5100
Definition: wizchip_conf.c:575
void wizchip_bus_writedata(uint32_t AddrSel, iodata_t wb)
Default function to write in direct or indirect interface.
Definition: wizchip_conf.c:109
Generated on Wed May 4 2016 16:43:58 for Socket APIs by
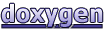