Socket APIs
|
wizchip_conf.h
Go to the documentation of this file.
452 //void reg_wizchip_bus_cbfunc(uint8_t (*bus_rb)(uint32_t addr), void (*bus_wb)(uint32_t addr, uint8_t wb));
453 void reg_wizchip_bus_cbfunc(iodata_t (*bus_rb)(uint32_t addr), void (*bus_wb)(uint32_t addr, iodata_t wb));
473 void reg_wizchip_spiburst_cbfunc(void (*spi_rb)(uint8_t* pBuf, uint16_t len), void (*spi_wb)(uint8_t* pBuf, uint16_t len));
501 * but You can call these functions for code size reduction instead of ctlwizchip() and ctlnetwork().
netmode_type wizchip_getnetmode(void)
Get the network mode such WOL, PPPoE, Ping Block, and etc.
Definition: wizchip_conf.c:769
void wizchip_gettimeout(wiz_NetTimeout *nettime)
Get retry time value(RTR) and retry count(RCR).
Definition: wizchip_conf.c:780
void wizphy_setphyconf(wiz_PhyConf *phyconf)
Set the phy information for WIZCHIP without power mode.
Definition: wizchip_conf.c:627
union __WIZCHIP::_IF IF
Definition: wizchip_conf.h:208
void(* _write_data)(uint32_t AddrSel, iodata_t wb)
Definition: wizchip_conf.h:238
struct __WIZCHIP _WIZCHIP
The set of callback functions for W5500:WIZCHIP I/O functions W5200:WIZCHIP I/O functions.
Get network mode as WOL, PPPoE, Ping Block, and Force ARP mode.
Definition: wizchip_conf.h:298
void wizphy_getphyconf(wiz_PhyConf *phyconf)
Get phy configuration information.
Definition: wizchip_conf.c:657
Get PHY operation mode in internal register. Valid Only W5500.
Definition: wizchip_conf.h:278
W5200 HAL Header File.
Definition: wizchip_conf.h:216
void reg_wizchip_bus_cbfunc(iodata_t(*bus_rb)(uint32_t addr), void(*bus_wb)(uint32_t addr, iodata_t wb))
Registers call back function for bus interface.
Definition: wizchip_conf.c:216
Get PHY Power mode as down or normal, Valid Only W5100, W5200.
Definition: wizchip_conf.h:284
Definition: wizchip_conf.h:224
Set network mode as WOL, PPPoE, Ping Block, and Force ARP mode.
Definition: wizchip_conf.h:297
W5500 HAL Header File.
void(* _read_burst)(uint8_t *pBuf, uint16_t len)
Definition: wizchip_conf.h:248
struct wiz_PhyConf_t wiz_PhyConf
void wizchip_getnetinfo(wiz_NetInfo *pnetinfo)
Get the network information for WIZCHIP.
Definition: wizchip_conf.c:742
int8_t wizphy_getphylink(void)
get the link status of phy in WIZCHIP. No use in W5100
Definition: wizchip_conf.c:575
struct wiz_NetTimeout_t wiz_NetTimeout
struct __WIZCHIP::_IF::@1 SPI
intr_kind wizchip_getinterruptmask(void)
Get Interrupt mask of WIZCHIP.
Definition: wizchip_conf.c:546
int8_t ctlnetwork(ctlnetwork_type cntype, void *arg)
Controls to network.
Definition: wizchip_conf.c:359
struct __WIZCHIP::_CRIS CRIS
Set interval time between the current and next interrupt.
Definition: wizchip_conf.h:271
Force to APP send whenever udp data is sent. Valid only in W5500.
Definition: wizchip_conf.h:406
void reg_wizchip_cs_cbfunc(void(*cs_sel)(void), void(*cs_desel)(void))
Registers call back function for WIZCHIP select & deselect.
Definition: wizchip_conf.c:200
Initializes to WIZCHIP with SOCKET buffer size 2 or 1 dimension array typed uint8_t.
Definition: wizchip_conf.h:265
void wizchip_setinterruptmask(intr_kind intr)
Mask or Unmask Interrupt of WIZCHIP.
Definition: wizchip_conf.c:522
The set of callback functions for W5500:WIZCHIP I/O functions W5200:WIZCHIP I/O functions.
Definition: wizchip_conf.h:201
Definition: wizchip_conf.h:417
Set PHY power mode as normal and down when PHYSTATUS.OPMD == 1. Valid Only W5500. ...
Definition: wizchip_conf.h:280
int8_t wizphy_getphypmode(void)
get the power mode of PHY in WIZCHIP. No use in W5100
Definition: wizchip_conf.c:596
int8_t wizphy_setphypmode(uint8_t pmode)
set the power mode of phy inside WIZCHIP. Refer to PHYCFGR in W5500, PHYSTATUS in W5200 ...
Definition: wizchip_conf.c:703
struct wiz_NetInfo_t wiz_NetInfo
W5100 HAL Header File.
int8_t wizchip_init(uint8_t *txsize, uint8_t *rxsize)
Initializes WIZCHIP with socket buffer size.
Definition: wizchip_conf.c:412
void wizchip_settimeout(wiz_NetTimeout *nettime)
Set retry time value(RTR) and retry count(RCR).
Definition: wizchip_conf.c:774
void wizchip_setnetinfo(wiz_NetInfo *pnetinfo)
Set the network information for WIZCHIP.
Definition: wizchip_conf.c:729
void(* _write_burst)(uint8_t *pBuf, uint16_t len)
Definition: wizchip_conf.h:249
Set interval time between the current and next interrupt.
Definition: wizchip_conf.h:270
int8_t ctlwizchip(ctlwizchip_type cwtype, void *arg)
Controls to the WIZCHIP.
Definition: wizchip_conf.c:277
W5300 HAL implement File.
struct __WIZCHIP::_CS CS
void reg_wizchip_cris_cbfunc(void(*cris_en)(void), void(*cris_ex)(void))
Registers call back function for critical section of I/O functions such as WIZCHIP_READ, WIZCHIP_WRITE, WIZCHIP_READ_BUF and WIZCHIP_WRITE_BUF.
Definition: wizchip_conf.c:186
void reg_wizchip_spiburst_cbfunc(void(*spi_rb)(uint8_t *pBuf, uint16_t len), void(*spi_wb)(uint8_t *pBuf, uint16_t len))
Registers call back function for SPI interface.
Definition: wizchip_conf.c:261
int8_t wizchip_setnetmode(netmode_type netmode)
Set the network mode such WOL, PPPoE, Ping Block, and etc.
Definition: wizchip_conf.c:755
void reg_wizchip_spi_cbfunc(uint8_t(*spi_rb)(void), void(*spi_wb)(uint8_t wb))
Registers call back function for SPI interface.
Definition: wizchip_conf.c:244
Definition: wizchip_conf.h:389
struct __WIZCHIP::_IF::@0 BUS
Definition: wizchip_conf.h:364
When PHY configured by internal register, PHY operation mode (Manual/Auto, 10/100, Half/Full). Valid Only W5000.
Definition: wizchip_conf.h:277
Generated on Wed May 4 2016 16:43:58 for Socket APIs by
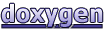