Socket APIs
|
WIZnet socket APIs are based on Berkeley socket APIs, thus it has much similar name and interface. But there is a little bit of difference. More...
Functions | |
int8_t | socket (uint8_t sn, uint8_t protocol, uint16_t port, uint8_t flag) |
Open a socket. More... | |
int8_t | close (uint8_t sn) |
Close a socket. More... | |
int8_t | listen (uint8_t sn) |
Listen to a connection request from a client. More... | |
int8_t | connect (uint8_t sn, uint8_t *addr, uint16_t port) |
Try to connect a server. More... | |
int8_t | disconnect (uint8_t sn) |
Try to disconnect a connection socket. More... | |
int32_t | send (uint8_t sn, uint8_t *buf, uint16_t len) |
Send data to the connected peer in TCP socket. More... | |
int32_t | recv (uint8_t sn, uint8_t *buf, uint16_t len) |
Receive data from the connected peer. More... | |
int32_t | sendto (uint8_t sn, uint8_t *buf, uint16_t len, uint8_t *addr, uint16_t port) |
Sends datagram to the peer with destination IP address and port number passed as parameter. More... | |
int32_t | recvfrom (uint8_t sn, uint8_t *buf, uint16_t len, uint8_t *addr, uint16_t *port) |
Receive datagram of UDP or MACRAW. More... | |
int8_t | ctlsocket (uint8_t sn, ctlsock_type cstype, void *arg) |
Control socket. More... | |
int8_t | setsockopt (uint8_t sn, sockopt_type sotype, void *arg) |
set socket options More... | |
int8_t | getsockopt (uint8_t sn, sockopt_type sotype, void *arg) |
get socket options More... | |
Detailed Description
WIZnet socket APIs are based on Berkeley socket APIs, thus it has much similar name and interface. But there is a little bit of difference.
Comparison between WIZnet and Berkeley SOCKET APIs
API | WIZnet | Berkeley |
socket() | O | O |
bind() | X | O |
listen() | O | O |
connect() | O | O |
accept() | X | O |
recv() | O | O |
send() | O | O |
recvfrom() | O | O |
sendto() | O | O |
closesocket() | O close() & disconnect() | O |
There are bind() and accept() functions in Berkeley SOCKET API but, not in WIZnet SOCKET API. Because socket() of WIZnet is not only creating a SOCKET but also binding a local port number, and listen() of WIZnet is not only listening to connection request from client but also accepting the connection request.
When you program "TCP SERVER" with Berkeley SOCKET API, you can use only one listen port. When the listen SOCKET accepts a connection request from a client, it keeps listening. After accepting the connection request, a new SOCKET is created and the new SOCKET is used in communication with the client.
Following figure shows network flow diagram by Berkeley SOCKET API.
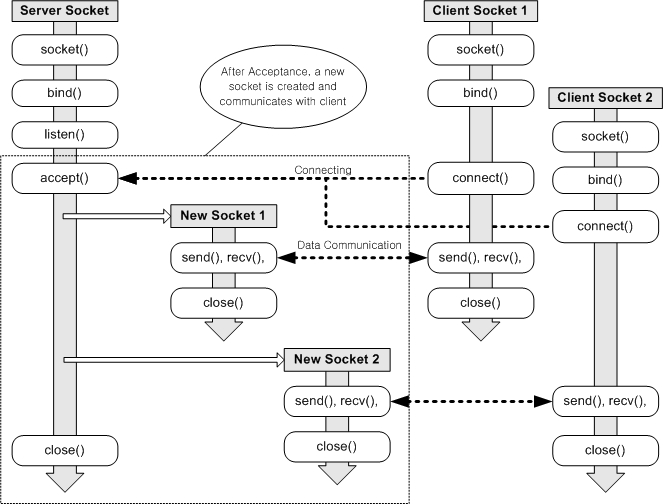
But, When you program "TCP SERVER" with WIZnet SOCKET API, you can use as many as 8 listen SOCKET with same port number.
Because there's no accept() in WIZnet SOCKET APIs, when the listen SOCKET accepts a connection request from a client, it is changed in order to communicate with the client. And the changed SOCKET is not listening any more and is dedicated for communicating with the client.
If there're many listen SOCKET with same listen port number and a client requests a connection, the SOCKET which has the smallest SOCKET number accepts the request and is changed as communication SOCKET.
Following figure shows network flow diagram by WIZnet SOCKET API.
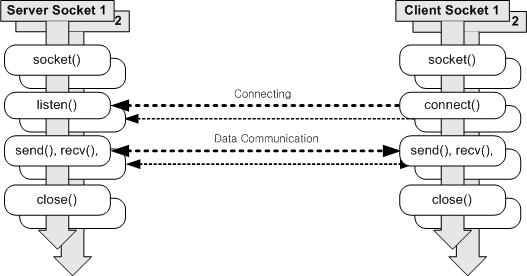
Function Documentation
int8_t socket | ( | uint8_t | sn, |
uint8_t | protocol, | ||
uint16_t | port, | ||
uint8_t | flag | ||
) |
Open a socket.
Initializes the socket with 'sn' passed as parameter and open.
- Parameters
-
sn Socket number. It should be 0 ~ _WIZCHIP_SOCK_NUM_. protocol Protocol type to operate such as TCP, UDP and MACRAW. port Port number to be bined. flag Socket flags as SF_ETHER_OWN, SF_IGMP_VER2, SF_TCP_NODELAY, SF_MULTI_ENABLE, SF_IO_NONBLOCK and so on.
Valid flags only in W5500 : SF_BROAD_BLOCK, SF_MULTI_BLOCK, SF_IPv6_BLOCK, and SF_UNI_BLOCK.
- See also
- Sn_MR
- Returns
- Success : The socket number 'sn' passed as parameter
Fail :
SOCKERR_SOCKNUM - Invalid socket number
SOCKERR_SOCKMODE - Not support socket mode as TCP, UDP, and so on.
SOCKERR_SOCKFLAG - Invaild socket flag.
Definition at line 105 of file socket.c.
References CHECK_SOCKNUM, close(), getSIPR, getSn_CR, getSn_SR, PACK_COMPLETED, setSn_CR, setSn_MR, setSn_PORT, SF_IGMP_VER2, SF_IO_NONBLOCK, SF_MULTI_ENABLE, SF_TCP_NODELAY, SF_UNI_BLOCK, Sn_CR_OPEN, Sn_MR_IPRAW, Sn_MR_MACRAW, Sn_MR_PPPoE, Sn_MR_TCP, Sn_MR_UDP, SOCK_ANY_PORT_NUM, SOCK_CLOSED, sock_pack_info, SOCKERR_SOCKFLAG, SOCKERR_SOCKINIT, and SOCKERR_SOCKMODE.
int8_t close | ( | uint8_t | sn | ) |
Close a socket.
It closes the socket with 'sn' passed as parameter.
- Parameters
-
sn Socket number. It should be 0 ~ _WIZCHIP_SOCK_NUM_.
- Returns
- Success : SOCK_OK
Fail : SOCKERR_SOCKNUM - Invalid socket number
Definition at line 197 of file socket.c.
References CHECK_SOCKNUM, getSn_CR, getSn_MR, getSn_SR, getSn_TX_FSR(), getSn_TxMAX, sendto(), setSn_CR, setSn_IR, setSn_MR, setSn_PORTR, Sn_CR_CLOSE, Sn_CR_OPEN, Sn_MR_TCP, Sn_MR_UDP, SOCK_CLOSED, SOCK_OK, sock_pack_info, and SOCK_UDP.
Referenced by disconnect(), listen(), recv(), recvfrom(), send(), and socket().
int8_t listen | ( | uint8_t | sn | ) |
Listen to a connection request from a client.
It is listening to a connection request from a client. If connection request is accepted successfully, the connection is established. Socket sn is used in passive(server) mode.
- Parameters
-
sn Socket number. It should be 0 ~ _WIZCHIP_SOCK_NUM_.
- Returns
- Success : SOCK_OK
Fail :
SOCKERR_SOCKINIT - Socket is not initialized
SOCKERR_SOCKCLOSED - Socket closed unexpectedly.
Definition at line 240 of file socket.c.
References CHECK_SOCKINIT, CHECK_SOCKMODE, CHECK_SOCKNUM, close(), getSn_CR, getSn_SR, setSn_CR, Sn_CR_LISTEN, Sn_MR_TCP, SOCK_CLOSED, SOCK_LISTEN, SOCK_OK, and SOCKERR_SOCKCLOSED.
int8_t connect | ( | uint8_t | sn, |
uint8_t * | addr, | ||
uint16_t | port | ||
) |
Try to connect a server.
It requests connection to the server with destination IP address and port number passed as parameter.
- Note
- It is valid only in TCP client mode. In block io mode, it does not return until connection is completed. In Non-block io mode, it return SOCK_BUSY immediately.
- Parameters
-
sn Socket number. It should be 0 ~ _WIZCHIP_SOCK_NUM_. addr Pointer variable of destination IP address. It should be allocated 4 bytes. port Destination port number.
- Returns
- Success : SOCK_OK
Fail :
SOCKERR_SOCKNUM - Invalid socket number
SOCKERR_SOCKMODE - Invalid socket mode
SOCKERR_SOCKINIT - Socket is not initialized
SOCKERR_IPINVALID - Wrong server IP address
SOCKERR_PORTZERO - Server port zero
SOCKERR_TIMEOUT - Timeout occurred during request connection
SOCK_BUSY - In non-block io mode, it returned immediately
Definition at line 259 of file socket.c.
References CHECK_SOCKINIT, CHECK_SOCKMODE, CHECK_SOCKNUM, getSn_CR, getSn_IR, getSn_SR, setSn_CR, setSn_DIPR, setSn_DPORT, setSn_IR, Sn_CR_CONNECT, Sn_IR_TIMEOUT, Sn_MR_TCP, SOCK_BUSY, SOCK_CLOSED, SOCK_ESTABLISHED, SOCK_OK, SOCKERR_IPINVALID, SOCKERR_PORTZERO, SOCKERR_SOCKCLOSED, and SOCKERR_TIMEOUT.
int8_t disconnect | ( | uint8_t | sn | ) |
Try to disconnect a connection socket.
It sends request message to disconnect the TCP socket 'sn' passed as parameter to the server or client.
- Note
- It is valid only in TCP server or client mode.
In block io mode, it does not return until disconnection is completed.
In Non-block io mode, it return SOCK_BUSY immediately.
- Parameters
-
sn Socket number. It should be 0 ~ _WIZCHIP_SOCK_NUM_.
- Returns
- Success : SOCK_OK
Fail :
SOCKERR_SOCKNUM - Invalid socket number
SOCKERR_SOCKMODE - Invalid operation in the socket
SOCKERR_TIMEOUT - Timeout occurred
SOCK_BUSY - Socket is busy.
Definition at line 299 of file socket.c.
References CHECK_SOCKMODE, CHECK_SOCKNUM, close(), getSn_CR, getSn_IR, getSn_SR, setSn_CR, Sn_CR_DISCON, Sn_IR_TIMEOUT, Sn_MR_TCP, SOCK_BUSY, SOCK_CLOSED, SOCK_OK, and SOCKERR_TIMEOUT.
int32_t send | ( | uint8_t | sn, |
uint8_t * | buf, | ||
uint16_t | len | ||
) |
Send data to the connected peer in TCP socket.
It is used to send outgoing data to the connected socket.
- Note
- It is valid only in TCP server or client mode. It can't send data greater than socket buffer size.
In block io mode, It doesn't return until data send is completed - socket buffer size is greater than data.
In non-block io mode, It return SOCK_BUSY immediately when socket buffer is not enough.
- Parameters
-
sn Socket number. It should be 0 ~ _WIZCHIP_SOCK_NUM_. buf Pointer buffer containing data to be sent. len The byte length of data in buf.
- Returns
- Success : The sent data size
Fail :
SOCKERR_SOCKSTATUS - Invalid socket status for socket operation
SOCKERR_TIMEOUT - Timeout occurred
SOCKERR_SOCKMODE - Invalid operation in the socket
SOCKERR_SOCKNUM - Invalid socket number
SOCKERR_DATALEN - zero data length
SOCK_BUSY - Socket is busy.
Definition at line 319 of file socket.c.
References CHECK_SOCKDATA, CHECK_SOCKMODE, CHECK_SOCKNUM, close(), getSn_CR, getSn_IR, getSn_SR, getSn_TX_FSR(), getSn_TX_RD, getSn_TxMAX, setSn_CR, setSn_IR, setSn_TX_WRSR, Sn_CR_SEND, Sn_IR_SENDOK, Sn_IR_TIMEOUT, Sn_MR_TCP, SOCK_BUSY, SOCK_CLOSE_WAIT, SOCK_ESTABLISHED, SOCKERR_SOCKSTATUS, SOCKERR_TIMEOUT, and wiz_send_data().
int32_t recv | ( | uint8_t | sn, |
uint8_t * | buf, | ||
uint16_t | len | ||
) |
Receive data from the connected peer.
It is used to read incoming data from the connected socket.
It waits for data as much as the application wants to receive.
- Note
- It is valid only in TCP server or client mode. It can't receive data greater than socket buffer size.
In block io mode, it doesn't return until data reception is completed - data is filled as len in socket buffer.
In non-block io mode, it return SOCK_BUSY immediately when len is greater than data size in socket buffer.
- Parameters
-
sn Socket number. It should be 0 ~ _WIZCHIP_SOCK_NUM_. buf Pointer buffer to read incoming data. len The max data length of data in buf.
- Returns
- Success : The real received data size
Fail :
SOCKERR_SOCKSTATUS - Invalid socket status for socket operation
SOCKERR_SOCKMODE - Invalid operation in the socket
SOCKERR_SOCKNUM - Invalid socket number
SOCKERR_DATALEN - zero data length
SOCK_BUSY - Socket is busy.
Definition at line 387 of file socket.c.
References CHECK_SOCKDATA, CHECK_SOCKMODE, CHECK_SOCKNUM, close(), getMR, getSn_CR, getSn_MR, getSn_RX_RSR(), getSn_RxMAX, getSn_SR, getSn_TX_FSR(), getSn_TxMAX, MR_FS, PACK_COMPLETED, PACK_FIFOBYTE, PACK_FIRST, PACK_REMAINED, setSn_CR, Sn_CR_RECV, Sn_MR_ALIGN, Sn_MR_TCP, SOCK_BUSY, SOCK_CLOSE_WAIT, SOCK_ESTABLISHED, sock_pack_info, SOCKERR_SOCKSTATUS, and wiz_recv_data().
int32_t sendto | ( | uint8_t | sn, |
uint8_t * | buf, | ||
uint16_t | len, | ||
uint8_t * | addr, | ||
uint16_t | port | ||
) |
Sends datagram to the peer with destination IP address and port number passed as parameter.
It sends datagram of UDP or MACRAW to the peer with destination IP address and port number passed as parameter.
Even if the connectionless socket has been previously connected to a specific address, the address and port number parameters override the destination address for that particular datagram only.
- Note
- In block io mode, It doesn't return until data send is completed - socket buffer size is greater than len. In non-block io mode, It return SOCK_BUSY immediately when socket buffer is not enough.
- Parameters
-
sn Socket number. It should be 0 ~ _WIZCHIP_SOCK_NUM_. buf Pointer buffer to send outgoing data. len The byte length of data in buf. addr Pointer variable of destination IP address. It should be allocated 4 bytes. port Destination port number.
- Returns
- Success : The sent data size
Fail :
SOCKERR_SOCKNUM - Invalid socket number
SOCKERR_SOCKMODE - Invalid operation in the socket
SOCKERR_SOCKSTATUS - Invalid socket status for socket operation
SOCKERR_DATALEN - zero data length
SOCKERR_IPINVALID - Wrong server IP address
SOCKERR_PORTZERO - Server port zero
SOCKERR_SOCKCLOSED - Socket unexpectedly closed
SOCKERR_TIMEOUT - Timeout occurred
SOCK_BUSY - Socket is busy.
Definition at line 492 of file socket.c.
References CHECK_SOCKDATA, CHECK_SOCKNUM, getSIPR, getSn_CR, getSn_IR, getSn_MR, getSn_SR, getSn_TX_FSR(), getSn_TxMAX, getSUBR, setSn_CR, setSn_DIPR, setSn_DPORT, setSn_IR, setSn_TX_WRSR, setSUBR, Sn_CR_SEND, Sn_IR_SENDOK, Sn_IR_TIMEOUT, Sn_MR_MACRAW, Sn_MR_UDP, SOCK_BUSY, SOCK_CLOSED, SOCK_MACRAW, SOCK_UDP, SOCKERR_IPINVALID, SOCKERR_PORTZERO, SOCKERR_SOCKCLOSED, SOCKERR_SOCKMODE, SOCKERR_SOCKSTATUS, SOCKERR_TIMEOUT, and wiz_send_data().
Referenced by close().
int32_t recvfrom | ( | uint8_t | sn, |
uint8_t * | buf, | ||
uint16_t | len, | ||
uint8_t * | addr, | ||
uint16_t * | port | ||
) |
Receive datagram of UDP or MACRAW.
This function is an application I/F function which is used to receive the data in other then TCP mode.
This function is used to receive UDP and MAC_RAW mode, and handle the header as well. This function can divide to received the packet data. On the MACRAW SOCKET, the addr and port parameters are ignored.
- Note
- In block io mode, it doesn't return until data reception is completed - data is filled as len in socket buffer In non-block io mode, it return SOCK_BUSY immediately when len is greater than data size in socket buffer.
- Parameters
-
sn Socket number. It should be 0 ~ _WIZCHIP_SOCK_NUM_. buf Pointer buffer to read incoming data. len The max data length of data in buf. When the received packet size <= len, receives data as packet sized. When others, receives data as len. addr Pointer variable of destination IP address. It should be allocated 4 bytes. It is valid only when the first call recvfrom for receiving the packet. When it is valid, packinfo[7] should be set as '1' after call getsockopt(sn, SO_PACKINFO, &packinfo). port Pointer variable of destination port number. It is valid only when the first call recvform for receiving the packet. When it is valid, packinfo[7] should be set as '1' after call getsockopt(sn, SO_PACKINFO, &packinfo).
- Returns
- Success : This function return real received data size for success.
Fail : SOCKERR_DATALEN - zero data length
SOCKERR_SOCKMODE - Invalid operation in the socket
SOCKERR_SOCKNUM - Invalid socket number
SOCKBUSY - Socket is busy.
Definition at line 588 of file socket.c.
References CHECK_SOCKDATA, CHECK_SOCKNUM, close(), getMR, getSn_CR, getSn_MR, getSn_RX_RSR(), getSn_SR, MR_FS, PACK_COMPLETED, PACK_FIFOBYTE, PACK_FIRST, PACK_REMAINED, setSn_CR, Sn_CR_RECV, Sn_MR_IPRAW, Sn_MR_MACRAW, Sn_MR_PPPoE, Sn_MR_UDP, SOCK_BUSY, SOCK_CLOSED, sock_pack_info, SOCKERR_SOCKCLOSED, SOCKERR_SOCKMODE, SOCKFATAL_PACKLEN, wiz_recv_data(), and wiz_recv_ignore().
int8_t ctlsocket | ( | uint8_t | sn, |
ctlsock_type | cstype, | ||
void * | arg | ||
) |
Control socket.
Control IO mode, Interrupt & Mask of socket and get the socket buffer information. Refer to ctlsock_type.
- Parameters
-
sn socket number cstype type of control socket. refer to ctlsock_type. arg Data type and value is determined according to ctlsock_type.
cstype data type value CS_SET_IOMODE
CS_GET_IOMODEuint8_t SOCK_IO_BLOCK SOCK_IO_NONBLOCK CS_GET_MAXTXBUF
CS_GET_MAXRXBUFuint16_t 0 ~ 16K CS_CLR_INTERRUPT
CS_GET_INTERRUPT
CS_SET_INTMASK
CS_GET_INTMASKsockint_kind SIK_CONNECTED, etc.
- Returns
- Success SOCK_OK
fail SOCKERR_ARG - Invalid argument
Definition at line 764 of file socket.c.
References CHECK_SOCKNUM, CS_CLR_INTERRUPT, CS_GET_INTERRUPT, CS_GET_INTMASK, CS_GET_IOMODE, CS_GET_MAXRXBUF, CS_GET_MAXTXBUF, CS_SET_INTMASK, CS_SET_IOMODE, getSn_IMR, getSn_IR, getSn_RxMAX, getSn_TxMAX, setSn_IMR, setSn_IR, SIK_ALL, SOCK_IO_BLOCK, SOCK_IO_NONBLOCK, SOCK_OK, and SOCKERR_ARG.
int8_t setsockopt | ( | uint8_t | sn, |
sockopt_type | sotype, | ||
void * | arg | ||
) |
set socket options
Set socket option like as TTL, MSS, TOS, and so on. Refer to sockopt_type.
- Parameters
-
sn socket number sotype socket option type. refer to sockopt_type arg Data type and value is determined according to sotype.
sotype data type value SO_TTL uint8_t 0 ~ 255 SO_TOS uint8_t 0 ~ 255 SO_MSS uint16_t 0 ~ 65535 SO_DESTIP uint8_t[4] SO_DESTPORT uint16_t 0 ~ 65535 SO_KEEPALIVESEND null null SO_KEEPALIVEAUTO uint8_t 0 ~ 255
- Returns
- Success : SOCK_OK
- Fail
- SOCKERR_SOCKNUM - Invalid Socket number
- SOCKERR_SOCKMODE - Invalid socket mode
- SOCKERR_SOCKOPT - Invalid socket option or its value
- SOCKERR_TIMEOUT - Timeout occurred when sending keep-alive packet
- SOCKERR_SOCKNUM - Invalid Socket number
- Success : SOCK_OK
Definition at line 810 of file socket.c.
References CHECK_SOCKMODE, CHECK_SOCKNUM, getSn_CR, getSn_IR, getSn_KPALVTR, setSn_CR, setSn_DIPR, setSn_DPORT, setSn_IR, setSn_KPALVTR, setSn_MSSR, setSn_TOS, setSn_TTL, Sn_CR_SEND_KEEP, Sn_IR_TIMEOUT, Sn_MR_TCP, SO_DESTIP, SO_DESTPORT, SO_KEEPALIVEAUTO, SO_KEEPALIVESEND, SO_MSS, SO_TOS, SO_TTL, SOCK_OK, SOCKERR_ARG, SOCKERR_SOCKOPT, and SOCKERR_TIMEOUT.
int8_t getsockopt | ( | uint8_t | sn, |
sockopt_type | sotype, | ||
void * | arg | ||
) |
get socket options
Get socket option like as FLAG, TTL, MSS, and so on. Refer to sockopt_type
- Parameters
-
sn socket number sotype socket option type. refer to sockopt_type arg Data type and value is determined according to sotype.
sotype data type value SO_FLAG uint8_t SF_ETHER_OWN, etc... SO_TOS uint8_t 0 ~ 255 SO_MSS uint16_t 0 ~ 65535 SO_DESTIP uint8_t[4] SO_DESTPORT uint16_t SO_KEEPALIVEAUTO uint8_t 0 ~ 255 SO_SENDBUF uint16_t 0 ~ 65535 SO_RECVBUF uint16_t 0 ~ 65535 SO_STATUS uint8_t SOCK_ESTABLISHED, etc.. SO_REMAINSIZE uint16_t 0~ 65535 SO_PACKINFO uint8_t PACK_FIRST, etc...
- Returns
- Success : SOCK_OK
- Fail
- SOCKERR_SOCKNUM - Invalid Socket number
- SOCKERR_SOCKOPT - Invalid socket option or its value
- SOCKERR_SOCKMODE - Invalid socket mode
- SOCKERR_SOCKNUM - Invalid Socket number
- Success : SOCK_OK
- Note
- The option as PACK_REMAINED and SO_PACKINFO is valid only in NON-TCP mode and after call recvfrom().
When SO_PACKINFO value is PACK_FIRST and the return value of recvfrom() is zero, This means the zero byte UDP data(UDP Header only) received.
Definition at line 863 of file socket.c.
References CHECK_SOCKMODE, CHECK_SOCKNUM, getSn_DIPR, getSn_DPORT, getSn_KPALVTR, getSn_MR, getSn_MSSR, getSn_RX_RSR(), getSn_SR, getSn_TOS, getSn_TTL, getSn_TX_FSR(), Sn_MR_TCP, SO_DESTIP, SO_DESTPORT, SO_FLAG, SO_KEEPALIVEAUTO, SO_MSS, SO_PACKINFO, SO_RECVBUF, SO_REMAINSIZE, SO_SENDBUF, SO_STATUS, SO_TOS, SO_TTL, SOCK_OK, sock_pack_info, and SOCKERR_SOCKOPT.
Generated on Wed May 4 2016 16:43:59 for Socket APIs by
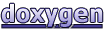